Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / System / Windows / PropertyPathConverter.cs / 1 / PropertyPathConverter.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: PropertyPathConverter.cs // // Description: Contains converter for creating PropertyPath from string // and saving PropertyPath to string // // History: // 05/15/2004 - peterost - Initial implementation // //--------------------------------------------------------------------------- using System; using System.ComponentModel; using System.Collections.ObjectModel; using System.Text; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Windows; using System.Windows.Markup; using MS.Internal; using MS.Utility; #pragma warning disable 1634, 1691 // suppressing PreSharp warnings namespace System.Windows { ////// PropertyPathConverter - Converter class for converting instances of other types /// to and from PropertyPath instances. /// public sealed class PropertyPathConverter: TypeConverter { //------------------------------------------------------------------- // // Public Methods // //------------------------------------------------------------------- #region Public Methods ////// CanConvertFrom - Returns whether or not this class can convert from a given type. /// ////// bool - True if this converter can convert from the provided type, false if not. /// /// The ITypeDescriptorContext for this call. /// The Type being queried for support. public override bool CanConvertFrom(ITypeDescriptorContext typeDescriptorContext, Type sourceType) { // We can only handle strings if (sourceType == typeof(string)) { return true; } else { return false; } } ////// CanConvertTo - Returns whether or not this class can convert to a given type. /// ////// bool - True if this converter can convert to the provided type, false if not. /// /// The ITypeDescriptorContext for this call. /// The Type being queried for support. public override bool CanConvertTo(ITypeDescriptorContext typeDescriptorContext, Type destinationType) { // We can convert to a string. if (destinationType == typeof(string)) { return true; } else { return false; } } ////// ConvertFrom - Attempt to convert to a PropertyPath from the given object /// ////// The PropertyPath which was constructed. /// ////// An ArgumentNullException is thrown if the example object is null. /// ////// An ArgumentException is thrown if the example object is not null and is not a valid type /// which can be converted to a PropertyPath. /// /// The ITypeDescriptorContext for this call. /// The CultureInfo which is respected when converting. /// The object to convert to a PropertyPath. public override object ConvertFrom(ITypeDescriptorContext typeDescriptorContext, CultureInfo cultureInfo, object source) { if (source == null) { throw new ArgumentNullException("source"); } if (source is string) { return new PropertyPath((string)source, typeDescriptorContext); } #pragma warning suppress 6506 // source is obviously not null throw new ArgumentException(SR.Get(SRID.CannotConvertType, source.GetType().FullName, typeof(PropertyPath))); } ////// ConvertTo - Attempt to convert a PropertyPath to the given type /// ////// The object which was constructed. /// ////// An ArgumentNullException is thrown if the example object is null. /// ////// An ArgumentException is thrown if the example object is not null and is not a Brush, /// or if the destinationType isn't one of the valid destination types. /// /// The ITypeDescriptorContext for this call. /// If this is null, then no