Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / XmlUtils / System / Xml / Xsl / Runtime / StringConcat.cs / 1 / StringConcat.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; using System.ComponentModel; namespace System.Xml.Xsl.Runtime { ////// Efficiently concatenates strings when the number of string is not known beforehand, and /// yet it is common for only one string to be concatenated. StringBuilder is not good for /// this purpose, since it *always* allocates objects, even if only one string is appended. /// [EditorBrowsable(EditorBrowsableState.Never)] public struct StringConcat { private string s1, s2, s3, s4; private string delimiter; private ListstrList; int idxStr; /// /// Clear the result string. /// public void Clear() { this.idxStr = 0; this.delimiter = null; } ////// Gets or sets the string that delimits concatenated strings. /// public string Delimiter { get { return this.delimiter; } set { this.delimiter = value; } } ////// Return the number of concatenated strings, including delimiters. /// internal int Count { get { return this.idxStr; } } ////// Concatenate a new string to the result. /// public void Concat(string value) { Debug.Assert(value != null); if (this.delimiter != null && this.idxStr != 0) { // Add delimiter ConcatNoDelimiter(this.delimiter); } ConcatNoDelimiter(value); } ////// Get the result string. /// public string GetResult() { switch (this.idxStr) { case 0: return string.Empty; case 1: return this.s1; case 2: return string.Concat(this.s1, this.s2); case 3: return string.Concat(this.s1, this.s2, this.s3); case 4: return string.Concat(this.s1, this.s2, this.s3, this.s4); } return string.Concat(this.strList.ToArray()); } ////// Concatenate a new string to the result without adding a delimiter. /// internal void ConcatNoDelimiter(string s) { switch (this.idxStr) { case 0: this.s1 = s; break; case 1: this.s2 = s; break; case 2: this.s3 = s; break; case 3: this.s4 = s; break; case 4: // Calling Clear() is expensive, allocate a new List instead int capacity = (this.strList == null) ? 8 : this.strList.Count; ListstrList = this.strList = new List (capacity); strList.Add(this.s1); strList.Add(this.s2); strList.Add(this.s3); strList.Add(this.s4); goto default; default: this.strList.Add(s); break; } this.idxStr++; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; using System.ComponentModel; namespace System.Xml.Xsl.Runtime { ////// Efficiently concatenates strings when the number of string is not known beforehand, and /// yet it is common for only one string to be concatenated. StringBuilder is not good for /// this purpose, since it *always* allocates objects, even if only one string is appended. /// [EditorBrowsable(EditorBrowsableState.Never)] public struct StringConcat { private string s1, s2, s3, s4; private string delimiter; private ListstrList; int idxStr; /// /// Clear the result string. /// public void Clear() { this.idxStr = 0; this.delimiter = null; } ////// Gets or sets the string that delimits concatenated strings. /// public string Delimiter { get { return this.delimiter; } set { this.delimiter = value; } } ////// Return the number of concatenated strings, including delimiters. /// internal int Count { get { return this.idxStr; } } ////// Concatenate a new string to the result. /// public void Concat(string value) { Debug.Assert(value != null); if (this.delimiter != null && this.idxStr != 0) { // Add delimiter