Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataWeb / Client / System / Data / Services / Client / ALinq / InputReferenceExpression.cs / 1 / InputReferenceExpression.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Respresents a reference to a resource set in a resource bound expression tree. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Client { using System; using System.Linq.Expressions; using System.Collections.Generic; using System.Diagnostics; ////// Represents a reference to a bound resource set in the resource path /// internal sealed class InputReferenceExpression : Expression { ///The resource or set referred to by this input reference expression private ResourceExpression target; ////// Constructs a new input reference expression that refers to the specified resource set /// /// The result type of this expression - must be the same as the element type of/// The target resource set that the new expression will reference internal InputReferenceExpression(Type inputElementType, ResourceExpression target) : base((ExpressionType)ResourceExpressionType.InputReference, inputElementType) { Debug.Assert(target != null, "Target resource set cannot be null"); Debug.Assert( inputElementType != null && ((target is NavigationPropertySingletonExpression) || inputElementType.Equals(((ResourceSetExpression)target).ResourceType)), "Invalid input element type"); this.target = target; } /// /// Retrieves the resource set referred to by this input reference expression /// internal ResourceExpression Target { get { return this.target; } } ////// Retargets this input reference to point to the resource set specified by /// The. /// that this input reference should use as its target internal void OverrideTarget(ResourceSetExpression newTarget) { Debug.Assert(newTarget != null, "Resource set cannot be null"); Debug.Assert(newTarget.ResourceType.Equals(this.Type), "Cannot reference a resource set with a different resource type"); this.target = newTarget; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // //// Respresents a reference to a resource set in a resource bound expression tree. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Client { using System; using System.Linq.Expressions; using System.Collections.Generic; using System.Diagnostics; ////// Represents a reference to a bound resource set in the resource path /// internal sealed class InputReferenceExpression : Expression { ///The resource or set referred to by this input reference expression private ResourceExpression target; ////// Constructs a new input reference expression that refers to the specified resource set /// /// The result type of this expression - must be the same as the element type of/// The target resource set that the new expression will reference internal InputReferenceExpression(Type inputElementType, ResourceExpression target) : base((ExpressionType)ResourceExpressionType.InputReference, inputElementType) { Debug.Assert(target != null, "Target resource set cannot be null"); Debug.Assert( inputElementType != null && ((target is NavigationPropertySingletonExpression) || inputElementType.Equals(((ResourceSetExpression)target).ResourceType)), "Invalid input element type"); this.target = target; } /// /// Retrieves the resource set referred to by this input reference expression /// internal ResourceExpression Target { get { return this.target; } } ////// Retargets this input reference to point to the resource set specified by /// The. /// that this input reference should use as its target internal void OverrideTarget(ResourceSetExpression newTarget) { Debug.Assert(newTarget != null, "Resource set cannot be null"); Debug.Assert(newTarget.ResourceType.Equals(this.Type), "Cannot reference a resource set with a different resource type"); this.target = newTarget; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
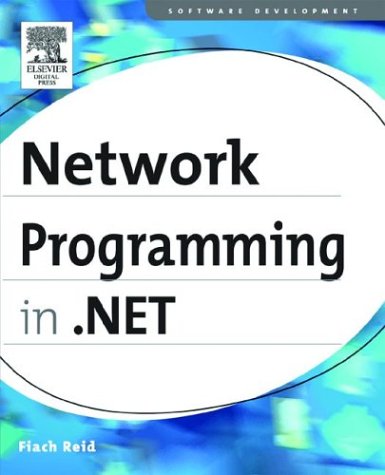
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Configuration.cs
- ChineseLunisolarCalendar.cs
- ClientSettings.cs
- HTTPNotFoundHandler.cs
- TextLineBreak.cs
- DesignTimeTemplateParser.cs
- GorillaCodec.cs
- messageonlyhwndwrapper.cs
- Triplet.cs
- CombinedHttpChannel.cs
- ThemeableAttribute.cs
- Base64Stream.cs
- IIS7WorkerRequest.cs
- XmlILConstructAnalyzer.cs
- ArgumentNullException.cs
- EntityCommandExecutionException.cs
- BitmapEffect.cs
- SingleBodyParameterMessageFormatter.cs
- DynamicILGenerator.cs
- EventWaitHandleSecurity.cs
- UInt64Converter.cs
- FirstMatchCodeGroup.cs
- GridViewCellAutomationPeer.cs
- SchemaImporterExtensionElement.cs
- LabelLiteral.cs
- ExpressionBuilder.cs
- AggregateException.cs
- DynamicQueryableWrapper.cs
- SignatureHelper.cs
- SByte.cs
- SerializerProvider.cs
- DataBindingHandlerAttribute.cs
- ListViewDesigner.cs
- PreProcessInputEventArgs.cs
- DataGridViewTextBoxColumn.cs
- MetadataProperty.cs
- AdjustableArrowCap.cs
- Enum.cs
- ProtocolImporter.cs
- FilteredAttributeCollection.cs
- TextEditorDragDrop.cs
- CompatibleComparer.cs
- DocumentGridContextMenu.cs
- TextFormatter.cs
- DetailsViewInsertedEventArgs.cs
- XmlCharType.cs
- XsdBuilder.cs
- CultureData.cs
- PopupRoot.cs
- HttpListenerElement.cs
- CommandLibraryHelper.cs
- ValidationResult.cs
- EventSourceCreationData.cs
- HandlerMappingMemo.cs
- BulletDecorator.cs
- ServerType.cs
- TextInfo.cs
- DbMetaDataFactory.cs
- sqlcontext.cs
- LogEntrySerialization.cs
- TypeInfo.cs
- __FastResourceComparer.cs
- PinnedBufferMemoryStream.cs
- ActivityBindForm.Designer.cs
- RealProxy.cs
- StreamGeometry.cs
- DeploymentSectionCache.cs
- MappingMetadataHelper.cs
- DataServiceRequest.cs
- DesignerExtenders.cs
- ProgressBarHighlightConverter.cs
- Win32Interop.cs
- ColumnBinding.cs
- ClientApiGenerator.cs
- ResourceCategoryAttribute.cs
- FontFaceLayoutInfo.cs
- NegotiationTokenAuthenticatorState.cs
- HttpCacheVary.cs
- FormatPage.cs
- FolderBrowserDialog.cs
- CheckBoxPopupAdapter.cs
- PropertyGridEditorPart.cs
- EventHandlerList.cs
- ObjectDataSourceDisposingEventArgs.cs
- CngKeyCreationParameters.cs
- ApplicationBuildProvider.cs
- VBIdentifierTrimConverter.cs
- WebBrowserSiteBase.cs
- DataGridViewRowHeightInfoPushedEventArgs.cs
- DelegatedStream.cs
- SecurityContext.cs
- Rotation3DAnimationUsingKeyFrames.cs
- XmlSchemaAttribute.cs
- StrongTypingException.cs
- ArgumentException.cs
- HttpListenerPrefixCollection.cs
- RemoteDebugger.cs
- AutomationIdentifierGuids.cs
- AssemblyName.cs
- SqlAliasesReferenced.cs