Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Base / MS / Internal / IO / Packaging / DeflateEmulationStream.cs / 1 / DeflateEmulationStream.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // Implementation of a helper class that provides a fully functional Stream on a restricted functionality // Compression stream (System.IO.Compression.DeflateStream). // // History: // 10/05/2005: BruceMac: Split out from CompressEmulationStream //----------------------------------------------------------------------------- using System; using System.IO; using System.IO.Compression; // for DeflateStream using System.Diagnostics; using System.IO.Packaging; using System.Windows; namespace MS.Internal.IO.Packaging { //----------------------------------------------------- // // Internal Members // //----------------------------------------------------- ////// Emulates a fully functional stream using restricted functionality DeflateStream /// internal class DeflateEmulationTransform : IDeflateTransform { ////// Extract from DeflateStream to temp stream /// ///Caller is responsible for correctly positioning source and sink stream pointers before calling. public void Decompress(Stream source, Stream sink) { // for non-empty stream create deflate stream that can // actually decompress using (DeflateStream deflateStream = new DeflateStream( source, // source of compressed data CompressionMode.Decompress, // compress or decompress true)) // leave base stream open when the deflate stream is closed { int bytesRead = 0; do { bytesRead = deflateStream.Read(Buffer, 0, Buffer.Length); if (bytesRead > 0) sink.Write(Buffer, 0, bytesRead); } while (bytesRead > 0); } } ////// Compress from the temp stream into the base stream /// ///Caller is responsible for correctly positioning source and sink stream pointers before calling. public void Compress(Stream source, Stream sink) { // create deflate stream that can actually compress or decompress using (DeflateStream deflateStream = new DeflateStream( sink, // destination for compressed data CompressionMode.Compress, // compress or decompress true)) // leave base stream open when the deflate stream is closed { // persist to deflated stream from working stream int bytesRead = 0; do { bytesRead = source.Read(Buffer, 0, Buffer.Length); if (bytesRead > 0) deflateStream.Write(Buffer, 0, bytesRead); } while (bytesRead > 0); } // truncate if necessary and possible if (sink.CanSeek) sink.SetLength(sink.Position); } //------------------------------------------------------ // // Private Properties // //----------------------------------------------------- private byte[] Buffer { get { if (_buffer == null) _buffer = new byte[0x1000]; // 4k return _buffer; } } //------------------------------------------------------ // // Private Members // //------------------------------------------------------ private byte[] _buffer; // alloc and re-use to reduce memory fragmentation // this is safe because we are not thread-safe } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // Implementation of a helper class that provides a fully functional Stream on a restricted functionality // Compression stream (System.IO.Compression.DeflateStream). // // History: // 10/05/2005: BruceMac: Split out from CompressEmulationStream //----------------------------------------------------------------------------- using System; using System.IO; using System.IO.Compression; // for DeflateStream using System.Diagnostics; using System.IO.Packaging; using System.Windows; namespace MS.Internal.IO.Packaging { //----------------------------------------------------- // // Internal Members // //----------------------------------------------------- ////// Emulates a fully functional stream using restricted functionality DeflateStream /// internal class DeflateEmulationTransform : IDeflateTransform { ////// Extract from DeflateStream to temp stream /// ///Caller is responsible for correctly positioning source and sink stream pointers before calling. public void Decompress(Stream source, Stream sink) { // for non-empty stream create deflate stream that can // actually decompress using (DeflateStream deflateStream = new DeflateStream( source, // source of compressed data CompressionMode.Decompress, // compress