Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataWeb / Client / System / Data / Services / Client / ALinq / UriWriter.cs / 3 / UriWriter.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Translates resource bound expression trees into URIs. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Client { using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Text; using System.Linq; using System.Linq.Expressions; using System.Xml; ////// Translates resource bound expression trees into URIs. /// internal class UriWriter : DataServiceExpressionVisitor { ///Data context used to generate type names for types. private readonly DataServiceContext context; ///stringbuilder for constructed URI private readonly StringBuilder uriBuilder; ////// Private constructor for creating UriWriter /// /// Data context used to generate type names for types. private UriWriter(DataServiceContext context) { Debug.Assert(context != null, "context != null"); this.context = context; this.uriBuilder = new StringBuilder(); } ////// Translates resource bound expression tree to a URI. /// /// Data context used to generate type names for types. /// The expression to translate ///Uri as a string internal static string Translate(DataServiceContext context, Expression e) { var writer = new UriWriter(context); writer.Visit(e); return writer.uriBuilder.ToString(); } ////// MethodCallExpression visit method /// /// The MethodCallExpression expression to visit ///The visited MethodCallExpression expression internal override Expression VisitMethodCall(MethodCallExpression m) { throw Error.MethodNotSupported(m); } ////// UnaryExpression visit method /// /// The UnaryExpression expression to visit ///The visited UnaryExpression expression internal override Expression VisitUnary(UnaryExpression u) { throw new NotSupportedException(Strings.ALinq_UnaryNotSupported(u.NodeType.ToString())); } ////// BinaryExpression visit method /// /// The BinaryExpression expression to visit ///The visited BinaryExpression expression internal override Expression VisitBinary(BinaryExpression b) { throw new NotSupportedException(Strings.ALinq_BinaryNotSupported(b.NodeType.ToString())); } ////// ConstantExpression visit method /// /// The ConstantExpression expression to visit ///The visited ConstantExpression expression internal override Expression VisitConstant(ConstantExpression c) { throw new NotSupportedException(Strings.ALinq_ConstantNotSupported(c.Value)); } ////// TypeBinaryExpression visit method /// /// The TypeBinaryExpression expression to visit ///The visited TypeBinaryExpression expression internal override Expression VisitTypeIs(TypeBinaryExpression b) { throw new NotSupportedException(Strings.ALinq_TypeBinaryNotSupported); } ////// ConditionalExpression visit method /// /// The ConditionalExpression expression to visit ///The visited ConditionalExpression expression internal override Expression VisitConditional(ConditionalExpression c) { throw new NotSupportedException(Strings.ALinq_ConditionalNotSupported); } ////// ParameterExpression visit method /// /// The ParameterExpression expression to visit ///The visited ParameterExpression expression internal override Expression VisitParameter(ParameterExpression p) { throw new NotSupportedException(Strings.ALinq_ParameterNotSupported); } ////// MemberExpression visit method /// /// The MemberExpression expression to visit ///The visited MemberExpression expression internal override Expression VisitMemberAccess(MemberExpression m) { throw new NotSupportedException(Strings.ALinq_MemberAccessNotSupported(m.Member.Name)); } ////// LambdaExpression