Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / TreeChangeInfo.cs / 1305600 / TreeChangeInfo.cs
//---------------------------------------------------------------------------- // // File: TreeChangeInfo.cs // // Description: // This data-structure is used // 1. As the data that is passed around by the DescendentsWalker // during an AncestorChanged tree-walk. // // Copyright (C) by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; using System.Windows.Media; using System.Windows.Controls; using System.Windows.Documents; using MS.Internal; using MS.Utility; namespace System.Windows { ////// This is the data that is passed through the DescendentsWalker /// during an AncestorChange tree-walk. /// internal struct TreeChangeInfo { #region Properties public TreeChangeInfo(DependencyObject root, DependencyObject parent, bool isAddOperation) { _rootOfChange = root; _isAddOperation = isAddOperation; _topmostCollapsedParentNode = null; _rootInheritableValues = null; _inheritablePropertiesStack = null; _valueIndexer = 0; // Create the InheritableProperties cache for the parent // and push it to start the stack ... we don't need to // pop this when we're done because we'll be throing the // stack away at that point. InheritablePropertiesStack.Push(CreateParentInheritableProperties(root, parent, isAddOperation)); } // // This method // 1. Is called from AncestorChange InvalidateTree. // 2. It is used to create the InheritableProperties on the given node. // 3. It also accumulates oldValues for the inheritable properties that are about to be invalidated // internal FrugalObjectListCreateParentInheritableProperties( DependencyObject d, DependencyObject parent, bool isAddOperation) { Debug.Assert(d != null, "Must have non-null current node"); if (parent == null) { return new FrugalObjectList (0); } DependencyObjectType treeObjDOT = d.DependencyObjectType; // See if we have a cached value. EffectiveValueEntry[] parentEffectiveValues = null; uint parentEffectiveValuesCount = 0; uint inheritablePropertiesCount = 0; // If inheritable properties aren't cached on you then use the effective // values cache on the parent to discover those inherited properties that // may need to be invalidated on the children nodes. if (!parent.IsSelfInheritanceParent) { DependencyObject inheritanceParent = parent.InheritanceParent; if (inheritanceParent != null) { parentEffectiveValues = inheritanceParent.EffectiveValues; parentEffectiveValuesCount = inheritanceParent.EffectiveValuesCount; inheritablePropertiesCount = inheritanceParent.InheritableEffectiveValuesCount; } } else { parentEffectiveValues = parent.EffectiveValues; parentEffectiveValuesCount = parent.EffectiveValuesCount; inheritablePropertiesCount = parent.InheritableEffectiveValuesCount; } FrugalObjectList inheritableProperties = new FrugalObjectList ((int) inheritablePropertiesCount); if (inheritablePropertiesCount == 0) { return inheritableProperties; } _rootInheritableValues = new InheritablePropertyChangeInfo[(int) inheritablePropertiesCount]; int inheritableIndex = 0; FrameworkObject foParent = new FrameworkObject(parent); for (uint i=0; i /// This is a stack that is used during an AncestorChange operation. /// I holds the InheritableProperties cache on the parent nodes in /// the tree in the order of traversal. The top of the stack holds /// the cache for the immediate parent node. /// internal Stack > InheritablePropertiesStack { get { if (_inheritablePropertiesStack == null) { _inheritablePropertiesStack = new Stack >(1); } return _inheritablePropertiesStack; } } /// /// When we enter a Visibility=Collapsed subtree, we remember