Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / Input / TextComposition.cs / 1 / TextComposition.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: TextComposition class is the object that contains // the input text. The text from keyboard input // is packed in this class when TextInput event is generated. // And this class also packs the state of the composition text when // the input text is being composed (for EA input, Speech). // // History: // 11/18/2003 : [....] created // //--------------------------------------------------------------------------- using System; using System.ComponentModel; using System.Diagnostics; using System.Text; using System.Windows.Threading; using System.Windows; using System.Security; using System.Security.Permissions; using MS.Win32; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Input { //----------------------------------------------------- // // TextCompositionAutoComplete enum // //----------------------------------------------------- ////// The switch for automatic termination of the text composition /// public enum TextCompositionAutoComplete { ////// AutomaticComplete is off. /// Off = 0, ////// AutomaticComplete is on. /// TextInput event will be generated automatically by TextCompositionManager after /// TextInputStart event is processed. /// On = 1, } internal enum TextCompositionStage { ////// The composition is not started yet. /// None = 0, ////// The composition has started. /// Started = 1, ////// The composition has completed or canceled. /// Done = 2, } ////// Text Composition class contains the result text of the text input and the state of the composition text. /// public class TextComposition : DispatcherObject { //------------------------------------------------------ // // Constructors // //----------------------------------------------------- #region Constructors ////// The constrcutor of TextComposition class. /// public TextComposition(InputManager inputManager, IInputElement source, string resultText) : this(inputManager, source, resultText, TextCompositionAutoComplete.On) { } ////// The constrcutor of TextComposition class. /// ////// Critical: This code peeks Into InputManager.Current /// PublicOK: It does not expose the InputManager and keyboard device is safe to expose /// [SecurityCritical] public TextComposition(InputManager inputManager, IInputElement source, string resultText, TextCompositionAutoComplete autoComplete) : this(inputManager, source, resultText, autoComplete, InputManager.Current.PrimaryKeyboardDevice) { // We should avoid using Enum.IsDefined for performance and correct versioning. if ((autoComplete != TextCompositionAutoComplete.Off) && (autoComplete != TextCompositionAutoComplete.On)) { throw new InvalidEnumArgumentException("TextCompositionAutoComplete", (int)autoComplete, typeof(TextCompositionAutoComplete)); } } // // An internal constructore to specify InputDevice directly. // ////// Critical - stores critical data ( _inputManager). /// TreatAsSafe - inputmanager is stored in a non-public critical member. Usage of InputManager is tracked. /// [SecurityCritical, SecurityTreatAsSafe ] internal TextComposition(InputManager inputManager, IInputElement source, string resultText, TextCompositionAutoComplete autoComplete, InputDevice inputDevice) { _inputManager = inputManager; _inputDevice = inputDevice; if (resultText == null) { throw new ArgumentException(SR.Get(SRID.TextComposition_NullResultText)); } _resultText = resultText; _compositionText = ""; _systemText = ""; _systemCompositionText = ""; _controlText = ""; _autoComplete = autoComplete; _stage = TextCompositionStage.None; // source of this text composition. _source = source; } #endregion Constructors //------------------------------------------------------ // // Public Methods // //------------------------------------------------------ ////// Finalize the composition. /// ////// Callers must have UIPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical: Calls into CompleteComposition /// PublicOk: This operation is blocked from external consumers via a link demand /// [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] public virtual void Complete() { // VerifyAccess(); TextCompositionManager.CompleteComposition(this); } //----------------------------------------------------- // // Public Properties // //------------------------------------------------------ ////// The result text of the text input. /// [CLSCompliant(false)] public string Text { get { // VerifyAccess(); return _resultText; } protected set { // VerifyAccess(); _resultText = value; } } ////// The current composition text. /// [CLSCompliant(false)] public string CompositionText { get { // VerifyAccess(); return _compositionText; } protected set { // VerifyAccess(); _compositionText = value; } } ////// The current system text. /// [CLSCompliant(false)] public string SystemText { get { // VerifyAccess(); return _systemText; } protected set { // VerifyAccess(); _systemText = value; } } ////// The current system text. /// [CLSCompliant(false)] public string ControlText { get { // VerifyAccess(); return _controlText; } protected set { // VerifyAccess(); _controlText = value; } } ////// The current system text. /// [CLSCompliant(false)] public string SystemCompositionText { get { // VerifyAccess(); return _systemCompositionText; } protected set { // VerifyAccess(); _systemCompositionText = value; } } ////// The switch for automatic termination. /// public TextCompositionAutoComplete AutoComplete { get { // VerifyAccess(); return _autoComplete; } } //----------------------------------------------------- // // Public Events // //----------------------------------------------------- //----------------------------------------------------- // // Protected