Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / Tools / System.Activities.Presentation / System / Activities / Presentation / Base / Core / PropertyEditing / newitemfactory.cs / 1305376 / newitemfactory.cs
namespace System.Activities.Presentation.PropertyEditing { using System; using System.Windows; using System.Reflection; using System.IO; using System.Windows.Markup; using System.Windows.Media.Imaging; using System.Windows.Controls; using System.Activities.Presentation.Internal; using System.Diagnostics.CodeAnalysis; using System.Activities.Presentation; ////// Base class that represents a factory for creating new items for a collection or /// for a property value. 3rd party control developers may choose to derive from this class /// to override the default behavior of specifying names and images that are used by /// collection editor and sub-property editor when creating instances of custom controls. /// class NewItemFactory { private Type[] NoTypes = new Type[0]; ////// Default constructor /// public NewItemFactory() { } ////// Returns an object that can be set as the Content of a ContentControl /// and that will be used an icon for the requested type by the property editing host. /// The default implementation of this method uses naming convention, searching for /// the embedded resources in the same assembly as the control, that are named the /// same as the control (including namespace), followed by ".Icon", followed by /// the extension for the file type itself. Currently, only ".png", ".bmp", ".gif", /// ".jpg", and ".jpeg" extensions are recognized. /// /// Type of the object to look up /// The desired size of the image to retrieve. If multiple /// images are available this method retrieves the image that most closely /// resembles the requested size. However, it is not guaranteed to return an image /// that matches the desired size exactly. ///An image for the specified type. ///If type is null public virtual object GetImage(Type type, Size desiredSize) { if (type == null) throw FxTrace.Exception.ArgumentNull("type"); return ManifestImages.GetImage(type, desiredSize); } ////// Returns the name for the item this factory adds for the passed in type. This is /// the name that will be used in the "Add Item" drop down to identify the type being added. /// The default implementation returns the short type name. /// /// Type to retrieve the display name for. ///The display name for the specified type ///If type is null [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters", Justification = "The intended usage in the larger scope of the class is the stronger type")] public virtual string GetDisplayName(Type type) { if (type == null) throw FxTrace.Exception.ArgumentNull("type"); return type.Name; } ////// Returns an instance of an item that is added to the collection for the passed in Type. /// The default implementation looks for public constructors that take no arguments. /// If no such constructors are found, null is returned. /// /// Type of the object to create ///Instance of the specified type, or null if no appropriate constructor was found /// ///If type is null public virtual object CreateInstance(Type type) { if (type == null) throw FxTrace.Exception.ArgumentNull("type"); ConstructorInfo ctor = type.GetConstructor( BindingFlags.Public | BindingFlags.Instance, null, NoTypes, null); return ctor == null ? null : ctor.Invoke(null); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. namespace System.Activities.Presentation.PropertyEditing { using System; using System.Windows; using System.Reflection; using System.IO; using System.Windows.Markup; using System.Windows.Media.Imaging; using System.Windows.Controls; using System.Activities.Presentation.Internal; using System.Diagnostics.CodeAnalysis; using System.Activities.Presentation; ////// Base class that represents a factory for creating new items for a collection or /// for a property value. 