Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Framework / MS / Internal / Data / CollectionViewGroupInternal.cs / 1 / CollectionViewGroupInternal.cs
//---------------------------------------------------------------------------- // //// Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // // // // Description: A CollectionViewGroupInternal, as created by a CollectionView according to a GroupDescription. // // See spec at http://avalon/connecteddata/Specs/Grouping.mht // //--------------------------------------------------------------------------- using System; using System.Collections; // IEnumerator using System.ComponentModel; // PropertyChangedEventArgs, GroupDescription using System.Windows; // DependencyProperty.UnsetValue using System.Windows.Data; // CollectionViewGroup namespace MS.Internal.Data { internal class CollectionViewGroupInternal : CollectionViewGroup { #region Constructors //----------------------------------------------------- // // Constructors // //----------------------------------------------------- internal CollectionViewGroupInternal(object name, CollectionViewGroupInternal parent) : base(name) { _parentGroup = parent; } #endregion Constructors #region Public Properties //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- ////// Is this group at the bottom level (not further subgrouped). /// public override bool IsBottomLevel { get { return (_groupBy == null); } } #endregion Public Properties #region Internal Properties //------------------------------------------------------ // // Internal Properties // //------------------------------------------------------ // how this group divides into subgroups internal GroupDescription GroupBy { get { return _groupBy; } set { bool oldIsBottomLevel = IsBottomLevel; if (_groupBy != null) ((INotifyPropertyChanged)_groupBy).PropertyChanged -= new PropertyChangedEventHandler(OnGroupByChanged); _groupBy = value; if (_groupBy != null) ((INotifyPropertyChanged)_groupBy).PropertyChanged += new PropertyChangedEventHandler(OnGroupByChanged); if (oldIsBottomLevel != IsBottomLevel) { OnPropertyChanged(new PropertyChangedEventArgs("IsBottomLevel")); } } } // the number of items and groups in the subtree under this group internal int FullCount { get { return _fullCount; } set { _fullCount = value; } } // the most recent index where actvity took place internal int LastIndex { get { return _lastIndex; } set { _lastIndex = value; } } // the first item (leaf) added to this group. If this can't be determined, // DependencyProperty.UnsetValue. internal object SeedItem { get { if (ItemCount > 0 && (GroupBy == null || GroupBy.GroupNames.Count == 0)) { // look for first item, child by child for (int k=0, n=Items.Count; k0) { // child is a nonempty subgroup - ask it return subgroup.SeedItem; } // otherwise child is an empty subgroup - go to next child } // we shouldn't get here, but just in case... return DependencyProperty.UnsetValue; } else { // the group is empty, or it has explicit subgroups. // In either case, we cannot determine the first item - // it could have gone into any of the subgroups. return DependencyProperty.UnsetValue; } } } #endregion Properties #region Internal methods //----------------------------------------------------- // // Internal methods // //------------------------------------------------------ internal void Add(object item) { ChangeCounts(item, +1); ProtectedItems.Add(item); } internal int Remove(object item, bool returnLeafIndex) { int index = -1; int localIndex = ProtectedItems.IndexOf(item); if (localIndex >= 0) { if (returnLeafIndex) { index = LeafIndexFromItem(null, localIndex); } ChangeCounts(item, -1); ProtectedItems.RemoveAt(localIndex); } return index; } internal void Clear() { ProtectedItems.Clear(); FullCount = 1; ProtectedItemCount = 0; } // return the index of the given item within the list of leaves governed // by this group internal int LeafIndexOf(object item) { int leaves = 0; // number of leaves we've passed over so far for (int k=0, n=Items.Count; k = groupBy.GroupNames.Count) { parent.Remove(group, false); } } } void OnGroupByChanged(object sender, System.ComponentModel.PropertyChangedEventArgs e) { OnGroupByChanged(); } #endregion Private methods #region Private fields //----------------------------------------------------- // // Private fields // //------------------------------------------------------ GroupDescription _groupBy; CollectionViewGroupInternal _parentGroup; int _fullCount = 1; int _lastIndex; int _version; // for detecting stale enumerators #endregion Private fields #region Private classes //------------------------------------------------------ // // Private classes // //----------------------------------------------------- private class LeafEnumerator : IEnumerator { public LeafEnumerator(CollectionViewGroupInternal group) { _group = group; DoReset(); // don't call virtual Reset in ctor } void IEnumerator.Reset() { DoReset(); } void DoReset() { _version = _group._version; _index = -1; _subEnum = null; } bool IEnumerator.MoveNext() { // check for invalidated enumerator if (_group._version != _version) throw new InvalidOperationException(); // move forward to the next leaf while (_subEnum == null || !