Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WebForms / System / Web / UI / Design / WebControlToolBoxItem.cs / 1 / WebControlToolBoxItem.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.Design { using System; using System.Design; using System.Reflection; using System.Runtime.Serialization; using System.ComponentModel; using System.ComponentModel.Design; using System.Diagnostics; using System.Drawing; using System.Drawing.Design; using System.IO; using System.Web.UI; ////// /// [ System.Security.Permissions.SecurityPermission(System.Security.Permissions.SecurityAction.Demand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode), Serializable ] public class WebControlToolboxItem : ToolboxItem { private string toolData = null; private int persistChildren = -1; ///public WebControlToolboxItem() { } /// /// /// public WebControlToolboxItem(Type type) : base(type) { BuildMetadataCache(type); } protected WebControlToolboxItem(SerializationInfo info, StreamingContext context) { Deserialize(info, context); } private void BuildMetadataCache(Type type) { toolData = ExtractToolboxData(type); persistChildren = ExtractPersistChildrenAttribute(type); } ///Initializes a new instance of the ///class. /// /// protected override IComponent[] CreateComponentsCore(IDesignerHost host) { throw new Exception(SR.GetString(SR.Toolbox_OnWebformsPage)); } ////// Creates objects from each Type contained in this ////// , and adds /// them to the specified designer. /// protected override void Deserialize(SerializationInfo info, StreamingContext context) { base.Deserialize(info, context); toolData = info.GetString("ToolData"); persistChildren = info.GetInt32("PersistChildren"); } /// /// Extracts the value of the PersistChildrenAttribute attribute associated with the tool /// private static int ExtractPersistChildrenAttribute(Type type) { Debug.Assert(type != null); if (type != null) { object[] attrs = type.GetCustomAttributes(typeof(PersistChildrenAttribute), /*inherit*/ true); if ((attrs != null) && (attrs.Length == 1)) { Debug.Assert(attrs[0] is PersistChildrenAttribute); PersistChildrenAttribute pca = (PersistChildrenAttribute)attrs[0]; return (pca.Persist ? 1 : 0); } } return (PersistChildrenAttribute.Default.Persist ? 1 : 0); } ////// Extracts the value of the ToolboxData attribute associated with the tool /// private static string ExtractToolboxData(Type type) { Debug.Assert(type != null); string toolData = String.Empty; if (type != null) { object[] attrs = type.GetCustomAttributes(typeof(ToolboxDataAttribute), /*inherit*/ false); if ((attrs != null) && (attrs.Length == 1)) { Debug.Assert(attrs[0] is ToolboxDataAttribute); ToolboxDataAttribute toolDataAttr = (ToolboxDataAttribute)attrs[0]; toolData = toolDataAttr.Data; } else { string typeName = type.Name; toolData = "<{0}:" + typeName + " runat=\"server\">{0}:" + typeName + ">"; } } return toolData; } ///public object GetToolAttributeValue(IDesignerHost host, Type attributeType) { if (attributeType == typeof(PersistChildrenAttribute)) { if (persistChildren == -1) { Type toolType = GetToolType(host); persistChildren = ExtractPersistChildrenAttribute(toolType); } return ((persistChildren == 1) ? true : false); } throw new ArgumentException(SR.GetString(SR.Toolbox_BadAttributeType)); } /// /// /// public string GetToolHtml(IDesignerHost