Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Documents / TextParentUndoUnit.cs / 1305600 / TextParentUndoUnit.cs
//---------------------------------------------------------------------------- //--------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // // See spec at http://avalon/uis/Stock%20Services/Undo%20spec.htm // // History: // 03/23/2004 : eveselov - created // //--------------------------------------------------------------------------- using System; using MS.Internal; using System.Windows.Input; using System.Windows.Controls; using System.Windows.Documents.Internal; using System.Windows.Threading; using System.ComponentModel; using System.Windows.Media; using System.Windows.Markup; using System.Text; using MS.Utility; using MS.Internal.Documents; namespace System.Windows.Documents { ////// TextParentUndoUnit /// internal class TextParentUndoUnit : ParentUndoUnit { //----------------------------------------------------- // // Constructors // //------------------------------------------------------ #region Constructors ////// Constructor /// /// /// TextSelection before executing the operation. /// internal TextParentUndoUnit(ITextSelection selection) : this(selection, selection.AnchorPosition, selection.MovingPosition) { } internal TextParentUndoUnit(ITextSelection selection, ITextPointer anchorPosition, ITextPointer movingPosition) : base(String.Empty) { _selection = selection; _undoAnchorPositionOffset = anchorPosition.Offset; _undoAnchorPositionDirection = anchorPosition.LogicalDirection; _undoMovingPositionOffset = movingPosition.Offset; _undoMovingPositionDirection = movingPosition.LogicalDirection; // Bug 1706768: we are seeing unitialized values when the undo // undo is pulled off the undo stack. _redoAnchorPositionOffset // and _redoMovingPositionOffset are supposed to be initialized // with calls to RecordRedoSelectionState before that happens. // // This code path is being left enabled in DEBUG to help track down // the underlying bug post V1. #if DEBUG _redoAnchorPositionOffset = -1; _redoMovingPositionOffset = -1; #else _redoAnchorPositionOffset = 0; _redoMovingPositionOffset = 0; #endif } ////// Creates a redo unit from an undo unit. /// protected TextParentUndoUnit(TextParentUndoUnit undoUnit) : base(String.Empty) { _selection = undoUnit._selection; _undoAnchorPositionOffset = undoUnit._redoAnchorPositionOffset; _undoAnchorPositionDirection = undoUnit._redoAnchorPositionDirection; _undoMovingPositionOffset = undoUnit._redoMovingPositionOffset; _undoMovingPositionDirection = undoUnit._redoMovingPositionDirection; // Bug 1706768: we are seeing unitialized values when the undo // undo is pulled off the undo stack. _redoAnchorPositionOffset // and _redoMovingPositionOffset are supposed to be initialized // with calls to RecordRedoSelectionState before that happens. // // This code path is being left enabled in DEBUG to help track down // the underlying bug post V1. #if DEBUG _redoAnchorPositionOffset = -1; _redoMovingPositionOffset = -1; #else _redoAnchorPositionOffset = 0; _redoMovingPositionOffset = 0; #endif } #endregion Constructors //----------------------------------------------------- // // Public Methods // //------------------------------------------------------ #region Public Methods ////// Implements IUndoUnit::Do(). For IParentUndoUnit, this means iterating through /// all contained units and calling their Do(). /// public override void Do() { base.Do(); // Note: TextParentUndoUnit will be created here by our callback CreateParentUndoUnitForSelf. ITextContainer textContainer = _selection.Start.TextContainer; ITextPointer anchorPosition = textContainer.CreatePointerAtOffset(_undoAnchorPositionOffset, _undoAnchorPositionDirection); ITextPointer movingPosition = textContainer.CreatePointerAtOffset(_undoMovingPositionOffset, _undoMovingPositionDirection); _selection.Select(anchorPosition, movingPosition); _redoUnit.RecordRedoSelectionState(); } #endregion Public Methods //------------------------------------------------------ // // Protected Methods // //----------------------------------------------------- #region Protected Methods ////// Implements a callback called from base.Do method for /// creating appropriate ParentUndoUnit for redo. /// ///protected override IParentUndoUnit CreateParentUndoUnitForSelf() { _redoUnit = CreateRedoUnit(); return _redoUnit; } protected virtual TextParentUndoUnit CreateRedoUnit() { return new TextParentUndoUnit(this); } protected void MergeRedoSelectionState(TextParentUndoUnit