Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / ComponentResourceKey.cs / 1305600 / ComponentResourceKey.cs
using System; using System.Reflection; using System.Text; using System.ComponentModel; namespace System.Windows { ////// Key class for custom components to define the names of their resources to be loaded by SystemResources. /// [TypeConverter(typeof(System.Windows.Markup.ComponentResourceKeyConverter))] public class ComponentResourceKey : ResourceKey { ////// Default constructor. Type and ID are null. /// public ComponentResourceKey() { } ////// Type and ID are initialized to the specified parameters. /// /// The Type to which this key is associated. /// A unique ID to differentiate this key from others associated with this type. public ComponentResourceKey(Type typeInTargetAssembly, object resourceId) { if (typeInTargetAssembly == null) { throw new ArgumentNullException("typeInTargetAssembly"); } if (resourceId == null) { throw new ArgumentNullException("resourceId"); } _typeInTargetAssembly = typeInTargetAssembly; _typeInTargetAssemblyInitialized = true; _resourceId = resourceId; _resourceIdInitialized = true; } ////// The Type associated with this resources. Must be in assembly where the resource is located. /// public Type TypeInTargetAssembly { get { return _typeInTargetAssembly; } set { if (value == null) { throw new ArgumentNullException("value"); } if (_typeInTargetAssemblyInitialized) { throw new InvalidOperationException(SR.Get(SRID.ChangingTypeNotAllowed)); } _typeInTargetAssembly = value; _typeInTargetAssemblyInitialized = true; } } ////// Used to determine where to look for the resource dictionary that holds this resource. /// public override Assembly Assembly { get { return (_typeInTargetAssembly != null) ? _typeInTargetAssembly.Assembly : null; } } ////// A unique Id to differentiate this key from other keys associated with the same type. /// public object ResourceId { get { return _resourceId; } set { if (_resourceIdInitialized) { throw new InvalidOperationException(SR.Get(SRID.ChangingIdNotAllowed)); } _resourceId = value; _resourceIdInitialized = true; } } ////// Determines if the passed in object is equal to this object. /// Two keys will be equal if they both have equal Types and IDs. /// /// The object to compare with. ///True if the objects are equal. False otherwise. public override bool Equals(object o) { ComponentResourceKey key = o as ComponentResourceKey; if (key != null) { return ((key._typeInTargetAssembly != null) ? key._typeInTargetAssembly.Equals(this._typeInTargetAssembly) : (this._typeInTargetAssembly == null)) && ((key._resourceId != null) ? key._resourceId.Equals(this._resourceId) : (this._resourceId == null)); } return false; } ////// Serves as a hash function for a particular type. /// public override int GetHashCode() { return ((_typeInTargetAssembly != null) ? _typeInTargetAssembly.GetHashCode() : 0) ^ ((_resourceId != null) ? _resourceId.GetHashCode() : 0); } ////// return string representation of this key /// ///the string representation of the key public override string ToString() { StringBuilder strBuilder = new StringBuilder(256); strBuilder.Append("TargetType="); strBuilder.Append((_typeInTargetAssembly != null) ? _typeInTargetAssembly.FullName : "null"); strBuilder.Append(" ID="); strBuilder.Append((_resourceId != null) ? _resourceId.ToString() : "null"); return strBuilder.ToString(); } private Type _typeInTargetAssembly; private bool _typeInTargetAssemblyInitialized; private object _resourceId; private bool _resourceIdInitialized; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Reflection; using System.Text; using System.ComponentModel; namespace System.Windows { ////// Key class for custom components to define the names of their resources to be loaded by SystemResources. /// [TypeConverter(typeof(System.Windows.Markup.ComponentResourceKeyConverter))] public class ComponentResourceKey : ResourceKey { ////// Default constructor. Type and ID are null. /// public ComponentResourceKey() { } ////// Type and ID are initialized to the specified parameters. /// /// The Type to which this key is associated. /// A unique ID to differentiate this key from others associated with this type. public ComponentResourceKey(Type typeInTargetAssembly, object resourceId) { if (typeInTargetAssembly == null) { throw new ArgumentNullException("typeInTargetAssembly"); } if (resourceId == null) { throw new ArgumentNullException("resourceId"); } _typeInTargetAssembly = typeInTargetAssembly; _typeInTargetAssemblyInitialized = true; _resourceId = resourceId; _resourceIdInitialized = true; } ////// The Type associated with this resources. Must be in assembly where the resource is