visit method /// /// The LambdaExpression to visit ///The visited LambdaExpression internal override Expression VisitLambda(LambdaExpression lambda) { throw new NotSupportedException(Strings.ALinq_LambdaNotSupported); } ////// NewExpression visit method /// /// The NewExpression to visit ///The visited NewExpression internal override NewExpression VisitNew(NewExpression nex) { throw new NotSupportedException(Strings.ALinq_NewNotSupported); } ////// MemberInitExpression visit method /// /// The MemberInitExpression to visit ///The visited MemberInitExpression internal override Expression VisitMemberInit(MemberInitExpression init) { throw new NotSupportedException(Strings.ALinq_MemberInitNotSupported); } ////// ListInitExpression visit method /// /// The ListInitExpression to visit ///The visited ListInitExpression internal override Expression VisitListInit(ListInitExpression init) { throw new NotSupportedException(Strings.ALinq_ListInitNotSupported); } ////// NewArrayExpression visit method /// /// The NewArrayExpression to visit ///The visited NewArrayExpression internal override Expression VisitNewArray(NewArrayExpression na) { throw new NotSupportedException(Strings.ALinq_NewArrayNotSupported); } ////// InvocationExpression visit method /// /// The InvocationExpression to visit ///The visited InvocationExpression internal override Expression VisitInvocation(InvocationExpression iv) { throw new NotSupportedException(Strings.ALinq_InvocationNotSupported); } ////// NavigationPropertySingletonExpression visit method. /// /// NavigationPropertySingletonExpression expression to visit ///Visited NavigationPropertySingletonExpression expression internal override Expression VisitNavigationPropertySingletonExpression(NavigationPropertySingletonExpression npse) { this.Visit(npse.Source); this.uriBuilder.Append(UriHelper.FORWARDSLASH).Append(this.ExpressionToString(npse.MemberExpression)); this.VisitQueryOptions(npse); return npse; } ////// ResourceSetExpression visit method. /// /// ResourceSetExpression expression to visit ///Visited ResourceSetExpression expression internal override Expression VisitResourceSetExpression(ResourceSetExpression rse) { if ((ResourceExpressionType)rse.NodeType == ResourceExpressionType.ResourceNavigationProperty) { this.Visit(rse.Source); this.uriBuilder.Append(UriHelper.FORWARDSLASH).Append(this.ExpressionToString(rse.MemberExpression)); } else { this.uriBuilder.Append(UriHelper.FORWARDSLASH).Append((string)((ConstantExpression)rse.MemberExpression).Value); } if (rse.KeyPredicate != null) { this.uriBuilder.Append(UriHelper.LEFTPAREN); if (rse.KeyPredicate.Count == 1) { this.uriBuilder.Append(this.ExpressionToString(rse.KeyPredicate.Values.First())); } else { bool addComma = false; foreach (var kvp in rse.KeyPredicate) { if (addComma) { this.uriBuilder.Append(UriHelper.COMMA); } this.uriBuilder.Append(kvp.Key.Name); this.uriBuilder.Append(UriHelper.EQUALSSIGN); this.uriBuilder.Append(this.ExpressionToString(kvp.Value)); addComma = true; } } this.uriBuilder.Append(UriHelper.RIGHTPAREN); } else if (rse.IsLeafResource) { // if resourceset is on the leaf, append () this.uriBuilder.Append(UriHelper.LEFTPAREN); this.uriBuilder.Append(UriHelper.RIGHTPAREN); } this.VisitQueryOptions(rse); return rse; } ////// Visit Query options for Resource /// /// Resource Expression with query options internal void VisitQueryOptions(ResourceExpression re) { bool needAmpersand = false; if (re.HasQueryOptions) { this.uriBuilder.Append(UriHelper.QUESTIONMARK); ResourceSetExpression rse = re as ResourceSetExpression; if (rse != null && rse.HasSequenceQueryOptions) { needAmpersand = true; IEnumerator options = rse.SequenceQueryOptions.GetEnumerator(); options.MoveNext(); while (true) { Expression e = ((Expression)options.Current); ResourceExpressionType et = (ResourceExpressionType)e.NodeType; switch (et) { case ResourceExpressionType.SkipQueryOption: this.VisitQueryOptionExpression((SkipQueryOptionExpression)e); break; case ResourceExpressionType.TakeQueryOption: this.VisitQueryOptionExpression((TakeQueryOptionExpression)e); break; case ResourceExpressionType.OrderByQueryOption: this.VisitQueryOptionExpression((OrderByQueryOptionExpression)e); break; case ResourceExpressionType.FilterQueryOption: this.VisitQueryOptionExpression((FilterQueryOptionExpression)e); break; default: Debug.Assert(false, "Unexpected expression type " + (int)et); break; } if (!options.MoveNext()) { break; } this.uriBuilder.Append(UriHelper.AMPERSAND); } } if (re.ExpandPaths.Count > 0) { if (needAmpersand) { this.uriBuilder.Append(UriHelper.AMPERSAND); } this.VisitExpandOptions(re.ExpandPaths); needAmpersand = true; } if (re.CustomQueryOptions.Count > 0) { if (needAmpersand) { this.uriBuilder.Append(UriHelper.AMPERSAND); } this.VisitCustomQueryOptions(re.CustomQueryOptions); needAmpersand = true; } } } ////// SkipQueryOptionExpression visit method. /// /// SkipQueryOptionExpression expression to visit internal void VisitQueryOptionExpression(SkipQueryOptionExpression sqoe) { this.uriBuilder.Append(UriHelper.DOLLARSIGN); this.uriBuilder.Append(UriHelper.OPTIONSKIP); this.uriBuilder.Append(UriHelper.EQUALSSIGN); this.uriBuilder.Append(this.ExpressionToString(sqoe.SkipAmount)); } ////// TakeQueryOptionExpression visit method. /// /// TakeQueryOptionExpression expression to visit internal void VisitQueryOptionExpression(TakeQueryOptionExpression tqoe) { this.uriBuilder.Append(UriHelper.DOLLARSIGN); this.uriBuilder.Append(UriHelper.OPTIONTOP); this.uriBuilder.Append(UriHelper.EQUALSSIGN); this.uriBuilder.Append(this.ExpressionToString(tqoe.TakeAmount)); } ////// FilterQueryOptionExpression visit method. /// /// FilterQueryOptionExpression expression to visit internal void VisitQueryOptionExpression(FilterQueryOptionExpression fqoe) { this.uriBuilder.Append(UriHelper.DOLLARSIGN); this.uriBuilder.Append(UriHelper.OPTIONFILTER); this.uriBuilder.Append(UriHelper.EQUALSSIGN); this.uriBuilder.Append(this.ExpressionToString(fqoe.Predicate)); } ////// OrderByQueryOptionExpression visit method. /// /// OrderByQueryOptionExpression expression to visit internal void VisitQueryOptionExpression(OrderByQueryOptionExpression oboe) { this.uriBuilder.Append(UriHelper.DOLLARSIGN); this.uriBuilder.Append(UriHelper.OPTIONORDERBY); this.uriBuilder.Append(UriHelper.EQUALSSIGN); int ii = 0; while (true) { var selector = oboe.Selectors[ii]; this.uriBuilder.Append(this.ExpressionToString(selector.Expression)); if (selector.Descending) { this.uriBuilder.Append(UriHelper.SPACE); this.uriBuilder.Append(UriHelper.OPTIONDESC); } if (++ii == oboe.Selectors.Count) { break; } this.uriBuilder.Append(UriHelper.COMMA); } } ////// VisitExpandOptions visit method. /// /// Expand Paths internal void VisitExpandOptions(Listpaths) { this.uriBuilder.Append(UriHelper.DOLLARSIGN); this.uriBuilder.Append(UriHelper.OPTIONEXPAND); this.uriBuilder.Append(UriHelper.EQUALSSIGN); int ii = 0; while (true) { ConstantExpression path = paths[ii]; this.uriBuilder.Append(path.Value); if (++ii == paths.Count) { break; } this.uriBuilder.Append(UriHelper.COMMA); } } /// /// VisitCustomQueryOptions visit method. /// /// Custom query options internal void VisitCustomQueryOptions(Dictionaryoptions) { List