Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / Microsoft / Scripting / Ast / CatchBlock.cs / 1305376 / CatchBlock.cs
/* **************************************************************************** * * Copyright (c) Microsoft Corporation. * * This source code is subject to terms and conditions of the Microsoft Public License. A * copy of the license can be found in the License.html file at the root of this distribution. If * you cannot locate the Microsoft Public License, please send an email to * dlr@microsoft.com. By using this source code in any fashion, you are agreeing to be bound * by the terms of the Microsoft Public License. * * You must not remove this notice, or any other, from this software. * * * ***************************************************************************/ using System.Diagnostics; using System.Dynamic.Utils; #if SILVERLIGHT using System.Core; #endif namespace System.Linq.Expressions { ////// Represents a catch statement in a try block. /// This must have the same return type (i.e., the type of #if !SILVERLIGHT [DebuggerTypeProxy(typeof(Expression.CatchBlockProxy))] #endif public sealed class CatchBlock { private readonly Type _test; private readonly ParameterExpression _var; private readonly Expression _body; private readonly Expression _filter; internal CatchBlock(Type test, ParameterExpression variable, Expression body, Expression filter) { _test = test; _var = variable; _body = body; _filter = filter; } ///) as the try block it is associated with. /// /// Gets a reference to the public ParameterExpression Variable { get { return _var; } } ///object caught by this handler. /// /// Gets the type of public Type Test { get { return _test; } } ///this handler catches. /// /// Gets the body of the catch block. /// public Expression Body { get { return _body; } } ////// Gets the body of the public Expression Filter { get { return _filter; } } ///'s filter. /// /// Returns a ///that represents the current . /// A public override string ToString() { return ExpressionStringBuilder.CatchBlockToString(this); } ///that represents the current . /// Creates a new expression that is like this one, but using the /// supplied children. If all of the children are the same, it will /// return this expression. /// /// Theproperty of the result. /// The property of the result. /// The property of the result. /// This expression if no children changed, or an expression with the updated children. public CatchBlock Update(ParameterExpression variable, Expression filter, Expression body) { if (variable == Variable && filter == Filter && body == Body) { return this; } return Expression.MakeCatchBlock(Test, variable, body, filter); } } public partial class Expression { ////// Creates a /// Therepresenting a catch statement. /// The of object to be caught can be specified but no reference to the object /// will be available for use in the . /// of this will handle. /// The body of the catch statement. /// The created public static CatchBlock Catch(Type type, Expression body) { return MakeCatchBlock(type, null, body, null); } ///. /// Creates a /// Arepresenting a catch statement with a reference to the caught object for use in the handler body. /// representing a reference to the object caught by this handler. /// The body of the catch statement. /// The created public static CatchBlock Catch(ParameterExpression variable, Expression body) { ContractUtils.RequiresNotNull(variable, "variable"); return MakeCatchBlock(variable.Type, variable, body, null); } ///. /// Creates a /// Therepresenting a catch statement with /// an filter but no reference to the caught object. /// of this will handle. /// The body of the catch statement. /// The body of the filter. /// The created public static CatchBlock Catch(Type type, Expression body, Expression filter) { return MakeCatchBlock(type, null, body, filter); } ///. /// Creates a /// Arepresenting a catch statement with /// an filter and a reference to the caught object. /// representing a reference to the object caught by this handler. /// The body of the catch statement. /// The body of the filter. /// The created public static CatchBlock Catch(ParameterExpression variable, Expression body, Expression filter) { ContractUtils.RequiresNotNull(variable, "variable"); return MakeCatchBlock(variable.Type, variable, body, filter); } ///. /// Creates a /// Therepresenting a catch statement with the specified elements. /// of this will handle. /// A representing a reference to the object caught by this handler. /// The body of the catch statement. /// The body of the filter. /// The created ///. public static CatchBlock MakeCatchBlock(Type type, ParameterExpression variable, Expression body, Expression filter) { ContractUtils.RequiresNotNull(type, "type"); ContractUtils.Requires(variable == null || TypeUtils.AreEquivalent(variable.Type, type), "variable"); if (variable != null && variable.IsByRef) { throw Error.VariableMustNotBeByRef(variable, variable.Type); } RequiresCanRead(body, "body"); if (filter != null) { RequiresCanRead(filter, "filter"); if (filter.Type != typeof(bool)) throw Error.ArgumentMustBeBoolean(); } return new CatchBlock(type, variable, body, filter); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. must be non-null and match the type of (if it is supplied).
