Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WCF / IdentityModel / System / IdentityModel / HashStream.cs / 1305376 / HashStream.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.IdentityModel { using System.IO; using System.Security.Cryptography; using System.IdentityModel.Diagnostics; sealed class HashStream : Stream { HashAlgorithm hash; long length; bool hashNeedsReset; MemoryStream logStream; public HashStream(HashAlgorithm hash) { if (hash == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("hash"); Reset(hash); } public override bool CanRead { get { return false; } } public override bool CanWrite { get { return true; } } public override bool CanSeek { get { return false; } } public HashAlgorithm Hash { get { return this.hash; } } public override long Length { get { return this.length; } } public override long Position { get { return this.length; } set { // PreSharp Bug: Property get methods should not throw exceptions. #pragma warning suppress 56503 throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotSupportedException()); } } public override void Flush() { } public void FlushHash() { this.hash.TransformFinalBlock(CryptoHelper.EmptyBuffer, 0, 0); if (DigestTraceRecordHelper.ShouldTraceDigest) DigestTraceRecordHelper.TraceDigest(this.logStream, this.hash); } public byte[] FlushHashAndGetValue() { FlushHash(); return this.hash.Hash; } public override int Read(byte[] buffer, int offset, int count) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotSupportedException()); } public void Reset() { if (this.hashNeedsReset) { this.hash.Initialize(); this.hashNeedsReset = false; } this.length = 0; if (DigestTraceRecordHelper.ShouldTraceDigest) this.logStream = new MemoryStream(); } public void Reset(HashAlgorithm hash) { this.hash = hash; this.hashNeedsReset = false; this.length = 0; if (DigestTraceRecordHelper.ShouldTraceDigest) this.logStream = new MemoryStream(); } public override void Write(byte[] buffer, int offset, int count) { this.hash.TransformBlock(buffer, offset, count, buffer, offset); this.length += count; this.hashNeedsReset = true; if (DigestTraceRecordHelper.ShouldTraceDigest) this.logStream.Write(buffer, offset, count); } public override long Seek(long offset, SeekOrigin origin) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotSupportedException()); } public override void SetLength(long length) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotSupportedException()); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.IdentityModel { using System.IO; using System.Security.Cryptography; using System.IdentityModel.Diagnostics; sealed class HashStream : Stream { HashAlgorithm hash; long length; bool hashNeedsReset; MemoryStream logStream; public HashStream(HashAlgorithm hash) { if (hash == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("hash"); Reset(hash); } public override bool CanRead { get { return false; } } public override bool CanWrite { get { return true; } } public override bool CanSeek { get { return false; } } public HashAlgorithm Hash { get { return this.hash; } } public override long Length { get { return this.length; } } public override long Position { get { return this.length; } set { // PreSharp Bug: Property get methods should not throw exceptions. #pragma warning suppress 56503 throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotSupportedException()); } } public override void Flush() { } public void FlushHash() { this.hash.TransformFinalBlock(CryptoHelper.EmptyBuffer, 0, 0); if (DigestTraceRecordHelper.ShouldTraceDigest) DigestTraceRecordHelper.TraceDigest(this.logStream, this.hash); } public byte[] FlushHashAndGetValue() { FlushHash(); return