Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / clr / src / BCL / System / IO / DirectoryInfo.cs / 1 / DirectoryInfo.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: DirectoryInfo ** ** ** Purpose: Exposes routines for enumerating through a ** directory. ** ** April 11,2000 ** ===========================================================*/ using System; using System.Collections; using System.Security; #if !FEATURE_PAL using System.Security.AccessControl; #endif using System.Security.Permissions; using Microsoft.Win32; using System.Text; using System.Runtime.InteropServices; using System.Globalization; using System.Runtime.Serialization; using System.Runtime.Versioning; namespace System.IO { [Serializable] [ComVisible(true)] public sealed class DirectoryInfo : FileSystemInfo { private String[] demandDir; [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public DirectoryInfo(String path) { if (path==null) throw new ArgumentNullException("path"); // Special case ":" to point to " " instead if ((path.Length == 2) && (path[1] == ':')) OriginalPath = "."; else OriginalPath = path; // Must fully qualify the path for the security check String fullPath = Path.GetFullPathInternal(path); demandDir = new String[] {Directory.GetDemandDir(fullPath, true)}; new FileIOPermission(FileIOPermissionAccess.Read, demandDir, false, false ).Demand(); FullPath = fullPath; } [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] internal DirectoryInfo(String fullPath, bool junk) { BCLDebug.Assert(Path.GetRootLength(fullPath) > 0, "fullPath must be fully qualified!"); // Fast path when we know a DirectoryInfo exists. OriginalPath = Path.GetFileName(fullPath); FullPath = fullPath; demandDir = new String[] {Directory.GetDemandDir(fullPath, true)}; } private DirectoryInfo(SerializationInfo info, StreamingContext context) : base(info, context) { demandDir = new String[] {Directory.GetDemandDir(FullPath, true)}; new FileIOPermission(FileIOPermissionAccess.Read, demandDir, false, false ).Demand(); } public override String Name { [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] get { // FullPath might be either "c:\bar" or "c:\bar\". Handle // those cases, as well as avoiding mangling "c:\". String s = FullPath; if (s.Length > 3) { if (s.EndsWith(Path.DirectorySeparatorChar)) s = FullPath.Substring(0, FullPath.Length - 1); return Path.GetFileName(s); } return FullPath; // For rooted paths, like "c:\" } } public DirectoryInfo Parent { [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] get { String parentName; // FullPath might be either "c:\bar" or "c:\bar\". Handle // those cases, as well as avoiding mangling "c:\". String s = FullPath; if (s.Length > 3 && s.EndsWith(Path.DirectorySeparatorChar)) s = FullPath.Substring(0, FullPath.Length - 1); parentName = Path.GetDirectoryName(s); if (parentName==null) return null; DirectoryInfo dir = new DirectoryInfo(parentName,false); new FileIOPermission(FileIOPermissionAccess.PathDiscovery | FileIOPermissionAccess.Read, dir.demandDir, false, false).Demand(); return dir; } } [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public DirectoryInfo CreateSubdirectory(String path) { #if !FEATURE_PAL return CreateSubdirectory(path, null); } [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public DirectoryInfo CreateSubdirectory(String path, DirectorySecurity directorySecurity) { #endif if (path==null) throw new ArgumentNullException("path"); String newDirs = Path.InternalCombine(FullPath, path); String fullPath = Path.GetFullPathInternal(newDirs); if (0!=String.Compare(FullPath,0,fullPath,0, FullPath.Length,StringComparison.OrdinalIgnoreCase)) { String displayPath = __Error.GetDisplayablePath(OriginalPath, false); throw new ArgumentException(String.Format(CultureInfo.CurrentCulture, Environment.GetResourceString("Argument_InvalidSubPath"), path, displayPath)); } // Ensure we have permission to create this subdirectory. String demandDir = Directory.GetDemandDir(fullPath, true); new FileIOPermission(FileIOPermissionAccess.Write, new String[] { demandDir }, false, false ).Demand(); #if !FEATURE_PAL Directory.InternalCreateDirectory(fullPath, path, directorySecurity); #else Directory.InternalCreateDirectory(fullPath, path); #endif // Check for read permission to directory we hand back by calling this