Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / Media3D / Generated / DiffuseMaterial.cs / 2 / DiffuseMaterial.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see [....]/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.Collections; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.Text; using System.Windows.Markup; using System.Windows.Media.Media3D.Converters; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using System.Windows.Media.Imaging; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows.Media.Media3D { sealed partial class DiffuseMaterial : Material { #region Constructors //----------------------------------------------------- // // Constructors // //----------------------------------------------------- static DiffuseMaterial() { // We check our static default fields which are of type Freezable // to make sure that they are not mutable, otherwise we will throw // if these get touched by more than one thread in the lifetime // of your app. (Windows OS Bug #947272) // Debug.Assert(s_Brush == null || s_Brush.IsFrozen, "Detected context bound default value DiffuseMaterial.s_Brush (See OS Bug #947272)."); } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Shadows inherited Clone() with a strongly typed /// version for convenience. /// public new DiffuseMaterial Clone() { return (DiffuseMaterial)base.Clone(); } ////// Shadows inherited CloneCurrentValue() with a strongly typed /// version for convenience. /// public new DiffuseMaterial CloneCurrentValue() { return (DiffuseMaterial)base.CloneCurrentValue(); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //------------------------------------------------------ private static void ColorPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { DiffuseMaterial target = ((DiffuseMaterial) d); target.PropertyChanged(ColorProperty); } private static void AmbientColorPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { DiffuseMaterial target = ((DiffuseMaterial) d); target.PropertyChanged(AmbientColorProperty); } private static void BrushPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { if (e.IsASubPropertyChange) { return; } DiffuseMaterial target = ((DiffuseMaterial) d); Brush oldV = (Brush) e.OldValue; Brush newV = (Brush) e.NewValue; System.Windows.Threading.Dispatcher dispatcher = target.Dispatcher; if (dispatcher != null) { DUCE.IResource targetResource = (DUCE.IResource)target; using (CompositionEngineLock.Acquire()) { int channelCount = targetResource.GetChannelCount(); for (int channelIndex = 0; channelIndex < channelCount; channelIndex++) { DUCE.Channel channel = targetResource.GetChannel(channelIndex); Debug.Assert(!channel.IsOutOfBandChannel); Debug.Assert(!targetResource.GetHandle(channel).IsNull); target.ReleaseResource(oldV,channel); target.AddRefResource(newV,channel); } } } target.PropertyChanged(BrushProperty); } #region Public Properties ////// Color - Color. Default value is Colors.White. /// public Color Color { get { return (Color) GetValue(ColorProperty); } set { SetValueInternal(ColorProperty, value); } } ////// AmbientColor - Color. Default value is Colors.White. /// public Color AmbientColor { get { return (Color) GetValue(AmbientColorProperty); } set { SetValueInternal(AmbientColorProperty, value); } } ////// Brush - Brush. Default value is null. /// public Brush Brush { get { return (Brush) GetValue(BrushProperty); } set { SetValueInternal(BrushProperty, value); } } #endregion Public Properties //----------------------------------------------------- // // Protected Methods // //------------------------------------------------------ #region Protected Methods ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new DiffuseMaterial(); } #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods ////// Critical: This code calls into an unsafe code block /// TreatAsSafe: This code does not return any critical data.It is ok to expose /// Channels are safe to call into and do not go cross domain and cross process /// [SecurityCritical,SecurityTreatAsSafe] internal override void UpdateResource(DUCE.Channel channel, bool skipOnChannelCheck) { // If we're told we can skip the channel check, then we must be on channel Debug.Assert(!skipOnChannelCheck || _duceResource.IsOnChannel(channel)); if (skipOnChannelCheck || _duceResource.IsOnChannel(channel)) { base.UpdateResource(channel, skipOnChannelCheck); // Read values of properties into local variables Brush vBrush = Brush; // Obtain handles for properties that implement DUCE.IResource DUCE.ResourceHandle hBrush = vBrush != null ? ((DUCE.IResource)vBrush).GetHandle(channel) : DUCE.ResourceHandle.Null; // Pack & send command packet DUCE.MILCMD_DIFFUSEMATERIAL data; unsafe { data.Type = MILCMD.MilCmdDiffuseMaterial; data.Handle = _duceResource.GetHandle(channel); data.color = CompositionResourceManager.ColorToMIL_COLORF(Color); data.ambientColor = CompositionResourceManager.ColorToMIL_COLORF(AmbientColor); data.hbrush = hBrush; // Send packed command structure channel.SendCommand( (byte*)&data, sizeof(DUCE.MILCMD_DIFFUSEMATERIAL)); } } } internal override DUCE.ResourceHandle AddRefOnChannelCore(DUCE.Channel channel) { if (_duceResource.CreateOrAddRefOnChannel(channel, System.Windows.Media.Composition.DUCE.ResourceType.TYPE_DIFFUSEMATERIAL)) { Brush vBrush = Brush; if (vBrush != null) ((DUCE.IResource)vBrush).AddRefOnChannel(channel); AddRefOnChannelAnimations(channel); UpdateResource(channel, true /* skip "on channel" check - we already know that we're on channel */ ); } return _duceResource.GetHandle(channel); } internal override void ReleaseOnChannelCore(DUCE.Channel channel) { Debug.Assert(_duceResource.IsOnChannel(channel)); if (_duceResource.ReleaseOnChannel(channel)) { Brush vBrush = Brush; if (vBrush != null) ((DUCE.IResource)vBrush).ReleaseOnChannel(channel); ReleaseOnChannelAnimations(channel); } } internal override DUCE.ResourceHandle GetHandleCore(DUCE.Channel channel) { // Note that we are in a lock here already. return _duceResource.GetHandle(channel); } internal override int GetChannelCountCore() { // must already be in composition lock here return _duceResource.GetChannelCount(); } internal override DUCE.Channel GetChannelCore(int index) { // Note that we are in a lock here already. return _duceResource.GetChannel(index); } #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //------------------------------------------------------ #region Internal Properties // // This property finds the correct initial size for the _effectiveValues store on the // current DependencyObject as a performance optimization // // This includes: // Brush // internal override int EffectiveValuesInitialSize { get { return 1; } } #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties ////// The DependencyProperty for the DiffuseMaterial.Color property. /// public static readonly DependencyProperty ColorProperty = RegisterProperty("Color", typeof(Color), typeof(DiffuseMaterial), Colors.White, new PropertyChangedCallback(ColorPropertyChanged), null, /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); ////// The DependencyProperty for the DiffuseMaterial.AmbientColor property. /// public static readonly DependencyProperty AmbientColorProperty = RegisterProperty("AmbientColor", typeof(Color), typeof(DiffuseMaterial), Colors.White, new PropertyChangedCallback(AmbientColorPropertyChanged), null, /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); ////// The DependencyProperty for the DiffuseMaterial.Brush property. /// public static readonly DependencyProperty BrushProperty = RegisterProperty("Brush", typeof(Brush), typeof(DiffuseMaterial), null, new PropertyChangedCallback(BrushPropertyChanged), null, /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); #endregion Dependency Properties //------------------------------------------------------ // // Internal Fields // //----------------------------------------------------- #region Internal Fields internal System.Windows.Media.Composition.DUCE.MultiChannelResource _duceResource = new System.Windows.Media.Composition.DUCE.MultiChannelResource(); internal static Color s_Color = Colors.White; internal static Color s_AmbientColor = Colors.White; internal static Brush s_Brush = null; #endregion Internal Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
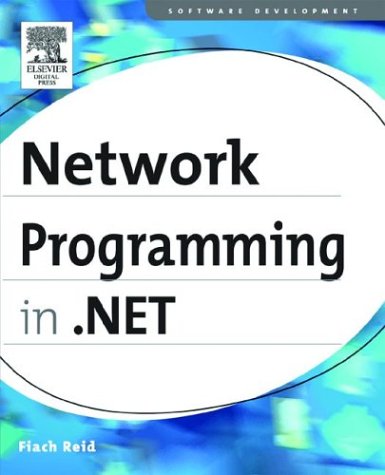
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XamlPointCollectionSerializer.cs
- SecurityRuntime.cs
- Soap12ProtocolReflector.cs
- XmlAnyAttributeAttribute.cs
- MultitargetUtil.cs
- InputLanguageProfileNotifySink.cs
- PackagePartCollection.cs
- ServiceBuildProvider.cs
- ObjectDataSource.cs
- QueryGeneratorBase.cs
- XPathEmptyIterator.cs
- BaseTemplateParser.cs
- ToolStripPanel.cs
- CqlGenerator.cs
- Int16KeyFrameCollection.cs
- AttachedAnnotationChangedEventArgs.cs
- TextSearch.cs
- BitArray.cs
- HtmlLink.cs
- SpeechAudioFormatInfo.cs
- Model3D.cs
- XmlQualifiedName.cs
- ColorConverter.cs
- DbDeleteCommandTree.cs
- Geometry.cs
- EditorAttribute.cs
- EncryptedData.cs
- connectionpool.cs
- ListChangedEventArgs.cs
- ProcessModelSection.cs
- UInt64Storage.cs
- PropertyManager.cs
- ByteStreamGeometryContext.cs
- StaticResourceExtension.cs
- BindingElementCollection.cs
- DoubleCollectionValueSerializer.cs
- SplashScreen.cs
- TreeViewImageKeyConverter.cs
- DataGridViewSelectedRowCollection.cs
- ProjectionCamera.cs
- FlagsAttribute.cs
- HitTestWithGeometryDrawingContextWalker.cs
- Activator.cs
- RtfFormatStack.cs
- FactoryId.cs
- FormClosingEvent.cs
- ClientTarget.cs
- GenericPrincipal.cs
- UriScheme.cs
- TimeZone.cs
- Stack.cs
- EpmCustomContentWriterNodeData.cs
- SocketPermission.cs
- ValidationErrorCollection.cs
- LocationSectionRecord.cs
- AppDomainManager.cs
- WebPartEditorApplyVerb.cs
- Variable.cs
- HttpClientCertificate.cs
- InputScope.cs
- tooltip.cs
- XmlSchemaFacet.cs
- DeviceContext.cs
- ClassDataContract.cs
- ProcessManager.cs
- XmlElementAttributes.cs
- TagPrefixAttribute.cs
- BaseParser.cs
- NullableLongMinMaxAggregationOperator.cs
- ExpandSegment.cs
- DetailsViewInsertedEventArgs.cs
- PageRequestManager.cs
- DoubleLink.cs
- WebPartUtil.cs
- UnhandledExceptionEventArgs.cs
- Graph.cs
- RelationshipManager.cs
- WebEventTraceProvider.cs
- HttpsChannelFactory.cs
- NamedPermissionSet.cs
- _DomainName.cs
- UTF32Encoding.cs
- ScrollViewer.cs
- DateTimeConverter.cs
- CopyNodeSetAction.cs
- DataGridViewColumn.cs
- CacheMode.cs
- SequentialWorkflowRootDesigner.cs
- validationstate.cs
- SqlClientPermission.cs
- NumberFormatter.cs
- XPathSelfQuery.cs
- ConnectionStringSettingsCollection.cs
- MiniConstructorInfo.cs
- XmlChoiceIdentifierAttribute.cs
- SudsWriter.cs
- TableItemStyle.cs
- TextElementEditingBehaviorAttribute.cs
- PageVisual.cs
- SecurityManager.cs