Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / Compilation / SourceFileBuildProvider.cs / 1305376 / SourceFileBuildProvider.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Compilation { using System; using System.IO; using System.Collections; using System.CodeDom; using System.CodeDom.Compiler; using System.Web.Hosting; using System.Web.Util; using System.Web.UI; internal sealed class SourceFileBuildProvider: InternalBuildProvider { private CodeSnippetCompileUnit _snippetCompileUnit; private BuildProvider _owningBuildProvider; public override CompilerType CodeCompilerType { get { return CompilationUtil.GetCompilerInfoFromVirtualPath(VirtualPathObject); } } private void EnsureCodeCompileUnit() { if (_snippetCompileUnit == null) { // Read the contents of the file string sourceString = Util.StringFromVirtualPath(VirtualPathObject); _snippetCompileUnit = new CodeSnippetCompileUnit(sourceString); _snippetCompileUnit.LinePragma = BaseCodeDomTreeGenerator.CreateCodeLinePragmaHelper( VirtualPath, 1); } } public override void GenerateCode(AssemblyBuilder assemblyBuilder) { EnsureCodeCompileUnit(); assemblyBuilder.AddCodeCompileUnit(this, _snippetCompileUnit); } protected internal override CodeCompileUnit GetCodeCompileUnit(out IDictionary linePragmasTable) { EnsureCodeCompileUnit(); linePragmasTable = new Hashtable(); linePragmasTable[1] = _snippetCompileUnit.LinePragma; return _snippetCompileUnit; } // The owning build provider in case this course file is a partial compile-with code besides internal BuildProvider OwningBuildProvider { get { return _owningBuildProvider; } set { _owningBuildProvider = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Compilation { using System; using System.IO; using System.Collections; using System.CodeDom; using System.CodeDom.Compiler; using System.Web.Hosting; using System.Web.Util; using System.Web.UI; internal sealed class SourceFileBuildProvider: InternalBuildProvider { private CodeSnippetCompileUnit _snippetCompileUnit; private BuildProvider _owningBuildProvider; public override CompilerType CodeCompilerType { get { return CompilationUtil.GetCompilerInfoFromVirtualPath(VirtualPathObject); } } private void EnsureCodeCompileUnit() { if (_snippetCompileUnit == null) { // Read the contents of the file string sourceString = Util.StringFromVirtualPath(VirtualPathObject); _snippetCompileUnit = new CodeSnippetCompileUnit(sourceString); _snippetCompileUnit.LinePragma = BaseCodeDomTreeGenerator.CreateCodeLinePragmaHelper( VirtualPath, 1); } } public override void GenerateCode(AssemblyBuilder assemblyBuilder) { EnsureCodeCompileUnit(); assemblyBuilder.AddCodeCompileUnit(this, _snippetCompileUnit); } protected internal override CodeCompileUnit GetCodeCompileUnit(out IDictionary linePragmasTable) { EnsureCodeCompileUnit(); linePragmasTable = new Hashtable(); linePragmasTable[1] = _snippetCompileUnit.LinePragma; return _snippetCompileUnit; } // The owning build provider in case this course file is a partial compile-with code besides internal BuildProvider OwningBuildProvider { get { return _owningBuildProvider; } set { _owningBuildProvider = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
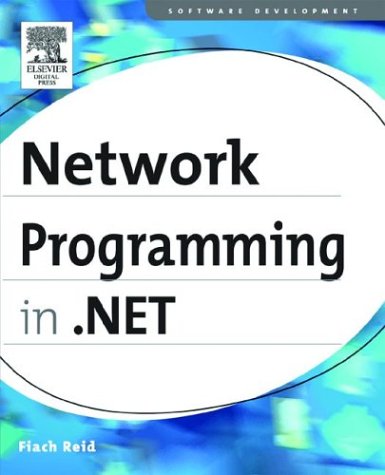
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ImagingCache.cs
- ParameterCollection.cs
- NativeMethods.cs
- NonVisualControlAttribute.cs
- PrimaryKeyTypeConverter.cs
- WS2007HttpBindingCollectionElement.cs
- ProviderBase.cs
- WindowsSecurityToken.cs
- SecurityHelper.cs
- SemanticValue.cs
- DrawingBrush.cs
- CommentEmitter.cs
- DataGridTextBoxColumn.cs
- ManipulationBoundaryFeedbackEventArgs.cs
- InkCanvasSelection.cs
- OleDbConnection.cs
- CustomAttributeSerializer.cs
- SmiEventSink_Default.cs
- ActivityMarkupSerializer.cs
- Fonts.cs
- EntityException.cs
- CreateUserWizardStep.cs
- MimeImporter.cs
- Types.cs
- ReliableChannelListener.cs
- ImageClickEventArgs.cs
- CollectionContainer.cs
- WebBaseEventKeyComparer.cs
- SpellerStatusTable.cs
- _Events.cs
- WorkflowDefinitionDispenser.cs
- FloaterBaseParaClient.cs
- PersistenceTypeAttribute.cs
- ContextInformation.cs
- CodeTypeDeclarationCollection.cs
- ListViewGroupItemCollection.cs
- Root.cs
- DoubleLinkListEnumerator.cs
- returneventsaver.cs
- HttpStreamMessageEncoderFactory.cs
- TerminateSequenceResponse.cs
- AssemblyAttributes.cs
- AmbientValueAttribute.cs
- SystemThemeKey.cs
- StylusDevice.cs
- PropertyTab.cs
- RelationshipType.cs
- XmlResolver.cs
- RsaKeyIdentifierClause.cs
- ExpressionBindings.cs
- LogicalMethodInfo.cs
- CompensatableTransactionScopeActivity.cs
- Variable.cs
- FixedBufferAttribute.cs
- addressfiltermode.cs
- StorageMappingItemCollection.cs
- Rights.cs
- XmlSchemaDocumentation.cs
- XsltLoader.cs
- PointLightBase.cs
- EdmToObjectNamespaceMap.cs
- Timer.cs
- XmlSchemaElement.cs
- CompilerError.cs
- Queue.cs
- KeyValueConfigurationElement.cs
- PtsContext.cs
- SolidColorBrush.cs
- GridItemProviderWrapper.cs
- ILGenerator.cs
- XmlDocumentSerializer.cs
- WebPartHelpVerb.cs
- ManipulationStartingEventArgs.cs
- BinaryMethodMessage.cs
- WebPartManager.cs
- ReflectionUtil.cs
- FormViewRow.cs
- SaveFileDialog.cs
- InvalidProgramException.cs
- ToolZoneDesigner.cs
- SortedList.cs
- SecurityRuntime.cs
- TCEAdapterGenerator.cs
- DelegatingTypeDescriptionProvider.cs
- ImpersonateTokenRef.cs
- InfoCardRSAOAEPKeyExchangeDeformatter.cs
- InvalidProgramException.cs
- BypassElement.cs
- PermissionSetTriple.cs
- RtfToXamlReader.cs
- Selection.cs
- WebPartUserCapability.cs
- CellConstantDomain.cs
- ContractAdapter.cs
- EntityDataSourceWrapperPropertyDescriptor.cs
- PostBackOptions.cs
- EntityContainer.cs
- Simplifier.cs
- ScrollChangedEventArgs.cs
- Vector3dCollection.cs