_subEnum.MoveNext()) { // done with the current top-level item. Move to the next one. ++ _index; if (_index >= _group.Items.Count) return false; CollectionViewGroupInternal subgroup = _group.Items[_index] as CollectionViewGroupInternal; if (subgroup == null) { // current item is a leaf - it's the new Current _current = _group.Items[_index]; _subEnum = null; return true; } else { // current item is a subgroup - get its enumerator _subEnum = subgroup.GetLeafEnumerator(); } } // the loop terminates only when we have a subgroup enumerator // positioned at the new Current item _current = _subEnum.Current; return true; } object IEnumerator.Current { get { if (_index < 0 || _index >= _group.Items.Count) throw new InvalidOperationException(); return _current; } } CollectionViewGroupInternal _group; // parent group int _version; // parent group's version at ctor int _index; // current index into Items IEnumerator _subEnum; // enumerator over current subgroup object _current; // current item } #endregion Private classes } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // // Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // // // // Description: A CollectionViewGroupInternal, as created by a CollectionView according to a GroupDescription. // // See spec at http://avalon/connecteddata/Specs/Grouping.mht // //--------------------------------------------------------------------------- using System; using System.Collections; // IEnumerator using System.ComponentModel; // PropertyChangedEventArgs, GroupDescription using System.Windows; // DependencyProperty.UnsetValue using System.Windows.Data; // CollectionViewGroup namespace MS.Internal.Data { internal class CollectionViewGroupInternal : CollectionViewGroup { #region Constructors //----------------------------------------------------- // // Constructors // //----------------------------------------------------- internal CollectionViewGroupInternal(object name, CollectionViewGroupInternal parent) : base(name) { _parentGroup = parent; } #endregion Constructors #region Public Properties //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- ////// Is this group at the bottom level (not further subgrouped). /// public override bool IsBottomLevel { get { return (_groupBy == null); } } #endregion Public Properties #region Internal Properties //------------------------------------------------------ // // Internal Properties // //------------------------------------------------------ // how this group divides into subgroups internal GroupDescription GroupBy { get { return _groupBy; } set { bool oldIsBottomLevel = IsBottomLevel; if (_groupBy != null) ((INotifyPropertyChanged)_groupBy).PropertyChanged -= new PropertyChangedEventHandler(OnGroupByChanged); _groupBy = value; if (_groupBy != null) ((INotifyPropertyChanged)_groupBy).PropertyChanged += new PropertyChangedEventHandler(OnGroupByChanged); if (oldIsBottomLevel != IsBottomLevel) { OnPropertyChanged(new PropertyChangedEventArgs("IsBottomLevel")); } } } // the number of items and groups in the subtree under this group internal int FullCount { get { return _fullCount; } set { _fullCount = value; } } // the most recent index where actvity took place internal int LastIndex { get { return _lastIndex; } set { _lastIndex = value; } } // the first item (leaf) added to this group. If this can't be determined, // DependencyProperty.UnsetValue. internal object SeedItem { get { if (ItemCount > 0 && (GroupBy == null || GroupBy.GroupNames.Count == 0)) { // look for first item, child by child for (int k=0, n=Items.Count; k0) { // child is a nonempty subgroup - ask it return subgroup.SeedItem; } // otherwise child is an empty subgroup - go to next child } // we shouldn't get here, but just in case... return DependencyProperty.UnsetValue; } else { // the group is empty, or it has explicit subgroups. // In either case, we cannot determine the first item - // it could have gone into any of the subgroups. return DependencyProperty.UnsetValue; } } } #endregion Properties #region Internal methods //----------------------------------------------------- // // Internal methods // //------------------------------------------------------ internal void Add(object item) { ChangeCounts(item, +1); ProtectedItems.Add(item); } internal int Remove(object item, bool returnLeafIndex) { int index = -1; int localIndex = ProtectedItems.IndexOf(item); if (localIndex >= 0) { if (returnLeafIndex) { index = LeafIndexFromItem(null, localIndex); } ChangeCounts(item, -1); ProtectedItems.RemoveAt(localIndex); } return index; } internal void Clear() { ProtectedItems.Clear(); FullCount = 1; ProtectedItemCount = 0; } // return the