keys = options.Keys.ToList(); List values = options.Values.ToList(); int ii = 0; while (true) { this.uriBuilder.Append(keys[ii].Value); this.uriBuilder.Append(UriHelper.EQUALSSIGN); this.uriBuilder.Append(values[ii].Value); if (++ii == keys.Count) { break; } this.uriBuilder.Append(UriHelper.AMPERSAND); } } /// Serializes an expression to a string. /// Expression to serialize ///The serialized expression. private string ExpressionToString(Expression expression) { return ExpressionWriter.ExpressionToString(this.context, expression); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Translates resource bound expression trees into URIs. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Client { using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Text; using System.Linq; using System.Linq.Expressions; using System.Xml; ////// Translates resource bound expression trees into URIs. /// internal class UriWriter : DataServiceExpressionVisitor { ///Data context used to generate type names for types. private readonly DataServiceContext context; ///stringbuilder for constructed URI private readonly StringBuilder uriBuilder; ////// Private constructor for creating UriWriter /// /// Data context used to generate type names for types. private UriWriter(DataServiceContext context) { Debug.Assert(context != null, "context != null"); this.context = context; this.uriBuilder = new StringBuilder(); } ////// Translates resource bound expression tree to a URI. /// /// Data context used to generate type names for types. /// The expression to translate ///Uri as a string internal static string Translate(DataServiceContext context, Expression e) { var writer = new UriWriter(context); writer.Visit(e); return writer.uriBuilder.ToString(); } ////// MethodCallExpression visit method /// /// The MethodCallExpression expression to visit ///The visited MethodCallExpression expression internal override Expression VisitMethodCall(MethodCallExpression m) { throw Error.MethodNotSupported(m); } ////// UnaryExpression visit method /// /// The UnaryExpression expression to visit ///The visited UnaryExpression expression internal override Expression VisitUnary(UnaryExpression u) { throw new NotSupportedException(Strings.ALinq_UnaryNotSupported(u.NodeType.ToString())); } ////// BinaryExpression visit method /// /// The BinaryExpression expression to visit ///The visited BinaryExpression expression internal override Expression VisitBinary(BinaryExpression b) { throw new NotSupportedException(Strings.ALinq_BinaryNotSupported(b.NodeType.ToString())); } ////// ConstantExpression visit method /// /// The ConstantExpression expression to visit ///The visited ConstantExpression expression internal override Expression VisitConstant(ConstantExpression c) { throw new NotSupportedException(Strings.ALinq_ConstantNotSupported(c.Value)); } ////// TypeBinaryExpression visit method /// /// The TypeBinaryExpression expression to visit ///The visited TypeBinaryExpression expression internal override Expression VisitTypeIs(TypeBinaryExpression b) { throw new NotSupportedException(Strings.ALinq_TypeBinaryNotSupported); } ////// ConditionalExpression visit method /// /// The ConditionalExpression expression to visit ///The visited ConditionalExpression expression internal override Expression VisitConditional(ConditionalExpression c) { throw new NotSupportedException(Strings.ALinq_ConditionalNotSupported); } ////// ParameterExpression visit method /// /// The ParameterExpression expression to visit ///The visited ParameterExpression expression internal override Expression