undoUnit) { _redoAnchorPositionOffset = undoUnit._redoAnchorPositionOffset; _redoAnchorPositionDirection = undoUnit._redoAnchorPositionDirection; _redoMovingPositionOffset = undoUnit._redoMovingPositionOffset; _redoMovingPositionDirection = undoUnit._redoMovingPositionDirection; } #endregion Protected Methods //------------------------------------------------------ // // Internal Methods // //----------------------------------------------------- #region Internal Methods /// /// This method should be called just before the undo unit is closed. It will capture /// the current selectionStart and selectionEnd offsets for use later when this undo unit /// gets Redone. /// internal void RecordRedoSelectionState() { RecordRedoSelectionState(_selection.AnchorPosition, _selection.MovingPosition); } ////// This method should be called just before the undo unit is closed. It will capture /// the current selectionStart and selectionEnd offsets for use later when this undo unit /// gets Redone. /// internal void RecordRedoSelectionState(ITextPointer anchorPosition, ITextPointer movingPosition) { _redoAnchorPositionOffset = anchorPosition.Offset; _redoAnchorPositionDirection = anchorPosition.LogicalDirection; _redoMovingPositionOffset = movingPosition.Offset; _redoMovingPositionDirection = movingPosition.LogicalDirection; } #endregion Internal Methods //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields private readonly ITextSelection _selection; private readonly int _undoAnchorPositionOffset; private readonly LogicalDirection _undoAnchorPositionDirection; private readonly int _undoMovingPositionOffset; private readonly LogicalDirection _undoMovingPositionDirection; private int _redoAnchorPositionOffset; private LogicalDirection _redoAnchorPositionDirection; private int _redoMovingPositionOffset; private LogicalDirection _redoMovingPositionDirection; private TextParentUndoUnit _redoUnit; #if DEBUG // Debug-only unique identifier for this instance. private readonly int _debugId = _debugIdCounter++; // Debug-only id counter. private static int _debugIdCounter; #endif #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
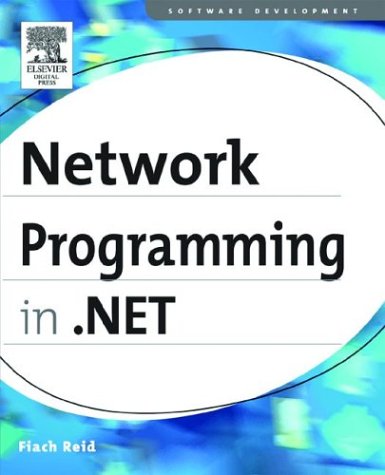
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Simplifier.cs
- TypeValidationEventArgs.cs
- GroupQuery.cs
- AttachedPropertyMethodSelector.cs
- SoapHeaders.cs
- AdapterUtil.cs
- Collection.cs
- CheckBoxPopupAdapter.cs
- WSFederationHttpSecurityMode.cs
- DiagnosticTraceSchemas.cs
- TableColumnCollection.cs
- StickyNoteAnnotations.cs
- PatternMatcher.cs
- WebServiceEnumData.cs
- AnnotationStore.cs
- ReadOnlyCollection.cs
- VectorCollectionConverter.cs
- DataGridTextBox.cs
- DataRowExtensions.cs
- BindingContext.cs
- xmlfixedPageInfo.cs
- TrustSection.cs
- EventProviderWriter.cs
- StackOverflowException.cs
- StickyNoteAnnotations.cs
- InputScope.cs
- BoundPropertyEntry.cs
- AmbiguousMatchException.cs
- NodeFunctions.cs
- MemberProjectionIndex.cs
- OutputBuffer.cs
- UnaryExpression.cs
- StreamAsIStream.cs
- ServiceContractGenerationContext.cs
- TouchFrameEventArgs.cs
- rsa.cs
- WebServiceReceive.cs
- SymLanguageVendor.cs
- TreeNodeConverter.cs
- HttpBufferlessInputStream.cs
- FrugalList.cs
- Scripts.cs
- GeneralTransform2DTo3D.cs
- XPathAxisIterator.cs
- LongTypeConverter.cs
- MgmtConfigurationRecord.cs
- HttpWebRequestElement.cs
- Regex.cs
- ScriptResourceInfo.cs
- HtmlAnchor.cs
- DragEventArgs.cs
- printdlgexmarshaler.cs
- ScriptManagerProxy.cs
- RequestCacheManager.cs
- FormatVersion.cs
- BlockCollection.cs
- SiblingIterators.cs
- OAVariantLib.cs
- TimeZone.cs
- RefreshPropertiesAttribute.cs
- ListViewUpdateEventArgs.cs
- PageSetupDialog.cs
- RepeatInfo.cs
- Console.cs
- baseaxisquery.cs
- ContextMarshalException.cs
- ContextMenuStrip.cs
- EncryptedXml.cs
- HtmlTableRow.cs
- ModifierKeysValueSerializer.cs
- RequestTimeoutManager.cs
- CssTextWriter.cs
- sapiproxy.cs
- CodeGeneratorOptions.cs
- ToolStripPanelRenderEventArgs.cs
- StateFinalizationDesigner.cs
- ContextMenuService.cs
- CompilerScope.Storage.cs
- ClientOperationFormatterProvider.cs
- PropertyMapper.cs
- WmlPanelAdapter.cs
- PropertyTabAttribute.cs
- StaticSiteMapProvider.cs
- SqlDataRecord.cs
- XhtmlBasicPanelAdapter.cs
- MemberAccessException.cs
- HttpContext.cs
- Delegate.cs
- figurelengthconverter.cs
- TriggerActionCollection.cs
- GradientStop.cs
- GeometryGroup.cs
- InitializerFacet.cs
- Messages.cs
- ListBoxItemAutomationPeer.cs
- ThreadInterruptedException.cs
- XpsFixedPageReaderWriter.cs
- FileUpload.cs
- Transform.cs
- DbDataReader.cs