3rd party control developers may choose to derive from this class /// to override the default behavior of specifying names and images that are used by /// collection editor and sub-property editor when creating instances of custom controls. /// class NewItemFactory { private Type[] NoTypes = new Type[0]; ////// Default constructor /// public NewItemFactory() { } ////// Returns an object that can be set as the Content of a ContentControl /// and that will be used an icon for the requested type by the property editing host. /// The default implementation of this method uses naming convention, searching for /// the embedded resources in the same assembly as the control, that are named the /// same as the control (including namespace), followed by ".Icon", followed by /// the extension for the file type itself. Currently, only ".png", ".bmp", ".gif", /// ".jpg", and ".jpeg" extensions are recognized. /// /// Type of the object to look up /// The desired size of the image to retrieve. If multiple /// images are available this method retrieves the image that most closely /// resembles the requested size. However, it is not guaranteed to return an image /// that matches the desired size exactly. ///An image for the specified type. ///If type is null public virtual object GetImage(Type type, Size desiredSize) { if (type == null) throw FxTrace.Exception.ArgumentNull("type"); return ManifestImages.GetImage(type, desiredSize); } ////// Returns the name for the item this factory adds for the passed in type. This is /// the name that will be used in the "Add Item" drop down to identify the type being added. /// The default implementation returns the short type name. /// /// Type to retrieve the display name for. ///The display name for the specified type ///If type is null [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters", Justification = "The intended usage in the larger scope of the class is the stronger type")] public virtual string GetDisplayName(Type type) { if (type == null) throw FxTrace.Exception.ArgumentNull("type"); return type.Name; } ////// Returns an instance of an item that is added to the collection for the passed in Type. /// The default implementation looks for public constructors that take no arguments. /// If no such constructors are found, null is returned. /// /// Type of the object to create ///Instance of the specified type, or null if no appropriate constructor was found /// ///If type is null public virtual object CreateInstance(Type type) { if (type == null) throw FxTrace.Exception.ArgumentNull("type"); ConstructorInfo ctor = type.GetConstructor( BindingFlags.Public | BindingFlags.Instance, null, NoTypes, null); return ctor == null ? null : ctor.Invoke(null); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
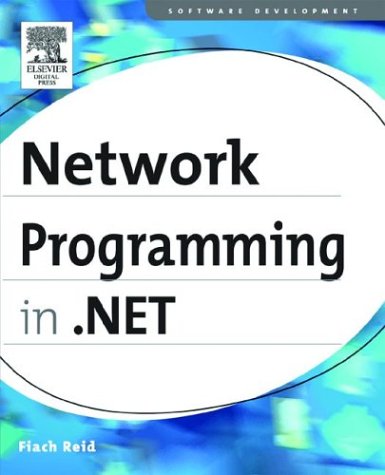
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ServiceOperationWrapper.cs
- ConcurrentStack.cs
- AttributeCollection.cs
- SmiContextFactory.cs
- SmtpCommands.cs
- FontStyleConverter.cs
- TemplateNameScope.cs
- SubqueryTrackingVisitor.cs
- LogWriteRestartAreaState.cs
- SecureEnvironment.cs
- IOException.cs
- ToolStrip.cs
- CleanUpVirtualizedItemEventArgs.cs
- PrintPreviewGraphics.cs
- WSSecurityJan2004.cs
- EntityDataSourceChangingEventArgs.cs
- TextTreeNode.cs
- WebPartPersonalization.cs
- ColorConvertedBitmap.cs
- XslCompiledTransform.cs
- translator.cs
- TcpTransportElement.cs
- ContainerVisual.cs
- Utils.cs
- ColumnMap.cs
- ValueHandle.cs
- SecurityTimestamp.cs
- TypedTableHandler.cs
- RowCache.cs
- CompositionAdorner.cs
- CryptoKeySecurity.cs
- CheckBoxStandardAdapter.cs
- SQLGuidStorage.cs
- DesignerActionItemCollection.cs
- RecognizedAudio.cs
- DeclarativeCatalogPartDesigner.cs
- Trigger.cs
- FillRuleValidation.cs
- HttpCacheVary.cs
- UserNamePasswordValidator.cs
- ConfigurationLockCollection.cs
- DLinqTableProvider.cs
- XamlReaderConstants.cs
- EnumerableRowCollectionExtensions.cs
- DeobfuscatingStream.cs
- FileNotFoundException.cs
- BinaryObjectReader.cs
- ToolBar.cs
- SHA1Managed.cs
- PointAnimation.cs
- FontResourceCache.cs
- TrackingProfileManager.cs
- RoutedEventHandlerInfo.cs
- Shape.cs
- CommentAction.cs
- SHA512Cng.cs
- SafeRightsManagementSessionHandle.cs
- COM2ExtendedUITypeEditor.cs
- AuthorizationRuleCollection.cs
- TypeInfo.cs
- SingleObjectCollection.cs
- IndentedTextWriter.cs
- TableTextElementCollectionInternal.cs
- NamedPermissionSet.cs
- TextBlock.cs
- BindingMemberInfo.cs
- _LocalDataStore.cs
- CodeStatementCollection.cs
- XmlChoiceIdentifierAttribute.cs
- BufferModeSettings.cs
- MonthCalendarDesigner.cs
- SafeFindHandle.cs
- TypeReference.cs
- DrawingCollection.cs
- BitmapEffectDrawing.cs
- TypeDescriptor.cs
- ConnectionConsumerAttribute.cs
- BuildTopDownAttribute.cs
- InputProviderSite.cs
- SQLBytesStorage.cs
- SqlMethodCallConverter.cs
- Geometry3D.cs
- StylusDevice.cs
- ServiceInfo.cs
- AcceptorSessionSymmetricTransportSecurityProtocol.cs
- XmlSerializerFormatAttribute.cs
- TransactionOptions.cs
- StylusButtonCollection.cs
- TrackBarRenderer.cs
- ComponentResourceKeyConverter.cs
- MarkupCompilePass1.cs
- PathGradientBrush.cs
- Util.cs
- Selector.cs
- XamlBrushSerializer.cs
- CharUnicodeInfo.cs
- DefaultValueTypeConverter.cs
- CookieHandler.cs
- SystemIPv6InterfaceProperties.cs
- COM2IManagedPerPropertyBrowsingHandler.cs