Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / MS / Internal / Controls / TemplatedAdorner.cs / 1 / TemplatedAdorner.cs
//---------------------------------------------------------------------------- // //// Copyright (C) 2005 by Microsoft Corporation. All rights reserved. // // // // Description: // TemplatedAdorner applies the style provided in the ctor to a // control and provides a transform via GetDesiredTransform that // will cause the AdornedElementPlaceholder to be positioned directly // over the AdornedElement. // // See specs at [....]/connecteddata/Specs/Validation.mht // // History: // 02/01/2005 [....]: created. // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.Diagnostics; using System.Windows.Media; using System.Windows; using System.Windows.Data; using System.Windows.Controls; using System.Windows.Documents; using MS.Utility; namespace MS.Internal.Controls { ////// This class is sealed because it calls OnVisualChildrenChanged virtual in the /// constructor and it does not override it, but derived classes could. /// internal sealed class TemplatedAdorner: Adorner { private Control _child; ////// The clear the single child of a TemplatedAdorner /// public void ClearChild() { this.RemoveVisualChild(_child); _child = null; } public TemplatedAdorner(UIElement adornedElement, ControlTemplate adornerTemplate) : base(adornedElement) { Debug.Assert(adornedElement != null, "adornedElement should not be null"); Debug.Assert(adornerTemplate != null, "adornerTemplate should not be null"); Control control = new Control(); control.DataContext = Validation.GetErrors(adornedElement); //control.IsEnabled = false; // Hittest should not work on visual subtree control.Template = adornerTemplate; _child = control; this.AddVisualChild(_child); } ////// Adorners don't always want to be transformed in the same way as the elements they /// adorn. Adorners which adorn points, such as resize handles, want to be translated /// and rotated but not scaled. Adorners adorning an object, like a marquee, may want /// all transforms. This method is called by AdornerLayer to allow the adorner to /// filter out the transforms it doesn't want and return a new transform with just the /// transforms it wants applied. An adorner can also add an additional translation /// transform at this time, allowing it to be positioned somewhere other than the upper /// left corner of its adorned element. /// /// The transform applied to the object the adorner adorns ///Transform to apply to the adorner public override GeneralTransform GetDesiredTransform(GeneralTransform transform) { if (ReferenceElement == null) return transform; GeneralTransformGroup group = new GeneralTransformGroup(); group.Children.Add(transform); GeneralTransform t = this.TransformToDescendant(ReferenceElement); if (t != null) { group.Children.Add(t); } return group; } public FrameworkElement ReferenceElement { get { return _referenceElement; } set { _referenceElement = value; } } ////// Derived class must implement to support Visual children. The method must return /// the child at the specified index. Index must be between 0 and GetVisualChildrenCount-1. /// /// By default a Visual does not have any children. /// /// Remark: /// During this virtual call it is not valid to modify the Visual tree. /// protected override Visual GetVisualChild(int index) { if(_child == null || index != 0) { throw new ArgumentOutOfRangeException("index", index, SR.Get(SRID.Visual_ArgumentOutOfRange)); } return _child; } ////// Derived classes override this property to enable the Visual code to enumerate /// the Visual children. Derived classes need to return the number of children /// from this method. /// /// By default a Visual does not have any children. /// /// Remark: During this virtual method the Visual tree must not be modified. /// protected override int VisualChildrenCount { get { return _child != null ? 