index of the given item within the list of leaves governed // by this group internal int LeafIndexOf(object item) { int leaves = 0; // number of leaves we've passed over so far for (int k=0, n=Items.Count; k = groupBy.GroupNames.Count) { parent.Remove(group, false); } } } void OnGroupByChanged(object sender, System.ComponentModel.PropertyChangedEventArgs e) { OnGroupByChanged(); } #endregion Private methods #region Private fields //----------------------------------------------------- // // Private fields // //------------------------------------------------------ GroupDescription _groupBy; CollectionViewGroupInternal _parentGroup; int _fullCount = 1; int _lastIndex; int _version; // for detecting stale enumerators #endregion Private fields #region Private classes //------------------------------------------------------ // // Private classes // //----------------------------------------------------- private class LeafEnumerator : IEnumerator { public LeafEnumerator(CollectionViewGroupInternal group) { _group = group; DoReset(); // don't call virtual Reset in ctor } void IEnumerator.Reset() { DoReset(); } void DoReset() { _version = _group._version; _index = -1; _subEnum = null; } bool IEnumerator.MoveNext() { // check for invalidated enumerator if (_group._version != _version) throw new InvalidOperationException(); // move forward to the next leaf while (_subEnum == null || !_subEnum.MoveNext()) { // done with the current top-level item. Move to the next one. ++ _index; if (_index >= _group.Items.Count) return false; CollectionViewGroupInternal subgroup = _group.Items[_index] as CollectionViewGroupInternal; if (subgroup == null) { // current item is a leaf - it's the new Current _current = _group.Items[_index]; _subEnum = null; return true; } else { // current item is a subgroup - get its enumerator _subEnum = subgroup.GetLeafEnumerator(); } } // the loop terminates only when we have a subgroup enumerator // positioned at the new Current item _current = _subEnum.Current; return true; } object IEnumerator.Current { get { if (_index < 0 || _index >= _group.Items.Count) throw new InvalidOperationException(); return _current; } } CollectionViewGroupInternal _group; // parent group int _version; // parent group's version at ctor int _index; // current index into Items IEnumerator _subEnum; // enumerator over current subgroup object _current; // current item } #endregion Private classes } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
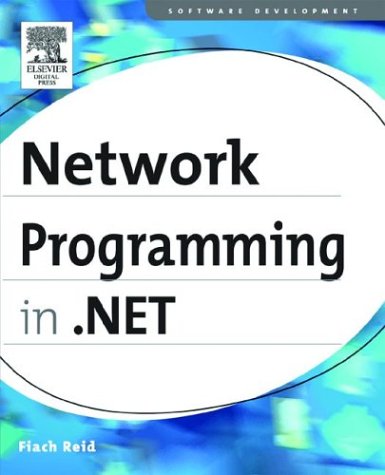
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- OleTxTransaction.cs
- CodeTypeDeclarationCollection.cs
- SchemaName.cs
- ReferenceEqualityComparer.cs
- EntityException.cs
- WindowsTooltip.cs
- Button.cs
- HtmlTernaryTree.cs
- UTF8Encoding.cs
- CompareValidator.cs
- storepermissionattribute.cs
- Faults.cs
- DataListCommandEventArgs.cs
- Vector3DCollection.cs
- TextTabProperties.cs
- SecurityTokenValidationException.cs
- PngBitmapDecoder.cs
- Asn1IntegerConverter.cs
- FieldToken.cs
- LogicalExpr.cs
- SegmentInfo.cs
- SmiRequestExecutor.cs
- ArrangedElementCollection.cs
- DesignerVerb.cs
- XmlNode.cs
- PixelShader.cs
- XamlSerializationHelper.cs
- MetadataItemCollectionFactory.cs
- XmlFormatExtensionPointAttribute.cs
- MetadataArtifactLoaderResource.cs
- SqlDataSourceEnumerator.cs
- BooleanConverter.cs
- GridViewSortEventArgs.cs
- ConfigurationSchemaErrors.cs
- ItemCheckedEvent.cs
- TextHintingModeValidation.cs
- DateTimeValueSerializerContext.cs
- MetaData.cs
- DependencyObjectProvider.cs
- SmtpTransport.cs
- OdbcConnectionStringbuilder.cs
- SqlUnionizer.cs
- HttpPostProtocolReflector.cs
- WindowsListViewItemStartMenu.cs
- DbProviderFactoriesConfigurationHandler.cs
- SelectorItemAutomationPeer.cs
- Binding.cs
- PreProcessor.cs
- SiteMap.cs
- ServerValidateEventArgs.cs
- PersianCalendar.cs
- NetworkInformationPermission.cs
- WebProxyScriptElement.cs
- DataGridToolTip.cs
- ExclusiveHandleList.cs
- ObjectCache.cs
- InputScope.cs
- EventDescriptor.cs
- TypeElement.cs
- RowToFieldTransformer.cs
- SplayTreeNode.cs
- codemethodreferenceexpression.cs
- DataGridViewCellStateChangedEventArgs.cs
- EventSinkHelperWriter.cs
- XamlParser.cs
- SQLInt32.cs
- EtwProvider.cs
- MachinePropertyVariants.cs
- XmlAtomicValue.cs
- WebControl.cs
- BitmapEffectOutputConnector.cs
- ControlSerializer.cs
- StreamingContext.cs
- IconConverter.cs
- SqlDataSourceCache.cs
- PolicyException.cs
- EventSourceCreationData.cs
- Tokenizer.cs
- HorizontalAlignConverter.cs
- WindowsTab.cs
- State.cs
- CodeMemberField.cs
- MetadataItemEmitter.cs
- VideoDrawing.cs
- RsaSecurityTokenAuthenticator.cs
- PageCodeDomTreeGenerator.cs
- EventlogProvider.cs
- EasingKeyFrames.cs
- PageOutputQuality.cs
- Boolean.cs
- TextLineResult.cs
- System.Data_BID.cs
- AssemblyNameEqualityComparer.cs
- ItemsChangedEventArgs.cs
- Calendar.cs
- EntityContainerEmitter.cs
- XmlSiteMapProvider.cs
- TdsParserSessionPool.cs
- Converter.cs
- Aes.cs