Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / MIT / System / Web / UI / MobileControls / MobileControlBuilder.cs / 1305376 / MobileControlBuilder.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Diagnostics; using System.Drawing; using System.Globalization; using System.Web; using System.Web.UI; using System.Web.UI.Design.WebControls; using System.Web.UI.WebControls; using System.Security.Permissions; namespace System.Web.UI.MobileControls { /* * Control builder for mobile controls. * * Copyright (c) 2000 Microsoft Corporation */ ///[AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] [Obsolete("The System.Web.Mobile.dll assembly has been deprecated and should no longer be used. For information about how to develop ASP.NET mobile applications, see http://go.microsoft.com/fwlink/?LinkId=157231.")] public class MobileControlBuilder : ControlBuilder { /// public override bool AllowWhitespaceLiterals() { return false; } /// public override Type GetChildControlType(String tagName, IDictionary attributes) { Type type; if (String.Compare(tagName, typeof(DeviceSpecific).Name, StringComparison.OrdinalIgnoreCase) == 0) { type = typeof(DeviceSpecific); } else { type = base.GetChildControlType(tagName, attributes); //if (type == null) //{ // type = Parser.RootBuilder.GetChildControlType(tagName, attributes); //} } // enforce valid control nesting behaviour if (typeof(Form).IsAssignableFrom(type)) { throw new Exception( SR.GetString(SR.MobileControlBuilder_ControlMustBeTopLevelOfPage, "Form")); } if (typeof(StyleSheet).IsAssignableFrom(type)) { throw new Exception( SR.GetString(SR.MobileControlBuilder_ControlMustBeTopLevelOfPage, "StyleSheet")); } if (typeof(Style).IsAssignableFrom(type) && !typeof(StyleSheet).IsAssignableFrom(ControlType)) { throw new Exception( SR.GetString(SR.MobileControlBuilder_StyleMustBeInStyleSheet)); } return type; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Diagnostics; using System.Drawing; using System.Globalization; using System.Web; using System.Web.UI; using System.Web.UI.Design.WebControls; using System.Web.UI.WebControls; using System.Security.Permissions; namespace System.Web.UI.MobileControls { /* * Control builder for mobile controls. * * Copyright (c) 2000 Microsoft Corporation */ ///[AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] [Obsolete("The System.Web.Mobile.dll assembly has been deprecated and should no longer be used. For information about how to develop ASP.NET mobile applications, see http://go.microsoft.com/fwlink/?LinkId=157231.")] public class MobileControlBuilder : ControlBuilder { /// public override bool AllowWhitespaceLiterals() { return false; } /// public override Type GetChildControlType(String tagName, IDictionary attributes) { Type type; if (String.Compare(tagName, typeof(DeviceSpecific).Name, StringComparison.OrdinalIgnoreCase) == 0) { type = typeof(DeviceSpecific); } else { type = base.GetChildControlType(tagName, attributes); //if (type == null) //{ // type = Parser.RootBuilder.GetChildControlType(tagName, attributes); //} } // enforce valid control nesting behaviour