Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Net / System / Net / WebException.cs / 1 / WebException.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net { using System; using System.Runtime.Serialization; using System.Security.Permissions; /*++ Abstract: Contains the defintion for the WebException object. This is a subclass of Exception that contains a WebExceptionStatus and possible a reference to a WebResponse. --*/ ////// [Serializable] public class WebException : InvalidOperationException, ISerializable { private WebExceptionStatus m_Status = WebExceptionStatus.UnknownError; //Should be changed to GeneralFailure; private WebResponse m_Response; [NonSerialized] private WebExceptionInternalStatus m_InternalStatus = WebExceptionInternalStatus.RequestFatal; ////// Provides network communication exceptions to the application. /// /// This is the exception that is thrown by WebRequests when something untoward /// happens. It's a subclass of WebException that contains a WebExceptionStatus and possibly /// a reference to a WebResponse. The WebResponse is only present if we actually /// have a response from the remote server. /// ////// public WebException() { } ////// Creates a new instance of the ////// class with the default status /// from the /// values. /// /// public WebException(string message) : this(message, null) { } ////// Creates a new instance of the ///class with the specified error /// message. /// /// public WebException(string message, Exception innerException) : base(message, innerException) { } public WebException(string message, WebExceptionStatus status) : this(message, null, status, null) { } ////// Creates a new instance of the ///class with the specified error /// message and nested exception. /// /// Message - Message string for exception. /// InnerException - Exception that caused this exception. /// /// /// internal WebException(string message, WebExceptionStatus status, WebExceptionInternalStatus internalStatus, Exception innerException) : this(message, innerException, status, null, internalStatus) { } ////// Creates a new instance of the ///class with the specified error /// message and status. /// /// Message - Message string for exception. /// Status - Network status of exception /// /// public WebException(string message, Exception innerException, WebExceptionStatus status, WebResponse response) : this(message, null, innerException, status, response) { } internal WebException(string message, string data, Exception innerException, WebExceptionStatus status, WebResponse response) : base(message + (data != null ? ": '" + data + "'" : ""), innerException) { m_Status = status; m_Response = response; } internal WebException(string message, Exception innerException, WebExceptionStatus status, WebResponse response, WebExceptionInternalStatus internalStatus) : this(message, null, innerException, status, response, internalStatus) { } internal WebException(string message, string data, Exception innerException, WebExceptionStatus status, WebResponse response, WebExceptionInternalStatus internalStatus) : base(message + (data != null ? ": '" + data + "'" : ""), innerException) { m_Status = status; m_Response = response; m_InternalStatus = internalStatus; } protected WebException(SerializationInfo serializationInfo, StreamingContext streamingContext) : base(serializationInfo, streamingContext) { // m_Status = (WebExceptionStatus)serializationInfo.GetInt32("Status"); // m_InternalStatus = (WebExceptionInternalStatus)serializationInfo.GetInt32("InternalStatus"); } ////// Creates a new instance of the ///class with the specified error /// message, nested exception, status and response. /// /// Message - Message string for exception. /// InnerException - The exception that caused this