Methods // //------------------------------------------------------ //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ ////// The current composition text. /// internal void SetText(string resultText) { _resultText = resultText; } ////// The current composition text. /// internal void SetCompositionText(string compositionText) { _compositionText = compositionText; } ////// Convert this composition to system composition. /// internal void MakeSystem() { _systemText = _resultText; _systemCompositionText = _compositionText; _resultText = ""; _compositionText = ""; _controlText = ""; } ////// Convert this composition to system composition. /// internal void MakeControl() { // Onlt control char should be in _controlText. Debug.Assert((_resultText.Length == 1) && Char.IsControl(_resultText[0])); _controlText = _resultText; _resultText = ""; _systemText = ""; _compositionText = ""; _systemCompositionText = ""; } ////// Clear all the current texts. /// internal void ClearTexts() { _resultText = ""; _compositionText = ""; _systemText = ""; _systemCompositionText = ""; _controlText = ""; } //------------------------------------------------------ // // Internal Properties // //----------------------------------------------------- ////// The source of this text composition. /// internal IInputElement Source { get { return _source; } } // return the input device for this text composition. internal InputDevice _InputDevice { get {return _inputDevice;} } // return the input manager for this text composition. ////// Gives out critical data. /// internal InputManager _InputManager { [SecurityCritical] get { return _inputManager; } } // the stage of this text composition internal TextCompositionStage Stage { get {return _stage;} set {_stage = value;} } //------------------------------------------------------ // // Internal Events // //----------------------------------------------------- //----------------------------------------------------- // // Private Methods // //----------------------------------------------------- //------------------------------------------------------ // // Private Properties // //----------------------------------------------------- //------------------------------------------------------ // // Private Fields // //------------------------------------------------------ // InputManager for this TextComposition. ////// Critical data. InputManager ctor is critical. /// [SecurityCritical] private readonly InputManager _inputManager; // InputDevice for this TextComposition. private readonly InputDevice _inputDevice; // The finalized and result string. private string _resultText; // The composition string. private string _compositionText; // The system string. private string _systemText; // The control string. private string _controlText; // The system composition string. private string _systemCompositionText; // If this is true, TextComposition Manager will terminate the compositon automatically. private readonly TextCompositionAutoComplete _autoComplete; // TextComposition stage. private TextCompositionStage _stage; // source of this text composition. private IInputElement _source; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
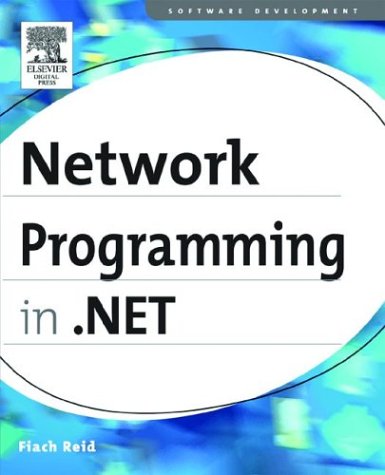
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- InlinedAggregationOperatorEnumerator.cs
- HealthMonitoringSection.cs
- ToolStripPanelCell.cs
- AccessDataSourceView.cs
- ValueChangedEventManager.cs
- Region.cs
- DataGridViewControlCollection.cs
- LostFocusEventManager.cs
- EncryptedType.cs
- SqlProfileProvider.cs
- DbConnectionStringBuilder.cs
- XmlSchemaDatatype.cs
- ComplexBindingPropertiesAttribute.cs
- DataSourceHelper.cs
- TreeNodeEventArgs.cs
- DynamicValidator.cs
- SecurityUtils.cs
- bidPrivateBase.cs
- TabItemWrapperAutomationPeer.cs
- OutOfMemoryException.cs
- SchemaLookupTable.cs
- WebSysDescriptionAttribute.cs
- BreakSafeBase.cs
- AppDomainProtocolHandler.cs
- ForwardPositionQuery.cs
- PauseStoryboard.cs
- PointLight.cs
- TextEditorCharacters.cs
- PrivilegedConfigurationManager.cs
- CommonObjectSecurity.cs
- HttpResponse.cs
- SymLanguageVendor.cs
- ParseChildrenAsPropertiesAttribute.cs
- UIElementPropertyUndoUnit.cs
- GrammarBuilderRuleRef.cs
- SqlCommand.cs
- OleDbMetaDataFactory.cs
- InvalidCastException.cs
- BoundsDrawingContextWalker.cs
- ChannelDispatcher.cs
- GifBitmapEncoder.cs
- UdpChannelListener.cs
- relpropertyhelper.cs
- TextParentUndoUnit.cs
- XmlUnspecifiedAttribute.cs
- SoapIgnoreAttribute.cs
- XmlAttributeCache.cs
- UIntPtr.cs
- SkipStoryboardToFill.cs
- XPathNodeInfoAtom.cs
- OneOfConst.cs
- BamlMapTable.cs
- WebControlsSection.cs
- UIPermission.cs
- InstallerTypeAttribute.cs
- PbrsForward.cs
- SrgsRulesCollection.cs
- ApplicationDirectoryMembershipCondition.cs
- HyperLinkColumn.cs
- KeyInterop.cs
- InstanceView.cs
- ListViewInsertionMark.cs
- QueryCacheManager.cs
- ObjectHandle.cs
- IndexingContentUnit.cs
- DependencyPropertyValueSerializer.cs
- CanExecuteRoutedEventArgs.cs
- EntitySqlQueryBuilder.cs
- SoapTransportImporter.cs
- WebRequestModuleElement.cs
- ResourcePart.cs
- DataBindingCollection.cs
- RubberbandSelector.cs
- SourceFileInfo.cs
- UndoEngine.cs
- Material.cs
- FormsAuthentication.cs
- NamespaceQuery.cs
- XmlSchemaSequence.cs
- Menu.cs
- PriorityChain.cs
- WinInetCache.cs
- _emptywebproxy.cs
- CompilerTypeWithParams.cs
- Certificate.cs
- CryptoProvider.cs
- CodeGotoStatement.cs
- EqualityComparer.cs
- initElementDictionary.cs
- SafeMemoryMappedViewHandle.cs
- ModulesEntry.cs
- TransactionChannelFactory.cs
- SystemIdentity.cs
- XPathNodeList.cs
- SortAction.cs
- ToolstripProfessionalRenderer.cs
- ColumnHeaderConverter.cs
- PixelFormatConverter.cs
- SessionStateUtil.cs
- BookmarkInfo.cs