Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Controls / Primitives / LayoutInformation.cs / 1305600 / LayoutInformation.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2005 // // File: LayoutInformation // Spec: http://team/sites/Avalon/Specs/LayoutInformation%20class.doc //----------------------------------------------------------------------------- using System; using System.Windows.Media; using System.Windows.Threading; namespace System.Windows.Controls.Primitives { ////// This interface exposes additional layout information not exposed otherwise on FrameworkElement. /// This information is mostly used by the designer programs to produce additional visual clues for the user /// during interactive editing of the elements and layout properties. /// public static class LayoutInformation { private static void CheckArgument(FrameworkElement element) { if (element == null) { throw new ArgumentNullException("element"); } } ////// Returns the rectangle that represents Layout Slot - the layout partition reserved for the /// child by the layout parent. This info is in the coordinte system of the layout parent. /// public static Rect GetLayoutSlot(FrameworkElement element) { CheckArgument(element); return element.PreviousArrangeRect; } ////// Returns a geometry which was computed by layout for the child. This is generally a visible region of the child. /// Layout can compute automatic clip region when the child is larger then layout constraints or has ClipToBounds /// property set. Note that because of LayoutTransform, this could be a non-rectangular geometry. While general geometry is somewhat /// complex to operate with, it is possible to check if the Geometry returned is RectangularGeometry or, if not - use Geometry.Bounds /// property to get bounding box of the visible portion of the element. /// public static Geometry GetLayoutClip(FrameworkElement element) { CheckArgument(element); return element.GetLayoutClipInternal(); } ////// Returns a UIElement which was being processed by Layout Engine at the moment /// an unhandled exception casued Layout Engine to abandon the operation and unwind. /// Returns non-null result only for a period of time before next layout update is /// initiated. Can be examined from the application exception handler. /// /// The Dispatcher object that specifies the scope of operation. There is one Layout Engine per Dispatcher. public static UIElement GetLayoutExceptionElement(Dispatcher dispatcher) { if(dispatcher == null) throw new ArgumentNullException("dispatcher"); UIElement e = null; ContextLayoutManager lm = ContextLayoutManager.From(dispatcher); if(lm != null) e = lm.GetLastExceptionElement(); return e; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
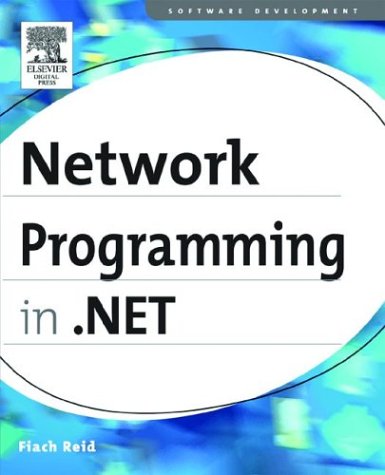
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataConnectionHelper.cs
- DataException.cs
- PtsHost.cs
- WindowPattern.cs
- ProtocolsConfigurationHandler.cs
- DesignTimeTemplateParser.cs
- SuppressIldasmAttribute.cs
- OracleConnectionString.cs
- WebControlsSection.cs
- ClientRuntimeConfig.cs
- DataGridViewRowHeightInfoNeededEventArgs.cs
- NamedPermissionSet.cs
- ArgumentsParser.cs
- ResourceSetExpression.cs
- TraceProvider.cs
- SettingsPropertyCollection.cs
- MetadataItemEmitter.cs
- QilStrConcat.cs
- SerializationInfoEnumerator.cs
- mediaclock.cs
- IsolationInterop.cs
- StructuralType.cs
- ExpandableObjectConverter.cs
- SimplePropertyEntry.cs
- ComplusEndpointConfigContainer.cs
- SafeWaitHandle.cs
- LocationReferenceEnvironment.cs
- NonParentingControl.cs
- UnmanagedBitmapWrapper.cs
- SoapReflectionImporter.cs
- FormViewUpdateEventArgs.cs
- errorpatternmatcher.cs
- LoadedEvent.cs
- XmlBinaryReader.cs
- OleCmdHelper.cs
- oledbmetadatacollectionnames.cs
- SapiAttributeParser.cs
- FormatConvertedBitmap.cs
- PersistenceTypeAttribute.cs
- PerformanceCounterLib.cs
- DocumentSequenceHighlightLayer.cs
- StaticContext.cs
- SettingsPropertyCollection.cs
- SecurityTokenRequirement.cs
- odbcmetadatacollectionnames.cs
- AccessDataSourceWizardForm.cs
- ToolStripRenderer.cs
- OleDbError.cs
- UIElement.cs
- StackOverflowException.cs
- cryptoapiTransform.cs
- LabelLiteral.cs
- infer.cs
- ObjectListSelectEventArgs.cs
- IntSumAggregationOperator.cs
- XPathSelectionIterator.cs
- WebPartUserCapability.cs
- StringConcat.cs
- LinkDescriptor.cs
- BadImageFormatException.cs
- TiffBitmapEncoder.cs
- RegistrySecurity.cs
- templategroup.cs
- PrincipalPermissionMode.cs
- ValueConversionAttribute.cs
- ProfileParameter.cs
- Util.cs
- XPathNodeIterator.cs
- RelationshipEntry.cs
- ErrorFormatter.cs
- ErrorEventArgs.cs
- XsdDuration.cs
- OutKeywords.cs
- HtmlElementCollection.cs
- VoiceChangeEventArgs.cs
- PolyBezierSegment.cs
- XappLauncher.cs
- XmlReaderSettings.cs
- ProvideValueServiceProvider.cs
- ModifiableIteratorCollection.cs
- ExpressionBuilder.cs
- ArrangedElement.cs
- DynamicActivityProperty.cs
- BaseTemplateBuildProvider.cs
- Columns.cs
- DataGridViewRowCancelEventArgs.cs
- Random.cs
- ListBoxItemAutomationPeer.cs
- StrokeSerializer.cs
- XmlSchemaChoice.cs
- TraceSection.cs
- StringBuilder.cs
- AbstractExpressions.cs
- DiagnosticsConfigurationHandler.cs
- MulticastDelegate.cs
- SoapAttributeOverrides.cs
- LayoutEngine.cs
- ColorConverter.cs
- SynchronizedCollection.cs
- BaseDataList.cs