Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / UI / WebControls / unitconverter.cs / 1 / unitconverter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Globalization; using System.Reflection; using System.Security.Permissions; using System.Web.Util; [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class UnitConverter : TypeConverter { ////// /// Returns a value indicating whether the unit converter can /// convert from the specified source type. /// public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) { return true; } else { return base.CanConvertFrom(context, sourceType); } } ////// /// Returns a value indicating whether the converter can /// convert to the specified destination type. /// public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if ((destinationType == typeof(string)) || (destinationType == typeof(InstanceDescriptor))) { return true; } else { return base.CanConvertTo(context, destinationType); } } ////// /// Performs type conversion from the given value into a Unit. /// public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { if (value == null) return null; string stringValue = value as string; if (stringValue != null) { string textValue = stringValue.Trim(); if (textValue.Length == 0) { return Unit.Empty; } if (culture != null) { return Unit.Parse(textValue, culture); } else { return Unit.Parse(textValue, CultureInfo.CurrentCulture); } } else { return base.ConvertFrom(context, culture, value); } } ////// /// Performs type conversion to the specified destination type /// public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == typeof(string)) { if ((value == null) || ((Unit)value).IsEmpty) return String.Empty; else return ((Unit)value).ToString(culture); } else if ((destinationType == typeof(InstanceDescriptor)) && (value != null)) { Unit u = (Unit)value; MemberInfo member = null; object[] args = null; if (u.IsEmpty) { member = typeof(Unit).GetField("Empty"); } else { member = typeof(Unit).GetConstructor(new Type[] { typeof(double), typeof(UnitType) }); args = new object[] { u.Value, u.Type }; } Debug.Assert(member != null, "Looks like we're missing Unit.Empty or Unit::ctor(double, UnitType)"); if (member != null) { return new InstanceDescriptor(member, args); } else { return null; } } else { return base.ConvertTo(context, culture, value, destinationType); } } } }
Link Menu
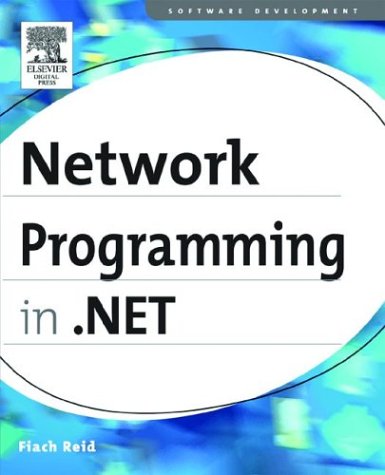
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ConstraintStruct.cs
- WebPartsPersonalizationAuthorization.cs
- WebEventCodes.cs
- KeyGestureConverter.cs
- httpstaticobjectscollection.cs
- WebPartAuthorizationEventArgs.cs
- ArraySortHelper.cs
- ImageSource.cs
- Content.cs
- XPathNavigatorKeyComparer.cs
- BinaryParser.cs
- StrokeCollectionConverter.cs
- ColorConvertedBitmapExtension.cs
- ExtentKey.cs
- ManagementOptions.cs
- ImageSourceValueSerializer.cs
- HttpApplication.cs
- SortedList.cs
- EntityStoreSchemaFilterEntry.cs
- Overlapped.cs
- WebPartTransformerAttribute.cs
- CryptoApi.cs
- MissingManifestResourceException.cs
- XmlNamespaceMapping.cs
- SoapAttributes.cs
- InputQueue.cs
- OracleParameterCollection.cs
- RemotingAttributes.cs
- XmlDownloadManager.cs
- XhtmlBasicValidatorAdapter.cs
- CalloutQueueItem.cs
- WebPartEditorCancelVerb.cs
- DependsOnAttribute.cs
- StorageAssociationTypeMapping.cs
- IgnorePropertiesAttribute.cs
- StrokeRenderer.cs
- SiteMapNodeItemEventArgs.cs
- PropertyNames.cs
- DataRelationCollection.cs
- AttachedPropertyBrowsableAttribute.cs
- TypeHelper.cs
- RuleConditionDialog.cs
- Rotation3D.cs
- MessageBox.cs
- QuaternionConverter.cs
- CacheOutputQuery.cs
- PartBasedPackageProperties.cs
- SlipBehavior.cs
- CompilerInfo.cs
- BitmapEffectInputConnector.cs
- ContainerSelectorGlyph.cs
- ContentPosition.cs
- Constraint.cs
- PlatformCulture.cs
- BatchWriter.cs
- ScriptControlDescriptor.cs
- EventListener.cs
- XmlSchemaProviderAttribute.cs
- ColumnClickEvent.cs
- GridErrorDlg.cs
- Trigger.cs
- ControlAdapter.cs
- ApplyImportsAction.cs
- DataExchangeServiceBinder.cs
- SoapProtocolImporter.cs
- DocumentPageHost.cs
- CheckStoreFileValidityRequest.cs
- DataIdProcessor.cs
- DoubleAnimation.cs
- XmlSchemaSet.cs
- Viewport3DVisual.cs
- LinqDataSourceView.cs
- DataGridViewCellValidatingEventArgs.cs
- RecognizedPhrase.cs
- ContainerFilterService.cs
- SmtpAuthenticationManager.cs
- XmlSchemaValidationException.cs
- ModuleBuilderData.cs
- CalendarDateRangeChangingEventArgs.cs
- ColorConverter.cs
- TextTreeUndo.cs
- ContextMenuStripGroup.cs
- DetailsViewPageEventArgs.cs
- ConfigurationCollectionAttribute.cs
- SpeakProgressEventArgs.cs
- PageParserFilter.cs
- RecognizerBase.cs
- DefaultSettingsSection.cs
- ApplicationSettingsBase.cs
- OdbcConnectionPoolProviderInfo.cs
- DataGridViewCellValidatingEventArgs.cs
- CategoryGridEntry.cs
- HtmlShimManager.cs
- WindowsAuthenticationModule.cs
- BinaryObjectReader.cs
- EmptyElement.cs
- TextBreakpoint.cs
- MDIClient.cs
- TemplateKeyConverter.cs
- EncryptedKey.cs