Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / DEVDIV / depot / DevDiv / releases / whidbey / QFE / ndp / fx / src / xsp / System / Web / Security / CookieProtection.cs / 1 / CookieProtection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Security { using System.Security.Cryptography; using System.Web.Configuration; using System.Web.Management; public enum CookieProtection { None, Validation, Encryption, All } internal class CookieProtectionHelper { internal static string Encode (CookieProtection cookieProtection, byte [] buf, int count) { if (cookieProtection == CookieProtection.All || cookieProtection == CookieProtection.Validation) { byte[] bMac = MachineKeySection.HashData (buf, null, 0, count); if (bMac == null || bMac.Length != 20) return null; if (buf.Length >= count + 20) { Buffer.BlockCopy (bMac, 0, buf, count, 20); } else { byte[] bTemp = buf; buf = new byte[count + 20]; Buffer.BlockCopy (bTemp, 0, buf, 0, count); Buffer.BlockCopy (bMac, 0, buf, count, 20); } count += 20; } if (cookieProtection == CookieProtection.All || cookieProtection == CookieProtection.Encryption) { buf = MachineKeySection.EncryptOrDecryptData (true, buf, null, 0, count); count = buf.Length; } if (count < buf.Length) { byte[] bTemp = buf; buf = new byte[count]; Buffer.BlockCopy (bTemp, 0, buf, 0, count); } return HttpServerUtility.UrlTokenEncode(buf); } internal static byte[] Decode (CookieProtection cookieProtection, string data) { byte [] buf = HttpServerUtility.UrlTokenDecode(data); if (buf == null || cookieProtection == CookieProtection.None) return buf; if (cookieProtection == CookieProtection.All || cookieProtection == CookieProtection.Encryption) { buf = MachineKeySection.EncryptOrDecryptData (false, buf, null, 0, buf.Length); if (buf == null) return null; } if (cookieProtection == CookieProtection.All || cookieProtection == CookieProtection.Validation) { ////////////////////////////////////////////////////////////////////// // Step 2: Get the MAC: Last 20 bytes if (buf.Length <= 20) return null; byte[] buf2 = new byte[buf.Length - 20]; Buffer.BlockCopy (buf, 0, buf2, 0, buf2.Length); byte[] bMac = MachineKeySection.HashData (buf2, null, 0, buf2.Length); ////////////////////////////////////////////////////////////////////// // Step 3: Make sure the MAC is correct if (bMac == null || bMac.Length != 20) return null; for (int iter = 0; iter < 20; iter++) if (bMac[iter] != buf[buf2.Length + iter]) return null; buf = buf2; } return buf; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Security { using System.Security.Cryptography; using System.Web.Configuration; using System.Web.Management; public enum CookieProtection { None, Validation, Encryption, All } internal class CookieProtectionHelper { internal static string Encode (CookieProtection cookieProtection, byte [] buf, int count) { if (cookieProtection == CookieProtection.All || cookieProtection == CookieProtection.Validation) { byte[] bMac = MachineKeySection.HashData (buf, null, 0, count); if (bMac == null || bMac.Length != 20) return null; if (buf.Length >= count + 20) { Buffer.BlockCopy (bMac, 0, buf, count, 20); } else { byte[] bTemp = buf; buf = new byte[count + 20]; Buffer.BlockCopy (bTemp, 0, buf, 0, count); Buffer.BlockCopy (bMac, 0, buf, count, 20); } count += 20; } if (cookieProtection == CookieProtection.All || cookieProtection == CookieProtection.Encryption) { buf = MachineKeySection.EncryptOrDecryptData (true, buf, null, 0, count); count = buf.Length; } if (count < buf.Length) { byte[] bTemp = buf; buf = new byte[count]; Buffer.BlockCopy (bTemp, 0, buf, 0, count); } return HttpServerUtility.UrlTokenEncode(buf); } internal static byte[] Decode (CookieProtection cookieProtection, string data) { byte [] buf = HttpServerUtility.UrlTokenDecode(data); if (buf == null || cookieProtection == CookieProtection.None) return buf; if (cookieProtection == CookieProtection.All || cookieProtection == CookieProtection.Encryption) { buf = MachineKeySection.EncryptOrDecryptData (false, buf, null, 0, buf.Length); if (buf == null) return null; } if (cookieProtection == CookieProtection.All || cookieProtection == CookieProtection.Validation) { ////////////////////////////////////////////////////////////////////// // Step 2: Get the MAC: Last 20 bytes if (buf.Length <= 20) return null; byte[] buf2 = new byte[buf.Length - 20]; Buffer.BlockCopy (buf, 0, buf2, 0, buf2.Length); byte[] bMac = MachineKeySection.HashData (buf2, null, 0, buf2.Length); ////////////////////////////////////////////////////////////////////// // Step 3: Make sure the MAC is correct if (bMac == null || bMac.Length != 20) return null; for (int iter = 0; iter < 20; iter++) if (bMac[iter] != buf[buf2.Length + iter]) return null; buf = buf2; } return buf; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
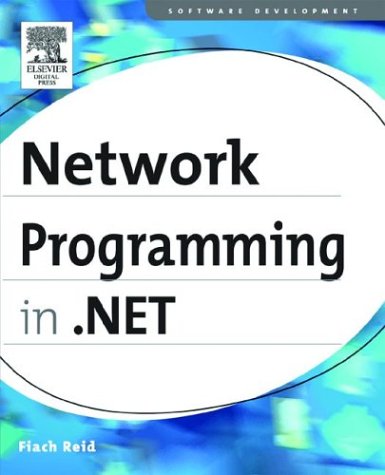
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HyperlinkAutomationPeer.cs
- DbgCompiler.cs
- StorageMappingItemCollection.cs
- ToolStripSystemRenderer.cs
- ImageCollectionCodeDomSerializer.cs
- PaintEvent.cs
- DataTrigger.cs
- CodeObjectCreateExpression.cs
- FlowSwitch.cs
- ErrorTableItemStyle.cs
- COM2TypeInfoProcessor.cs
- DivideByZeroException.cs
- Polygon.cs
- CompensatableTransactionScopeActivityDesigner.cs
- WindowsAuthenticationEventArgs.cs
- SerializationHelper.cs
- SolidBrush.cs
- ToolboxComponentsCreatingEventArgs.cs
- BigInt.cs
- ListViewSelectEventArgs.cs
- PlaceHolder.cs
- ObjectTokenCategory.cs
- DirectoryNotFoundException.cs
- EntityDataSourceWrapperCollection.cs
- VectorKeyFrameCollection.cs
- MessageQueueAccessControlEntry.cs
- HeaderCollection.cs
- HierarchicalDataBoundControl.cs
- OutputCacheModule.cs
- FixedPage.cs
- LoadWorkflowCommand.cs
- XmlDataSourceNodeDescriptor.cs
- WebPartRestoreVerb.cs
- PersistenceContext.cs
- DateTimeUtil.cs
- EventMappingSettings.cs
- SerialPort.cs
- EnumerableWrapperWeakToStrong.cs
- DataServiceKeyAttribute.cs
- PerformanceCountersElement.cs
- ELinqQueryState.cs
- SyndicationDeserializer.cs
- _AutoWebProxyScriptWrapper.cs
- SqlDataRecord.cs
- X509InitiatorCertificateServiceElement.cs
- OleDbPropertySetGuid.cs
- DataIdProcessor.cs
- RangeValidator.cs
- WindowsRegion.cs
- ChtmlMobileTextWriter.cs
- LiteralControl.cs
- ProxyGenerator.cs
- FlowDocumentPageViewerAutomationPeer.cs
- Section.cs
- DiscoveryMessageSequenceGenerator.cs
- SystemIPAddressInformation.cs
- Binding.cs
- UIElement3DAutomationPeer.cs
- AutoResizedEvent.cs
- ItemCollection.cs
- EventLogPermissionEntry.cs
- FileEnumerator.cs
- DataBinder.cs
- CheckBox.cs
- DesignerRegionCollection.cs
- HtmlElementEventArgs.cs
- TraceData.cs
- ExpressionBuilderCollection.cs
- XomlCompiler.cs
- SafeIUnknown.cs
- XmlNodeChangedEventArgs.cs
- HtmlTable.cs
- UrlMappingsSection.cs
- RepeaterCommandEventArgs.cs
- HashRepartitionStream.cs
- SynchronizationValidator.cs
- ModelPropertyImpl.cs
- TemplateLookupAction.cs
- MemoryRecordBuffer.cs
- CodeNamespaceImportCollection.cs
- MetadataException.cs
- IsolatedStorage.cs
- SequentialUshortCollection.cs
- SurrogateDataContract.cs
- MouseCaptureWithinProperty.cs
- MenuEventArgs.cs
- CompiledQueryCacheEntry.cs
- WindowPatternIdentifiers.cs
- UnauthorizedWebPart.cs
- MemberExpressionHelper.cs
- TextTreeText.cs
- SQLMembershipProvider.cs
- ProcessStartInfo.cs
- WorkflowHostingResponseContext.cs
- MenuItemBinding.cs
- WebBrowserHelper.cs
- ImmutableCommunicationTimeouts.cs
- ObjectContext.cs
- DataBindingHandlerAttribute.cs
- ModifierKeysConverter.cs