Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / Media3D / MaterialGroup.cs / 1 / MaterialGroup.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Material group // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Diagnostics; using System.Windows; using System.Windows.Media; using System.Windows.Media.Media3D; using System.Windows.Markup; using MS.Internal; using MS.Internal.Media3D; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Media3D { ////// Material group /// [ContentProperty("Children")] public sealed partial class MaterialGroup : Material { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Default constructor. /// public MaterialGroup() {} #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods #endregion Public Methods //------------------------------------------------------ // // Internal Methods // //------------------------------------------------------ #region Internal Methods // If a child changes or is added/removed to this, // that will fire changed which will mark the GeometryModel3D // containing this as DirtyForPreCompute which will // cause PreCompute() to be called on us. // // Thus, we don't need to override OnChanged() and update // _requiresRealization here in MaterialGroup. internal override bool PreCompute() { _requiresRealization = false; MaterialCollection children = Children; if (children != null) { int count = children.Count; for (int i = 0; i < count; i++) { // Can't early exit on first 'true' because PreCompute // does work that's necessary for every Material that // has text in it _requiresRealization |= children.Internal_GetItem(i).PreCompute(); } } return _requiresRealization; } internal override void UpdateRealizations( RealizationContext ctx, ref Rect bounds ) { Debug.Assert(_requiresRealization); MaterialCollection children = Children; if (children != null) { int count = children.Count; for (int i = 0; i < count; i++) { Material material = children.Internal_GetItem(i); if (material.RequiresRealization) { material.UpdateRealizations(ctx, ref bounds); } } } } #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //------------------------------------------------------ #region Internal Properties internal override bool RequiresRealization { get { return _requiresRealization; } } #endregion Internal Properties //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields private bool _requiresRealization = false; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
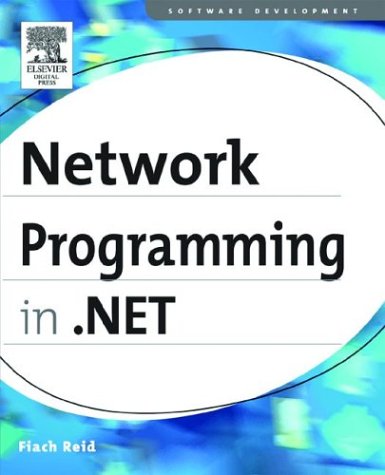
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TransformCryptoHandle.cs
- UpdateCommand.cs
- CompileXomlTask.cs
- AccessViolationException.cs
- TextWriter.cs
- PackageDigitalSignature.cs
- TdsParameterSetter.cs
- ActiveXHelper.cs
- DotExpr.cs
- CustomAttributeFormatException.cs
- IconConverter.cs
- Token.cs
- RadioButton.cs
- SortExpressionBuilder.cs
- EntityContainerAssociationSetEnd.cs
- followingquery.cs
- QilFactory.cs
- DiscreteKeyFrames.cs
- NamedPipeProcessProtocolHandler.cs
- DocumentManager.cs
- ServiceTimeoutsElement.cs
- TemplateContentLoader.cs
- MediaPlayer.cs
- WebAdminConfigurationHelper.cs
- ToolStripLabel.cs
- ValidatorUtils.cs
- ZoomPercentageConverter.cs
- PointConverter.cs
- WpfWebRequestHelper.cs
- AttachedPropertyBrowsableAttribute.cs
- FillRuleValidation.cs
- InputBinder.cs
- RepeaterDesigner.cs
- SystemNetworkInterface.cs
- IsolatedStorage.cs
- FileEnumerator.cs
- SplineKeyFrames.cs
- Point3DKeyFrameCollection.cs
- TypeLibConverter.cs
- EventLog.cs
- AtomicFile.cs
- SystemException.cs
- InvalidPropValue.cs
- SqlDataSourceCache.cs
- RemotingException.cs
- URLString.cs
- ListItemParagraph.cs
- DataSourceXmlTextReader.cs
- EndpointConfigContainer.cs
- ReadOnlyHierarchicalDataSourceView.cs
- ConfigurationProperty.cs
- SystemWebExtensionsSectionGroup.cs
- HttpCookiesSection.cs
- Utility.cs
- SharedUtils.cs
- PointCollection.cs
- ServiceModelSecurityTokenTypes.cs
- MLangCodePageEncoding.cs
- BooleanSwitch.cs
- UpdateTracker.cs
- HandlerFactoryWrapper.cs
- ThreadPoolTaskScheduler.cs
- SimpleType.cs
- UserControlParser.cs
- TableLayoutSettings.cs
- NumericUpDown.cs
- ExpressionBuilderCollection.cs
- XmlUTF8TextReader.cs
- EnumerableCollectionView.cs
- DataGridViewToolTip.cs
- CompareInfo.cs
- PrimitiveXmlSerializers.cs
- WinInet.cs
- BuildProviderAppliesToAttribute.cs
- WebPartTransformer.cs
- Int32Storage.cs
- RegexRunnerFactory.cs
- ListViewTableRow.cs
- StateWorkerRequest.cs
- WindowsListViewGroupHelper.cs
- CompilationPass2Task.cs
- CheckBox.cs
- ChameleonKey.cs
- ServiceModelConfigurationSectionCollection.cs
- QueryInterceptorAttribute.cs
- NamedElement.cs
- CacheOutputQuery.cs
- Range.cs
- DataObjectSettingDataEventArgs.cs
- BindingValueChangedEventArgs.cs
- HandlerBase.cs
- OleDbConnection.cs
- SafeBitVector32.cs
- PathBox.cs
- DataBoundLiteralControl.cs
- XmlTypeAttribute.cs
- SkewTransform.cs
- Quack.cs
- Token.cs
- CurrentTimeZone.cs