1 : 0; } } ////// Measure adorner. Default behavior is to size to match the adorned element. /// protected override Size MeasureOverride(Size constraint) { Debug.Assert(_child != null, "_child should not be null"); if (ReferenceElement != null && AdornedElement != null && AdornedElement.IsMeasureValid && !DoubleUtil.AreClose(ReferenceElement.DesiredSize, AdornedElement.DesiredSize) ) { ReferenceElement.InvalidateMeasure(); } (_child).Measure(constraint); return (_child).DesiredSize; } ////// Default control arrangement is to only arrange /// the first visual child. No transforms will be applied. /// protected override Size ArrangeOverride(Size size) { Size finalSize; finalSize = base.ArrangeOverride(size); if (_child != null) { _child.Arrange(new Rect(new Point(), finalSize)); } return finalSize; } private FrameworkElement _referenceElement; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
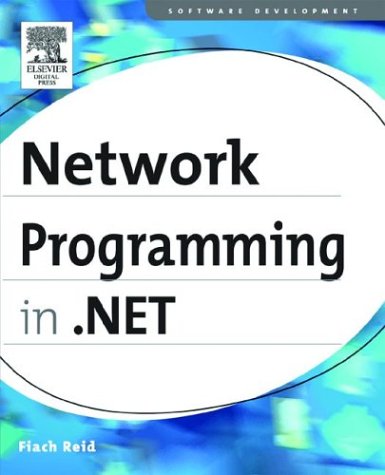
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UiaCoreApi.cs
- Speller.cs
- TrustManager.cs
- SolidColorBrush.cs
- SoapEnumAttribute.cs
- CheckPair.cs
- XmlUtf8RawTextWriter.cs
- WarningException.cs
- FrameworkElement.cs
- AnonymousIdentificationSection.cs
- DesignBindingValueUIHandler.cs
- PermissionSetEnumerator.cs
- XmlNodeList.cs
- XPathException.cs
- OutputCacheProviderCollection.cs
- SubMenuStyleCollection.cs
- CuspData.cs
- CompositeScriptReferenceEventArgs.cs
- CodeSubDirectoriesCollection.cs
- ListItem.cs
- RectangleConverter.cs
- DataColumn.cs
- HybridWebProxyFinder.cs
- SessionParameter.cs
- ToolStripContentPanelRenderEventArgs.cs
- ColorDialog.cs
- ContentDisposition.cs
- MultiView.cs
- TransformCollection.cs
- XmlSerializationGeneratedCode.cs
- WebControlsSection.cs
- DrawListViewItemEventArgs.cs
- Normalization.cs
- DictionaryEntry.cs
- recordstatefactory.cs
- EnterpriseServicesHelper.cs
- GlobalEventManager.cs
- ControlPaint.cs
- ToolTipAutomationPeer.cs
- TemplateColumn.cs
- ActivitySurrogate.cs
- GraphicsContainer.cs
- BitmapEffectOutputConnector.cs
- TraceHelpers.cs
- Perspective.cs
- XmlNavigatorStack.cs
- DataGridCaption.cs
- ProviderUtil.cs
- SortDescription.cs
- SqlSupersetValidator.cs
- ProtectedConfiguration.cs
- XmlSchemaObject.cs
- MasterPage.cs
- CatchBlock.cs
- IndexOutOfRangeException.cs
- FixedTextBuilder.cs
- TypeUsageBuilder.cs
- XmlSchemaSequence.cs
- AsyncPostBackErrorEventArgs.cs
- BamlStream.cs
- CompiledAction.cs
- cache.cs
- DeflateStream.cs
- BypassElement.cs
- DesignerOptionService.cs
- ActiveXSite.cs
- PlaceHolder.cs
- ParseElementCollection.cs
- DataListItem.cs
- Int64Storage.cs
- XmlProcessingInstruction.cs
- TransformConverter.cs
- DbMetaDataFactory.cs
- regiisutil.cs
- Debug.cs
- FileDialog.cs
- BuildResult.cs
- DefaultObjectSerializer.cs
- InputBinding.cs
- ColorKeyFrameCollection.cs
- HttpHandlersSection.cs
- BoundField.cs
- SafeTokenHandle.cs
- CompilerGeneratedAttribute.cs
- UIElement3D.cs
- OrderedEnumerableRowCollection.cs
- MdiWindowListItemConverter.cs
- AttributeAction.cs
- FusionWrap.cs
- TransformDescriptor.cs
- ButtonPopupAdapter.cs
- ImageAnimator.cs
- ImageButton.cs
- ParameterCollection.cs
- ZoomPercentageConverter.cs
- XmlSequenceWriter.cs
- HtmlString.cs
- UiaCoreProviderApi.cs
- XmlSchemaFacet.cs
- DefaultHttpHandler.cs