one. /// Status - Network status of exception /// Response - The WebResponse we have. /// [SecurityPermission(SecurityAction.LinkDemand, Flags = SecurityPermissionFlag.SerializationFormatter)] void ISerializable.GetObjectData(SerializationInfo serializationInfo, StreamingContext streamingContext) { GetObjectData(serializationInfo, streamingContext); } [SecurityPermissionAttribute(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.SerializationFormatter)] public override void GetObjectData(SerializationInfo serializationInfo, StreamingContext streamingContext){ base.GetObjectData(serializationInfo, streamingContext); //serializationInfo.AddValue("Status", (int)m_Status, typeof(int)); //serializationInfo.AddValue("InternalStatus", (int)m_InternalStatus, typeof(int)); } /// /// public WebExceptionStatus Status { get { return m_Status; } } ////// Gets the status of the response. /// ////// public WebResponse Response { get { return m_Response; } } ////// Gets the error message returned from the remote host. /// ////// internal WebExceptionInternalStatus InternalStatus { get { return m_InternalStatus; } } }; // class WebException internal enum WebExceptionInternalStatus { RequestFatal = 0, ServicePointFatal = 1, Recoverable = 2, Isolated = 3, } } // namespace System.Net/// Gets the error message returned from the remote host. /// ///
Link Menu
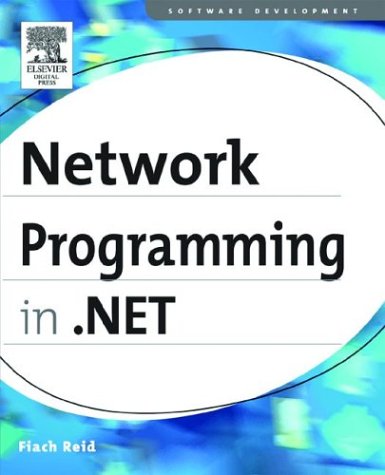
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataListItem.cs
- ExceptQueryOperator.cs
- PropertySegmentSerializer.cs
- StandardToolWindows.cs
- ListViewGroup.cs
- XmlCountingReader.cs
- EventEntry.cs
- CatalogPartChrome.cs
- ToolStripStatusLabel.cs
- ArrangedElement.cs
- SafeFindHandle.cs
- TabPanel.cs
- Slider.cs
- TableParaClient.cs
- HybridObjectCache.cs
- GraphicsState.cs
- Rijndael.cs
- DefaultAuthorizationContext.cs
- ProfileSettings.cs
- StylusEditingBehavior.cs
- ImageClickEventArgs.cs
- Int32CAMarshaler.cs
- CngUIPolicy.cs
- MutableAssemblyCacheEntry.cs
- ListChangedEventArgs.cs
- DispatcherFrame.cs
- AsymmetricSignatureFormatter.cs
- StringConcat.cs
- RoleServiceManager.cs
- ToolStripRendererSwitcher.cs
- ResXBuildProvider.cs
- SerializationObjectManager.cs
- DbReferenceCollection.cs
- Empty.cs
- SqlReorderer.cs
- StringComparer.cs
- StateManagedCollection.cs
- ProfileServiceManager.cs
- RegexBoyerMoore.cs
- SymbolMethod.cs
- TextViewBase.cs
- IResourceProvider.cs
- odbcmetadatafactory.cs
- SafeHandles.cs
- CustomCategoryAttribute.cs
- BCryptHashAlgorithm.cs
- SectionXmlInfo.cs
- ConfigurationErrorsException.cs
- ListCollectionView.cs
- TransactionCache.cs
- PropertyInformation.cs
- XmlExtensionFunction.cs
- GenerateTemporaryTargetAssembly.cs
- XmlnsCache.cs
- JsonReaderWriterFactory.cs
- DiscoveryProxy.cs
- TransformerTypeCollection.cs
- DBSchemaRow.cs
- FrameworkElementAutomationPeer.cs
- SqlPersonalizationProvider.cs
- WebPartUtil.cs
- _NetRes.cs
- TypeDescriptor.cs
- Message.cs
- HealthMonitoringSection.cs
- DateTimeConstantAttribute.cs
- LinkedResource.cs
- MDIWindowDialog.cs
- ListParagraph.cs
- SerializationSectionGroup.cs
- SmtpTransport.cs
- SoapReflector.cs
- HtmlEmptyTagControlBuilder.cs
- SecurityRuntime.cs
- columnmapfactory.cs
- CodeIdentifier.cs
- TypePropertyEditor.cs
- PropertyConverter.cs
- MetadataItem.cs
- ProfileService.cs
- Int32EqualityComparer.cs
- ApplicationInfo.cs
- MembershipSection.cs
- DrawingGroupDrawingContext.cs
- SkewTransform.cs
- IndexedEnumerable.cs
- BinaryExpression.cs
- DataStreams.cs
- StorageInfo.cs
- DefaultParameterValueAttribute.cs
- SoapConverter.cs
- Polygon.cs
- RelationshipEndMember.cs
- ProxyWebPartConnectionCollection.cs
- IndexerNameAttribute.cs
- ILGenerator.cs
- TableCellCollection.cs
- ComboBoxItem.cs
- PaintValueEventArgs.cs
- TextEditorThreadLocalStore.cs