Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / Abstractions / HttpPostedFileWrapper.cs / 1305376 / HttpPostedFileWrapper.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web { using System; using System.IO; using System.Runtime.CompilerServices; [TypeForwardedFrom("System.Web.Abstractions, Version=3.5.0.0, Culture=Neutral, PublicKeyToken=31bf3856ad364e35")] public class HttpPostedFileWrapper : HttpPostedFileBase { private HttpPostedFile _file; public HttpPostedFileWrapper(HttpPostedFile httpPostedFile) { if (httpPostedFile == null) { throw new ArgumentNullException("httpPostedFile"); } _file = httpPostedFile; } public override int ContentLength { get { return _file.ContentLength; } } public override string ContentType { get { return _file.ContentType; } } public override string FileName { get { return _file.FileName; } } public override Stream InputStream { get { return _file.InputStream; } } public override void SaveAs(string filename) { _file.SaveAs(filename); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web { using System; using System.IO; using System.Runtime.CompilerServices; [TypeForwardedFrom("System.Web.Abstractions, Version=3.5.0.0, Culture=Neutral, PublicKeyToken=31bf3856ad364e35")] public class HttpPostedFileWrapper : HttpPostedFileBase { private HttpPostedFile _file; public HttpPostedFileWrapper(HttpPostedFile httpPostedFile) { if (httpPostedFile == null) { throw new ArgumentNullException("httpPostedFile"); } _file = httpPostedFile; } public override int ContentLength { get { return _file.ContentLength; } } public override string ContentType { get { return _file.ContentType; } } public override string FileName { get { return _file.FileName; } } public override Stream InputStream { get { return _file.InputStream; } } public override void SaveAs(string filename) { _file.SaveAs(filename); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
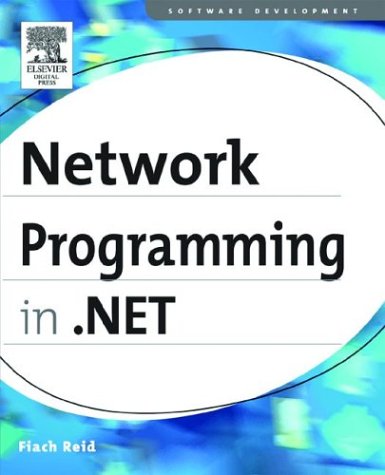
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SchemaImporterExtension.cs
- FontNamesConverter.cs
- DeferredElementTreeState.cs
- XmlSchemaSequence.cs
- MatrixTransform3D.cs
- PersonalizableTypeEntry.cs
- XsdValidatingReader.cs
- ValidatedMobileControlConverter.cs
- OdbcHandle.cs
- ManagementObjectSearcher.cs
- DataMemberFieldConverter.cs
- SaveFileDialog.cs
- WebControl.cs
- Normalization.cs
- NameValuePermission.cs
- HttpRawResponse.cs
- StylusCollection.cs
- ClientSideProviderDescription.cs
- SessionStateUtil.cs
- OleDbRowUpdatedEvent.cs
- TextSelectionHelper.cs
- CompilerParameters.cs
- ListItemsPage.cs
- WebServiceTypeData.cs
- Calendar.cs
- ObjectManager.cs
- QueryStringParameter.cs
- CqlLexerHelpers.cs
- BindableTemplateBuilder.cs
- ImageButton.cs
- InkPresenter.cs
- HttpException.cs
- QueryAccessibilityHelpEvent.cs
- ObjectConverter.cs
- PreviewKeyDownEventArgs.cs
- UriExt.cs
- LoginName.cs
- CaseInsensitiveComparer.cs
- Content.cs
- ListViewUpdateEventArgs.cs
- SafeNativeMethodsOther.cs
- BrowserInteropHelper.cs
- IndividualDeviceConfig.cs
- XmlSecureResolver.cs
- AsnEncodedData.cs
- TextTabProperties.cs
- CellPartitioner.cs
- TreeNodeMouseHoverEvent.cs
- MemberDomainMap.cs
- DBSqlParser.cs
- RawTextInputReport.cs
- SmtpSpecifiedPickupDirectoryElement.cs
- basevalidator.cs
- DefaultBinder.cs
- CipherData.cs
- RadioButtonRenderer.cs
- DataServiceKeyAttribute.cs
- ScopelessEnumAttribute.cs
- CodeComment.cs
- Attributes.cs
- ClientProtocol.cs
- EntityDataSourceStatementEditorForm.cs
- AsymmetricSignatureDeformatter.cs
- ExternalCalls.cs
- LazyTextWriterCreator.cs
- MouseEvent.cs
- JsonGlobals.cs
- SchemaMapping.cs
- WebServiceErrorEvent.cs
- CompositionTarget.cs
- AssociatedControlConverter.cs
- EnumUnknown.cs
- EventSinkActivity.cs
- EtwTrace.cs
- TemplateField.cs
- Matrix.cs
- ApplicationBuildProvider.cs
- PropertyGridView.cs
- ObjectItemLoadingSessionData.cs
- DeploymentSectionCache.cs
- ProgressiveCrcCalculatingStream.cs
- ILGenerator.cs
- SimpleMailWebEventProvider.cs
- ObjectStateEntryOriginalDbUpdatableDataRecord.cs
- DataGridParentRows.cs
- RowUpdatingEventArgs.cs
- WebExceptionStatus.cs
- BamlMapTable.cs
- FormClosedEvent.cs
- ExeConfigurationFileMap.cs
- Journaling.cs
- KeyValuePair.cs
- IncrementalCompileAnalyzer.cs
- Unit.cs
- invalidudtexception.cs
- SmuggledIUnknown.cs
- ConfigXmlReader.cs
- BitmapMetadataBlob.cs
- ThicknessConverter.cs
- PagerSettings.cs