constructor. return new DirectoryInfo(fullPath); } [ResourceExposure(ResourceScope.None)] [ResourceConsumption(ResourceScope.Machine, ResourceScope.Machine)] public void Create() { #if !FEATURE_PAL Directory.InternalCreateDirectory(FullPath, OriginalPath, null); #else Directory.InternalCreateDirectory(FullPath, OriginalPath); #endif } #if !FEATURE_PAL [ResourceExposure(ResourceScope.None)] [ResourceConsumption(ResourceScope.Machine, ResourceScope.Machine)] public void Create(DirectorySecurity directorySecurity) { Directory.InternalCreateDirectory(FullPath, OriginalPath, directorySecurity); } #endif // Tests if the given path refers to an existing DirectoryInfo on disk. // // Your application must have Read permission to the directory's // contents. // public override bool Exists { get { try { if (_dataInitialised == -1) Refresh(); if (_dataInitialised != 0) // Refresh was unable to initialise the data return false; return _data.fileAttributes != -1 && (_data.fileAttributes & Win32Native.FILE_ATTRIBUTE_DIRECTORY) != 0; } catch { return false; } } } #if !FEATURE_PAL [ResourceExposure(ResourceScope.None)] [ResourceConsumption(ResourceScope.Machine, ResourceScope.Machine)] public DirectorySecurity GetAccessControl() { return Directory.GetAccessControl(FullPath, AccessControlSections.Access | AccessControlSections.Owner | AccessControlSections.Group); } [ResourceExposure(ResourceScope.None)] [ResourceConsumption(ResourceScope.Machine, ResourceScope.Machine)] public DirectorySecurity GetAccessControl(AccessControlSections includeSections) { return Directory.GetAccessControl(FullPath, includeSections); } [ResourceExposure(ResourceScope.None)] [ResourceConsumption(ResourceScope.Machine, ResourceScope.Machine)] public void SetAccessControl(DirectorySecurity directorySecurity) { Directory.SetAccessControl(FullPath, directorySecurity); } #endif // Returns an array of Files in the current DirectoryInfo matching the // given search criteria (ie, "*.txt"). [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public FileInfo[] GetFiles(String searchPattern) { return GetFiles(searchPattern, SearchOption.TopDirectoryOnly); } // Converts the fully qualified user path returned by InternalGetFileDirectoryNames // into fullpath by combining the relevant portion of it with the fullpath of this directory // For ex, converts foo\bar.txt into 'c:\temp\foo\bar.txt', where FullPath is 'c:\temp\foo' // and OriginalPath (aka userpath) is 'foo' private string FixupFileDirFullPath(String fileDirUserPath) { BCLDebug.Assert(fileDirUserPath != null,"InternalGetFileDirectoryNames returned paths should not be null!"); BCLDebug.Assert(fileDirUserPath.StartsWith(OriginalPath, StringComparison.Ordinal),"InternalGetFileDirectoryNames returned paths should start with user path!"); String fileDirFullPath; if (OriginalPath.Length == 0) { fileDirFullPath = Path.InternalCombine(FullPath, fileDirUserPath); } else if (OriginalPath.EndsWith(Path.DirectorySeparatorChar) || OriginalPath.EndsWith(Path.AltDirectorySeparatorChar)) { BCLDebug.Assert((fileDirUserPath[OriginalPath.Length-1] == Path.DirectorySeparatorChar) || (fileDirUserPath[OriginalPath.Length-1] == Path.AltDirectorySeparatorChar),"InternalGetFileDirectoryNames returned incorrect user path!"); fileDirFullPath = Path.InternalCombine(FullPath, fileDirUserPath.Substring(OriginalPath.Length)); } else { BCLDebug.Assert((fileDirUserPath[OriginalPath.Length] == Path.DirectorySeparatorChar),"InternalGetFileDirectoryNames returned incorrect user path!"); fileDirFullPath = Path.InternalCombine(FullPath, fileDirUserPath.Substring(OriginalPath.Length + 1)); } return fileDirFullPath; } // Returns an array of Files in the current DirectoryInfo matching the // given search criteria (ie, "*.txt"). [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public FileInfo[] GetFiles(String searchPattern, SearchOption searchOption) { if (searchPattern==null) throw new ArgumentNullException("searchPattern"); String[] fileNames = Directory.InternalGetFileDirectoryNames(FullPath, OriginalPath, searchPattern, true, false, searchOption); // We need full path for permission check. // InternalGetFileDirectoryNames returns qualified user path, // i.e, path starts from OriginalPath. We need to convert this to fullpath. for (int i = 0; i < fileNames.Length; i++) { fileNames[i] = FixupFileDirFullPath(fileNames[i]); } if (fileNames.Length != 0) new FileIOPermission(FileIOPermissionAccess.Read, fileNames, false, false).Demand(); FileInfo[] files = new FileInfo[fileNames.Length]; for(int i=0; i :" to point to " " instead if ((path.Length == 2) && (path[1] == ':')) OriginalPath = "."; else OriginalPath = path; // Must fully qualify the path for the security check String fullPath = Path.GetFullPathInternal(path); demandDir = new String[] {Directory.GetDemandDir(fullPath, true)}; new FileIOPermission(FileIOPermissionAccess.Read, demandDir, false, false ).Demand(); FullPath = fullPath; } [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] internal DirectoryInfo(String fullPath, bool junk) { BCLDebug.Assert(Path.GetRootLength(fullPath) > 0, "fullPath must be fully qualified!"); // Fast path when we know a DirectoryInfo exists. OriginalPath = Path.GetFileName(fullPath); FullPath = fullPath; demandDir = new String[] {Directory.GetDemandDir(fullPath, true)}; } private DirectoryInfo(SerializationInfo info, StreamingContext context) : base(info, context) { demandDir = new String[] {Directory.GetDemandDir(FullPath, true)}; new FileIOPermission(FileIOPermissionAccess.Read, demandDir, false, false ).Demand(); } public override String Name { [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] get { // FullPath might be either "c:\bar" or "c:\bar\". Handle // those cases, as well as avoiding mangling "c:\". String s = FullPath; if (s.Length > 3) { if (s.EndsWith(Path.DirectorySeparatorChar)) s = FullPath.Substring(0, FullPath.Length - 1); return Path.GetFileName(s); } return FullPath; // For rooted paths, like "c:\" } } public DirectoryInfo Parent { [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] get { String parentName; // FullPath might be either "c:\bar" or "c:\bar\". Handle // those cases, as well as avoiding mangling "c:\". String s = FullPath; if (s.Length > 3 && s.EndsWith(Path.DirectorySeparatorChar)) s = FullPath.Substring(0, FullPath.Length - 1); parentName = Path.GetDirectoryName(s); if (parentName==null) return null; DirectoryInfo dir = new DirectoryInfo(parentName,false); new FileIOPermission(FileIOPermissionAccess.PathDiscovery | FileIOPermissionAccess.Read, dir.demandDir, false, false).Demand(); return dir; } } [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public DirectoryInfo CreateSubdirectory(String path) { #if !FEATURE_PAL return CreateSubdirectory(path, null); } [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public DirectoryInfo CreateSubdirectory(String path, DirectorySecurity directorySecurity) { #endif if (path==null) throw new ArgumentNullException("path"); String newDirs = Path.InternalCombine(FullPath, path); String fullPath = Path.GetFullPathInternal(newDirs); if (0!=String.Compare(FullPath,0,fullPath,0, FullPath.Length,StringComparison.OrdinalIgnoreCase)) { String displayPath = __Error.GetDisplayablePath(OriginalPath, false); throw new ArgumentException(String.Format(CultureInfo.CurrentCulture, Environment.GetResourceString("Argument_InvalidSubPath"), path, displayPath)); } // Ensure we have permission to create this subdirectory. String demandDir = Directory.GetDemandDir(fullPath, true); new FileIOPermission(FileIOPermissionAccess.Write, new String[] { demandDir }, false, false ).Demand(); #if !FEATURE_PAL Directory.InternalCreateDirectory(fullPath, path, directorySecurity); #else Directory.InternalCreateDirectory(fullPath, path); #endif // Check for read permission to directory we hand back by calling this constructor. return new DirectoryInfo(fullPath); } [ResourceExposure(ResourceScope.None)] [ResourceConsumption(ResourceScope.Machine, ResourceScope.Machine)] public void Create() { #if !FEATURE_PAL Directory.InternalCreateDirectory(FullPath, OriginalPath, null); #else Directory.InternalCreateDirectory(FullPath, OriginalPath); #endif } #if !FEATURE_PAL [ResourceExposure(ResourceScope.None)] [ResourceConsumption(ResourceScope.Machine, ResourceScope.Machine)] public void Create(DirectorySecurity directorySecurity) { Directory.InternalCreateDirectory(FullPath, OriginalPath, directorySecurity); } #endif // Tests if the given path refers to an existing DirectoryInfo on disk. // // Your application must have Read permission to the directory's // contents. // public