Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / Misc / GDI / WindowsRegion.cs / 1 / WindowsRegion.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- #if WINFORMS_NAMESPACE namespace System.Windows.Forms.Internal #elif DRAWING_NAMESPACE namespace System.Drawing.Internal #else namespace System.Experimental.Gdi #endif { using System; using System.Internal; using System.Runtime.InteropServices; using System.ComponentModel; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System.Drawing; using System.Globalization; ////// #if WINFORMS_PUBLIC_GRAPHICS_LIBRARY public #else internal #endif sealed partial class WindowsRegion : MarshalByRefObject, ICloneable, IDisposable { [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Reliability", "CA2006:UseSafeHandleToEncapsulateNativeResources")] private IntPtr nativeHandle; // The hRegion, this class always takes ownership of the hRegion. private bool ownHandle; #if GDI_FINALIZATION_WATCH private string AllocationSite = DbgUtil.StackTrace; #endif ////// Encapsulates a GDI Region object. /// ////// private WindowsRegion() { } ////// public WindowsRegion(Rectangle rect) { CreateRegion(rect); } ////// public WindowsRegion(int x, int y, int width, int height) { CreateRegion(new Rectangle(x,y,width, height)); } // Consider implementing a constructor that calls ExtCreateRegion(XFORM lpXform, DWORD nCount, RGNDATA lpRgnData) if needed. ////// Creates a WindowsRegion from a region handle, if 'takeOwnership' is true, the handle is added to the HandleCollector /// and is removed & destroyed on dispose. /// public static WindowsRegion FromHregion(IntPtr hRegion, bool takeOwnership) { WindowsRegion wr = new WindowsRegion(); // Note: Passing IntPtr.Zero for hRegion is ok. GDI+ infinite regions will have hRegion == null. // GDI's SelectClipRgn interprets null region handle as resetting the clip region (all region will be available for painting). if( hRegion != IntPtr.Zero ) { wr.nativeHandle = hRegion; if (takeOwnership) { wr.ownHandle = true; System.Internal.HandleCollector.Add(hRegion, IntSafeNativeMethods.CommonHandles.GDI); } } return wr; } ////// Creates a WindowsRegion from a System.Drawing.Region. /// public static WindowsRegion FromRegion( Region region, Graphics g ){ if (region.IsInfinite(g)){ // An infinite region would cover the entire device region which is the same as // not having a clipping region. Observe that this is not the same as having an // empty region, which when clipping to it has the effect of excluding the entire // device region. // To remove the clip region from a dc the SelectClipRgn() function needs to be // called with a null region ptr - that's why we use the empty constructor here. // GDI+ will return IntPtr.Zero for Region.GetHrgn(Graphics) when the region is // Infinite. return new WindowsRegion(); } return WindowsRegion.FromHregion(region.GetHrgn(g), true); } ////// public object Clone() { // WARNING: WindowsRegion currently supports rectangulare regions only, if the WindowsRegion was created // from an HRegion and it is not rectangular this method won't work as expected. // Note: This method is currently not used and is here just to implement ICloneable. return this.IsInfinite ? new WindowsRegion() : new WindowsRegion(this.ToRectangle()); } ////// Combines region1 & region2 into this region. The regions cannot be null. /// The three regions need not be distinct. For example, the sourceRgn1 can equal this region. /// public IntNativeMethods.RegionFlags CombineRegion(WindowsRegion region1, WindowsRegion region2, RegionCombineMode mode) { return IntUnsafeNativeMethods.CombineRgn(new HandleRef(this, this.HRegion), new HandleRef(region1, region1.HRegion), new HandleRef(region2, region2.HRegion), mode); } ////// private void CreateRegion(Rectangle rect) { Debug.Assert(nativeHandle == IntPtr.Zero, "nativeHandle should be null, we're leaking handle"); this.nativeHandle = IntSafeNativeMethods.CreateRectRgn(rect.X, rect.Y, rect.X+rect.Width, rect.Y+rect.Height); ownHandle = true; } ////// public void Dispose() { Dispose(true); } ////// public void Dispose(bool disposing) { if (this.nativeHandle != IntPtr.Zero) { DbgUtil.AssertFinalization(this, disposing); if( this.ownHandle ) { IntUnsafeNativeMethods.DeleteObject(new HandleRef(this, this.nativeHandle)); } this.nativeHandle = IntPtr.Zero; if (disposing) { GC.SuppressFinalize(this); } } } ////// ~WindowsRegion() { Dispose(false); } ////// The native region handle. /// public IntPtr HRegion { get { return this.nativeHandle; } } ////// public bool IsInfinite { get { return this.nativeHandle == IntPtr.Zero; } } ////// A rectangle representing the window region set with the SetWindowRgn function. /// public Rectangle ToRectangle() { if( this.IsInfinite ) { return new Rectangle( -Int32.MaxValue, -Int32.MaxValue, Int32.MaxValue, Int32.MaxValue ); } IntNativeMethods.RECT rect = new IntNativeMethods.RECT(); IntUnsafeNativeMethods.GetRgnBox(new HandleRef(this, this.nativeHandle), ref rect); return new Rectangle(new Point(rect.left, rect.top), rect.Size); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- #if WINFORMS_NAMESPACE namespace System.Windows.Forms.Internal #elif DRAWING_NAMESPACE namespace System.Drawing.Internal #else namespace System.Experimental.Gdi #endif { using System; using System.Internal; using System.Runtime.InteropServices; using System.ComponentModel; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System.Drawing; using System.Globalization; ////// #if WINFORMS_PUBLIC_GRAPHICS_LIBRARY public #else internal #endif sealed partial class WindowsRegion : MarshalByRefObject, ICloneable, IDisposable { [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Reliability", "CA2006:UseSafeHandleToEncapsulateNativeResources")] private IntPtr nativeHandle; // The hRegion, this class always takes ownership of the hRegion. private bool ownHandle; #if GDI_FINALIZATION_WATCH private string AllocationSite = DbgUtil.StackTrace; #endif ////// Encapsulates a GDI Region object. /// ////// private WindowsRegion() { } ////// public WindowsRegion(Rectangle rect) { CreateRegion(rect); } ////// public WindowsRegion(int x, int y, int width, int height) { CreateRegion(new Rectangle(x,y,width, height)); } // Consider implementing a constructor that calls ExtCreateRegion(XFORM lpXform, DWORD nCount, RGNDATA lpRgnData) if needed. ////// Creates a WindowsRegion from a region handle, if 'takeOwnership' is true, the handle is added to the HandleCollector /// and is removed & destroyed on dispose. /// public static WindowsRegion FromHregion(IntPtr hRegion, bool takeOwnership) { WindowsRegion wr = new WindowsRegion(); // Note: Passing IntPtr.Zero for hRegion is ok. GDI+ infinite regions will have hRegion == null. // GDI's SelectClipRgn interprets null region handle as resetting the clip region (all region will be available for painting). if( hRegion != IntPtr.Zero ) { wr.nativeHandle = hRegion; if (takeOwnership) { wr.ownHandle = true; System.Internal.HandleCollector.Add(hRegion, IntSafeNativeMethods.CommonHandles.GDI); } } return wr; } ////// Creates a WindowsRegion from a System.Drawing.Region. /// public static WindowsRegion FromRegion( Region region, Graphics g ){ if (region.IsInfinite(g)){ // An infinite region would cover the entire device region which is the same as // not having a clipping region. Observe that this is not the same as having an // empty region, which when clipping to it has the effect of excluding the entire // device region. // To remove the clip region from a dc the SelectClipRgn() function needs to be // called with a null region ptr - that's why we use the empty constructor here. // GDI+ will return IntPtr.Zero for Region.GetHrgn(Graphics) when the region is // Infinite. return new WindowsRegion(); } return WindowsRegion.FromHregion(region.GetHrgn(g), true); } ////// public object Clone() { // WARNING: WindowsRegion currently supports rectangulare regions only, if the WindowsRegion was created // from an HRegion and it is not rectangular this method won't work as expected. // Note: This method is currently not used and is here just to implement ICloneable. return this.IsInfinite ? new WindowsRegion() : new WindowsRegion(this.ToRectangle()); } ////// Combines region1 & region2 into this region. The regions cannot be null. /// The three regions need not be distinct. For example, the sourceRgn1 can