Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Mapping / StoragePropertyMapping.cs / 1305376 / StoragePropertyMapping.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Text; using System.Xml; using System.Data.Metadata.Edm; namespace System.Data.Mapping { ////// Mapping metadata for all types of property mappings. /// ////// For Example if conceptually you could represent the CS MSL file as following /// --Mapping /// --EntityContainerMapping ( CNorthwind-->SNorthwind ) /// --EntitySetMapping /// --EntityTypeMapping /// --TableMappingFragment /// --EntityKey /// --ScalarPropertyMap /// --ScalarPropertyMap /// --EntityTypeMapping /// --TableMappingFragment /// --EntityKey /// --ScalarPropertyMap /// --ComplexPropertyMap /// --ScalarPropertyMap /// --ScalarProperyMap /// --ScalarPropertyMap /// --AssociationSetMapping /// --AssociationTypeMapping /// --TableMappingFragment /// --EndPropertyMap /// --ScalarPropertyMap /// --ScalarProperyMap /// --EndPropertyMap /// --ScalarPropertyMap /// --EntityContainerMapping ( CMyDatabase-->SMyDatabase ) /// --CompositionSetMapping /// --CompositionTypeMapping /// --TableMappingFragment /// --ParentEntityKey /// --ScalarPropertyMap /// --ScalarPropertyMap /// --EntityKey /// --ScalarPropertyMap /// --ScalarPropertyMap /// --ComplexPropertyMap /// --ScalarPropertyMap /// --ScalarProperyMap /// --ScalarPropertyMap /// This class represents the metadata for all property map elements in the /// above example. This includes the scalar property maps, complex property maps /// and end property maps. /// internal abstract class StoragePropertyMapping { #region Constructors ////// Construct a new EdmProperty mapping object /// /// The PropertyMetadata object that represents the member for which mapping is being specified internal StoragePropertyMapping(EdmProperty cdmMember) { this.m_cdmMember = cdmMember; } #endregion #region Fields EdmProperty m_cdmMember; //EdmProperty metadata representing the Cdm member for which the mapping is specified #endregion #region Properties ////// The PropertyMetadata object that represents the member for which mapping is being specified /// internal virtual EdmProperty EdmProperty { get { return this.m_cdmMember; } } #endregion #region Methods ////// This method is primarily for debugging purposes. /// Will be removed shortly. /// /// internal virtual void Print(int index) { } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Text; using System.Xml; using System.Data.Metadata.Edm; namespace System.Data.Mapping { ////// Mapping metadata for all types of property mappings. /// ////// For Example if conceptually you could represent the CS MSL file as following /// --Mapping /// --EntityContainerMapping ( CNorthwind-->SNorthwind ) /// --EntitySetMapping /// --EntityTypeMapping /// --TableMappingFragment /// --EntityKey /// --ScalarPropertyMap /// --ScalarPropertyMap /// --EntityTypeMapping /// --TableMappingFragment /// --EntityKey /// --ScalarPropertyMap /// --ComplexPropertyMap /// --ScalarPropertyMap /// --ScalarProperyMap /// --ScalarPropertyMap /// --AssociationSetMapping /// --AssociationTypeMapping /// --TableMappingFragment /// --EndPropertyMap /// --ScalarPropertyMap /// --ScalarProperyMap /// --EndPropertyMap /// --ScalarPropertyMap /// --EntityContainerMapping ( CMyDatabase-->SMyDatabase ) /// --CompositionSetMapping /// --CompositionTypeMapping /// --TableMappingFragment /// --ParentEntityKey /// --ScalarPropertyMap /// --ScalarPropertyMap /// --EntityKey /// --ScalarPropertyMap /// --ScalarPropertyMap /// --ComplexPropertyMap /// --ScalarPropertyMap /// --ScalarProperyMap /// --ScalarPropertyMap /// This class represents the metadata for all property map elements in the /// above example. This includes the scalar property maps, complex property maps /// and end property maps. /// internal abstract class StoragePropertyMapping { #region Constructors ////// Construct a new EdmProperty mapping object /// /// The PropertyMetadata object that represents the member for which mapping is being specified internal StoragePropertyMapping(EdmProperty cdmMember) { this.m_cdmMember = cdmMember; } #endregion #region Fields EdmProperty m_cdmMember; //EdmProperty metadata representing the Cdm member for which the mapping is specified #endregion #region Properties ////// The PropertyMetadata object that represents the member for which mapping is being specified /// internal virtual EdmProperty EdmProperty { get { return this.m_cdmMember; } } #endregion #region Methods ////// This method is primarily for debugging purposes. /// Will be removed shortly. /// /// internal virtual void Print(int index) { } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
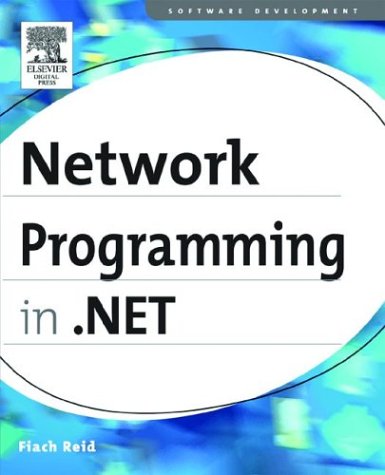
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ActivityDesignerLayoutSerializers.cs
- PseudoWebRequest.cs
- Timer.cs
- IntranetCredentialPolicy.cs
- WebPartCloseVerb.cs
- TemplateControlCodeDomTreeGenerator.cs
- ErrorProvider.cs
- SHA1Managed.cs
- UnsettableComboBox.cs
- GenerateScriptTypeAttribute.cs
- String.cs
- SQLBytesStorage.cs
- XamlSerializerUtil.cs
- XamlWrappingReader.cs
- WindowsStreamSecurityElement.cs
- SoapTransportImporter.cs
- KnowledgeBase.cs
- Attributes.cs
- MergablePropertyAttribute.cs
- UIElementParagraph.cs
- CngProvider.cs
- ServiceManagerHandle.cs
- MessageTraceRecord.cs
- MsmqBindingFilter.cs
- TerminatorSinks.cs
- AsymmetricSignatureDeformatter.cs
- GlyphShapingProperties.cs
- SessionStateItemCollection.cs
- FileSecurity.cs
- ConnectionsZone.cs
- PageContent.cs
- SqlDataSourceStatusEventArgs.cs
- HashCodeCombiner.cs
- XmlBindingWorker.cs
- NameValueCollection.cs
- ObjectNotFoundException.cs
- WebPartDescription.cs
- RegisteredArrayDeclaration.cs
- SimpleWebHandlerParser.cs
- CngAlgorithmGroup.cs
- UrlPropertyAttribute.cs
- OperationPickerDialog.designer.cs
- BmpBitmapDecoder.cs
- ObjectDataSourceDisposingEventArgs.cs
- KeyValueSerializer.cs
- DbTypeMap.cs
- BinaryWriter.cs
- CodeObject.cs
- DocumentPageTextView.cs
- FunctionCommandText.cs
- XmlSerializer.cs
- JoinSymbol.cs
- PackageRelationshipCollection.cs
- XamlPoint3DCollectionSerializer.cs
- PixelFormats.cs
- ProxyBuilder.cs
- HandlerWithFactory.cs
- OracleInfoMessageEventArgs.cs
- XmlBinaryReaderSession.cs
- SHA384Managed.cs
- DependencySource.cs
- RelationshipEndMember.cs
- RijndaelManaged.cs
- DesignerEditorPartChrome.cs
- ProcessInfo.cs
- CompilerParameters.cs
- WebHttpBinding.cs
- CollectionBase.cs
- OperationAbortedException.cs
- Sql8ConformanceChecker.cs
- URI.cs
- PrinterResolution.cs
- AssociatedControlConverter.cs
- SchemaSetCompiler.cs
- KeySplineConverter.cs
- CqlWriter.cs
- DataGridViewUtilities.cs
- Misc.cs
- FixedTextPointer.cs
- _AutoWebProxyScriptWrapper.cs
- FixedLineResult.cs
- ImageCodecInfo.cs
- BindingList.cs
- PackageProperties.cs
- WeakReferenceList.cs
- RemotingAttributes.cs
- SQLGuidStorage.cs
- IntegerValidator.cs
- ObjectDisposedException.cs
- CustomGrammar.cs
- Interlocked.cs
- SqlDeflator.cs
- RuleProcessor.cs
- HebrewCalendar.cs
- DockPanel.cs
- TemplateControlBuildProvider.cs
- VariableQuery.cs
- Floater.cs
- BindableTemplateBuilder.cs
- Calendar.cs