Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / TransactionBridge / Microsoft / Transactions / Wsat / Recovery / LogEntrySerialization.cs / 1 / LogEntrySerialization.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- // This file contains the main entry points for the rest of log entry serialization // and deserialization using System; using System.Diagnostics; using System.IO; using System.ServiceModel; using Microsoft.Transactions.Bridge; using Microsoft.Transactions.Wsat.Messaging; using Microsoft.Transactions.Wsat.Protocol; using DiagnosticUtility = Microsoft.Transactions.Bridge.DiagnosticUtility; namespace Microsoft.Transactions.Wsat.Recovery { class LogEntrySerialization { ProtocolState state; ProtocolVersion protocolVersion; int maxLogEntrySize; public LogEntrySerialization(ProtocolState state) { if(state == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException ("state")); this.state = state; this.protocolVersion = state.ProtocolVersion; this.maxLogEntrySize = this.state.TransactionManager.MaxLogEntrySize; } public LogEntrySerialization(int maxLogEntrySize, ProtocolVersion protocolVersion) { this.protocolVersion = protocolVersion; this.maxLogEntrySize = maxLogEntrySize; } public byte[] Serialize(CoordinatorEnlistment coordinator) { Enlistment enlistment = coordinator.Enlistment; LogEntry logEntry = new LogEntry(enlistment.RemoteTransactionId, enlistment.LocalTransactionId, coordinator.EnlistmentId, coordinator.CoordinatorProxy.To); return SerializeLogEntry(logEntry); } public byte[] Serialize(ParticipantEnlistment participant) { Enlistment enlistment = participant.Enlistment; LogEntry logEntry = new LogEntry(enlistment.RemoteTransactionId, enlistment.LocalTransactionId, participant.EnlistmentId, participant.ParticipantProxy.To); return SerializeLogEntry(logEntry); } public ParticipantEnlistment DeserializeParticipant(Enlistment enlistment) { byte[] buffer = enlistment.GetRecoveryData(); LogEntry logEntry = DeserializeLogEntry(buffer, enlistment.LocalTransactionId); enlistment.RemoteTransactionId = logEntry.RemoteTransactionId; return new ParticipantEnlistment(state, enlistment, logEntry.LocalEnlistmentId, logEntry.Endpoint); } public CoordinatorEnlistment DeserializeCoordinator(Enlistment enlistment) { byte[] buffer = enlistment.GetRecoveryData(); LogEntry logEntry = DeserializeLogEntry(buffer, enlistment.LocalTransactionId); enlistment.RemoteTransactionId = logEntry.RemoteTransactionId; return new CoordinatorEnlistment(state, enlistment, logEntry.LocalEnlistmentId, logEntry.Endpoint); } // // Implementation // byte[] SerializeLogEntry(LogEntry logEntry) { LogEntrySerializer serializer = new WsATv1LogEntrySerializer(logEntry, this.protocolVersion); byte[] buffer = serializer.Serialize(); if (DebugTrace.Verbose) { DebugTrace.Trace(TraceLevel.Verbose, "Serialized {0} byte buffer", buffer.Length); } if (buffer.Length > this.maxLogEntrySize) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new SerializationException(SR.GetString(SR.SerializationLogEntryTooBig, buffer.Length))); } return buffer; } LogEntry DeserializeLogEntry(byte[] buffer, Guid localTransactionId) { if (DebugTrace.Verbose) { DebugTrace.Trace(TraceLevel.Verbose, "Deserializing {0} byte buffer", buffer.Length); } // Deserialize the header MemoryStream mem = new MemoryStream(buffer, 0, buffer.Length, false, true); LogEntry logEntry = DeserializeHeader(mem, localTransactionId); // Find a deserializer for the extended endpoint information LogEntryDeserializer