namespace prefixes will be included. /// The CultureInfo which is respected when converting. /// The PropertyPath to convert. /// The type to which to convert the PropertyPath instance. public override object ConvertTo(ITypeDescriptorContext typeDescriptorContext, CultureInfo cultureInfo, object value, Type destinationType) { if (null == value) { throw new ArgumentNullException("value"); } if (null == destinationType) { throw new ArgumentNullException("destinationType"); } if (destinationType != typeof(String)) { throw new ArgumentException(SR.Get(SRID.CannotConvertType, typeof(PropertyPath), destinationType.FullName)); } PropertyPath path = value as PropertyPath; if (path == null) { throw new ArgumentException(SR.Get(SRID.UnexpectedParameterType, value.GetType(), typeof(PropertyPath)), "value"); } if (path.PathParameters.Count == 0) { // if the path didn't use paramaters, just write it out as it is return path.Path; } else { // if the path used parameters, convert them to (NamespacePrefix:OwnerType.DependencyPropertyName) syntax string originalPath = path.Path; Collection
Link Menu
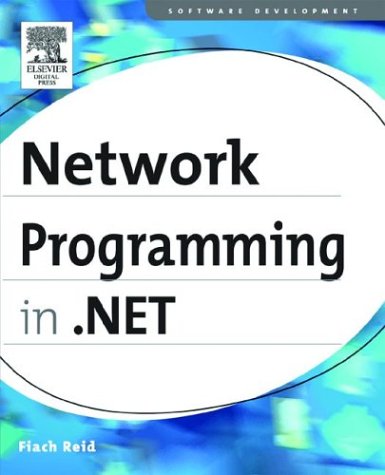
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- IPAddress.cs
- CodeTypeMember.cs
- Rect.cs
- DBProviderConfigurationHandler.cs
- EventHandlersStore.cs
- WindowsProgressbar.cs
- ViewGenResults.cs
- SkewTransform.cs
- CalendarDayButton.cs
- TextSelectionHelper.cs
- VisualTreeUtils.cs
- DependencyPropertyAttribute.cs
- DataControlButton.cs
- SoapAttributeAttribute.cs
- CodeBlockBuilder.cs
- DataPointer.cs
- SecurityUniqueId.cs
- FeatureAttribute.cs
- ExpressionServices.cs
- DropSource.cs
- CheckableControlBaseAdapter.cs
- List.cs
- ScriptingJsonSerializationSection.cs
- CommandManager.cs
- ToolStripPanelSelectionGlyph.cs
- counter.cs
- BitmapScalingModeValidation.cs
- DesignRelation.cs
- XmlException.cs
- RegexCompiler.cs
- LineGeometry.cs
- OdbcReferenceCollection.cs
- QueryConverter.cs
- MessageSmuggler.cs
- FontResourceCache.cs
- HttpListenerPrefixCollection.cs
- DictionaryContent.cs
- Aggregates.cs
- ZoneMembershipCondition.cs
- SemanticResolver.cs
- VisualTreeUtils.cs
- Oid.cs
- SynchronousSendBindingElement.cs
- ListControl.cs
- PropertyGeneratedEventArgs.cs
- ProfileGroupSettings.cs
- RowToParametersTransformer.cs
- X509Chain.cs
- Privilege.cs
- InvokeHandlers.cs
- XpsSerializationManager.cs
- newitemfactory.cs
- ExtenderProvidedPropertyAttribute.cs
- PropertyIDSet.cs
- TextPattern.cs
- DataGridColumnFloatingHeader.cs
- CodeValidator.cs
- AppDomainProtocolHandler.cs
- UnsafeNativeMethods.cs
- MulticastOption.cs
- QuaternionAnimation.cs
- MultiByteCodec.cs
- TextTreePropertyUndoUnit.cs
- TraceInternal.cs
- XmlElementCollection.cs
- ColorBlend.cs
- ListViewUpdatedEventArgs.cs
- DataMisalignedException.cs
- CatalogZoneBase.cs
- Cursors.cs
- CommandLibraryHelper.cs
- SapiRecognizer.cs
- GridView.cs
- InstanceCreationEditor.cs
- BrushValueSerializer.cs
- StackSpiller.cs
- DataError.cs
- WindowsGraphicsWrapper.cs
- AlgoModule.cs
- DrawingGroupDrawingContext.cs
- Geometry.cs
- QueryRewriter.cs
- FixedPage.cs
- PreviewPrintController.cs
- COM2PropertyDescriptor.cs
- LinqDataSourceDisposeEventArgs.cs
- TokenizerHelper.cs
- ElementNotAvailableException.cs
- WindowsFormsSectionHandler.cs
- WeakEventTable.cs
- Operator.cs
- CodeAttachEventStatement.cs
- ThreadWorkerController.cs
- PenContext.cs
- ItemCheckEvent.cs
- XmlObjectSerializerReadContextComplexJson.cs
- InfoCardBaseException.cs
- SchemaCreator.cs
- EqualityComparer.cs
- XmlTextEncoder.cs