Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / ComIntegration / MonikerBuilder.cs / 1 / MonikerBuilder.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.ComIntegration { using System; using System.Reflection; using System.Collections.Generic; using System.Threading; using System.ServiceModel.Channels; using System.Runtime.Remoting.Proxies; using System.Runtime.Remoting; using System.Runtime.Remoting.Services; using System.Runtime.InteropServices; internal class MonikerBuilder : IProxyCreator { ComProxy comProxy = null; private MonikerBuilder () { } void IDisposable.Dispose () { } ComProxy IProxyCreator.CreateProxy (IntPtr outer, ref Guid riid) { if ((riid != typeof (IMoniker).GUID) && (riid != typeof (IParseDisplayName).GUID)) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidCastException(SR.GetString(SR.NoInterface, riid))); if (outer == IntPtr.Zero) { DiagnosticUtility.DebugAssert("OuterProxy cannot be null"); throw DiagnosticUtility.ExceptionUtility.ThrowHelperInternal(false); } if (comProxy == null) { ServiceMonikerInternal moniker = null; try { moniker = new ServiceMonikerInternal (); comProxy = ComProxy.Create (outer, moniker, moniker); return comProxy; } finally { if ((comProxy == null) && (moniker != null)) ((IDisposable)moniker).Dispose (); } } else return comProxy.Clone (); } bool IProxyCreator.SupportsErrorInfo (ref Guid riid) { if ((riid != typeof (IMoniker).GUID) && (riid != typeof (IParseDisplayName).GUID)) return false; else return true; } bool IProxyCreator.SupportsDispatch () { return false; } bool IProxyCreator.SupportsIntrinsics () { return false; } public static MarshalByRefObject CreateMonikerInstance () { IProxyCreator serviceChannelBuilder = new MonikerBuilder ();; IProxyManager proxyManager = new ProxyManager (serviceChannelBuilder); Guid iid = typeof (IMoniker).GUID; IntPtr ppv = OuterProxyWrapper.CreateOuterProxyInstance (proxyManager, ref iid); MarshalByRefObject ret = EnterpriseServicesHelper.WrapIUnknownWithComObject (ppv) as MarshalByRefObject; Marshal.Release(ppv); return ret; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
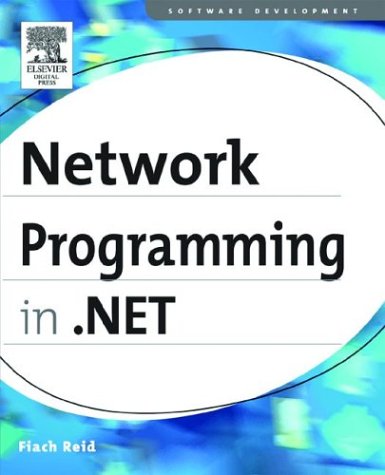
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Switch.cs
- DataBoundLiteralControl.cs
- FontUnitConverter.cs
- EncodingTable.cs
- HostingEnvironmentException.cs
- ExportException.cs
- ToolTipAutomationPeer.cs
- WebException.cs
- _AutoWebProxyScriptWrapper.cs
- ParameterModifier.cs
- QuotaThrottle.cs
- SeekStoryboard.cs
- CodeCompileUnit.cs
- WindowsPen.cs
- Panel.cs
- PieceDirectory.cs
- KeyValueInternalCollection.cs
- WebEncodingValidatorAttribute.cs
- ErrorFormatter.cs
- StructuralComparisons.cs
- FontStyles.cs
- ChineseLunisolarCalendar.cs
- CollectionViewProxy.cs
- SQLInt16Storage.cs
- XmlSchemaAttributeGroupRef.cs
- LoginName.cs
- UserPreferenceChangedEventArgs.cs
- WebPartMenu.cs
- SoapSchemaImporter.cs
- CodeDirectoryCompiler.cs
- PropertyToken.cs
- Comparer.cs
- Bits.cs
- Int32AnimationBase.cs
- FixedStringLookup.cs
- BinaryQueryOperator.cs
- SQLSingle.cs
- DataSourceControl.cs
- CancellableEnumerable.cs
- EventMappingSettings.cs
- ErrorFormatter.cs
- XmlSerializerVersionAttribute.cs
- Win32KeyboardDevice.cs
- SplineQuaternionKeyFrame.cs
- RoleManagerSection.cs
- DataViewManagerListItemTypeDescriptor.cs
- ButtonField.cs
- EntitySqlQueryState.cs
- PolyBezierSegment.cs
- BufferedWebEventProvider.cs
- PasswordBoxAutomationPeer.cs
- Converter.cs
- Object.cs
- ConfigurationErrorsException.cs
- MouseWheelEventArgs.cs
- BaseTemplatedMobileComponentEditor.cs
- DeferredElementTreeState.cs
- ToolStripContentPanel.cs
- SectionInput.cs
- ObjectStateEntryDbDataRecord.cs
- DirectoryNotFoundException.cs
- TextDecorations.cs
- CompilationUtil.cs
- XmlnsCompatibleWithAttribute.cs
- BaseCollection.cs
- RepeaterItemEventArgs.cs
- ServerValidateEventArgs.cs
- CultureInfoConverter.cs
- TargetControlTypeCache.cs
- DocumentGridPage.cs
- IdentityHolder.cs
- FileUpload.cs
- ContextMenuService.cs
- OrderedDictionaryStateHelper.cs
- IpcServerChannel.cs
- Int16.cs
- XmlSchemaCollection.cs
- EntityWithChangeTrackerStrategy.cs
- UnaryQueryOperator.cs
- ComponentConverter.cs
- ToolStripPanelSelectionBehavior.cs
- ping.cs
- NativeRecognizer.cs
- CanonicalXml.cs
- PowerStatus.cs
- CheckableControlBaseAdapter.cs
- FormsAuthenticationTicket.cs
- DataServiceRequestOfT.cs
- ClientOptions.cs
- AutoResizedEvent.cs
- DataGridViewCellCollection.cs
- CmsInterop.cs
- WorkflowViewElement.cs
- TextBoxRenderer.cs
- TextFindEngine.cs
- WebCategoryAttribute.cs
- RowToParametersTransformer.cs
- ObjectNotFoundException.cs
- ColorComboBox.cs
- DesignerValidationSummaryAdapter.cs