Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Shared / MS / Internal / DecoderFallbackWithFailureFlag.cs / 1305600 / DecoderFallbackWithFailureFlag.cs
//---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // Description: DecoderFallbackWithFailureFlag is used when the developer wants Encoding.GetChars() method to fail // without throwing an exception when decoding cannot be performed. // Usage pattern is: // DecoderFallbackWithFailureFlag fallback = new DecoderFallbackWithFailureFlag(); // Encoding e = Encoding.GetEncoding(codePage, EncoderFallback.ExceptionFallback, fallback); // e.GetChars(bytesToDecode); // if (fallback.HasFailed) // { // // Perform fallback and reset the failure flag. // fallback.HasFailed = false; // } // // History: // 05/03/2005 : mleonov - Created // //--------------------------------------------------------------------------- using System.Text; namespace MS.Internal { ////// This class is similar to the standard DecoderExceptionFallback class, /// except that for performance reasons it sets a Boolean failure flag /// instead of throwing exception. /// internal class DecoderFallbackWithFailureFlag : DecoderFallback { public DecoderFallbackWithFailureFlag() { } public override DecoderFallbackBuffer CreateFallbackBuffer() { return new FallbackBuffer(this); } ////// The maximum number of characters this instance can return. /// public override int MaxCharCount { get { return 0; } } ////// Returns whether decoding failed. /// public bool HasFailed { get { return _hasFailed; } set { _hasFailed = value; } } private bool _hasFailed; // false by default ////// A special implementation of DecoderFallbackBuffer that sets the failure flag /// in the parent DecoderFallbackWithFailureFlag class. /// private class FallbackBuffer : DecoderFallbackBuffer { public FallbackBuffer(DecoderFallbackWithFailureFlag parent) { _parent = parent; } ////// Indicates whether a substitute string can be emitted if an input byte sequence cannot be decoded. /// Parameters specify an input byte sequence, and the index position of a byte in the input. /// /// An input array of bytes. /// The index position of a byte in bytesUnknown. ///true if a string exists that can be inserted in the output /// instead of decoding the byte specified in bytesUnknown; /// false if the input byte should be ignored. public override bool Fallback(byte[] bytesUnknown, int index) { _parent.HasFailed = true; return false; } ////// Retrieves the next character in the fallback buffer. /// ///The next Unicode character in the fallback buffer. public override char GetNextChar() { return (char)0; } ////// Prepares the GetNextChar method to retrieve the preceding character in the fallback buffer. /// ///true if the MovePrevious operation was successful; otherwise, false. public override bool MovePrevious() { return false; } ////// Gets the number of characters in this instance of DecoderFallbackBuffer that remain to be processed. /// public override int Remaining { get { return 0; } } private DecoderFallbackWithFailureFlag _parent; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
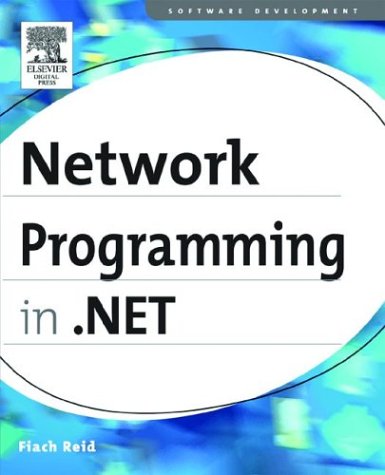
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HandleCollector.cs
- TextMessageEncoder.cs
- DataBinder.cs
- securitymgrsite.cs
- WebPartConnectionsCloseVerb.cs
- TdsParserSessionPool.cs
- MsmqIntegrationValidationBehavior.cs
- NumericUpDownAccelerationCollection.cs
- filewebresponse.cs
- _DomainName.cs
- DocumentPageView.cs
- FontDriver.cs
- IDataContractSurrogate.cs
- UIElement3D.cs
- ResourcePool.cs
- ComplexTypeEmitter.cs
- SqlBuffer.cs
- DataGridItemCollection.cs
- ArrayMergeHelper.cs
- URLMembershipCondition.cs
- KeyedHashAlgorithm.cs
- ColumnHeaderConverter.cs
- LinkUtilities.cs
- OwnerDrawPropertyBag.cs
- AspNetSynchronizationContext.cs
- ObjectListDesigner.cs
- SmiSettersStream.cs
- DiffuseMaterial.cs
- DynamicUpdateCommand.cs
- TargetException.cs
- IisTraceWebEventProvider.cs
- ConvertersCollection.cs
- XmlAnyElementAttribute.cs
- String.cs
- BooleanExpr.cs
- XmlNodeWriter.cs
- DetailsViewPageEventArgs.cs
- NavigationEventArgs.cs
- SizeConverter.cs
- ZoneLinkButton.cs
- PersistenceTypeAttribute.cs
- ShadowGlyph.cs
- GeometryModel3D.cs
- WhiteSpaceTrimStringConverter.cs
- NameValueConfigurationCollection.cs
- SchemaManager.cs
- InstallerTypeAttribute.cs
- WsatServiceCertificate.cs
- TextRenderer.cs
- StringConcat.cs
- path.cs
- MediaPlayer.cs
- Error.cs
- DataGridViewCellValueEventArgs.cs
- RegexGroup.cs
- ToolboxItem.cs
- XmlDataSourceDesigner.cs
- ScriptResourceHandler.cs
- SqlClientWrapperSmiStream.cs
- Helpers.cs
- ClientUIRequest.cs
- Frame.cs
- SafeEventHandle.cs
- WebHttpElement.cs
- SafeArchiveContext.cs
- HyperLinkStyle.cs
- Model3DCollection.cs
- MSAAEventDispatcher.cs
- SoapAttributes.cs
- HandlerBase.cs
- MILUtilities.cs
- WebServiceEnumData.cs
- MetadataUtilsSmi.cs
- UpDownBase.cs
- Point3D.cs
- Span.cs
- XPathMultyIterator.cs
- ZipIOExtraFieldZip64Element.cs
- DetailsViewUpdatedEventArgs.cs
- DefaultPropertyAttribute.cs
- PersonalizationDictionary.cs
- GeometryModel3D.cs
- ClrPerspective.cs
- RequestCachePolicy.cs
- PerfService.cs
- ReflectionTypeLoadException.cs
- GlyphRun.cs
- OleDbInfoMessageEvent.cs
- TextProviderWrapper.cs
- MonthCalendarDesigner.cs
- SemanticTag.cs
- XmlFormatExtensionAttribute.cs
- TextView.cs
- StructuredCompositeActivityDesigner.cs
- DataKeyArray.cs
- DataGridViewComponentPropertyGridSite.cs
- Manipulation.cs
- Constraint.cs
- XmlTextReader.cs
- ResourceType.cs