Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / System / Windows / Input / Stylus / PenThreadPool.cs / 1 / PenThreadPool.cs
using System; using System.Collections.Generic; using System.Threading; using System.Windows.Threading; using System.Security; using System.Security.Permissions; using MS.Win32.Penimc; namespace System.Windows.Input { ///////////////////////////////////////////////////////////////////////// ////// /// internal class PenThreadPool { ////// Critical - Constructor for singleton of our PenThreadPool. /// marking this critical to prevent inadvertant access by transparent code /// /// Called by critical methods: /// Instance (above) /// /// [SecurityCritical] static PenThreadPool() { } ///////////////////////////////////////////////////////////////////// ////// /// ////// Critical - marking this critical to prevent inadvertant /// access by transparent code /// /// [SecurityCritical] [ThreadStatic] private static PenThreadPool _penThreadPool; ///////////////////////////////////////////////////////////////////// ////// ////// Critical - Returns a PenThread (creates as needed). /// marking this critical to prevent inadvertant access by transparent code /// /// Called by critical methods: /// PenContext.Dispose /// PenContext.Enable /// PenContext.Disable /// /// [SecurityCritical] internal static PenThread GetPenThreadForPenContext(PenContext penContext) { // Create the threadstatic DynamicRendererThreadManager as needed for calling thread. // It only creates one if (_penThreadPool == null) { _penThreadPool = new PenThreadPool(); } return _penThreadPool.GetPenThreadForPenContextHelper(penContext); // Adds to weak ref list if creating new one. } ///////////////////////////////////////////////////////////////////// ////// /// ////// Critical - marking this critical to prevent inadvertant /// access by transparent code /// /// [SecurityCritical] private List_penThreadWeakRefList; ///////////////////////////////////////////////////////////////////// /// /// /// ////// Critical - Initializes critical data: m_PenThreads /// /// [SecurityCritical] internal PenThreadPool() { _penThreadWeakRefList = new List(); } /// /// Critical - Calls SecurityCritical code (PenThread constructor). /// Called by BeginService. /// TreatAsSafe boundry is Stylus.EnableCore, Stylus.RegisterHwndForInput /// and HwndWrapperHook class (via HwndSource.InputFilterMessage). /// [SecurityCritical] private PenThread GetPenThreadForPenContextHelper(PenContext penContext) { bool needCleanup = false; PenThread penThread = null; int i; // Scan existing penthreads to see if we have an available slot for context. for (i=0; i < _penThreadWeakRefList.Count; i++) { PenThread penThreadFound = _penThreadWeakRefList[i].Target as PenThread; if (penThreadFound == null) { needCleanup = true; } else { // See if we can use this one if (penContext == null || penThreadFound.AddPenContext(penContext)) { // We can use this one. penThread = penThreadFound; break; } } } if (needCleanup) { // prune invalid refs for (i=_penThreadWeakRefList.Count - 1; i >= 0; i--) { if (_penThreadWeakRefList[i].Target == null) { _penThreadWeakRefList.RemoveAt(i); } } } if (penThread == null) { penThread = new PenThread(); // Make sure we add this context to the penthread if (penContext != null) { penThread.AddPenContext(penContext); } _penThreadWeakRefList.Add(new WeakReference(penThread)); } return penThread; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Collections.Generic; using System.Threading; using System.Windows.Threading; using System.Security; using System.Security.Permissions; using MS.Win32.Penimc; namespace System.Windows.Input { ///////////////////////////////////////////////////////////////////////// ////// /// internal class PenThreadPool { ////// Critical - Constructor for singleton of our PenThreadPool. /// marking this critical to prevent inadvertant access by transparent code /// /// Called by critical methods: /// Instance (above) /// /// [SecurityCritical] static PenThreadPool() { } ///////////////////////////////////////////////////////////////////// ////// /// ////// Critical - marking this critical to prevent inadvertant /// access by transparent code /// /// [SecurityCritical] [ThreadStatic] private static PenThreadPool _penThreadPool; ///////////////////////////////////////////////////////////////////// ////// ////// Critical - Returns a PenThread (creates as needed). /// marking this critical to prevent inadvertant access by transparent code /// /// Called by critical methods: /// PenContext.Dispose /// PenContext.Enable /// PenContext.Disable /// /// [SecurityCritical] internal