located. /// public Type TypeInTargetAssembly { get { return _typeInTargetAssembly; } set { if (value == null) { throw new ArgumentNullException("value"); } if (_typeInTargetAssemblyInitialized) { throw new InvalidOperationException(SR.Get(SRID.ChangingTypeNotAllowed)); } _typeInTargetAssembly = value; _typeInTargetAssemblyInitialized = true; } } ////// Used to determine where to look for the resource dictionary that holds this resource. /// public override Assembly Assembly { get { return (_typeInTargetAssembly != null) ? _typeInTargetAssembly.Assembly : null; } } ////// A unique Id to differentiate this key from other keys associated with the same type. /// public object ResourceId { get { return _resourceId; } set { if (_resourceIdInitialized) { throw new InvalidOperationException(SR.Get(SRID.ChangingIdNotAllowed)); } _resourceId = value; _resourceIdInitialized = true; } } ////// Determines if the passed in object is equal to this object. /// Two keys will be equal if they both have equal Types and IDs. /// /// The object to compare with. ///True if the objects are equal. False otherwise. public override bool Equals(object o) { ComponentResourceKey key = o as ComponentResourceKey; if (key != null) { return ((key._typeInTargetAssembly != null) ? key._typeInTargetAssembly.Equals(this._typeInTargetAssembly) : (this._typeInTargetAssembly == null)) && ((key._resourceId != null) ? key._resourceId.Equals(this._resourceId) : (this._resourceId == null)); } return false; } ////// Serves as a hash function for a particular type. /// public override int GetHashCode() { return ((_typeInTargetAssembly != null) ? _typeInTargetAssembly.GetHashCode() : 0) ^ ((_resourceId != null) ? _resourceId.GetHashCode() : 0); } ////// return string representation of this key /// ///the string representation of the key public override string ToString() { StringBuilder strBuilder = new StringBuilder(256); strBuilder.Append("TargetType="); strBuilder.Append((_typeInTargetAssembly != null) ? _typeInTargetAssembly.FullName : "null"); strBuilder.Append(" ID="); strBuilder.Append((_resourceId != null) ? _resourceId.ToString() : "null"); return strBuilder.ToString(); } private Type _typeInTargetAssembly; private bool _typeInTargetAssemblyInitialized; private object _resourceId; private bool _resourceIdInitialized; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
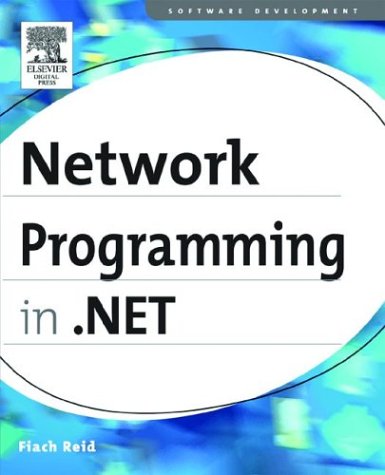
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PropertyGridEditorPart.cs
- CompilerParameters.cs
- X509ChainPolicy.cs
- SqlFormatter.cs
- AutoResetEvent.cs
- DataGridViewRowHeightInfoNeededEventArgs.cs
- ObjectParameterCollection.cs
- CheckedListBox.cs
- CompatibleComparer.cs
- OrthographicCamera.cs
- CodeSnippetExpression.cs
- ImageBrush.cs
- MailAddressCollection.cs
- TheQuery.cs
- SeekStoryboard.cs
- HashSetEqualityComparer.cs
- OleStrCAMarshaler.cs
- WindowsRegion.cs
- NodeFunctions.cs
- NamespaceDisplay.xaml.cs
- IList.cs
- TdsParserSessionPool.cs
- MetabaseSettingsIis7.cs
- FrameworkObject.cs
- WhitespaceRuleReader.cs
- FrameDimension.cs
- ButtonFieldBase.cs
- Transform3DCollection.cs
- DelegatedStream.cs
- TextBreakpoint.cs
- StateRuntime.cs
- InnerItemCollectionView.cs
- KeyValueInternalCollection.cs
- ClientCredentials.cs
- Identity.cs
- KeyboardDevice.cs
- GradientBrush.cs
- RoleManagerEventArgs.cs
- FixedStringLookup.cs
- XomlDesignerLoader.cs
- XmlWrappingReader.cs
- UITypeEditor.cs
- XPathSingletonIterator.cs
- BaseParaClient.cs
- DataGridDesigner.cs
- SQLUtility.cs
- FixedDocument.cs
- ToolStripLocationCancelEventArgs.cs
- OracleCommand.cs
- PackUriHelper.cs
- ApplicationManager.cs
- AccessDataSource.cs
- ObjectDataSource.cs
- XmlSerializerAssemblyAttribute.cs
- MarshalByRefObject.cs
- SqlMethodCallConverter.cs
- Positioning.cs
- SocketAddress.cs
- ISO2022Encoding.cs
- HttpAsyncResult.cs
- ToolStripActionList.cs
- HtmlContainerControl.cs
- GlobalId.cs
- ArgIterator.cs
- ExpressionBuilder.cs
- AutoFocusStyle.xaml.cs
- RefType.cs
- Vertex.cs
- DbInsertCommandTree.cs
- RowCache.cs
- WebControlToolBoxItem.cs
- FileIOPermission.cs
- Item.cs
- DataRelationCollection.cs
- Selection.cs
- TextEndOfParagraph.cs
- xmlsaver.cs
- ValuePatternIdentifiers.cs
- SecurityException.cs
- ActivityExecutor.cs
- TypeDelegator.cs
- QilInvoke.cs
- PartBasedPackageProperties.cs
- XmlSerializerSection.cs
- MetadataArtifactLoaderCompositeFile.cs
- CredentialCache.cs
- ProfileInfo.cs
- Parser.cs
- HwndProxyElementProvider.cs
- DataTableMappingCollection.cs
- SourceItem.cs
- QueryContinueDragEventArgs.cs
- DoubleSumAggregationOperator.cs
- UpdatePanel.cs
- MemoryMappedFileSecurity.cs
- ResourceProperty.cs
- Rules.cs
- EdmPropertyAttribute.cs
- XmlCDATASection.cs
- Schema.cs