deserializer = CreateDeserializer(mem, logEntry); return deserializer.Deserialize(); } LogEntry DeserializeHeader(MemoryStream mem, Guid localTransactionId) { // Find the right header deserializer LogEntryHeaderDeserializer headerDeserializer; LogEntryHeaderVersion headerVersion = (LogEntryHeaderVersion) SerializationUtils.ReadByte(mem); switch (headerVersion) { case LogEntryHeaderVersion.v1: headerDeserializer = new LogEntryHeaderv1Deserializer(mem, localTransactionId); break; default: // An invalid Enum value on this internal code path indicates // a product bug and violates assumptions about // valid values in MSDTC. DiagnosticUtility.FailFast("Log entry with unsupported major version"); headerDeserializer = null; // Keep the compiler happy break; } // Deserialize the header return headerDeserializer.DeserializeHeader(); } LogEntryDeserializer CreateDeserializer(MemoryStream mem, LogEntry logEntry) { return new WsATv1LogEntryDeserializer(mem, logEntry, this.protocolVersion); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
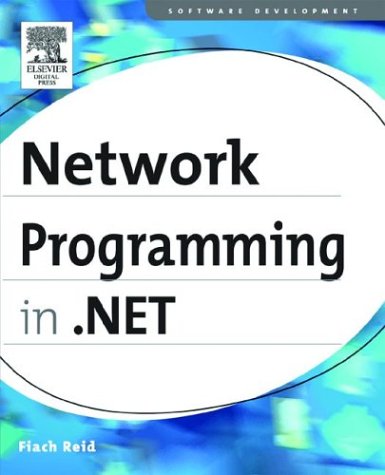
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlUtil.cs
- ContextMenuAutomationPeer.cs
- ToolstripProfessionalRenderer.cs
- PeerNameRecord.cs
- HeaderFilter.cs
- WebPartPersonalization.cs
- DataSourceNameHandler.cs
- KerberosRequestorSecurityToken.cs
- SplitContainer.cs
- EpmHelper.cs
- NativeMethods.cs
- SchemaLookupTable.cs
- DispatcherEventArgs.cs
- ChannelBinding.cs
- Attributes.cs
- ApplyImportsAction.cs
- TdsParameterSetter.cs
- SimpleParser.cs
- TimeIntervalCollection.cs
- ThemeableAttribute.cs
- MarshalByRefObject.cs
- SmtpAuthenticationManager.cs
- NavigationProgressEventArgs.cs
- OrderToken.cs
- EmbeddedMailObjectsCollection.cs
- SchemaMerger.cs
- PolicyLevel.cs
- HtmlInputText.cs
- GlyphTypeface.cs
- ToolStripPanelCell.cs
- MDIClient.cs
- ValidationUtility.cs
- CodeBlockBuilder.cs
- DataGridViewColumnTypeEditor.cs
- xmlglyphRunInfo.cs
- RsaSecurityTokenAuthenticator.cs
- DataGridViewComboBoxCell.cs
- BulletedListEventArgs.cs
- SoundPlayerAction.cs
- RuleSet.cs
- SerializerDescriptor.cs
- EncoderParameters.cs
- TerminatingOperationBehavior.cs
- TreeViewItemAutomationPeer.cs
- UniqueEventHelper.cs
- CookieParameter.cs
- PropertyGridEditorPart.cs
- DataListItem.cs
- HebrewCalendar.cs
- TraceUtils.cs
- TagMapInfo.cs
- NullEntityWrapper.cs
- loginstatus.cs
- Int16AnimationBase.cs
- Encoder.cs
- SimpleMailWebEventProvider.cs
- ElementInit.cs
- base64Transforms.cs
- DataGridColumnsPage.cs
- ChangeInterceptorAttribute.cs
- FlowDocumentPaginator.cs
- initElementDictionary.cs
- RuntimeResourceSet.cs
- ConfigurationSection.cs
- WindowsHyperlink.cs
- IsolationInterop.cs
- SqlExpander.cs
- ScrollPatternIdentifiers.cs
- VoiceSynthesis.cs
- QueryOptionExpression.cs
- WpfKnownMember.cs
- CommonXSendMessage.cs
- DataGridSortCommandEventArgs.cs
- SequentialOutput.cs
- SQLInt64.cs
- ObjectListDataBindEventArgs.cs
- SqlEnums.cs
- LogLogRecordHeader.cs
- ValueUnavailableException.cs
- SocketPermission.cs
- PiiTraceSource.cs
- FrameworkReadOnlyPropertyMetadata.cs
- ProfileGroupSettingsCollection.cs
- InstanceHandleConflictException.cs
- MaterializeFromAtom.cs
- commandenforcer.cs
- RenderData.cs
- SymmetricKeyWrap.cs
- ElapsedEventArgs.cs
- RemoveStoryboard.cs
- HiddenFieldPageStatePersister.cs
- DataGridViewElement.cs
- CustomDictionarySources.cs
- mactripleDES.cs
- xsdvalidator.cs
- LocationUpdates.cs
- WindowsStatic.cs
- ArrayConverter.cs
- VolatileResourceManager.cs
- QilList.cs