Link Menu
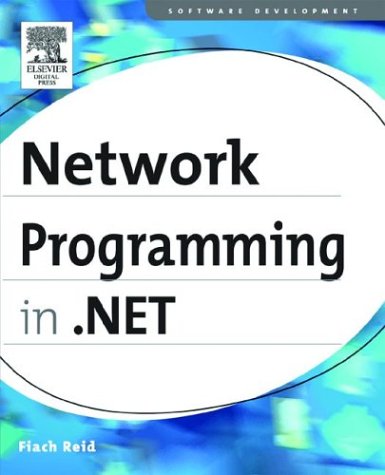
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BufferedReadStream.cs
- ObjectViewEntityCollectionData.cs
- BufferedReadStream.cs
- XmlSubtreeReader.cs
- DelegatedStream.cs
- SymbolMethod.cs
- TransformerConfigurationWizardBase.cs
- IssuedTokenParametersElement.cs
- IndexedString.cs
- DateTimeConverter2.cs
- securitymgrsite.cs
- TimeSpanSecondsConverter.cs
- TreeNodeStyleCollection.cs
- GeneralTransform3DCollection.cs
- GetRecipientListRequest.cs
- UnsafeCollabNativeMethods.cs
- LightweightCodeGenerator.cs
- TraceHandler.cs
- EdmToObjectNamespaceMap.cs
- StringKeyFrameCollection.cs
- X509UI.cs
- TextSelectionHighlightLayer.cs
- ToolStripManager.cs
- CultureInfo.cs
- DataServices.cs
- UInt64Storage.cs
- RbTree.cs
- DbDataSourceEnumerator.cs
- DateTimeOffset.cs
- StaticSiteMapProvider.cs
- DoubleKeyFrameCollection.cs
- XpsResourceDictionary.cs
- Bold.cs
- DbConnectionPoolCounters.cs
- PropertiesTab.cs
- EventPropertyMap.cs
- _IPv6Address.cs
- DataGridTextColumn.cs
- UInt64.cs
- MetadataFile.cs
- StoragePropertyMapping.cs
- xmlformatgeneratorstatics.cs
- DiagnosticsConfigurationHandler.cs
- SettingsProperty.cs
- __Filters.cs
- StylusDevice.cs
- OdbcEnvironment.cs
- StreamWithDictionary.cs
- BindableAttribute.cs
- XmlDataProvider.cs
- ComAdminInterfaces.cs
- RepeatButtonAutomationPeer.cs
- OrderedEnumerableRowCollection.cs
- UnknownWrapper.cs
- FixedNode.cs
- DiscoveryMessageSequence11.cs
- ConnectionStringsExpressionBuilder.cs
- ActivityPropertyReference.cs
- NegotiationTokenAuthenticatorStateCache.cs
- BooleanStorage.cs
- DelimitedListTraceListener.cs
- WebServicesSection.cs
- EntityContainerEntitySet.cs
- ScriptDescriptor.cs
- PageAsyncTask.cs
- XmlStreamNodeWriter.cs
- ExpandedWrapper.cs
- CryptographicAttribute.cs
- TouchFrameEventArgs.cs
- AssemblyFilter.cs
- QueryCacheEntry.cs
- SqlCacheDependencySection.cs
- SharedPerformanceCounter.cs
- ExternalException.cs
- BaseCodePageEncoding.cs
- ExpandCollapseProviderWrapper.cs
- FixedStringLookup.cs
- AuthenticatedStream.cs
- CryptoApi.cs
- WindowsUpDown.cs
- TextParentUndoUnit.cs
- XmlSchemaAll.cs
- MethodBuilderInstantiation.cs
- StrokeNodeData.cs
- SqlDataSourceConnectionPanel.cs
- XmlSchemaSequence.cs
- ImpersonateTokenRef.cs
- GuidTagList.cs
- ResourcesGenerator.cs
- EntityContainer.cs
- KernelTypeValidation.cs
- WorkflowServiceNamespace.cs
- XmlSignatureProperties.cs
- FolderLevelBuildProvider.cs
- ManualResetEvent.cs
- TypeToken.cs
- BindingManagerDataErrorEventArgs.cs
- GlobalAllocSafeHandle.cs
- TreeNodeClickEventArgs.cs
- CFStream.cs