ConcatNoDelimiter(this.delimiter); } ConcatNoDelimiter(value); } ////// Get the result string. /// public string GetResult() { switch (this.idxStr) { case 0: return string.Empty; case 1: return this.s1; case 2: return string.Concat(this.s1, this.s2); case 3: return string.Concat(this.s1, this.s2, this.s3); case 4: return string.Concat(this.s1, this.s2, this.s3, this.s4); } return string.Concat(this.strList.ToArray()); } ////// Concatenate a new string to the result without adding a delimiter. /// internal void ConcatNoDelimiter(string s) { switch (this.idxStr) { case 0: this.s1 = s; break; case 1: this.s2 = s; break; case 2: this.s3 = s; break; case 3: this.s4 = s; break; case 4: // Calling Clear() is expensive, allocate a new List instead int capacity = (this.strList == null) ? 8 : this.strList.Count; ListstrList = this.strList = new List (capacity); strList.Add(this.s1); strList.Add(this.s2); strList.Add(this.s3); strList.Add(this.s4); goto default; default: this.strList.Add(s); break; } this.idxStr++; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
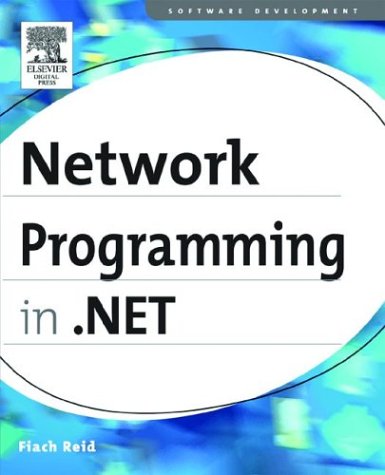
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DecoderFallbackWithFailureFlag.cs
- InitializingNewItemEventArgs.cs
- ComplexBindingPropertiesAttribute.cs
- CookieProtection.cs
- TcpChannelHelper.cs
- ColumnHeader.cs
- ExpandCollapsePattern.cs
- GroupByQueryOperator.cs
- SafeBitVector32.cs
- SiteMap.cs
- Events.cs
- CodeStatement.cs
- Memoizer.cs
- CodeIterationStatement.cs
- XmlSerializerNamespaces.cs
- ListBoxItemWrapperAutomationPeer.cs
- WebBrowser.cs
- Journaling.cs
- Interop.cs
- CacheOutputQuery.cs
- XamlWriter.cs
- XNodeValidator.cs
- ExpressionPrinter.cs
- CodeGenerationManager.cs
- X509Utils.cs
- HttpHeaderCollection.cs
- DataGridTextBoxColumn.cs
- XmlSerializationReader.cs
- BitmapInitialize.cs
- RegexCompiler.cs
- KnownTypes.cs
- UndoManager.cs
- PipelineModuleStepContainer.cs
- RequestCachingSection.cs
- CharKeyFrameCollection.cs
- HtmlInputFile.cs
- FileDetails.cs
- DataGridViewColumnCollectionEditor.cs
- TitleStyle.cs
- CompletedAsyncResult.cs
- ParameterToken.cs
- HighlightVisual.cs
- precedingsibling.cs
- HashUtility.cs
- XhtmlTextWriter.cs
- XmlEntityReference.cs
- FunctionImportElement.cs
- RegexRunner.cs
- GridViewUpdatedEventArgs.cs
- Send.cs
- ProjectionPath.cs
- OdbcError.cs
- HttpServerUtilityWrapper.cs
- Timer.cs
- NavigationProperty.cs
- glyphs.cs
- KeyNotFoundException.cs
- Point3DAnimationBase.cs
- TypeToken.cs
- ConsoleKeyInfo.cs
- Camera.cs
- WebSysDescriptionAttribute.cs
- ColorContext.cs
- iisPickupDirectory.cs
- CodeStatementCollection.cs
- DictionaryMarkupSerializer.cs
- DataBoundLiteralControl.cs
- HexParser.cs
- DataAccessor.cs
- ValueQuery.cs
- ConsoleCancelEventArgs.cs
- CompositeCollectionView.cs
- TableLayoutStyleCollection.cs
- ErrorFormatterPage.cs
- DependencyPropertyKind.cs
- UIHelper.cs
- TextWriterEngine.cs
- TreeNodeEventArgs.cs
- CodeDirectiveCollection.cs
- Sql8ExpressionRewriter.cs
- AutomationPatternInfo.cs
- RegularExpressionValidator.cs
- TrustLevel.cs
- _IPv6Address.cs
- NamedPipeConnectionPoolSettings.cs
- ConfigurationStrings.cs
- GridProviderWrapper.cs
- PixelShader.cs
- XmlBinaryReader.cs
- Group.cs
- DeclarationUpdate.cs
- Types.cs
- Size3D.cs
- SqlWriter.cs
- EdgeProfileValidation.cs
- RuntimeCompatibilityAttribute.cs
- TdsParserStateObject.cs
- IndependentlyAnimatedPropertyMetadata.cs
- IdnMapping.cs
- path.cs