Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / DynamicData / DynamicData / SimpleFieldTemplateUserControl.cs / 1305376 / SimpleFieldTemplateUserControl.cs
namespace System.Web.DynamicData { using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Web.UI.WebControls; using System.Web.UI; using System.Collections; using System.Collections.Specialized; // Dynamically creates a field template for text or boolean fields internal class SimpleFieldTemplateUserControl : FieldTemplateUserControl { private const string TextBoxID = "TextBox"; // Used for edit scenariors, since we only have one value for these // simple fieldtemplates private Func
Link Menu
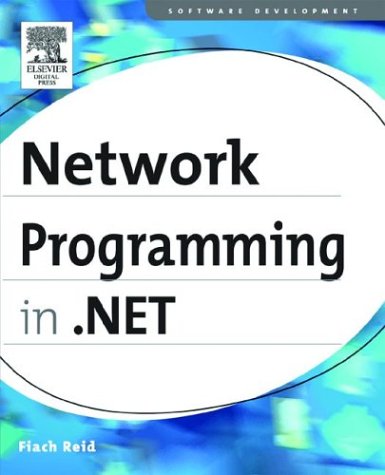
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TypeConverters.cs
- WindowsFormsHelpers.cs
- ExpressionLink.cs
- SecurityUtils.cs
- BrowserCapabilitiesCodeGenerator.cs
- ClientConfigurationSystem.cs
- OverloadGroupAttribute.cs
- IdlingCommunicationPool.cs
- EncoderParameter.cs
- SQLDecimalStorage.cs
- Camera.cs
- VirtualPathProvider.cs
- OpCellTreeNode.cs
- SqlTypeConverter.cs
- ServiceHttpHandlerFactory.cs
- PageCache.cs
- Property.cs
- Rectangle.cs
- ApplicationSecurityInfo.cs
- ApplicationSettingsBase.cs
- XmlEntityReference.cs
- NativeMethods.cs
- ProxyHelper.cs
- TagPrefixInfo.cs
- InputProviderSite.cs
- RsaKeyGen.cs
- AutomationPeer.cs
- PreProcessInputEventArgs.cs
- CreateUserWizard.cs
- DateTimeConverter2.cs
- BezierSegment.cs
- SynchronizedInputHelper.cs
- XmlSchemaSequence.cs
- CfgRule.cs
- ExpressionEditorAttribute.cs
- MarshalByRefObject.cs
- ConfigXmlCDataSection.cs
- TreeNode.cs
- DiscoveryClientReferences.cs
- _IPv4Address.cs
- BoundingRectTracker.cs
- XsdDuration.cs
- ModelPropertyImpl.cs
- DefaultPropertiesToSend.cs
- SkipQueryOptionExpression.cs
- Command.cs
- ContextDataSourceContextData.cs
- BamlBinaryReader.cs
- ModulesEntry.cs
- MetabaseReader.cs
- MobileTemplatedControlDesigner.cs
- XmlNamespaceMapping.cs
- SqlDataRecord.cs
- RemotingHelper.cs
- WebMessageEncoderFactory.cs
- DataGridViewCheckBoxColumn.cs
- DataSourceCacheDurationConverter.cs
- CounterSetInstance.cs
- SpellerInterop.cs
- EndEvent.cs
- TextFormatter.cs
- AuthenticationSection.cs
- PeerTransportSecurityElement.cs
- ListComponentEditorPage.cs
- ResXBuildProvider.cs
- XPathNode.cs
- ALinqExpressionVisitor.cs
- WebPartDisplayModeEventArgs.cs
- ChangeBlockUndoRecord.cs
- FontStretchConverter.cs
- TreeNodeBindingDepthConverter.cs
- CalendarTable.cs
- XamlTypeMapperSchemaContext.cs
- HandlerBase.cs
- FileInfo.cs
- WebPartCloseVerb.cs
- Component.cs
- AutomationPatternInfo.cs
- TypeReference.cs
- StrokeNodeOperations.cs
- StateWorkerRequest.cs
- SmtpSection.cs
- ComplexTypeEmitter.cs
- PaperSource.cs
- EmbossBitmapEffect.cs
- EntityDescriptor.cs
- HwndMouseInputProvider.cs
- Typeface.cs
- GrammarBuilderWildcard.cs
- UserMapPath.cs
- SymmetricAlgorithm.cs
- ContentIterators.cs
- CommonDialog.cs
- DataGridColumnCollection.cs
- ConfigurationSection.cs
- ThemeableAttribute.cs
- TreeIterator.cs
- WindowCollection.cs
- SafePEFileHandle.cs
- WinEventWrap.cs