Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Net / System / Net / Mail / DelegatedStream.cs / 1 / DelegatedStream.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net { using System; using System.Net.Sockets; using System.IO; internal class DelegatedStream : Stream { Stream stream; NetworkStream netStream; protected DelegatedStream() { } protected DelegatedStream(Stream stream) { if (stream == null) throw new ArgumentNullException("stream"); this.stream = stream; netStream = stream as NetworkStream; } protected Stream BaseStream { get { return this.stream; } } public override bool CanRead { get { return this.stream.CanRead; } } public override bool CanSeek { get { return this.stream.CanSeek; } } public override bool CanWrite { get { return this.stream.CanWrite; } } public override long Length { get { if (!CanSeek) throw new NotSupportedException(SR.GetString(SR.SeekNotSupported)); return this.stream.Length; } } public override long Position { get { if (!CanSeek) throw new NotSupportedException(SR.GetString(SR.SeekNotSupported)); return this.stream.Position; } set { if (!CanSeek) throw new NotSupportedException(SR.GetString(SR.SeekNotSupported)); this.stream.Position = value; } } public override IAsyncResult BeginRead(byte[] buffer, int offset, int count, AsyncCallback callback, object state) { if (!CanRead) throw new NotSupportedException(SR.GetString(SR.ReadNotSupported)); IAsyncResult result = null; if(netStream != null){ result = this.netStream.UnsafeBeginRead (buffer, offset, count, callback, state); } else{ result = this.stream.BeginRead (buffer, offset, count, callback, state); } return result; } public override IAsyncResult BeginWrite(byte[] buffer, int offset, int count, AsyncCallback callback, object state) { if (!CanWrite) throw new NotSupportedException(SR.GetString(SR.WriteNotSupported)); IAsyncResult result = null; if(netStream != null){ result = this.netStream.UnsafeBeginWrite(buffer, offset, count, callback, state); } else{ result = this.stream.BeginWrite (buffer, offset, count, callback, state); } return result; } //This calls close on the inner stream //however, the stream may not be actually closed, but simpy flushed public override void Close() { this.stream.Close(); } public override int EndRead(IAsyncResult asyncResult) { if (!CanRead) throw new NotSupportedException(SR.GetString(SR.ReadNotSupported)); int read = this.stream.EndRead (asyncResult); return read; } public override void EndWrite(IAsyncResult asyncResult) { if (!CanWrite) throw new NotSupportedException(SR.GetString(SR.WriteNotSupported)); this.stream.EndWrite (asyncResult); } public override void Flush() { this.stream.Flush(); } public override int Read(byte[] buffer, int offset, int count) { if (!CanRead) throw new NotSupportedException(SR.GetString(SR.ReadNotSupported)); int read = this.stream.Read(buffer, offset, count); return read; } public override long Seek(long offset, SeekOrigin origin) { if (!CanSeek) throw new NotSupportedException(SR.GetString(SR.SeekNotSupported)); long position = this.stream.Seek(offset, origin); return position; } public override void SetLength(long value) { if (!CanSeek) throw new NotSupportedException(SR.GetString(SR.SeekNotSupported)); this.stream.SetLength(value); } public override void Write(byte[] buffer, int offset, int count) { if (!CanWrite) throw new NotSupportedException(SR.GetString(SR.WriteNotSupported)); this.stream.Write(buffer, offset, count); } } }
Link Menu
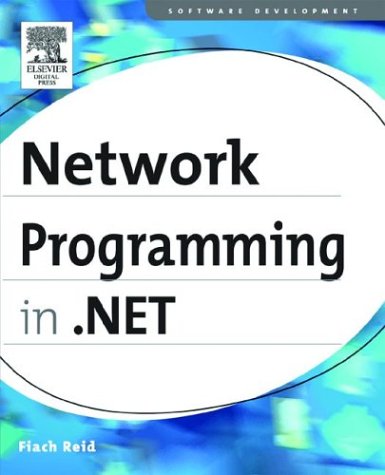
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AppDomainUnloadedException.cs
- Window.cs
- DesignerToolboxInfo.cs
- HtmlShimManager.cs
- Oid.cs
- PreviewPageInfo.cs
- ToolStripLocationCancelEventArgs.cs
- Missing.cs
- DesignerActionList.cs
- EditingMode.cs
- DataObject.cs
- UrlEncodedParameterWriter.cs
- ComIntegrationManifestGenerator.cs
- MonthCalendar.cs
- MapPathBasedVirtualPathProvider.cs
- DataRelationPropertyDescriptor.cs
- Annotation.cs
- HttpModuleCollection.cs
- XmlSchemaAll.cs
- ImpersonateTokenRef.cs
- ListViewUpdatedEventArgs.cs
- DataTableClearEvent.cs
- HttpStreamXmlDictionaryWriter.cs
- UnsafeNativeMethods.cs
- SystemIcons.cs
- DecoderFallback.cs
- XmlSchemaAny.cs
- BitmapCodecInfoInternal.cs
- DropShadowBitmapEffect.cs
- EmbeddedObject.cs
- Documentation.cs
- TemplatingOptionsDialog.cs
- SessionStateItemCollection.cs
- XmlLanguage.cs
- WhitespaceRule.cs
- MaterialCollection.cs
- PageFunction.cs
- OracleDateTime.cs
- RotateTransform.cs
- EditingScopeUndoUnit.cs
- XmlAttributeOverrides.cs
- SubtreeProcessor.cs
- CompilationSection.cs
- SendContent.cs
- FlowDocument.cs
- FormViewInsertEventArgs.cs
- _AcceptOverlappedAsyncResult.cs
- KnownAssemblyEntry.cs
- BrowserInteropHelper.cs
- DeclarativeExpressionConditionDeclaration.cs
- UserMapPath.cs
- PolicyValidationException.cs
- HttpModuleCollection.cs
- EntityContainerEntitySetDefiningQuery.cs
- RunClient.cs
- XmlDataSourceView.cs
- SystemGatewayIPAddressInformation.cs
- TextFindEngine.cs
- ProtocolElementCollection.cs
- XmlSchemaObjectTable.cs
- _SslSessionsCache.cs
- _HelperAsyncResults.cs
- RijndaelManaged.cs
- ResourceDictionary.cs
- ReflectionTypeLoadException.cs
- ApplicationServicesHostFactory.cs
- UIElement3DAutomationPeer.cs
- MenuItemAutomationPeer.cs
- DataListItemCollection.cs
- DataSourceBooleanViewSchemaConverter.cs
- ServiceReference.cs
- HttpResponseHeader.cs
- SqlProviderManifest.cs
- Hex.cs
- OracleColumn.cs
- DynamicRendererThreadManager.cs
- PhysicalAddress.cs
- ControlBuilder.cs
- PrivilegeNotHeldException.cs
- ThousandthOfEmRealPoints.cs
- DataRow.cs
- ResourcePermissionBase.cs
- TimerElapsedEvenArgs.cs
- TextTreeNode.cs
- CodeAttributeArgument.cs
- EdgeProfileValidation.cs
- PngBitmapEncoder.cs
- Win32SafeHandles.cs
- MarkupProperty.cs
- NameTable.cs
- ListViewItem.cs
- KerberosReceiverSecurityToken.cs
- ResourceReferenceKeyNotFoundException.cs
- EmptyStringExpandableObjectConverter.cs
- QilPatternFactory.cs
- ListViewGroup.cs
- ConfigurationStrings.cs
- XsltArgumentList.cs
- CurrentChangingEventManager.cs
- TrackingProfile.cs