equal this region. /// public IntNativeMethods.RegionFlags CombineRegion(WindowsRegion region1, WindowsRegion region2, RegionCombineMode mode) { return IntUnsafeNativeMethods.CombineRgn(new HandleRef(this, this.HRegion), new HandleRef(region1, region1.HRegion), new HandleRef(region2, region2.HRegion), mode); } ////// private void CreateRegion(Rectangle rect) { Debug.Assert(nativeHandle == IntPtr.Zero, "nativeHandle should be null, we're leaking handle"); this.nativeHandle = IntSafeNativeMethods.CreateRectRgn(rect.X, rect.Y, rect.X+rect.Width, rect.Y+rect.Height); ownHandle = true; } ////// public void Dispose() { Dispose(true); } ////// public void Dispose(bool disposing) { if (this.nativeHandle != IntPtr.Zero) { DbgUtil.AssertFinalization(this, disposing); if( this.ownHandle ) { IntUnsafeNativeMethods.DeleteObject(new HandleRef(this, this.nativeHandle)); } this.nativeHandle = IntPtr.Zero; if (disposing) { GC.SuppressFinalize(this); } } } ////// ~WindowsRegion() { Dispose(false); } ////// The native region handle. /// public IntPtr HRegion { get { return this.nativeHandle; } } ////// public bool IsInfinite { get { return this.nativeHandle == IntPtr.Zero; } } ////// A rectangle representing the window region set with the SetWindowRgn function. /// public Rectangle ToRectangle() { if( this.IsInfinite ) { return new Rectangle( -Int32.MaxValue, -Int32.MaxValue, Int32.MaxValue, Int32.MaxValue ); } IntNativeMethods.RECT rect = new IntNativeMethods.RECT(); IntUnsafeNativeMethods.GetRgnBox(new HandleRef(this, this.nativeHandle), ref rect); return new Rectangle(new Point(rect.left, rect.top), rect.Size); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
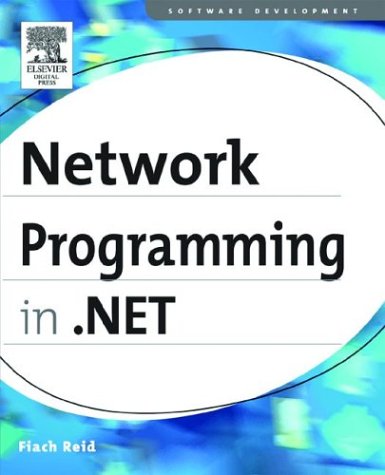
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- QuaternionRotation3D.cs
- CounterCreationData.cs
- RegexFCD.cs
- DesignerValidatorAdapter.cs
- KnownBoxes.cs
- MILUtilities.cs
- EmptyReadOnlyDictionaryInternal.cs
- ActivityBindForm.cs
- RegexMatch.cs
- FontStretches.cs
- OverflowException.cs
- SqlBulkCopy.cs
- TabItem.cs
- EntityContainerEntitySetDefiningQuery.cs
- CaseInsensitiveComparer.cs
- TemplatedControlDesigner.cs
- AsyncContentLoadedEventArgs.cs
- ListChangedEventArgs.cs
- OdbcFactory.cs
- EpmContentSerializerBase.cs
- FileClassifier.cs
- ContentElementAutomationPeer.cs
- AdRotatorDesigner.cs
- StrongNamePublicKeyBlob.cs
- MenuItemCollection.cs
- EntityDataSourceSelectedEventArgs.cs
- ProgressBarBrushConverter.cs
- XMLSchema.cs
- ScrollProperties.cs
- DataListItem.cs
- FragmentQueryProcessor.cs
- CorrelationToken.cs
- SoapSchemaExporter.cs
- PathFigure.cs
- FragmentQueryKB.cs
- Int16.cs
- SqlBulkCopyColumnMapping.cs
- InternalControlCollection.cs
- SoundPlayer.cs
- TextFormatterContext.cs
- MasterPage.cs
- DocumentXPathNavigator.cs
- StringArrayConverter.cs
- ExtendedTransformFactory.cs
- BaseParser.cs
- CompilerHelpers.cs
- serverconfig.cs
- SimpleHandlerFactory.cs
- ThreadStartException.cs
- InvalidFilterCriteriaException.cs
- TextOnlyOutput.cs
- StateBag.cs
- DataBoundControlActionList.cs
- CapabilitiesAssignment.cs
- basevalidator.cs
- ScriptingSectionGroup.cs
- XPathDescendantIterator.cs
- AsyncOperation.cs
- SmtpDateTime.cs
- PermissionRequestEvidence.cs
- X509Certificate2Collection.cs
- KeyFrames.cs
- ISAPIWorkerRequest.cs
- ItemDragEvent.cs
- WeakEventTable.cs
- TargetConverter.cs
- MobileComponentEditorPage.cs
- FloatUtil.cs
- GPRECT.cs
- UrlParameterWriter.cs
- EntityModelSchemaGenerator.cs
- DesignTimeTemplateParser.cs
- MobileControlBuilder.cs
- DeclaredTypeElementCollection.cs
- DataServiceProviderWrapper.cs
- LogStore.cs
- GroupStyle.cs
- EntityDataSourceDesigner.cs
- LogEntryHeaderDeserializer.cs
- EncryptedKey.cs
- CodeValidator.cs
- BaseCodePageEncoding.cs
- VariantWrapper.cs
- BaseDataBoundControl.cs
- XmlSchemaComplexContent.cs
- HwndMouseInputProvider.cs
- ColumnMapProcessor.cs
- DownloadProgressEventArgs.cs
- TrustLevelCollection.cs
- ColumnClickEvent.cs
- OverrideMode.cs
- WindowsTooltip.cs
- PromptBuilder.cs
- CollectionBuilder.cs
- GCHandleCookieTable.cs
- TableProviderWrapper.cs
- _AutoWebProxyScriptHelper.cs
- WebConfigurationHost.cs
- CodeSubDirectory.cs
- TextTrailingCharacterEllipsis.cs