Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / Xml / System / Xml / XPath / Internal / followingsibling.cs / 1 / followingsibling.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace MS.Internal.Xml.XPath { using System; using System.Xml; using System.Xml.XPath; using System.Diagnostics; using System.Collections.Generic; using StackNav = ClonableStack; internal sealed class FollSiblingQuery : BaseAxisQuery { StackNav elementStk; List parentStk; XPathNavigator nextInput; public FollSiblingQuery(Query qyInput, string name, string prefix, XPathNodeType type) : base (qyInput, name, prefix, type) { this.elementStk = new StackNav(); this.parentStk = new List (); } private FollSiblingQuery(FollSiblingQuery other) : base(other) { this.elementStk = other.elementStk.Clone(); this.parentStk = new List (other.parentStk); this.nextInput = Clone(other.nextInput); } public override void Reset() { elementStk.Clear(); parentStk.Clear(); nextInput = null; base.Reset(); } private bool Visited(XPathNavigator nav) { XPathNavigator parent = nav.Clone(); parent.MoveToParent(); for (int i = 0; i < parentStk.Count; i++) { if (parent.IsSamePosition(parentStk[i])) { return true; } } parentStk.Add(parent); return false; } private XPathNavigator FetchInput() { XPathNavigator input; do { input = qyInput.Advance(); if (input == null) { return null; } } while (Visited(input)); return input.Clone(); } public override XPathNavigator Advance() { while (true) { if (currentNode == null) { if (nextInput == null) { nextInput = FetchInput(); // This can happen at the begining and at the end } if (elementStk.Count == 0) { if (nextInput == null) { return null; } currentNode = nextInput; nextInput = FetchInput(); } else { currentNode = elementStk.Pop(); } } while (currentNode.IsDescendant(nextInput)) { elementStk.Push(currentNode); currentNode = nextInput; nextInput = qyInput.Advance(); if (nextInput != null) { nextInput = nextInput.Clone(); } } while (currentNode.MoveToNext()) { if (matches(currentNode)) { position++; return currentNode; } } currentNode = null; } } // Advance public override XPathNodeIterator Clone() { return new FollSiblingQuery(this); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace MS.Internal.Xml.XPath { using System; using System.Xml; using System.Xml.XPath; using System.Diagnostics; using System.Collections.Generic; using StackNav = ClonableStack; internal sealed class FollSiblingQuery : BaseAxisQuery { StackNav elementStk; List parentStk; XPathNavigator nextInput; public FollSiblingQuery(Query qyInput, string name, string prefix, XPathNodeType type) : base (qyInput, name, prefix, type) { this.elementStk = new StackNav(); this.parentStk = new List (); } private FollSiblingQuery(FollSiblingQuery other) : base(other) { this.elementStk = other.elementStk.Clone(); this.parentStk = new List (other.parentStk); this.nextInput = Clone(other.nextInput); } public override void Reset() { elementStk.Clear(); parentStk.Clear(); nextInput = null; base.Reset(); } private bool Visited(XPathNavigator nav) { XPathNavigator parent = nav.Clone(); parent.MoveToParent(); for (int i = 0; i < parentStk.Count; i++) { if (parent.IsSamePosition(parentStk[i])) { return true; } } parentStk.Add(parent); return false; } private XPathNavigator FetchInput() { XPathNavigator input; do { input = qyInput.Advance(); if (input == null) { return