Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / Media / textformatting / TextRunCache.cs / 1 / TextRunCache.cs
//------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation, 2001 // // File: TextRunCache.cs // // Contents: Cache of text and text properties of run // // Spec: [....]/sites/Avalon/Specs/Text%20Formatting%20API.doc // // Created: 2-25-2003 [....] ([....]) // //----------------------------------------------------------------------- using System; using System.Collections.Generic; using MS.Internal.PresentationCore; using MS.Internal.TextFormatting; namespace System.Windows.Media.TextFormatting { ////// TextFormatter caches runs it receives from GetTextRun callback. This cache /// object is managed by text layout client. /// /// This method is used to improve performance in application whose fetching the /// run has significant performance implication. Application using this caching /// mechanism is responsible for invalidating the content in the cache when /// its changed. /// public sealed class TextRunCache { ////// Constructing text run cache /// public TextRunCache() {} ////// Client to notify change in part of the cache when text or /// properties of the run is being added, removed or replaced. /// /// text source character index to specify where in the source text the change starts. /// the number of text source characters to be added in the source text /// the number of text source characters to be removed in the source text public void Change( int textSourceCharacterIndex, int addition, int removal ) { if(_imp != null) { _imp.Change( textSourceCharacterIndex, addition, removal ); } } ////// Client to invalidate the whole cache, in effect emptying the cache and /// cause the cache refill in subsequent call to Text Formatting API. /// public void Invalidate() { if(_imp != null) { _imp = null; } } ////// Return all cached TextRun in a TextSpan list. If TextRun is not cached for a particular character range, /// the TextSpan would contain null TextRun object. /// #if OPTIMALBREAK_API public IList> GetTextRunSpans() #else [FriendAccessAllowed] internal IList > GetTextRunSpans() #endif { if (_imp != null) { return _imp.GetTextRunSpans(); } // otherwise, return an empty collection return new TextSpan [0]; } /// /// Get/set the actual cache instance /// internal TextRunCacheImp Imp { get { return _imp; } set { _imp = value; } } private TextRunCacheImp _imp; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
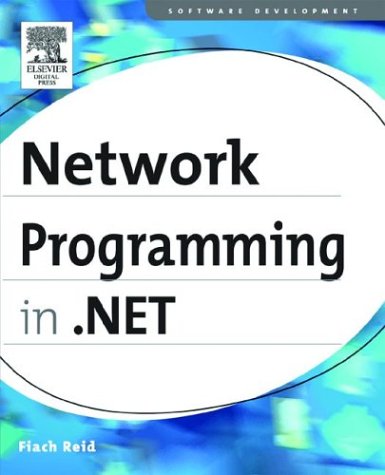
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ArraySegment.cs
- Monitor.cs
- QilDataSource.cs
- Point3DAnimationUsingKeyFrames.cs
- Int32AnimationUsingKeyFrames.cs
- ILGenerator.cs
- ManagementNamedValueCollection.cs
- DateTimeFormatInfo.cs
- FunctionDetailsReader.cs
- ClientConfigPaths.cs
- NavigationProperty.cs
- DateTimeUtil.cs
- StringComparer.cs
- DateTimeFormatInfo.cs
- Activator.cs
- ProcessHostServerConfig.cs
- manifestimages.cs
- RouteItem.cs
- PermissionRequestEvidence.cs
- System.Data.OracleClient_BID.cs
- HttpProfileGroupBase.cs
- SessionStateSection.cs
- HttpVersion.cs
- ListViewCancelEventArgs.cs
- ColumnResizeAdorner.cs
- ITreeGenerator.cs
- TypedTableHandler.cs
- Timer.cs
- GridViewAutoFormat.cs
- DataControlFieldHeaderCell.cs
- Win32Native.cs
- SQLBinary.cs
- PersonalizationStateInfoCollection.cs
- TraceHandlerErrorFormatter.cs
- _UriSyntax.cs
- TextServicesManager.cs
- BitmapFrameDecode.cs
- CollectionView.cs
- XMLSyntaxException.cs
- ListBase.cs
- AutomationAttributeInfo.cs
- HyperLinkStyle.cs
- AuthenticationSection.cs
- DbProviderSpecificTypePropertyAttribute.cs
- Condition.cs
- ColumnResizeAdorner.cs
- ToolStripGrip.cs
- Misc.cs
- CompoundFileIOPermission.cs
- PointAnimationUsingPath.cs
- PasswordBox.cs
- AuthenticationService.cs
- BinaryWriter.cs
- BufferAllocator.cs
- XmlCharCheckingWriter.cs
- FontFamily.cs
- View.cs
- SamlAssertionKeyIdentifierClause.cs
- RelationshipDetailsCollection.cs
- CfgParser.cs
- TypedRowGenerator.cs
- SqlAliasesReferenced.cs
- XmlSchemaImport.cs
- behaviorssection.cs
- PackageRelationshipSelector.cs
- FacetChecker.cs
- LazyTextWriterCreator.cs
- DesignerAutoFormat.cs
- DataGridViewColumnConverter.cs
- FixedPosition.cs
- ListSortDescription.cs
- _CookieModule.cs
- IDictionary.cs
- ReaderContextStackData.cs
- VisualBasicHelper.cs
- DetailsViewUpdatedEventArgs.cs
- ZipIOZip64EndOfCentralDirectoryBlock.cs
- TypeConverterHelper.cs
- WebPartCatalogAddVerb.cs
- CustomTypeDescriptor.cs
- TextFormatter.cs
- ClientApiGenerator.cs
- MetadataItem.cs
- AssociationSetEnd.cs
- CodeEntryPointMethod.cs
- RightsManagementInformation.cs
- PropertyFilter.cs
- OverflowException.cs
- IconBitmapDecoder.cs
- MethodImplAttribute.cs
- DefaultTextStore.cs
- QilFunction.cs
- Error.cs
- DesignerLoader.cs
- BmpBitmapEncoder.cs
- HtmlHead.cs
- EditingScope.cs
- ColumnClickEvent.cs
- DataGridTableCollection.cs
- AuthStoreRoleProvider.cs