this.hash.Hash; } public override int Read(byte[] buffer, int offset, int count) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotSupportedException()); } public void Reset() { if (this.hashNeedsReset) { this.hash.Initialize(); this.hashNeedsReset = false; } this.length = 0; if (DigestTraceRecordHelper.ShouldTraceDigest) this.logStream = new MemoryStream(); } public void Reset(HashAlgorithm hash) { this.hash = hash; this.hashNeedsReset = false; this.length = 0; if (DigestTraceRecordHelper.ShouldTraceDigest) this.logStream = new MemoryStream(); } public override void Write(byte[] buffer, int offset, int count) { this.hash.TransformBlock(buffer, offset, count, buffer, offset); this.length += count; this.hashNeedsReset = true; if (DigestTraceRecordHelper.ShouldTraceDigest) this.logStream.Write(buffer, offset, count); } public override long Seek(long offset, SeekOrigin origin) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotSupportedException()); } public override void SetLength(long length) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotSupportedException()); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
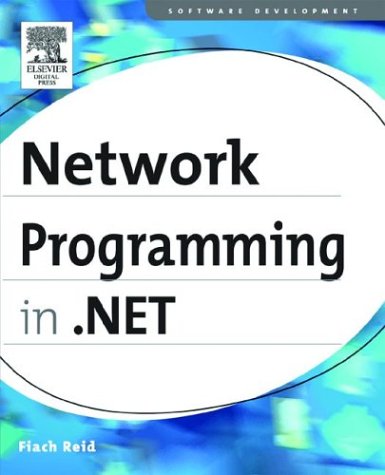
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DiscoveryDocument.cs
- XmlSchemaCollection.cs
- LineGeometry.cs
- RightsManagementLicense.cs
- BitFlagsGenerator.cs
- PolyLineSegment.cs
- NumberSubstitution.cs
- NetCodeGroup.cs
- SerTrace.cs
- ImageAnimator.cs
- X509SubjectKeyIdentifierClause.cs
- UnionExpr.cs
- IfElseDesigner.xaml.cs
- DataGridPagerStyle.cs
- GreaterThanOrEqual.cs
- PackagePartCollection.cs
- SqlBulkCopyColumnMappingCollection.cs
- IndicShape.cs
- CallSiteBinder.cs
- LabelAutomationPeer.cs
- SettingsPropertyValueCollection.cs
- ExtensionDataObject.cs
- DataControlLinkButton.cs
- DataGridColumnFloatingHeader.cs
- MulticastOption.cs
- Size3DConverter.cs
- ComPlusInstanceContextInitializer.cs
- FlowLayoutPanel.cs
- SettingsProperty.cs
- Deflater.cs
- EndPoint.cs
- CryptoProvider.cs
- safelink.cs
- InputLanguageCollection.cs
- JpegBitmapEncoder.cs
- SetState.cs
- _ListenerRequestStream.cs
- DictionaryEntry.cs
- Point3DCollection.cs
- WizardPanelChangingEventArgs.cs
- StyleBamlTreeBuilder.cs
- DataList.cs
- QEncodedStream.cs
- UriExt.cs
- DiagnosticsConfiguration.cs
- DocumentPaginator.cs
- OuterGlowBitmapEffect.cs
- Thickness.cs
- InlineCollection.cs
- XmlSerializer.cs
- ThemeDictionaryExtension.cs
- CanonicalFormWriter.cs
- ToolboxService.cs
- ThreadStartException.cs
- EntityDataSourceEntityTypeFilterConverter.cs
- FileReader.cs
- SqlClientPermission.cs
- XmlAttributeCollection.cs
- Compensate.cs
- PropertyGeneratedEventArgs.cs
- CompoundFileDeflateTransform.cs
- MetadataCache.cs
- DependencyPropertyChangedEventArgs.cs
- ProgressBarRenderer.cs
- DesignerLinkAdapter.cs
- InkCanvasInnerCanvas.cs
- BaseTemplateBuildProvider.cs
- CqlLexerHelpers.cs
- IgnoreSectionHandler.cs
- HWStack.cs
- SelectionPattern.cs
- DataColumnChangeEvent.cs
- Padding.cs
- TabItem.cs
- WmpBitmapDecoder.cs
- SerializationFieldInfo.cs
- NegotiateStream.cs
- MenuScrollingVisibilityConverter.cs
- Glyph.cs
- NavigateEvent.cs
- EntityDataSourceWizardForm.cs
- DetailsViewCommandEventArgs.cs
- PriorityBindingExpression.cs
- PreservationFileReader.cs
- DefaultAuthorizationContext.cs
- XmlBoundElement.cs
- IriParsingElement.cs
- HtmlInputRadioButton.cs
- CustomAttributeFormatException.cs
- Metafile.cs
- NativeMethods.cs
- WebDisplayNameAttribute.cs
- StreamGeometryContext.cs
- ProtocolException.cs
- MonitorWrapper.cs
- ProfileSettings.cs
- HttpHeaderCollection.cs
- SHA512Managed.cs
- ClonableStack.cs
- DeadCharTextComposition.cs