or decompress true)) // leave base stream open when the deflate stream is closed { int bytesRead = 0; do { bytesRead = deflateStream.Read(Buffer, 0, Buffer.Length); if (bytesRead > 0) sink.Write(Buffer, 0, bytesRead); } while (bytesRead > 0); } } ////// Compress from the temp stream into the base stream /// ///Caller is responsible for correctly positioning source and sink stream pointers before calling. public void Compress(Stream source, Stream sink) { // create deflate stream that can actually compress or decompress using (DeflateStream deflateStream = new DeflateStream( sink, // destination for compressed data CompressionMode.Compress, // compress or decompress true)) // leave base stream open when the deflate stream is closed { // persist to deflated stream from working stream int bytesRead = 0; do { bytesRead = source.Read(Buffer, 0, Buffer.Length); if (bytesRead > 0) deflateStream.Write(Buffer, 0, bytesRead); } while (bytesRead > 0); } // truncate if necessary and possible if (sink.CanSeek) sink.SetLength(sink.Position); } //------------------------------------------------------ // // Private Properties // //----------------------------------------------------- private byte[] Buffer { get { if (_buffer == null) _buffer = new byte[0x1000]; // 4k return _buffer; } } //------------------------------------------------------ // // Private Members // //------------------------------------------------------ private byte[] _buffer; // alloc and re-use to reduce memory fragmentation // this is safe because we are not thread-safe } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
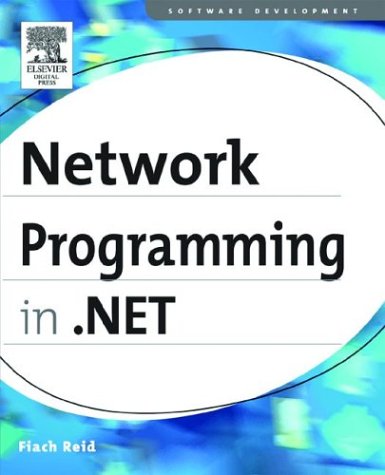
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MimeMultiPart.cs
- invalidudtexception.cs
- MetabaseServerConfig.cs
- __Filters.cs
- WebPartMenuStyle.cs
- SystemFonts.cs
- MarshalByRefObject.cs
- XPathDocumentNavigator.cs
- DataProviderNameConverter.cs
- DataBindingExpressionBuilder.cs
- RightsManagementInformation.cs
- ParameterReplacerVisitor.cs
- CodeGroup.cs
- DataViewSettingCollection.cs
- XmlSerializableWriter.cs
- WebPartConnectionCollection.cs
- NamespaceDisplayAutomationPeer.cs
- SoapSchemaMember.cs
- WinEventHandler.cs
- SqlProviderServices.cs
- StandardBindingReliableSessionElement.cs
- RowSpanVector.cs
- XPathSingletonIterator.cs
- InternalSafeNativeMethods.cs
- ListManagerBindingsCollection.cs
- MsmqInputChannelListenerBase.cs
- followingquery.cs
- MemberHolder.cs
- RuleSettingsCollection.cs
- GridViewRowCollection.cs
- ResizeGrip.cs
- HttpModulesSection.cs
- OdbcConnectionHandle.cs
- DetailsViewUpdatedEventArgs.cs
- PrivilegeNotHeldException.cs
- recordstatefactory.cs
- Rect3D.cs
- TypeConverterHelper.cs
- SafeNativeMethods.cs
- DataKeyArray.cs
- Accessible.cs
- SQLRoleProvider.cs
- SimpleApplicationHost.cs
- DbException.cs
- AuthenticationService.cs
- Int32.cs
- OutputCacheProfileCollection.cs
- ManifestResourceInfo.cs
- AccessibleObject.cs
- SspiSecurityTokenProvider.cs
- GridViewHeaderRowPresenter.cs
- AspProxy.cs
- DBCommand.cs
- ipaddressinformationcollection.cs
- BStrWrapper.cs
- followingsibling.cs
- ListView.cs
- AssemblyName.cs
- ObfuscationAttribute.cs
- ResXBuildProvider.cs
- SliderAutomationPeer.cs
- Border.cs
- XmlSerializerFaultFormatter.cs
- GatewayIPAddressInformationCollection.cs
- StrongNameIdentityPermission.cs
- ExpandCollapseProviderWrapper.cs
- PeerEndPoint.cs
- FrameworkTemplate.cs
- XhtmlBasicValidatorAdapter.cs
- ExtensionQuery.cs
- GeneralTransform.cs
- WebPartConnectionsCancelEventArgs.cs
- AsymmetricKeyExchangeFormatter.cs
- ObjectFactoryCodeDomTreeGenerator.cs
- PhysicalFontFamily.cs
- DirectoryNotFoundException.cs
- HttpHandlersSection.cs
- JpegBitmapDecoder.cs
- ThrowOnMultipleAssignment.cs
- DataServiceQueryException.cs
- NonVisualControlAttribute.cs
- RoleManagerSection.cs
- CodeVariableReferenceExpression.cs
- TableLayout.cs
- OpCellTreeNode.cs
- ResXFileRef.cs
- XPathChildIterator.cs
- WebZone.cs
- IndexOutOfRangeException.cs
- AsymmetricAlgorithm.cs
- CodeMethodInvokeExpression.cs
- CalendarDay.cs
- SendKeys.cs
- DesignerAdRotatorAdapter.cs
- RegisteredExpandoAttribute.cs
- PenThreadPool.cs
- ListViewItemMouseHoverEvent.cs
- SqlDependencyUtils.cs
- Listbox.cs
- MethodToken.cs