null; } } while (Visited(input)); return input.Clone(); } public override XPathNavigator Advance() { while (true) { if (currentNode == null) { if (nextInput == null) { nextInput = FetchInput(); // This can happen at the begining and at the end } if (elementStk.Count == 0) { if (nextInput == null) { return null; } currentNode = nextInput; nextInput = FetchInput(); } else { currentNode = elementStk.Pop(); } } while (currentNode.IsDescendant(nextInput)) { elementStk.Push(currentNode); currentNode = nextInput; nextInput = qyInput.Advance(); if (nextInput != null) { nextInput = nextInput.Clone(); } } while (currentNode.MoveToNext()) { if (matches(currentNode)) { position++; return currentNode; } } currentNode = null; } } // Advance public override XPathNodeIterator Clone() { return new FollSiblingQuery(this); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
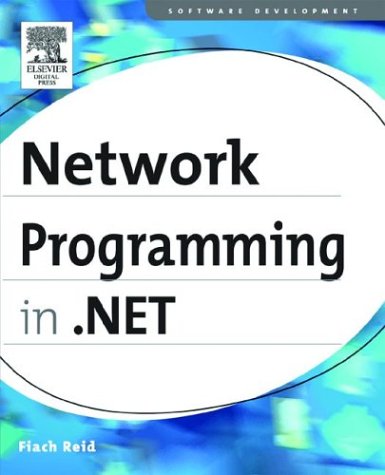
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FileDialogPermission.cs
- AnimationClock.cs
- DataGridRowsPresenter.cs
- CustomPopupPlacement.cs
- SelectionProcessor.cs
- UnknownBitmapEncoder.cs
- IntSecurity.cs
- ListViewSortEventArgs.cs
- PaginationProgressEventArgs.cs
- BookmarkScopeManager.cs
- XmlDocumentFragment.cs
- HierarchicalDataTemplate.cs
- HttpProfileBase.cs
- DefaultEventAttribute.cs
- messageonlyhwndwrapper.cs
- MsmqHostedTransportConfiguration.cs
- StringDictionary.cs
- AuthenticationService.cs
- MemberHolder.cs
- StringStorage.cs
- HtmlInputText.cs
- Attribute.cs
- DeviceContext2.cs
- XmlSerializableReader.cs
- SqlRowUpdatedEvent.cs
- ConfigPathUtility.cs
- AppDomainManager.cs
- ObjectDataSourceWizardForm.cs
- ZoneMembershipCondition.cs
- StylusPlugInCollection.cs
- TypeSystemProvider.cs
- RemotingException.cs
- ServicePointManagerElement.cs
- MetadataCache.cs
- MemberRelationshipService.cs
- GeneratedContractType.cs
- Icon.cs
- FixedPageStructure.cs
- XmlExpressionDumper.cs
- GridViewRow.cs
- RectangleHotSpot.cs
- ToolStripControlHost.cs
- XmlResolver.cs
- AmbientLight.cs
- ObjectStateEntryBaseUpdatableDataRecord.cs
- SolidColorBrush.cs
- DefaultAuthorizationContext.cs
- CompositionAdorner.cs
- ColumnPropertiesGroup.cs
- StopStoryboard.cs
- SingleAnimationBase.cs
- DefaultBinder.cs
- SortKey.cs
- HttpRawResponse.cs
- BaseTemplateParser.cs
- OdbcCommand.cs
- DecoderBestFitFallback.cs
- ExpressionBuilder.cs
- XomlCompilerHelpers.cs
- ToolZone.cs
- EdmComplexPropertyAttribute.cs
- ContentElementCollection.cs
- sqlstateclientmanager.cs
- HeaderedItemsControl.cs
- Attributes.cs
- MetabaseSettings.cs
- SystemIPv6InterfaceProperties.cs
- UmAlQuraCalendar.cs
- SqlConnectionHelper.cs
- CqlIdentifiers.cs
- RegexParser.cs
- ComponentDesigner.cs
- MembershipUser.cs
- XmlRawWriter.cs
- PanelStyle.cs
- ControlTemplate.cs
- ReadOnlyPermissionSet.cs
- WebProxyScriptElement.cs
- TimerElapsedEvenArgs.cs
- AsnEncodedData.cs
- ContextMenuStripGroupCollection.cs
- UnsafeNativeMethodsTablet.cs
- ErrorStyle.cs
- SafeUserTokenHandle.cs
- SymbolEqualComparer.cs
- ParallelQuery.cs
- WeakReferenceList.cs
- WorkflowNamespace.cs
- TransactionChannelFactory.cs
- EnumerableCollectionView.cs
- StringUtil.cs
- SqlComparer.cs
- LifetimeServices.cs
- DataGridViewUtilities.cs
- ContainerUIElement3D.cs
- DrawingVisual.cs
- ResourcesChangeInfo.cs
- CodeIndexerExpression.cs
- WindowsGraphicsWrapper.cs
- WorkflowWebHostingModule.cs