VisitParameter(ParameterExpression p) { throw new NotSupportedException(Strings.ALinq_ParameterNotSupported); } ////// MemberExpression visit method /// /// The MemberExpression expression to visit ///The visited MemberExpression expression internal override Expression VisitMemberAccess(MemberExpression m) { throw new NotSupportedException(Strings.ALinq_MemberAccessNotSupported(m.Member.Name)); } ////// LambdaExpression visit method /// /// The LambdaExpression to visit ///The visited LambdaExpression internal override Expression VisitLambda(LambdaExpression lambda) { throw new NotSupportedException(Strings.ALinq_LambdaNotSupported); } ////// NewExpression visit method /// /// The NewExpression to visit ///The visited NewExpression internal override NewExpression VisitNew(NewExpression nex) { throw new NotSupportedException(Strings.ALinq_NewNotSupported); } ////// MemberInitExpression visit method /// /// The MemberInitExpression to visit ///The visited MemberInitExpression internal override Expression VisitMemberInit(MemberInitExpression init) { throw new NotSupportedException(Strings.ALinq_MemberInitNotSupported); } ////// ListInitExpression visit method /// /// The ListInitExpression to visit ///The visited ListInitExpression internal override Expression VisitListInit(ListInitExpression init) { throw new NotSupportedException(Strings.ALinq_ListInitNotSupported); } ////// NewArrayExpression visit method /// /// The NewArrayExpression to visit ///The visited NewArrayExpression internal override Expression VisitNewArray(NewArrayExpression na) { throw new NotSupportedException(Strings.ALinq_NewArrayNotSupported); } ////// InvocationExpression visit method /// /// The InvocationExpression to visit ///The visited InvocationExpression internal override Expression VisitInvocation(InvocationExpression iv) { throw new NotSupportedException(Strings.ALinq_InvocationNotSupported); } ////// NavigationPropertySingletonExpression visit method. /// /// NavigationPropertySingletonExpression expression to visit ///Visited NavigationPropertySingletonExpression expression internal override Expression VisitNavigationPropertySingletonExpression(NavigationPropertySingletonExpression npse) { this.Visit(npse.Source); this.uriBuilder.Append(UriHelper.FORWARDSLASH).Append(this.ExpressionToString(npse.MemberExpression)); this.VisitQueryOptions(npse); return npse; } ////// ResourceSetExpression visit method. /// /// ResourceSetExpression expression to visit ///Visited ResourceSetExpression expression internal override Expression VisitResourceSetExpression(ResourceSetExpression rse) { if ((ResourceExpressionType)rse.NodeType == ResourceExpressionType.ResourceNavigationProperty) { this.Visit(rse.Source); this.uriBuilder.Append(UriHelper.FORWARDSLASH).Append(this.ExpressionToString(rse.MemberExpression)); } else { this.uriBuilder.Append(UriHelper.FORWARDSLASH).Append((string)((ConstantExpression)rse.MemberExpression).Value); } if (rse.KeyPredicate != null) { this.uriBuilder.Append(UriHelper.LEFTPAREN); if (rse.KeyPredicate.Count == 1) { this.uriBuilder.Append(this.ExpressionToString(rse.KeyPredicate.Values.First())); } else { bool addComma = false; foreach (var kvp in rse.KeyPredicate) { if (addComma) { this.uriBuilder.Append(UriHelper.COMMA); } this.uriBuilder.Append(kvp.Key.Name); this.uriBuilder.Append(UriHelper.EQUALSSIGN); this.uriBuilder.Append(this.ExpressionToString(kvp.Value)); addComma = true; } } this.uriBuilder.Append(UriHelper.RIGHTPAREN); } else if (rse.IsLeafResource) { // if resourceset is on the leaf, append () this.uriBuilder.Append(UriHelper.LEFTPAREN); this.uriBuilder.Append(UriHelper.RIGHTPAREN); } this.VisitQueryOptions(rse); return rse; } ////// Visit Query options for Resource /// /// Resource Expression with query options internal void VisitQueryOptions(ResourceExpression re) { bool needAmpersand = false; if (re.HasQueryOptions) { this.uriBuilder.Append(UriHelper.QUESTIONMARK); ResourceSetExpression rse = re as ResourceSetExpression; if (rse != null && rse.HasSequenceQueryOptions) { needAmpersand = true; IEnumerator options = rse.SequenceQueryOptions.GetEnumerator(); options.MoveNext(); while (true) { Expression e = ((Expression)options.Current); ResourceExpressionType et = (ResourceExpressionType)e.NodeType; switch (et) { case ResourceExpressionType.SkipQueryOption: this.VisitQueryOptionExpression((SkipQueryOptionExpression)e); break; case ResourceExpressionType.TakeQueryOption: this.VisitQueryOptionExpression((TakeQueryOptionExpression)e); break; case ResourceExpressionType.OrderByQueryOption: this.VisitQueryOptionExpression((OrderByQueryOptionExpression)e); break; case ResourceExpressionType.FilterQueryOption: this.VisitQueryOptionExpression((FilterQueryOptionExpression)e); break; default: Debug.Assert(false, "Unexpected expression type " + (int)et); break; } if (!options.MoveNext()) { break; } this.uriBuilder.Append(UriHelper.AMPERSAND); } } if (re.ExpandPaths.Count > 0) { if (needAmpersand) { this.uriBuilder.Append(UriHelper.AMPERSAND); } this.VisitExpandOptions(re.ExpandPaths); needAmpersand = true; } if (re.CustomQueryOptions.Count > 0) { if (needAmpersand) { this.uriBuilder.Append(UriHelper.AMPERSAND); } this.VisitCustomQueryOptions(re.CustomQueryOptions); needAmpersand = true; } } } ////// SkipQueryOptionExpression visit method. /// /// SkipQueryOptionExpression expression to visit internal void VisitQueryOptionExpression(SkipQueryOptionExpression sqoe) { this.uriBuilder.Append(UriHelper.DOLLARSIGN); this.uriBuilder.Append(UriHelper.OPTIONSKIP); this.uriBuilder.Append(UriHelper.EQUALSSIGN); this.uriBuilder.Append(this.ExpressionToString(sqoe.SkipAmount)); } ////// TakeQueryOptionExpression visit method. /// /// TakeQueryOptionExpression expression to visit internal void VisitQueryOptionExpression(TakeQueryOptionExpression tqoe) { this.uriBuilder.Append(UriHelper.DOLLARSIGN); this.uriBuilder.Append(UriHelper.OPTIONTOP); this.uriBuilder.Append(UriHelper.EQUALSSIGN); this.uriBuilder.Append(this.ExpressionToString(tqoe.TakeAmount)); } ////// FilterQueryOptionExpression visit method. /// /// FilterQueryOptionExpression expression to visit internal void VisitQueryOptionExpression(FilterQueryOptionExpression fqoe) { this.uriBuilder.Append(UriHelper.DOLLARSIGN); this.uriBuilder.Append(UriHelper.OPTIONFILTER); this.uriBuilder.Append(UriHelper.EQUALSSIGN); this.uriBuilder.Append(this.ExpressionToString(fqoe.Predicate)); } ////// OrderByQueryOptionExpression visit method. /// /// OrderByQueryOptionExpression expression to visit internal void VisitQueryOptionExpression(OrderByQueryOptionExpression oboe) { this.uriBuilder.Append(UriHelper.DOLLARSIGN); this.uriBuilder.Append(UriHelper.OPTIONORDERBY); this.uriBuilder.Append(UriHelper.EQUALSSIGN); int ii = 0; while (true) { var selector = oboe.Selectors[ii]; this.uriBuilder.Append(this.ExpressionToString(selector.Expression)); if (selector.Descending) { this.uriBuilder.Append(UriHelper.SPACE); this.uriBuilder.Append(UriHelper.OPTIONDESC); } if (++ii == oboe.Selectors.Count) { break; } this.uriBuilder.Append(UriHelper.COMMA); } } ////// VisitExpandOptions visit method. /// /// Expand Paths internal void