that node /// using this property. As we process the children of this collapsed /// node, we see this property as non-null and know we're collapsed. /// As we exit the subtree, this reference is nulled out which means /// we don't know whether we're in a collapsed tree and the optimizations /// based on Visibility=Collapsed are not valid and should not be used. /// internal object TopmostCollapsedParentNode { get { return _topmostCollapsedParentNode; } set { _topmostCollapsedParentNode = value; } } // Indicates if this is a add child tree operation internal bool IsAddOperation { get { return _isAddOperation; } } // This is the element at the root of the sub-tree that had a parent change. internal DependencyObject Root { get { return _rootOfChange; } } #endregion Properties #region Data private Stack> _inheritablePropertiesStack; private object _topmostCollapsedParentNode; private bool _isAddOperation; private DependencyObject _rootOfChange; private InheritablePropertyChangeInfo[] _rootInheritableValues; private int _valueIndexer; #endregion Data } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
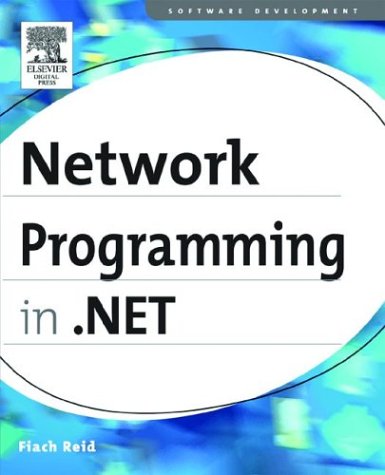
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ClusterRegistryConfigurationProvider.cs
- StylusCaptureWithinProperty.cs
- DeflateStream.cs
- SecurityHelper.cs
- RightsController.cs
- DivideByZeroException.cs
- CommunicationObjectAbortedException.cs
- DBCSCodePageEncoding.cs
- DataSourceXmlTextReader.cs
- MatchingStyle.cs
- XsltSettings.cs
- DependencyObjectPropertyDescriptor.cs
- XmlILCommand.cs
- Roles.cs
- ProfileProvider.cs
- DataSourceSelectArguments.cs
- ContentOperations.cs
- HttpServerVarsCollection.cs
- DataGridViewSortCompareEventArgs.cs
- SafeCertificateStore.cs
- SQlBooleanStorage.cs
- CalloutQueueItem.cs
- CharAnimationBase.cs
- CountAggregationOperator.cs
- TypeSemantics.cs
- EntityParameterCollection.cs
- EntityConnectionStringBuilder.cs
- BindingMemberInfo.cs
- XmlSchema.cs
- AtomMaterializerLog.cs
- PatternMatchRules.cs
- RectangleF.cs
- RawStylusActions.cs
- WindowsSlider.cs
- EncoderBestFitFallback.cs
- FramingEncoders.cs
- TextCollapsingProperties.cs
- _SSPIWrapper.cs
- WindowsTokenRoleProvider.cs
- SamlSecurityToken.cs
- DBCommand.cs
- HttpsHostedTransportConfiguration.cs
- InvalidFilterCriteriaException.cs
- ConnectionManagementSection.cs
- PreviewKeyDownEventArgs.cs
- RootProfilePropertySettingsCollection.cs
- FromReply.cs
- ScrollEventArgs.cs
- ReadWriteObjectLock.cs
- TaskResultSetter.cs
- Int16Storage.cs
- SectionInformation.cs
- InheritanceAttribute.cs
- GCHandleCookieTable.cs
- SendKeys.cs
- EncryptedKeyHashIdentifierClause.cs
- TableLayoutStyle.cs
- UrlUtility.cs
- ResourceDescriptionAttribute.cs
- ActivityStatusChangeEventArgs.cs
- control.ime.cs
- MailSettingsSection.cs
- Pick.cs
- XmlDomTextWriter.cs
- ToolStripProfessionalLowResolutionRenderer.cs
- ManagedIStream.cs
- RuntimeHelpers.cs
- FileLoadException.cs
- QilNode.cs
- SafeSecurityHandles.cs
- PartitionerQueryOperator.cs
- ProxyWebPart.cs
- SQLInt32.cs
- TextEditorCharacters.cs
- Label.cs
- HashStream.cs
- SplineKeyFrames.cs
- FacetValueContainer.cs
- StyleReferenceConverter.cs
- DashStyle.cs
- ACE.cs
- OleDbError.cs
- TextFindEngine.cs
- InvariantComparer.cs
- BasicKeyConstraint.cs
- IisTraceWebEventProvider.cs
- ExternalException.cs
- TemplatedWizardStep.cs
- ReflectEventDescriptor.cs
- CellParagraph.cs
- MultilineStringConverter.cs
- Opcode.cs
- ListItemConverter.cs
- QueryOperationResponseOfT.cs
- ListBoxAutomationPeer.cs
- TextSpanModifier.cs
- BasicHttpBindingElement.cs
- HttpListenerContext.cs
- XmlSchemaExternal.cs
- Binding.cs