Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Shared / MS / Internal / SafeSecurityHelper.cs / 2 / SafeSecurityHelper.cs
/****************************************************************************\ * * File: SafeSecurityHelper.cs * * Purpose: Helper functions that require elevation but are safe to use. * * History: * 10/15/04: [....] Created * * Copyright (C) 2004 by Microsoft Corporation. All rights reserved. * \***************************************************************************/ using System; using System.Globalization; using System.Security; using System.Security.Permissions; using System.Reflection; using MS.Win32; using System.Runtime.InteropServices; using Microsoft.Win32; #if PRESENTATIONFRAMEWORK using System.Windows; using System.Windows.Media; #endif // The SafeSecurityHelper class differs between assemblies and could not actually be // shared, so it is duplicated across namespaces to prevent name collision. #if WINDOWS_BASE namespace MS.Internal.WindowsBase #elif PRESENTATION_CORE namespace MS.Internal.PresentationCore #elif PRESENTATIONFRAMEWORK namespace MS.Internal.PresentationFramework #elif REACHFRAMEWORK namespace MS.Internal.ReachFramework #elif DRT namespace MS.Internal.Drt #else #error Class is being used from an unknown assembly. #endif { internal static partial class SafeSecurityHelper { #if PRESENTATION_CORE ////// Given a rectangle with coords in local screen coordinates. /// Return the rectangle in global screen coordinates. /// ////// Critical - calls a critical function. /// TreatAsSafe - handing out a transformed rect is considered safe. /// /// Although we are calling a function that passes an array, and no. of elements. /// We limit this call to only call this function with a 2 as the count of elements. /// Thereby containing any potential for the call to Win32 to cause a buffer overrun. /// [SecurityCritical, SecurityTreatAsSafe ] internal static void TransformLocalRectToScreen(HandleRef hwnd, ref NativeMethods.RECT rcWindowCoords) { int retval = MapWindowPoints(hwnd , new HandleRef(null, IntPtr.Zero), ref rcWindowCoords, 2); int win32Err = Marshal.GetLastWin32Error(); if(retval == 0 && win32Err != 0) { throw new System.ComponentModel.Win32Exception(win32Err); } } #endif #if PRESENTATION_CORE || PRESENTATIONFRAMEWORK ||REACHFRAMEWORK || DEBUG #if !WINDOWS_BASE ////// Given an assembly, returns the partial name of the assembly. /// ////// This code used to perform an elevation but no longer needs to. /// The method is being kept in this class to ease auditing and /// should have a security review if changed. /// The string returned does not contain path information. /// This code is duplicated in SafeSecurityHelperPBT.cs. /// internal static string GetAssemblyPartialName(Assembly assembly) { AssemblyName name = new AssemblyName(assembly.FullName); string partialName = name.Name; return (partialName != null) ? partialName : String.Empty; } #endif #endif #if PRESENTATIONFRAMEWORK ////// Get the full assembly name by combining the partial name passed in /// with everything else from proto assembly. /// ////// This code used to perform an elevation but no longer needs to. /// The method is being kept in this class to ease auditing and /// should have a security review if changed. /// The string returned does not contain path information. /// internal static string GetFullAssemblyNameFromPartialName( Assembly protoAssembly, string partialName) { AssemblyName name = new AssemblyName(protoAssembly.FullName); name.Name = partialName; return name.FullName; } ////// Critical: This code accesses PresentationSource which is critical but does not /// expose it. /// TreatAsSafe: PresentationSource is not exposed and Client to Screen co-ordinates is /// safe to expose /// [SecurityCritical,SecurityTreatAsSafe] internal static Point ClientToScreen(UIElement relativeTo, Point point) { GeneralTransform transform; PresentationSource source = PresentationSource.CriticalFromVisual(relativeTo); if (source == null) { return new Point(double.NaN, double.NaN); } transform = relativeTo.TransformToAncestor(source.RootVisual); Point ptRoot; transform.TryTransform(point, out ptRoot); Point ptClient = PointUtil.RootToClient(ptRoot, source); Point ptScreen = PointUtil.ClientToScreen(ptClient, source); return ptScreen; } #endif // PRESENTATIONFRAMEWORK #if WINDOWS_BASE || PRESENTATION_CORE ////// This function iterates through the assemblies loaded in the current /// AppDomain and finds one that has the same assembly name passed in. /// ////// The method is being kept in this class to ease auditing and /// should have a security review if changed. /// /// WARNING! Don't use this method for retrievals of assemblies that /// should rely on a strong match with the given assembly name /// since this method allows assemblies with the same short name /// to be returned even when other name parts are different. /// /// E.g. ---- /// matches ----, Version=2.0.0.0, Culture=en-us, PublicKeyToken=b03f5f7f11d50a3a /// internal static Assembly GetLoadedAssembly(AssemblyName assemblyName) { Version reqVersion = assemblyName.Version; CultureInfo reqCulture = assemblyName.CultureInfo; byte[] reqKeyToken = assemblyName.GetPublicKeyToken(); Assembly[] assemblies = AppDomain.CurrentDomain.GetAssemblies(); for (int i = assemblies.Length - 1; i >= 0; i--) { // // We use to AssemblyName ctor here because GetName demands FileIOPermission // and does load more than just the required infpmation. // Essentially we use AssemblyName just to help parsing the name, version, culture // and public key token from the assembly's name. // AssemblyName curAsmName = new AssemblyName(assemblies[i].FullName); Version curVersion = curAsmName.Version; CultureInfo curCulture = curAsmName.CultureInfo; byte[] curKeyToken = curAsmName.GetPublicKeyToken(); if ( (String.Compare(curAsmName.Name, assemblyName.Name, true, DOTNETCULTURE) == 0) && (reqVersion == null || reqVersion.Equals(curVersion)) && (reqCulture == null || reqCulture.Equals(curCulture)) && (reqKeyToken == null || IsSameKeyToken(reqKeyToken, curKeyToken) ) ) { return assemblies[i]; } } return null; } // // Determine if two Public Key Tokens are the same. // private static bool IsSameKeyToken(byte[] reqKeyToken, byte[] curKeyToken) { bool isSame = false; if (reqKeyToken == null && curKeyToken == null) { // Both Key Tokens are not set, treat them as same. isSame = true; } else if (reqKeyToken != null && curKeyToken != null) { // Both KeyTokens are set. if (reqKeyToken.Length == curKeyToken.Length) { isSame = true; for (int i = 0; i < reqKeyToken.Length; i++) { if (reqKeyToken[i] != curKeyToken[i]) { isSame = false; break; } } } } return isSame; } #endif // WINDOWS_BASE || PRESENTATION_CORE #if PRESENTATION_CORE || PRESENTATIONFRAMEWORK // enum to choose between the various keys internal enum KeyToRead { WebBrowserDisable = 0x01 , MediaAudioDisable = 0x02 , MediaVideoDisable = 0x04 , MediaImageDisable = 0x08 , MediaAudioOrVideoDisable = KeyToRead.MediaVideoDisable | KeyToRead.MediaAudioDisable , } ////// Critical: This code elevates to access registry /// TreatAsSafe: The information it exposes is safe to give out and all it does is read a specific key /// [SecurityCritical,SecurityTreatAsSafe] internal static bool IsFeatureDisabled(KeyToRead key) { string regValue = null; bool fResult = false; switch (key) { case KeyToRead.WebBrowserDisable: regValue = RegistryKeys.value_WebBrowserDisallow; break; case KeyToRead.MediaAudioDisable: regValue = RegistryKeys.value_MediaAudioDisallow; break; case KeyToRead.MediaVideoDisable: regValue = RegistryKeys.value_MediaVideoDisallow; break; case KeyToRead.MediaImageDisable: regValue = RegistryKeys.value_MediaImageDisallow; break; case KeyToRead.MediaAudioOrVideoDisable: regValue = RegistryKeys.value_MediaAudioDisallow; break; default:// throw exception for invalid key throw(new System.ArgumentException(key.ToString())); } RegistryKey