Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataEntity / System / Data / EntityModel / SchemaObjectModel / ReturnType.cs / 1 / ReturnType.cs
using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.Diagnostics; using System.Globalization; using System.Xml; using System.Xml.Schema; using System.Data; using System.IO; using System.Data.Metadata.Edm; using System.Data.Entity; namespace System.Data.EntityModel.SchemaObjectModel { class ReturnType : FacetEnabledSchemaElement { #region constructor ////// /// /// internal ReturnType(Function parentElement) : base(parentElement) { _typeUsageBuilder = new TypeUsageBuilder(this); } #endregion internal override SchemaElement Clone(SchemaElement parentElement) { ReturnType parameter = new ReturnType((Function)parentElement); parameter._type = _type; parameter.Name = this.Name; parameter._typeUsageBuilder = this._typeUsageBuilder; parameter._unresolvedType = this._unresolvedType; return parameter; } protected override bool HandleAttribute(XmlReader reader) { if (base.HandleAttribute(reader)) { return true; } else if (CanHandleAttribute(reader, XmlConstants.TypeElement)) { HandleTypeAttribute(reader); return true; } return false; } #region Private Methods ////// /// /// private void HandleTypeAttribute(XmlReader reader) { Debug.Assert(reader != null); Debug.Assert(this._unresolvedType == null); string type; if (!Utils.GetString(Schema, reader, out type)) return; if (!Utils.ValidateDottedName(Schema, reader, type)) return; this.UnresolvedType = type; } #endregion internal override void ResolveTopLevelNames() { Debug.Assert(!this.ParentElement.IsFunctionImport, "FunctionImports have return type as an attribute, so we should NEVER see them here"); base.ResolveTopLevelNames(); if (Schema.ResolveTypeName(this, UnresolvedType, out _type)) { if (!(_type is ScalarType)) { if (Schema.DataModel != SchemaDataModelOption.ProviderManifestModel) { AddError(ErrorCode.FunctionWithNonScalarTypeNotSupported, EdmSchemaErrorSeverity.Error, this, System.Data.Entity.Strings.FunctionWithNonScalarTypeNotSupported(_type.FQName, this.FQName)); } else { AddError(ErrorCode.FunctionWithNonScalarTypeNotSupported, EdmSchemaErrorSeverity.Error, this, System.Data.Entity.Strings.FunctionWithNonEdmTypeNotSupported(_type.FQName, this.FQName)); } } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.Diagnostics; using System.Globalization; using System.Xml; using System.Xml.Schema; using System.Data; using System.IO; using System.Data.Metadata.Edm; using System.Data.Entity; namespace System.Data.EntityModel.SchemaObjectModel { class ReturnType : FacetEnabledSchemaElement { #region constructor ////// /// /// internal ReturnType(Function parentElement) : base(parentElement) { _typeUsageBuilder = new TypeUsageBuilder(this); } #endregion internal override SchemaElement Clone(SchemaElement parentElement) { ReturnType parameter = new ReturnType((Function)parentElement); parameter._type = _type; parameter.Name = this.Name; parameter._typeUsageBuilder = this._typeUsageBuilder; parameter._unresolvedType = this._unresolvedType; return parameter; } protected override bool HandleAttribute(XmlReader reader) { if (base.HandleAttribute(reader)) { return true; } else if (CanHandleAttribute(reader, XmlConstants.TypeElement)) { HandleTypeAttribute(reader); return true; } return false; } #region Private Methods ////// /// /// private void HandleTypeAttribute(XmlReader reader) { Debug.Assert(reader != null); Debug.Assert(this._unresolvedType == null); string type; if (!Utils.GetString(Schema, reader, out type)) return; if (!Utils.ValidateDottedName(Schema, reader, type)) return; this.UnresolvedType = type; } #endregion internal override void ResolveTopLevelNames() { Debug.Assert(!this.ParentElement.IsFunctionImport, "FunctionImports have return type as an attribute, so we should NEVER see them here"); base.ResolveTopLevelNames(); if (Schema.ResolveTypeName(this, UnresolvedType, out _type)) { if (!