if (typeof(Form).IsAssignableFrom(type)) { throw new Exception( SR.GetString(SR.MobileControlBuilder_ControlMustBeTopLevelOfPage, "Form")); } if (typeof(StyleSheet).IsAssignableFrom(type)) { throw new Exception( SR.GetString(SR.MobileControlBuilder_ControlMustBeTopLevelOfPage, "StyleSheet")); } if (typeof(Style).IsAssignableFrom(type) && !typeof(StyleSheet).IsAssignableFrom(ControlType)) { throw new Exception( SR.GetString(SR.MobileControlBuilder_StyleMustBeInStyleSheet)); } return type; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
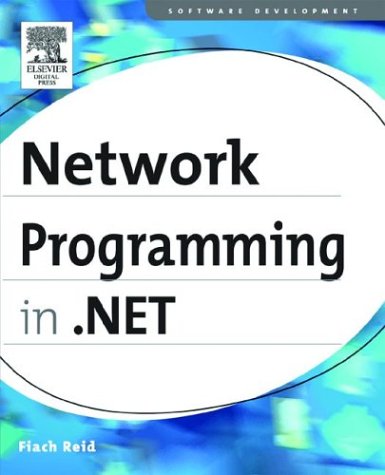
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StringConcat.cs
- UInt32Converter.cs
- IntegerFacetDescriptionElement.cs
- ToolStripDesigner.cs
- DispatchWrapper.cs
- TextElementEditingBehaviorAttribute.cs
- TypeRefElement.cs
- PersistChildrenAttribute.cs
- RSAOAEPKeyExchangeDeformatter.cs
- FamilyMapCollection.cs
- EditorZoneAutoFormat.cs
- RewritingPass.cs
- TraceListeners.cs
- SqlInternalConnectionSmi.cs
- ACL.cs
- MultiBindingExpression.cs
- FileRegion.cs
- COM2IProvidePropertyBuilderHandler.cs
- DataListItem.cs
- ColorBlend.cs
- DbMetaDataCollectionNames.cs
- IListConverters.cs
- DetailsViewInsertEventArgs.cs
- InfiniteIntConverter.cs
- SingleResultAttribute.cs
- UserControlDocumentDesigner.cs
- PackUriHelper.cs
- OrthographicCamera.cs
- HttpTransportBindingElement.cs
- RSAOAEPKeyExchangeDeformatter.cs
- DataGridViewCellStyleBuilderDialog.cs
- PriorityItem.cs
- DetailsViewInsertedEventArgs.cs
- ServiceXNameTypeConverter.cs
- LocalizableAttribute.cs
- cookieexception.cs
- TypeValidationEventArgs.cs
- XmlSchemaAnnotation.cs
- BuildProvidersCompiler.cs
- ObjectSet.cs
- sapiproxy.cs
- DisplayInformation.cs
- PointCollection.cs
- BulletedList.cs
- XmlNamespaceDeclarationsAttribute.cs
- GeneralTransform3DGroup.cs
- JapaneseCalendar.cs
- InsufficientExecutionStackException.cs
- SecondaryViewProvider.cs
- RepeaterItemCollection.cs
- FixedNode.cs
- AtomContentProperty.cs
- Geometry.cs
- FocusChangedEventArgs.cs
- ImageBrush.cs
- ExtensionMethods.cs
- MemberHolder.cs
- PropertySegmentSerializer.cs
- Parameter.cs
- InputProviderSite.cs
- WSSecureConversation.cs
- CaseStatement.cs
- XmlWrappingWriter.cs
- SpAudioStreamWrapper.cs
- ContextMenu.cs
- DataKey.cs
- ConfigurationErrorsException.cs
- MapPathBasedVirtualPathProvider.cs
- Baml2006ReaderFrame.cs
- XmlSchemaAll.cs
- HtmlMeta.cs
- RenderContext.cs
- XslVisitor.cs
- FormViewDeleteEventArgs.cs
- keycontainerpermission.cs
- CaretElement.cs
- StaticSiteMapProvider.cs
- MimeTypePropertyAttribute.cs
- DataGridViewHeaderCell.cs
- DummyDataSource.cs
- CodeSnippetStatement.cs
- XmlDictionaryReaderQuotas.cs
- CollectionChangeEventArgs.cs
- StyleSelector.cs
- HMACMD5.cs
- CommentAction.cs
- ListViewGroup.cs
- GroupItemAutomationPeer.cs
- TextRangeSerialization.cs
- FormsIdentity.cs
- FontFaceLayoutInfo.cs
- AudioException.cs
- XmlIgnoreAttribute.cs
- DbConnectionStringBuilder.cs
- DataGridViewSelectedCellCollection.cs
- DataTableNewRowEvent.cs
- BaseParser.cs
- ScaleTransform.cs
- X509CertificateCollection.cs
- KeyEventArgs.cs