Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / untmp / whidbey / QFE / ndp / fx / src / xsp / System / Web / HttpCacheVary.cs / 1 / HttpCacheVary.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Cache Vary class. Wraps Vary header * * Copyright (c) 1998 Microsoft Corporation */ namespace System.Web { using System.Text; using System.Runtime.InteropServices; using System.Web.Util; using System.Security.Permissions; ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class HttpCacheVaryByHeaders { bool _isModified; bool _varyStar; HttpDictionary _headers; internal HttpCacheVaryByHeaders() { Reset(); } internal void Reset() { _isModified = false; _varyStar = false; _headers = null; } /* * Reset based on the cached vary headers. */ internal void ResetFromHeaders(String[] headers) { int i, n; if (headers == null) { _isModified = false; _varyStar = false; _headers = null; } else { _isModified = true; if (headers[0].Equals("*")) { Debug.Assert(headers.Length == 1, "headers.Length == 1"); _varyStar = true; _headers = null; } else { _varyStar = false; _headers = new HttpDictionary(); for (i = 0, n = headers.Length; i < n; i++) { _headers.SetValue(headers[i], headers[i]); } } } } internal bool IsModified() { return _isModified; } /* * Construct header value string */ internal String ToHeaderString() { StringBuilder s; Object item; int i, n; if (_varyStar) { return "*"; } else if (_headers != null) { s = new StringBuilder(); for (i = 0, n = _headers.Size; i < n; i++) { item = _headers.GetValue(i); if (item != null) { HttpCachePolicy.AppendValueToHeader(s, (String)item); } } if (s.Length > 0) return s.ToString(); } return null; } /* * Returns the headers, for package access only. * * @return the headers. */ internal String[] GetHeaders() { String[] s = null; Object item; int i, j, c, n; if (_varyStar) { return new String[1] {"*"}; } else if (_headers != null) { n = _headers.Size; c = 0; for (i = 0; i < n; i++) { item = _headers.GetValue(i); if (item != null) { c++; } } if (c > 0) { s = new string[c]; j = 0; for (i = 0; i < n; i++) { item = _headers.GetValue(i); if (item != null) { s[j] = (String) item; j++; } } Debug.Assert(j == c, "j == c"); } } return s; } // // Public methods and properties // ///Indicates that a cache should contain multiple /// representations for a particular Uri. This class is an encapsulation that /// provides a rich, type-safe way to set the Vary header. ////// public void VaryByUnspecifiedParameters() { _isModified = true; _varyStar = true; _headers = null; } internal bool GetVaryByUnspecifiedParameters() { return _varyStar; } /* * Vary by accept types */ ///Sets the "Vary: *" header and causes all other Vary: /// header information to be dropped. ////// public bool AcceptTypes { get { return this["Accept"]; } set { _isModified = true; this["Accept"] = value; } } /* * Vary by accept language */ ///Retrieves or assigns a value indicating whether the cache should vary by Accept types. This causes the /// Vary: header to include an Accept field. ////// public bool UserLanguage { get { return this["Accept-Language"]; } set { _isModified = true; this["Accept-Language"] = value; } } /* * Vary by user agent */ ///Retrieves or assigns a Boolean value indicating whether /// the cache should vary by user language. ////// public bool UserAgent { get { return this["User-Agent"]; } set { _isModified = true; this["User-Agent"] = value; } } /* * Vary by charset */ ///Retrieves or assigns a Boolean value indicating whether /// the cache should vary by user agent. ////// public bool UserCharSet { get { return this["Accept-Charset"]; } set { _isModified = true; this["Accept-Charset"] = value; } } /* * Vary by a given header */ ///Retrieves or assigns a value indicating whether the /// cache should vary by browser character set. ////// public bool this[String header] { get { if (header == null) { throw new ArgumentNullException("header"); } if (header.Equals("*")) { return _varyStar; } else { return (_headers != null && _headers.GetValue(header) != null); } } set { if (header == null) { throw new ArgumentNullException("header"); } /* * Since adding a Vary header is more restrictive, we don't * want components to be able to set a Vary header to false * if another component has set it to true. */ if (value == false) { return; } _isModified = true; if (header.Equals("*")) { VaryByUnspecifiedParameters(); } else { // set value to header if true or null if false if (!_varyStar) { if (_headers == null) { _headers = new HttpDictionary(); } _headers.SetValue(header, header); } } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.Default property. /// Indexed property indicating that a cache should (or should not) vary according /// to a custom header. ///
Link Menu
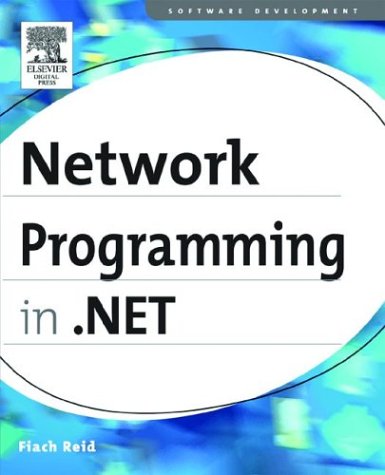
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ThicknessAnimationBase.cs
- TraceSection.cs
- InteropAutomationProvider.cs
- AssemblyName.cs
- SqlCaseSimplifier.cs
- PackWebResponse.cs
- ApplicationProxyInternal.cs
- WebControlToolBoxItem.cs
- Evidence.cs
- DataExpression.cs
- Button.cs
- DecimalKeyFrameCollection.cs
- SequenceNumber.cs
- ReferentialConstraint.cs
- TreeNode.cs
- KerberosReceiverSecurityToken.cs
- TransportContext.cs
- WindowsPen.cs
- NumberEdit.cs
- WebServiceMethodData.cs
- Metafile.cs
- InfiniteTimeSpanConverter.cs
- ReadonlyMessageFilter.cs
- DbConnectionFactory.cs
- XsdBuildProvider.cs
- XmlSchemaSimpleTypeUnion.cs
- DtrList.cs
- SettingsPropertyNotFoundException.cs
- CommonDialog.cs
- DoubleAnimation.cs
- ScriptRef.cs
- LocalizeDesigner.cs
- WindowsGraphics.cs
- CookieProtection.cs
- shaperfactoryquerycacheentry.cs
- DebugHandleTracker.cs
- XmlMapping.cs
- TranslateTransform3D.cs
- SolidColorBrush.cs
- BackgroundFormatInfo.cs
- DependencyObjectPropertyDescriptor.cs
- ControlCollection.cs
- SecurityHeaderLayout.cs
- ReachSerializationCacheItems.cs
- WindowsSolidBrush.cs
- DbBuffer.cs
- FileUtil.cs
- DiscriminatorMap.cs
- ToolBarButtonClickEvent.cs
- ToolStripComboBox.cs
- MD5.cs
- XmlCodeExporter.cs
- SqlServer2KCompatibilityCheck.cs
- MetadataPropertyCollection.cs
- AtomServiceDocumentSerializer.cs
- DesignerActionGlyph.cs
- MessageHeaderAttribute.cs
- XmlElement.cs
- NotifyCollectionChangedEventArgs.cs
- Rect3D.cs
- Point.cs
- Transform3D.cs
- WebPartEditorApplyVerb.cs
- MachineKeyConverter.cs
- VirtualDirectoryMappingCollection.cs
- ContextBase.cs
- DataBindingCollection.cs
- ButtonPopupAdapter.cs
- WebFaultClientMessageInspector.cs
- UnsafeNativeMethods.cs
- PropertyGroupDescription.cs
- SafeFileHandle.cs
- DateTime.cs
- ConnectionInterfaceCollection.cs
- TreeChangeInfo.cs
- BaseParaClient.cs
- Win32.cs
- XPathNavigator.cs
- XPathDescendantIterator.cs
- ExtendedPropertyDescriptor.cs
- SqlSelectClauseBuilder.cs
- ExtensionSimplifierMarkupObject.cs
- codemethodreferenceexpression.cs
- MobileListItem.cs
- SystemMulticastIPAddressInformation.cs
- XmlUnspecifiedAttribute.cs
- IncrementalReadDecoders.cs
- EventMetadata.cs
- ContextStaticAttribute.cs
- SafeBitVector32.cs
- UnmanagedMemoryStreamWrapper.cs
- NetworkStream.cs
- DeviceOverridableAttribute.cs
- HostingPreferredMapPath.cs
- TemplateBindingExpressionConverter.cs
- SiteOfOriginPart.cs
- TransformPatternIdentifiers.cs
- EntitySetBase.cs
- BamlTreeNode.cs
- OdbcParameter.cs