(_type is ScalarType)) { if (Schema.DataModel != SchemaDataModelOption.ProviderManifestModel) { AddError(ErrorCode.FunctionWithNonScalarTypeNotSupported, EdmSchemaErrorSeverity.Error, this, System.Data.Entity.Strings.FunctionWithNonScalarTypeNotSupported(_type.FQName, this.FQName)); } else { AddError(ErrorCode.FunctionWithNonScalarTypeNotSupported, EdmSchemaErrorSeverity.Error, this, System.Data.Entity.Strings.FunctionWithNonEdmTypeNotSupported(_type.FQName, this.FQName)); } } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
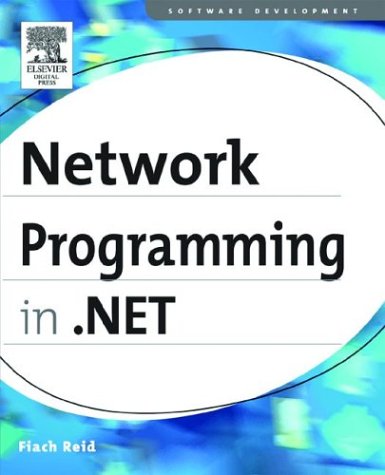
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- tibetanshape.cs
- EncryptedPackage.cs
- TextBoxView.cs
- RenderData.cs
- CodeMemberMethod.cs
- BufferedStream2.cs
- SequentialOutput.cs
- HttpContext.cs
- BehaviorEditorPart.cs
- NamedObject.cs
- CreateUserErrorEventArgs.cs
- WpfKnownMemberInvoker.cs
- CharConverter.cs
- MenuItemStyleCollection.cs
- ImportCatalogPart.cs
- PropertyGridView.cs
- UserNameServiceElement.cs
- ellipse.cs
- XmlSchemaComplexType.cs
- SqlClientPermission.cs
- typedescriptorpermissionattribute.cs
- ListBindingHelper.cs
- safex509handles.cs
- DoWorkEventArgs.cs
- SQLBinary.cs
- AssemblyName.cs
- WorkflowDesignerMessageFilter.cs
- ExtendedPropertyCollection.cs
- CatalogZoneBase.cs
- DetailsView.cs
- SqlCacheDependencyDatabaseCollection.cs
- FlowDocumentPage.cs
- DesignerHierarchicalDataSourceView.cs
- IsolatedStorageException.cs
- BoundColumn.cs
- ByteViewer.cs
- WindowsRegion.cs
- ConstraintStruct.cs
- WindowHideOrCloseTracker.cs
- StaticDataManager.cs
- Compiler.cs
- NameTable.cs
- TripleDESCryptoServiceProvider.cs
- CollectionConverter.cs
- StackOverflowException.cs
- ToolStripSplitStackLayout.cs
- CompositionTarget.cs
- MimeTypeAttribute.cs
- ScriptControlManager.cs
- RootProfilePropertySettingsCollection.cs
- Main.cs
- SqlReferenceCollection.cs
- ClientProxyGenerator.cs
- DataGridViewCellErrorTextNeededEventArgs.cs
- PointCollection.cs
- ObjectCacheSettings.cs
- HealthMonitoringSectionHelper.cs
- SiteOfOriginPart.cs
- ColorMatrix.cs
- ToolStripOverflowButton.cs
- SessionMode.cs
- SecurityPolicySection.cs
- TaskFileService.cs
- CommandHelpers.cs
- FlowNode.cs
- XmlTypeAttribute.cs
- AlphaSortedEnumConverter.cs
- TemplateInstanceAttribute.cs
- Package.cs
- DropSource.cs
- ConnectAlgorithms.cs
- SqlLiftIndependentRowExpressions.cs
- WebControl.cs
- TemplateField.cs
- X509Certificate2Collection.cs
- FixedPageStructure.cs
- WindowsIdentity.cs
- RawKeyboardInputReport.cs
- DefaultShape.cs
- ApplicationManager.cs
- Line.cs
- SourceFileInfo.cs
- StructuralType.cs
- NamedElement.cs
- EdmTypeAttribute.cs
- ToolStripPanel.cs
- StickyNoteHelper.cs
- MetabaseServerConfig.cs
- KeyedHashAlgorithm.cs
- DbException.cs
- TypefaceMap.cs
- DataBoundLiteralControl.cs
- QilIterator.cs
- Block.cs
- ClientConfigurationSystem.cs
- TileModeValidation.cs
- JournalEntryStack.cs
- HttpPostedFile.cs
- CompilerErrorCollection.cs
- UpDownBase.cs