featureKey; //Assert for read access to HKLM\Software\Microsoft\Windows\Avalon RegistryPermission regPerm = new RegistryPermission(RegistryPermissionAccess.Read,"HKEY_LOCAL_MACHINE\\"+RegistryKeys.WPF_Features);//BlessedAssert regPerm.Assert();//BlessedAssert try { object obj = null; bool keyValue = false; // open the key and read the value featureKey = Registry.LocalMachine.OpenSubKey(RegistryKeys.WPF_Features); if (featureKey != null) { // If key exists and value is 1 return true else false obj = featureKey.GetValue(regValue); keyValue = obj is int && ((int)obj == 1); if (keyValue) { fResult = true; } // special case for audio and video since they can be orred // this is in the condition that audio is enabled since that is // the path that MediaAudioVideoDisable defaults to // This is purely to optimize perf on the number of calls to assert // in the media or audio scenario. if ((fResult == false) && (key == KeyToRead.MediaAudioOrVideoDisable)) { regValue = RegistryKeys.value_MediaVideoDisallow; // If key exists and value is 1 return true else false obj = featureKey.GetValue(regValue); keyValue = obj is int && ((int)obj == 1); if (keyValue) { fResult = true; } } } } finally { RegistryPermission.RevertAssert(); } return fResult; } #endif //PRESENTATIONCORE||PRESENTATIONFRAMEWORK #if PRESENTATION_CORE ////// This function is a wrapper for CultureInfo.GetCultureInfoByIetfLanguageTag(). /// The wrapper works around a bug in that routine, which causes it to throw /// a SecurityException in Partial Trust. VSWhidbey bug #572162. /// ////// Critical: This code elevates to access registry /// TreatAsSafe: The information it exposes is safe to give out and all it does is read a specific key /// [SecurityCritical,SecurityTreatAsSafe] static internal CultureInfo GetCultureInfoByIetfLanguageTag(string languageTag) { CultureInfo culture = null; RegistryPermission regPerm = new RegistryPermission(RegistryPermissionAccess.Read, RegistryKeys.HKLM_IetfLanguage);//BlessedAssert regPerm.Assert();//BlessedAssert try { culture = CultureInfo.GetCultureInfoByIetfLanguageTag(languageTag); } finally { RegistryPermission.RevertAssert(); } return culture; } #endif //PRESENTATIONCORE // // Private p-invokes. // #if !PRESENTATIONFRAMEWORK && !WINDOWS_BASE ////// Critical - this function elevates /// [SecurityCritical, SuppressUnmanagedCodeSecurity ] [DllImport(ExternDll.User32, SetLastError = true, ExactSpelling=true, CharSet=CharSet.Auto)] private static extern int MapWindowPoints(HandleRef hWndFrom, HandleRef hWndTo, [In, Out] ref NativeMethods.RECT rect, int cPoints); #endif internal static readonly CultureInfo DOTNETCULTURE = System.Windows.Markup.TypeConverterHelper.EnglishUSCulture; internal const string IMAGE = "image"; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. /****************************************************************************\ * * File: SafeSecurityHelper.cs * * Purpose: Helper functions that require elevation but are safe to use. * * History: * 10/15/04: [....] Created * * Copyright (C) 2004 by Microsoft Corporation. All rights reserved. * \***************************************************************************/ using System; using System.Globalization; using System.Security; using System.Security.Permissions; using System.Reflection; using MS.Win32; using System.Runtime.InteropServices; using Microsoft.Win32; #if PRESENTATIONFRAMEWORK using System.Windows; using System.Windows.Media; #endif // The SafeSecurityHelper class differs between assemblies and could not actually be // shared, so it is duplicated across namespaces to prevent name collision. #if WINDOWS_BASE namespace MS.Internal.WindowsBase #elif PRESENTATION_CORE namespace MS.Internal.PresentationCore #elif PRESENTATIONFRAMEWORK namespace MS.Internal.PresentationFramework #elif REACHFRAMEWORK namespace MS.Internal.ReachFramework #elif DRT namespace MS.Internal.Drt #else #error Class is being used from an unknown assembly. #endif { internal static partial class SafeSecurityHelper { #if PRESENTATION_CORE ////// Given a rectangle with coords in local screen coordinates. /// Return the rectangle in global screen coordinates. /// ////// Critical - calls a critical function. /// TreatAsSafe - handing out a transformed rect is considered safe. /// /// Although we are calling a function that passes an array, and no. of elements. /// We limit this call to only call this function with a 2 as the count of elements. /// Thereby containing any potential for the call to Win32 to cause a buffer overrun. /// [SecurityCritical, SecurityTreatAsSafe ] internal static void TransformLocalRectToScreen(HandleRef hwnd, ref NativeMethods.RECT rcWindowCoords) { int retval = MapWindowPoints(hwnd , new HandleRef(null, IntPtr.Zero), ref rcWindowCoords, 2); int win32Err = Marshal.GetLastWin32Error(); if(retval == 0 && win32Err != 0) { throw new System.ComponentModel.Win32Exception(win32Err); } } #endif #if PRESENTATION_CORE || PRESENTATIONFRAMEWORK ||REACHFRAMEWORK || DEBUG #if !WINDOWS_BASE ////// Given an assembly, returns the partial name of the assembly. /// ////// This code used to perform an elevation but no longer needs to. /// The method is being kept in this class to ease auditing and /// should have a security review if changed. /// The string returned does not contain path information. /// This code is duplicated in SafeSecurityHelperPBT.cs. /// internal static string GetAssemblyPartialName(Assembly assembly) { AssemblyName name = new AssemblyName(assembly.FullName); string partialName = name.Name; return (partialName != null) ? partialName : String.Empty; } #endif #endif #if PRESENTATIONFRAMEWORK ////// Get the full assembly name by combining the partial name passed in /// with everything else from proto assembly. /// ////// This code used to perform an elevation but no longer needs to. /// The method is being kept in this class to ease auditing and /// should have a security review if changed. /// The string returned does not contain path information. /// internal static string GetFullAssemblyNameFromPartialName( Assembly protoAssembly, string partialName) { AssemblyName name = new AssemblyName(protoAssembly.FullName); name.Name = partialName; return name.FullName; } ////// Critical: This code accesses PresentationSource which is critical but does not /// expose it. /// TreatAsSafe: PresentationSource is not exposed and Client to Screen co-ordinates is /// safe to expose /// [SecurityCritical,SecurityTreatAsSafe] internal static Point ClientToScreen(UIElement relativeTo, Point point) { GeneralTransform transform; PresentationSource source = PresentationSource.CriticalFromVisual(relativeTo); if (source == null) { return new Point(double.NaN, double.NaN); } transform = relativeTo.TransformToAncestor(source.RootVisual); Point ptRoot; transform.TryTransform(point, out ptRoot); Point ptClient = PointUtil.RootToClient(ptRoot, source); Point ptScreen = PointUtil.ClientToScreen(ptClient, source); return ptScreen; } #endif // PRESENTATIONFRAMEWORK #if WINDOWS_BASE || PRESENTATION_CORE ////// This function iterates through the assemblies loaded in the current /// AppDomain and finds one that has the same assembly name passed in. /// ////// The method is being kept in this class to ease auditing and /// should have a security review if changed. /// /// WARNING! Don't use this method for retrievals of assemblies that /// should rely on a strong match with the given assembly name /// since this method allows assemblies with the same short name /// to be returned even when other name parts are different. /// /// E.g. ---- /// matches ----, Version=2.0.0.0, Culture=en-us, PublicKeyToken=b03f5f7f11d50a3a /// internal static Assembly GetLoadedAssembly(AssemblyName assemblyName) { Version reqVersion = assemblyName.Version; CultureInfo reqCulture = assemblyName.CultureInfo; byte[] reqKeyToken = assemblyName.GetPublicKeyToken(); Assembly[] assemblies = AppDomain.CurrentDomain.GetAssemblies(); for (int i = assemblies.Length - 1; i >= 0; i--) { // // We use to AssemblyName ctor here because GetName demands FileIOPermission // and does load more than just the required infpmation. // Essentially we use AssemblyName just to help parsing the name, version, culture // and public key token from the assembly's name. // AssemblyName curAsmName = new AssemblyName(assemblies[i].FullName); Version curVersion = curAsmName.Version; CultureInfo curCulture = curAsmName.CultureInfo; byte[] curKeyToken = curAsmName.GetPublicKeyToken(); if ( (String.Compare(curAsmName.Name, assemblyName.Name, true, DOTNETCULTURE) == 0) && (reqVersion == null || reqVersion.Equals(curVersion)) && (reqCulture == null || reqCulture.Equals(curCulture)) && (reqKeyToken == null || IsSameKeyToken(reqKeyToken, curKeyToken) ) ) { return assemblies[i]; } } return null; } // // Determine if two Public Key Tokens are the same. // private static bool IsSameKeyToken(byte[] reqKeyToken, byte[] curKeyToken) { bool isSame = false; if (reqKeyToken == null && curKeyToken == null) { // Both Key Tokens are not set, treat them as same. isSame = true; } else if (reqKeyToken != null && curKeyToken != null) { // Both KeyTokens are set. if (reqKeyToken.Length == curKeyToken.Length) { isSame = true; for (int i = 0; i < reqKeyToken.Length; i++) { if (reqKeyToken[i] != curKeyToken[i]) { isSame = false; break; } } } } return isSame; } #endif // WINDOWS_BASE || PRESENTATION_CORE #if PRESENTATION_CORE || PRESENTATIONFRAMEWORK // enum to choose between the various keys internal enum KeyToRead { WebBrowserDisable = 0x01 , MediaAudioDisable = 0x02 , MediaVideoDisable = 0x04 , MediaImageDisable = 0x08 , MediaAudioOrVideoDisable = KeyToRead.MediaVideoDisable | KeyToRead.MediaAudioDisable , } ////// Critical: This code elevates to access registry /// TreatAsSafe: The information it exposes is safe to give out and all it does is read a specific key /// [SecurityCritical,SecurityTreatAsSafe] internal static bool IsFeatureDisabled(KeyToRead key) { string regValue = null; bool fResult = false; switch (key) { case KeyToRead.WebBrowserDisable: regValue = RegistryKeys.value_WebBrowserDisallow; break; case KeyToRead.MediaAudioDisable: regValue = RegistryKeys.value_MediaAudioDisallow; break; case KeyToRead.MediaVideoDisable: regValue = RegistryKeys.value_MediaVideoDisallow; break; case KeyToRead.MediaImageDisable: regValue = RegistryKeys.value_MediaImageDisallow; break; case KeyToRead.MediaAudioOrVideoDisable: regValue = RegistryKeys.value_MediaAudioDisallow; break; default:// throw exception for invalid key throw(new System.ArgumentException(key.ToString())); } RegistryKey featureKey; //Assert for read access to HKLM\Software\Microsoft\Windows\Avalon RegistryPermission regPerm = new RegistryPermission(RegistryPermissionAccess.Read,"HKEY_LOCAL_MACHINE\\"+RegistryKeys.WPF_Features);//BlessedAssert regPerm.Assert();//BlessedAssert try { object obj = null; bool keyValue = false; // open the key and read the value featureKey = Registry.LocalMachine.OpenSubKey(RegistryKeys.WPF_Features); if (featureKey != null) { // If key exists and value is 1 return true else false obj = featureKey.GetValue(regValue); keyValue = obj is int && ((int)obj == 1); if (keyValue) { fResult = true; } // special case for audio and video since they can be orred // this is in the condition that audio is enabled since that is // the path that MediaAudioVideoDisable defaults to // This is purely to optimize perf on the number of calls to assert // in the media or audio scenario. if ((fResult == false) && (key == KeyToRead.MediaAudioOrVideoDisable)) { regValue = RegistryKeys.value_MediaVideoDisallow; // If key exists and value is 1 return true else false obj = featureKey.GetValue(regValue); keyValue = obj is int && ((int)obj == 1); if (keyValue) { fResult = true; } } } } finally { RegistryPermission.RevertAssert(); } return fResult; } #endif //PRESENTATIONCORE||PRESENTATIONFRAMEWORK #if PRESENTATION_CORE ////// This function is a wrapper for CultureInfo.GetCultureInfoByIetfLanguageTag(). /// The wrapper works around a bug in that routine, which causes it to throw /// a SecurityException in Partial Trust. VSWhidbey bug #572162. /// ////// Critical: This code elevates to access registry /// TreatAsSafe: The information it exposes is safe to give out and all it does is read a specific key /// [SecurityCritical,SecurityTreatAsSafe] static internal CultureInfo GetCultureInfoByIetfLanguageTag(string languageTag) { CultureInfo culture = null; RegistryPermission regPerm = new RegistryPermission(RegistryPermissionAccess.Read, RegistryKeys.HKLM_IetfLanguage);//BlessedAssert regPerm.Assert();//BlessedAssert try { culture = CultureInfo.GetCultureInfoByIetfLanguageTag(languageTag); } finally { RegistryPermission.RevertAssert(); } return culture; } #endif //PRESENTATIONCORE // // Private p-invokes. // #if !PRESENTATIONFRAMEWORK && !WINDOWS_BASE ////// Critical - this function elevates /// [SecurityCritical, SuppressUnmanagedCodeSecurity ] [DllImport(ExternDll.User32, SetLastError = true, ExactSpelling=true, CharSet=CharSet.Auto)] private static extern int MapWindowPoints(HandleRef hWndFrom, HandleRef hWndTo, [In, Out] ref NativeMethods.RECT rect, int cPoints); #endif internal static readonly CultureInfo DOTNETCULTURE = System.Windows.Markup.TypeConverterHelper.EnglishUSCulture; internal const string IMAGE = "image"; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
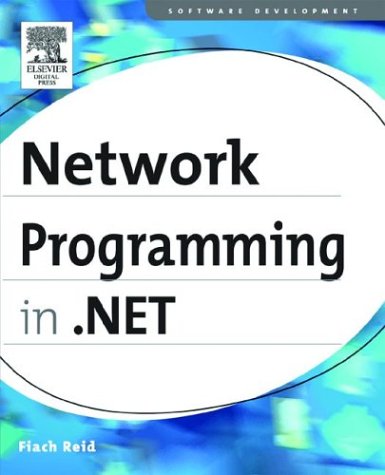
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FileLogRecordStream.cs
- ChannelBase.cs
- RegularExpressionValidator.cs
- XmlTextReaderImpl.cs
- OracleString.cs
- Automation.cs
- QuotedStringWriteStateInfo.cs
- UIElementCollection.cs
- SqlProvider.cs
- EventRouteFactory.cs
- ActivityDesignerAccessibleObject.cs
- TextRange.cs
- HitTestWithGeometryDrawingContextWalker.cs
- SlotInfo.cs
- HttpProcessUtility.cs
- XmlSchemaSequence.cs
- SmtpAuthenticationManager.cs
- UserNamePasswordValidator.cs
- SaveFileDialogDesigner.cs
- SQLDouble.cs
- ListCommandEventArgs.cs
- Transform.cs
- ToolStripArrowRenderEventArgs.cs
- Decimal.cs
- EntityDataSourceWrapperCollection.cs
- MulticastNotSupportedException.cs
- DesignTable.cs
- UndoEngine.cs
- OrderPreservingSpoolingTask.cs
- XmlILTrace.cs
- DataGridViewSelectedRowCollection.cs
- HighlightVisual.cs
- UserControl.cs
- XmlSchemaFacet.cs
- ApplicationFileParser.cs
- ByeOperationAsyncResult.cs
- KeyInterop.cs
- Timer.cs
- DirectoryObjectSecurity.cs
- CapiSymmetricAlgorithm.cs
- DesignerEditorPartChrome.cs
- CheckBoxStandardAdapter.cs
- CultureMapper.cs
- BaseHashHelper.cs
- ErrorHandler.cs
- Compensation.cs
- RegexGroup.cs
- TakeQueryOptionExpression.cs
- MailMessage.cs
- CalendarButton.cs
- XsltSettings.cs
- ColumnResult.cs
- CreateUserWizardStep.cs
- PlanCompilerUtil.cs
- DecoderReplacementFallback.cs
- TagMapCollection.cs
- Localizer.cs
- NamedPermissionSet.cs
- KnownBoxes.cs
- LoadedOrUnloadedOperation.cs
- AbstractDataSvcMapFileLoader.cs
- SafeLibraryHandle.cs
- ThemeInfoAttribute.cs
- DataGridViewColumn.cs
- TextReader.cs
- TraceSection.cs
- PeerContact.cs
- ThumbAutomationPeer.cs
- SID.cs
- CodeDirectiveCollection.cs
- BitVec.cs
- XmlExtensionFunction.cs
- BeginEvent.cs
- TimeSpanValidator.cs
- BitmapCacheBrush.cs
- ProfileSettingsCollection.cs
- SqlVersion.cs
- DecimalSumAggregationOperator.cs
- InputReportEventArgs.cs
- Assert.cs
- DateBoldEvent.cs
- DataException.cs
- HTTPNotFoundHandler.cs
- ContentType.cs
- AssemblyBuilder.cs
- SafeNativeMethodsCLR.cs
- TableSectionStyle.cs
- AnimatedTypeHelpers.cs
- BindingGroup.cs
- ValidatingPropertiesEventArgs.cs
- ListViewGroup.cs
- ISFTagAndGuidCache.cs
- DataGridViewCellStyleConverter.cs
- FormsIdentity.cs
- TypeLibConverter.cs
- OperationAbortedException.cs
- TextTreeInsertElementUndoUnit.cs
- DataViewManagerListItemTypeDescriptor.cs
- DependencyObject.cs
- CapabilitiesState.cs