Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / HttpAsyncResult.cs / 2 / HttpAsyncResult.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * ASP.NET simple internal implementation of IAsyncResult * * Copyright (c) 2000 Microsoft Corporation */ namespace System.Web { using System; using System.Threading; internal class HttpAsyncResult : IAsyncResult { private AsyncCallback _callback; private Object _asyncState; private bool _completed; private bool _completedSynchronously; private Object _result; private Exception _error; // pipeline support private RequestNotificationStatus _status; /* * Constructor with pending result */ internal HttpAsyncResult(AsyncCallback cb, Object state) { _callback = cb; _asyncState = state; _status = RequestNotificationStatus.Continue; } /* * Constructor with known result */ internal HttpAsyncResult(AsyncCallback cb, Object state, bool completed, Object result, Exception error) { _callback = cb; _asyncState = state; _completed = completed; _completedSynchronously = completed; _result = result; _error = error; _status = RequestNotificationStatus.Continue; if (_completed && _callback != null) _callback(this); } internal void SetComplete() { _completed = true; } /* * Helper method to process completions */ internal void Complete(bool synchronous, Object result, Exception error, RequestNotificationStatus status) { _completed = true; _completedSynchronously = synchronous; _result = result; _error = error; _status = status; if (_callback != null) _callback(this); } internal void Complete(bool synchronous, Object result, Exception error) { Complete(synchronous, result, error, RequestNotificationStatus.Continue); } /* * Helper method to implement End call to async method */ internal Object End() { if (_error != null) throw new HttpException(null, _error); return _result; } // // Properties that are not part of IAsyncResult // internal Exception Error { get { return _error;}} internal RequestNotificationStatus Status { get { return _status; } } // // IAsyncResult implementation // public bool IsCompleted { get { return _completed;}} public bool CompletedSynchronously { get { return _completedSynchronously;}} public Object AsyncState { get { return _asyncState;}} public WaitHandle AsyncWaitHandle { get { return null;}} // wait not supported } }
Link Menu
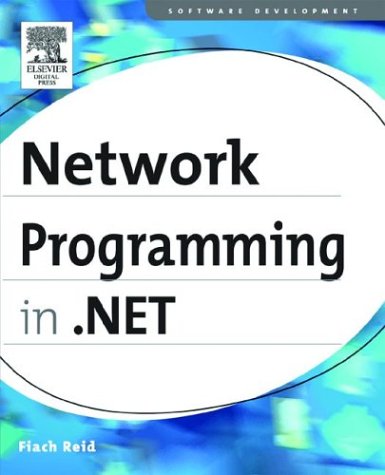
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PromptStyle.cs
- SinglePageViewer.cs
- DebugHandleTracker.cs
- XmlSchemaType.cs
- Label.cs
- ArithmeticException.cs
- Int32RectConverter.cs
- BuildProviderInstallComponent.cs
- OrderedEnumerableRowCollection.cs
- SectionInformation.cs
- MemberDomainMap.cs
- TaskHelper.cs
- securitymgrsite.cs
- StreamAsIStream.cs
- TextControlDesigner.cs
- TransformerTypeCollection.cs
- KeyboardDevice.cs
- BypassElementCollection.cs
- TypeTypeConverter.cs
- safex509handles.cs
- ContextItem.cs
- BindingRestrictions.cs
- XamlVector3DCollectionSerializer.cs
- NamedPipeAppDomainProtocolHandler.cs
- CardSpacePolicyElement.cs
- StoragePropertyMapping.cs
- Thread.cs
- DataServiceConfiguration.cs
- FunctionNode.cs
- TraceContext.cs
- ConnectionPoolManager.cs
- InputLanguage.cs
- StrongNameIdentityPermission.cs
- ItemsControlAutomationPeer.cs
- OleDbErrorCollection.cs
- SQLDateTime.cs
- CharacterHit.cs
- Simplifier.cs
- XPathDocumentBuilder.cs
- CalloutQueueItem.cs
- RadioButton.cs
- MessageQueueConverter.cs
- SamlSubject.cs
- SecurityException.cs
- AdPostCacheSubstitution.cs
- DelegateSerializationHolder.cs
- AppSettingsReader.cs
- MessageFilterException.cs
- DataGridPagerStyle.cs
- CodeStatement.cs
- AttachedPropertyMethodSelector.cs
- arclist.cs
- LinearKeyFrames.cs
- DisplayInformation.cs
- PenCursorManager.cs
- TextContainer.cs
- RegexFCD.cs
- Typography.cs
- ToolStripArrowRenderEventArgs.cs
- NavigationProperty.cs
- ConstructorNeedsTagAttribute.cs
- Menu.cs
- CallbackValidatorAttribute.cs
- LinqDataSourceSelectEventArgs.cs
- SubpageParaClient.cs
- GeometryHitTestResult.cs
- CodeTypeReferenceExpression.cs
- MenuItemBinding.cs
- DesignTimeVisibleAttribute.cs
- JournalEntryListConverter.cs
- SmtpLoginAuthenticationModule.cs
- ProxyWebPart.cs
- ReadOnlyMetadataCollection.cs
- DesignTimeData.cs
- HierarchicalDataSourceIDConverter.cs
- MimeTypePropertyAttribute.cs
- VBIdentifierName.cs
- XmlSchemaException.cs
- TextHidden.cs
- QilDataSource.cs
- ServiceThrottlingElement.cs
- PopupRoot.cs
- AtomEntry.cs
- TableCellCollection.cs
- Quad.cs
- HttpListenerException.cs
- RawMouseInputReport.cs
- DataGridTextBoxColumn.cs
- DefaultAuthorizationContext.cs
- BitmapFrameEncode.cs
- ListDataHelper.cs
- MimeBasePart.cs
- X509CertificateInitiatorServiceCredential.cs
- GenericTypeParameterConverter.cs
- DBCSCodePageEncoding.cs
- SqlFactory.cs
- cache.cs
- PolicyLevel.cs
- DES.cs
- LessThan.cs