static PenThread GetPenThreadForPenContext(PenContext penContext) { // Create the threadstatic DynamicRendererThreadManager as needed for calling thread. // It only creates one if (_penThreadPool == null) { _penThreadPool = new PenThreadPool(); } return _penThreadPool.GetPenThreadForPenContextHelper(penContext); // Adds to weak ref list if creating new one. } ///////////////////////////////////////////////////////////////////// ////// /// ////// Critical - marking this critical to prevent inadvertant /// access by transparent code /// /// [SecurityCritical] private List_penThreadWeakRefList; ///////////////////////////////////////////////////////////////////// /// /// /// ////// Critical - Initializes critical data: m_PenThreads /// /// [SecurityCritical] internal PenThreadPool() { _penThreadWeakRefList = new List(); } /// /// Critical - Calls SecurityCritical code (PenThread constructor). /// Called by BeginService. /// TreatAsSafe boundry is Stylus.EnableCore, Stylus.RegisterHwndForInput /// and HwndWrapperHook class (via HwndSource.InputFilterMessage). /// [SecurityCritical] private PenThread GetPenThreadForPenContextHelper(PenContext penContext) { bool needCleanup = false; PenThread penThread = null; int i; // Scan existing penthreads to see if we have an available slot for context. for (i=0; i < _penThreadWeakRefList.Count; i++) { PenThread penThreadFound = _penThreadWeakRefList[i].Target as PenThread; if (penThreadFound == null) { needCleanup = true; } else { // See if we can use this one if (penContext == null || penThreadFound.AddPenContext(penContext)) { // We can use this one. penThread = penThreadFound; break; } } } if (needCleanup) { // prune invalid refs for (i=_penThreadWeakRefList.Count - 1; i >= 0; i--) { if (_penThreadWeakRefList[i].Target == null) { _penThreadWeakRefList.RemoveAt(i); } } } if (penThread == null) { penThread = new PenThread(); // Make sure we add this context to the penthread if (penContext != null) { penThread.AddPenContext(penContext); } _penThreadWeakRefList.Add(new WeakReference(penThread)); } return penThread; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
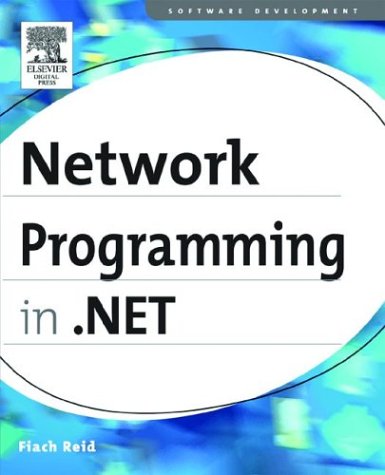
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FtpWebRequest.cs
- GB18030Encoding.cs
- Certificate.cs
- PasswordTextContainer.cs
- SiteMapHierarchicalDataSourceView.cs
- RemoveStoryboard.cs
- WebServiceClientProxyGenerator.cs
- CookieParameter.cs
- ApplicationSecurityInfo.cs
- BindingRestrictions.cs
- precedingquery.cs
- SurrogateEncoder.cs
- ConfigurationStrings.cs
- SpecialFolderEnumConverter.cs
- TypeLoadException.cs
- RuleAttributes.cs
- NoneExcludedImageIndexConverter.cs
- FontStretch.cs
- SafeHandle.cs
- NavigationEventArgs.cs
- CommandBinding.cs
- BooleanSwitch.cs
- FontStretchConverter.cs
- DataTablePropertyDescriptor.cs
- Substitution.cs
- QueryReaderSettings.cs
- HashCryptoHandle.cs
- WebReferenceOptions.cs
- AssemblyCollection.cs
- CustomCategoryAttribute.cs
- ConnectivityStatus.cs
- WebBrowserProgressChangedEventHandler.cs
- oledbmetadatacolumnnames.cs
- Int64Storage.cs
- SqlDependencyListener.cs
- SequentialUshortCollection.cs
- ComplexBindingPropertiesAttribute.cs
- HtmlInputControl.cs
- Delegate.cs
- CompareValidator.cs
- UpDownBase.cs
- WebPartMenu.cs
- MDIWindowDialog.cs
- ListenerHandler.cs
- ToolboxItemAttribute.cs
- XmlResolver.cs
- VScrollBar.cs
- ScriptingSectionGroup.cs
- CustomValidator.cs
- LongTypeConverter.cs
- TextViewElement.cs
- EdmSchemaError.cs
- CRYPTPROTECT_PROMPTSTRUCT.cs
- ParagraphResult.cs
- DesignerForm.cs
- ImageMetadata.cs
- FormViewUpdateEventArgs.cs
- DriveInfo.cs
- TextFormatter.cs
- Unit.cs
- EntityCommandDefinition.cs
- QueryProcessor.cs
- FlowLayoutPanelDesigner.cs
- EnumConverter.cs
- DataGridHyperlinkColumn.cs
- ClientUIRequest.cs
- ConfigXmlText.cs
- ToolStripPanelCell.cs
- NameObjectCollectionBase.cs
- AccessKeyManager.cs
- InheritanceContextHelper.cs
- RuleSetBrowserDialog.cs
- EditorPartChrome.cs
- EventDriven.cs
- AccessedThroughPropertyAttribute.cs
- SeekStoryboard.cs
- SqlDataSourceSelectingEventArgs.cs
- DisposableCollectionWrapper.cs
- Span.cs
- DurableInstanceProvider.cs
- Translator.cs
- RoleGroupCollection.cs
- SecurityIdentifierElementCollection.cs
- SymmetricKey.cs
- ExpressionVisitor.cs
- SecurityCriticalDataForSet.cs
- selecteditemcollection.cs
- DesignSurfaceEvent.cs
- WaitHandle.cs
- AsymmetricAlgorithm.cs
- HttpDictionary.cs
- hebrewshape.cs
- Divide.cs
- DataGridViewAutoSizeModeEventArgs.cs
- LZCodec.cs
- DataSourceSerializationException.cs
- ProfileSection.cs
- WsatRegistrationHeader.cs
- IncrementalHitTester.cs
- EncoderParameters.cs