host) { if (toolData != null) { return toolData; } // Create the HTML data that is to be droppped. Type toolType = GetToolType(host); toolData = ExtractToolboxData(toolType); return toolData; } ///Gets the toolbox HTML that represents the corresponding web control on the design surface. ///public Type GetToolType(IDesignerHost host) { if (host == null) { throw new ArgumentNullException("host"); } return GetType(host, AssemblyName, TypeName, true); } /// public override void Initialize(Type type) { base.Initialize(type); BuildMetadataCache(type); } /// /// protected override void Serialize(SerializationInfo info, StreamingContext context) { base.Serialize(info, context); info.AddValue("ToolData", toolData); info.AddValue("PersistChildren", persistChildren); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
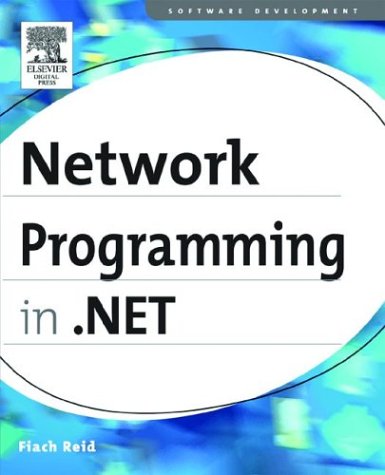
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DummyDataSource.cs
- RegexCharClass.cs
- SecurityTokenAuthenticator.cs
- Highlights.cs
- XmlLangPropertyAttribute.cs
- DirtyTextRange.cs
- HealthMonitoringSectionHelper.cs
- HttpCachePolicy.cs
- SizeAnimation.cs
- DataGridViewComboBoxEditingControl.cs
- GeneralTransform3D.cs
- TreeNodeClickEventArgs.cs
- ContractsBCL.cs
- DBSchemaRow.cs
- PackageDigitalSignature.cs
- BamlResourceDeserializer.cs
- MasterPageParser.cs
- ZipPackage.cs
- DockAndAnchorLayout.cs
- FontWeightConverter.cs
- StructuredTypeInfo.cs
- LogReservationCollection.cs
- TypeGeneratedEventArgs.cs
- MenuItemBindingCollection.cs
- LineBreakRecord.cs
- UDPClient.cs
- KeyedCollection.cs
- TextSegment.cs
- Main.cs
- RawMouseInputReport.cs
- AnnotationResourceChangedEventArgs.cs
- KeyInfo.cs
- CornerRadius.cs
- TextTreeExtractElementUndoUnit.cs
- WebPartMenu.cs
- CorrelationService.cs
- OpenCollectionAsyncResult.cs
- HttpContextServiceHost.cs
- CodeCommentStatement.cs
- AppDomainFactory.cs
- SessionStateContainer.cs
- Facet.cs
- SqlConnection.cs
- WorkflowMarkupSerializationManager.cs
- UserControlDesigner.cs
- ItemsControlAutomationPeer.cs
- DateTimeValueSerializerContext.cs
- SevenBitStream.cs
- FlowNode.cs
- StrokeNodeEnumerator.cs
- NetCodeGroup.cs
- _LoggingObject.cs
- OutputChannelBinder.cs
- Hyperlink.cs
- EntryPointNotFoundException.cs
- ConfigUtil.cs
- PersistChildrenAttribute.cs
- XmlDictionaryReaderQuotas.cs
- TemplateKey.cs
- AnimationClock.cs
- DataMemberFieldEditor.cs
- FormViewDeleteEventArgs.cs
- CustomError.cs
- formatter.cs
- BitmapEffectState.cs
- XomlCompilerHelpers.cs
- CodeCatchClauseCollection.cs
- QueryOutputWriter.cs
- StructuredTypeInfo.cs
- SystemIPAddressInformation.cs
- InputDevice.cs
- WmlLinkAdapter.cs
- PrePrepareMethodAttribute.cs
- Int32KeyFrameCollection.cs
- WhitespaceRule.cs
- PrintingPermissionAttribute.cs
- ViewBox.cs
- StrokeNodeOperations.cs
- UdpTransportBindingElement.cs
- WebBrowsableAttribute.cs
- EventProperty.cs
- SamlAttribute.cs
- RequestResponse.cs
- DataListItemCollection.cs
- SapiRecoInterop.cs
- AmbientValueAttribute.cs
- XmlObjectSerializer.cs
- WebPartConnectionsCloseVerb.cs
- PassportAuthenticationEventArgs.cs
- DiscoveryClientChannelBase.cs
- dsa.cs
- MenuCommands.cs
- Tile.cs
- QueryAccessibilityHelpEvent.cs
- DocumentGridPage.cs
- MenuItemStyle.cs
- DependencyPropertyDescriptor.cs
- DesignRelation.cs
- StructuredProperty.cs
- DependencyPropertyConverter.cs