Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.ServiceModel.Activities / System / ServiceModel / Activities / WorkflowHostingResponseContext.cs / 1305376 / WorkflowHostingResponseContext.cs
//---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.ServiceModel.Activities { using System.Runtime; using System.ServiceModel.Activities.Dispatcher; using System.Threading; [Fx.Tag.XamlVisible(false)] public sealed class WorkflowHostingResponseContext { AsyncWaitHandle responseWaitHandle; WorkflowOperationContext context; object returnValue; object[] outputs; // Used by Creation Endpoint internal WorkflowHostingResponseContext() { this.responseWaitHandle = new AsyncWaitHandle(EventResetMode.AutoReset); } // Used by BookmarkResumption Endpoint internal WorkflowHostingResponseContext(WorkflowOperationContext context) { this.context = context; } public void SendResponse(object returnValue, object[] outputs) { this.returnValue = returnValue; this.outputs = outputs ?? EmptyArray.Allocate(0); if (this.responseWaitHandle != null) { this.responseWaitHandle.Set(); } else { Fx.Assert(this.context != null, "context must not be null!"); if (this.returnValue is Exception) { this.context.SendFault((Exception)this.returnValue); } else { this.context.SendReply(this.returnValue, this.outputs); } } } object GetResponse(out object[] outputs) { if (this.returnValue is Exception) { throw FxTrace.Exception.AsError((Exception)this.returnValue); } outputs = this.outputs; return this.returnValue; } internal IAsyncResult BeginGetResponse(TimeSpan timeout, AsyncCallback callback, object state) { Fx.Assert(this.responseWaitHandle != null, "this.responseWaitHandle must not be null!"); return GetResponseAsyncResult.Create(this, timeout, callback, state); } internal object EndGetResponse(IAsyncResult result, out object[] outputs) { return GetResponseAsyncResult.End(result, out outputs); } class GetResponseAsyncResult : AsyncResult { static Action
Link Menu
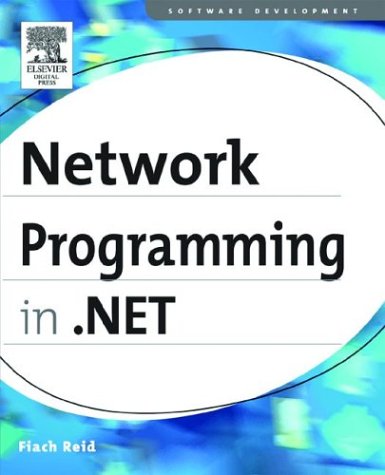
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TypeTypeConverter.cs
- PropertyGeneratedEventArgs.cs
- Span.cs
- TextServicesPropertyRanges.cs
- LiteralControl.cs
- _SslState.cs
- AnnotationResourceChangedEventArgs.cs
- EntryPointNotFoundException.cs
- UserMapPath.cs
- XmlTextWriter.cs
- HitTestParameters3D.cs
- BitVec.cs
- ClientTarget.cs
- DependentList.cs
- TextRunCacheImp.cs
- ContainerControlDesigner.cs
- SafeIUnknown.cs
- InstanceCreationEditor.cs
- XmlSchemaCollection.cs
- CharAnimationUsingKeyFrames.cs
- HMAC.cs
- ToolStripContainer.cs
- SaveFileDialogDesigner.cs
- ColorPalette.cs
- XmlSignatureProperties.cs
- SQLInt32.cs
- DirectionalLight.cs
- QilVisitor.cs
- Sorting.cs
- SoapIncludeAttribute.cs
- panel.cs
- ListItemCollection.cs
- TraversalRequest.cs
- ApplicationInterop.cs
- NamedElement.cs
- StateMachineExecutionState.cs
- Point4DValueSerializer.cs
- CriticalFinalizerObject.cs
- CustomTrackingQuery.cs
- SizeAnimationUsingKeyFrames.cs
- LineGeometry.cs
- HorizontalAlignConverter.cs
- Tokenizer.cs
- MouseButton.cs
- MergeFilterQuery.cs
- EventSinkActivityDesigner.cs
- ipaddressinformationcollection.cs
- TextInfo.cs
- WizardPanelChangingEventArgs.cs
- WebPartDeleteVerb.cs
- ActivityDesigner.cs
- Matrix3D.cs
- TextStore.cs
- MergablePropertyAttribute.cs
- safelinkcollection.cs
- TextEndOfSegment.cs
- SHA512.cs
- PriorityItem.cs
- Util.cs
- DataObject.cs
- XmlImplementation.cs
- Directory.cs
- PropertyGroupDescription.cs
- PartitionerStatic.cs
- Atom10FormatterFactory.cs
- RenamedEventArgs.cs
- FormsAuthenticationTicket.cs
- StateChangeEvent.cs
- compensatingcollection.cs
- TableParagraph.cs
- XmlEntity.cs
- ViewCellRelation.cs
- TextServicesPropertyRanges.cs
- PropagatorResult.cs
- ObjectKeyFrameCollection.cs
- VisualTreeHelper.cs
- ProgressBar.cs
- ApplyTemplatesAction.cs
- VisemeEventArgs.cs
- ErrorEventArgs.cs
- XmlDataSource.cs
- CloudCollection.cs
- Crypto.cs
- UrlAuthFailureHandler.cs
- ModuleBuilderData.cs
- CollectionDataContract.cs
- BeginStoryboard.cs
- HorizontalAlignConverter.cs
- TemplateApplicationHelper.cs
- ProjectionPathSegment.cs
- Trigger.cs
- TimelineClockCollection.cs
- XmlAtomicValue.cs
- HttpModuleActionCollection.cs
- GridViewSelectEventArgs.cs
- MailAddressCollection.cs
- SaveFileDialog.cs
- XmlBinaryWriterSession.cs
- OleDbConnection.cs
- WeakEventTable.cs