Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / xsp / System / Web / Util / ReadWriteObjectLock.cs / 1 / ReadWriteObjectLock.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * ReadWriteObjectLock * * Copyright (c) 1998-1999, Microsoft Corporation * */ namespace System.Web.Util { using System.Runtime.Serialization.Formatters; using System.Threading; class ReadWriteObjectLock { // Assumption: // -1 = a writer has the lock // 0 = no one has the lock // >0 = number of readers using the lock private int _lock; internal ReadWriteObjectLock() { } internal virtual void AcquireRead() { lock(this) { while (_lock == -1) { try { Monitor.Wait(this); } catch (ThreadInterruptedException) { // Just keep looping } } _lock++; } } internal virtual void ReleaseRead() { lock(this) { Debug.Assert(_lock > 0); _lock--; if (_lock == 0) { Monitor.PulseAll(this); } } } internal virtual void AcquireWrite() { lock(this) { while (_lock != 0) { try { Monitor.Wait(this); } catch (ThreadInterruptedException) { // Just keep looping } } _lock = -1; } } internal virtual void ReleaseWrite() { lock(this) { Debug.Assert(_lock == -1); _lock = 0; Monitor.PulseAll(this); } } /* internal virtual void AssertReadLock() { #if DBG Debug.Assert(_lock > 0); #endif } internal virtual void AssertWriteLock() { #if DBG Debug.Assert(_lock == -1); #endif } */ } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * ReadWriteObjectLock * * Copyright (c) 1998-1999, Microsoft Corporation * */ namespace System.Web.Util { using System.Runtime.Serialization.Formatters; using System.Threading; class ReadWriteObjectLock { // Assumption: // -1 = a writer has the lock // 0 = no one has the lock // >0 = number of readers using the lock private int _lock; internal ReadWriteObjectLock() { } internal virtual void AcquireRead() { lock(this) { while (_lock == -1) { try { Monitor.Wait(this); } catch (ThreadInterruptedException) { // Just keep looping } } _lock++; } } internal virtual void ReleaseRead() { lock(this) { Debug.Assert(_lock > 0); _lock--; if (_lock == 0) { Monitor.PulseAll(this); } } } internal virtual void AcquireWrite() { lock(this) { while (_lock != 0) { try { Monitor.Wait(this); } catch (ThreadInterruptedException) { // Just keep looping } } _lock = -1; } } internal virtual void ReleaseWrite() { lock(this) { Debug.Assert(_lock == -1); _lock = 0; Monitor.PulseAll(this); } } /* internal virtual void AssertReadLock() { #if DBG Debug.Assert(_lock > 0); #endif } internal virtual void AssertWriteLock() { #if DBG Debug.Assert(_lock == -1); #endif } */ } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
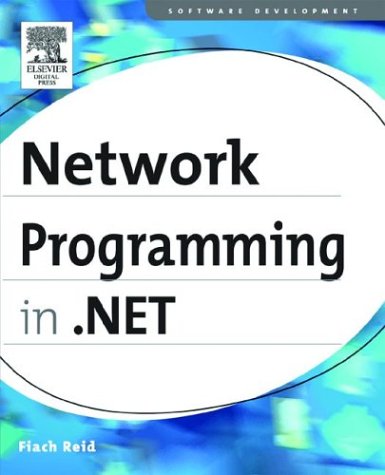
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AsyncOperation.cs
- QuaternionAnimationBase.cs
- SimpleFieldTemplateFactory.cs
- WorkflowLayouts.cs
- SortDescription.cs
- ControlUtil.cs
- nulltextcontainer.cs
- FindCriteria11.cs
- DocumentEventArgs.cs
- ErrorHandlerModule.cs
- ToolStripSeparator.cs
- SQLBoolean.cs
- AutoResetEvent.cs
- Int32Rect.cs
- WebReference.cs
- AddressingVersion.cs
- OleDbConnection.cs
- EdmToObjectNamespaceMap.cs
- ServiceReference.cs
- SymDocumentType.cs
- Regex.cs
- TraceHwndHost.cs
- IntranetCredentialPolicy.cs
- DocumentStream.cs
- DocumentGridPage.cs
- DataServiceQueryException.cs
- CompressionTransform.cs
- TypedTableBaseExtensions.cs
- UrlMappingsSection.cs
- CodeAttributeDeclaration.cs
- CurrentTimeZone.cs
- ScriptIgnoreAttribute.cs
- ToggleButton.cs
- PKCS1MaskGenerationMethod.cs
- Rotation3DAnimationBase.cs
- AutomationEventArgs.cs
- TransactionInformation.cs
- ThicknessAnimation.cs
- TextTreeTextNode.cs
- UnmanagedMarshal.cs
- SystemTcpConnection.cs
- TypeCollectionDesigner.xaml.cs
- TimeIntervalCollection.cs
- recordstate.cs
- ResourceDescriptionAttribute.cs
- MultiplexingDispatchMessageFormatter.cs
- MouseButton.cs
- _ConnectionGroup.cs
- FixedSOMTable.cs
- GeneralTransform2DTo3D.cs
- ElementsClipboardData.cs
- XmlAutoDetectWriter.cs
- XamlNamespaceHelper.cs
- Resources.Designer.cs
- UrlMappingsModule.cs
- EntityDataSourceStatementEditorForm.cs
- Blend.cs
- TraceContextRecord.cs
- WindowsFormsHelpers.cs
- ButtonBase.cs
- DataGridViewButtonColumn.cs
- PeerNameRecord.cs
- TextServicesCompartmentContext.cs
- IdentityModelDictionary.cs
- Int64KeyFrameCollection.cs
- WindowsRichEdit.cs
- TimeoutHelper.cs
- QualifiedCellIdBoolean.cs
- CollectionViewGroupRoot.cs
- webeventbuffer.cs
- ZeroOpNode.cs
- FileVersion.cs
- SmtpAuthenticationManager.cs
- SchemaTableColumn.cs
- Resources.Designer.cs
- EditingMode.cs
- ConnectionOrientedTransportChannelFactory.cs
- QilIterator.cs
- RootProfilePropertySettingsCollection.cs
- UpdateManifestForBrowserApplication.cs
- XmlHierarchicalDataSourceView.cs
- LineServices.cs
- xsdvalidator.cs
- ListViewInsertEventArgs.cs
- Translator.cs
- Win32MouseDevice.cs
- FixedSOMLineCollection.cs
- SwitchElementsCollection.cs
- SiteIdentityPermission.cs
- TaskResultSetter.cs
- ADMembershipProvider.cs
- InkCanvasSelection.cs
- TrustLevel.cs
- XNodeNavigator.cs
- SrgsItemList.cs
- arabicshape.cs
- RequestQueue.cs
- TabletCollection.cs
- EtwTrace.cs
- MaterialGroup.cs