VisitExpandOptions(Listpaths) { this.uriBuilder.Append(UriHelper.DOLLARSIGN); this.uriBuilder.Append(UriHelper.OPTIONEXPAND); this.uriBuilder.Append(UriHelper.EQUALSSIGN); int ii = 0; while (true) { ConstantExpression path = paths[ii]; this.uriBuilder.Append(path.Value); if (++ii == paths.Count) { break; } this.uriBuilder.Append(UriHelper.COMMA); } } /// /// VisitCustomQueryOptions visit method. /// /// Custom query options internal void VisitCustomQueryOptions(Dictionaryoptions) { List keys = options.Keys.ToList(); List values = options.Values.ToList(); int ii = 0; while (true) { this.uriBuilder.Append(keys[ii].Value); this.uriBuilder.Append(UriHelper.EQUALSSIGN); this.uriBuilder.Append(values[ii].Value); if (++ii == keys.Count) { break; } this.uriBuilder.Append(UriHelper.AMPERSAND); } } /// Serializes an expression to a string. /// Expression to serialize ///The serialized expression. private string ExpressionToString(Expression expression) { return ExpressionWriter.ExpressionToString(this.context, expression); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
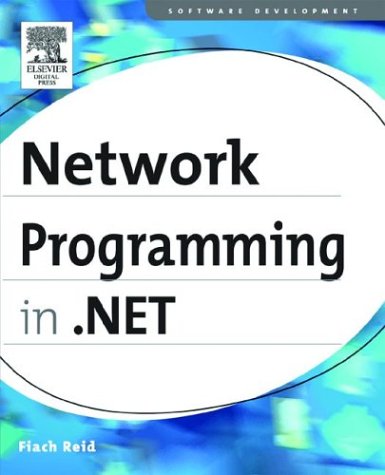
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AdapterDictionary.cs
- DisposableCollectionWrapper.cs
- RectValueSerializer.cs
- Model3D.cs
- SystemTcpStatistics.cs
- XdrBuilder.cs
- HMAC.cs
- RegexBoyerMoore.cs
- EntitySqlQueryCacheEntry.cs
- StoragePropertyMapping.cs
- DrawingDrawingContext.cs
- NotifyIcon.cs
- CommonObjectSecurity.cs
- RowToFieldTransformer.cs
- DataGridViewCheckBoxCell.cs
- ShaderRenderModeValidation.cs
- ScaleTransform.cs
- QueryGeneratorBase.cs
- AssociatedControlConverter.cs
- PrintControllerWithStatusDialog.cs
- ExclusiveTcpTransportManager.cs
- PropertyItemInternal.cs
- LinqMaximalSubtreeNominator.cs
- SimpleHandlerFactory.cs
- TextProperties.cs
- BoolExpr.cs
- AsyncStreamReader.cs
- TraceSource.cs
- ConsoleCancelEventArgs.cs
- DataGridViewRowEventArgs.cs
- RemotingConfiguration.cs
- WindowInteractionStateTracker.cs
- NegotiateStream.cs
- AlignmentYValidation.cs
- Padding.cs
- SuppressIldasmAttribute.cs
- PropertyIDSet.cs
- SymbolTable.cs
- NameValueSectionHandler.cs
- IndexerNameAttribute.cs
- CustomGrammar.cs
- Visitor.cs
- WebPartTracker.cs
- InvalidateEvent.cs
- ToolStripContentPanelRenderEventArgs.cs
- ColorMap.cs
- Item.cs
- ThreadAbortException.cs
- BitmapSource.cs
- RectAnimation.cs
- UniqueConstraint.cs
- ManifestSignedXml.cs
- ListMarkerLine.cs
- InkPresenter.cs
- OperationContractGenerationContext.cs
- Authorization.cs
- FormViewUpdateEventArgs.cs
- RelatedPropertyManager.cs
- BrowserDefinitionCollection.cs
- ComponentRenameEvent.cs
- DataServiceClientException.cs
- Vector3DCollection.cs
- ListControlDesigner.cs
- FixedFindEngine.cs
- HotSpot.cs
- IncrementalReadDecoders.cs
- XmlLoader.cs
- RichTextBoxAutomationPeer.cs
- ListViewGroupConverter.cs
- OptionUsage.cs
- SafeCancelMibChangeNotify.cs
- Html32TextWriter.cs
- UserControlCodeDomTreeGenerator.cs
- StringKeyFrameCollection.cs
- EntityViewGenerationConstants.cs
- AppDomainProtocolHandler.cs
- CodeMemberProperty.cs
- RootProfilePropertySettingsCollection.cs
- CustomBindingElementCollection.cs
- DesignerCategoryAttribute.cs
- NamedElement.cs
- HwndProxyElementProvider.cs
- CanonicalizationDriver.cs
- Helper.cs
- CalendarButtonAutomationPeer.cs
- BlockCollection.cs
- ThemeableAttribute.cs
- Parser.cs
- RenderTargetBitmap.cs
- GenerateScriptTypeAttribute.cs
- LabelLiteral.cs
- BamlTreeUpdater.cs
- AutomationTextAttribute.cs
- StorageAssociationTypeMapping.cs
- AudioException.cs
- HttpListenerPrefixCollection.cs
- ItemCollection.cs
- DynamicQueryableWrapper.cs
- FileDataSourceCache.cs
- WebPartConnectionCollection.cs