override bool Exists { get { try { if (_dataInitialised == -1) Refresh(); if (_dataInitialised != 0) // Refresh was unable to initialise the data return false; return _data.fileAttributes != -1 && (_data.fileAttributes & Win32Native.FILE_ATTRIBUTE_DIRECTORY) != 0; } catch { return false; } } } #if !FEATURE_PAL [ResourceExposure(ResourceScope.None)] [ResourceConsumption(ResourceScope.Machine, ResourceScope.Machine)] public DirectorySecurity GetAccessControl() { return Directory.GetAccessControl(FullPath, AccessControlSections.Access | AccessControlSections.Owner | AccessControlSections.Group); } [ResourceExposure(ResourceScope.None)] [ResourceConsumption(ResourceScope.Machine, ResourceScope.Machine)] public DirectorySecurity GetAccessControl(AccessControlSections includeSections) { return Directory.GetAccessControl(FullPath, includeSections); } [ResourceExposure(ResourceScope.None)] [ResourceConsumption(ResourceScope.Machine, ResourceScope.Machine)] public void SetAccessControl(DirectorySecurity directorySecurity) { Directory.SetAccessControl(FullPath, directorySecurity); } #endif // Returns an array of Files in the current DirectoryInfo matching the // given search criteria (ie, "*.txt"). [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public FileInfo[] GetFiles(String searchPattern) { return GetFiles(searchPattern, SearchOption.TopDirectoryOnly); } // Converts the fully qualified user path returned by InternalGetFileDirectoryNames // into fullpath by combining the relevant portion of it with the fullpath of this directory // For ex, converts foo\bar.txt into 'c:\temp\foo\bar.txt', where FullPath is 'c:\temp\foo' // and OriginalPath (aka userpath) is 'foo' private string FixupFileDirFullPath(String fileDirUserPath) { BCLDebug.Assert(fileDirUserPath != null,"InternalGetFileDirectoryNames returned paths should not be null!"); BCLDebug.Assert(fileDirUserPath.StartsWith(OriginalPath, StringComparison.Ordinal),"InternalGetFileDirectoryNames returned paths should start with user path!"); String fileDirFullPath; if (OriginalPath.Length == 0) { fileDirFullPath = Path.InternalCombine(FullPath, fileDirUserPath); } else if (OriginalPath.EndsWith(Path.DirectorySeparatorChar) || OriginalPath.EndsWith(Path.AltDirectorySeparatorChar)) { BCLDebug.Assert((fileDirUserPath[OriginalPath.Length-1] == Path.DirectorySeparatorChar) || (fileDirUserPath[OriginalPath.Length-1] == Path.AltDirectorySeparatorChar),"InternalGetFileDirectoryNames returned incorrect user path!"); fileDirFullPath = Path.InternalCombine(FullPath, fileDirUserPath.Substring(OriginalPath.Length)); } else { BCLDebug.Assert((fileDirUserPath[OriginalPath.Length] == Path.DirectorySeparatorChar),"InternalGetFileDirectoryNames returned incorrect user path!"); fileDirFullPath = Path.InternalCombine(FullPath, fileDirUserPath.Substring(OriginalPath.Length + 1)); } return fileDirFullPath; } // Returns an array of Files in the current DirectoryInfo matching the // given search criteria (ie, "*.txt"). [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public FileInfo[] GetFiles(String searchPattern, SearchOption searchOption) { if (searchPattern==null) throw new ArgumentNullException("searchPattern"); String[] fileNames = Directory.InternalGetFileDirectoryNames(FullPath, OriginalPath, searchPattern, true, false, searchOption); // We need full path for permission check. // InternalGetFileDirectoryNames returns qualified user path, // i.e, path starts from OriginalPath. We need to convert this to fullpath. for (int i = 0; i < fileNames.Length; i++) { fileNames[i] = FixupFileDirFullPath(fileNames[i]); } if (fileNames.Length != 0) new FileIOPermission(FileIOPermissionAccess.Read, fileNames, false, false).Demand(); FileInfo[] files = new FileInfo[fileNames.Length]; for(int i=0; i
Link Menu
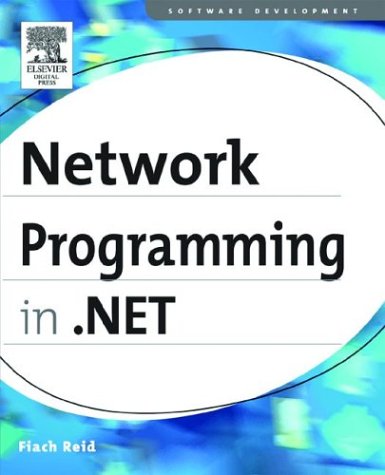
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ValidationPropertyAttribute.cs
- EditorZoneBase.cs
- CalendarDay.cs
- ValidatorCompatibilityHelper.cs
- WebPartHelpVerb.cs
- FieldAccessException.cs
- IisHelper.cs
- SqlParameterizer.cs
- _IPv4Address.cs
- HttpAsyncResult.cs
- PageAdapter.cs
- ControlLocalizer.cs
- ReadOnlyDictionary.cs
- ProviderBase.cs
- HttpValueCollection.cs
- BaseParser.cs
- EntityStoreSchemaFilterEntry.cs
- ControlEvent.cs
- TreeBuilderBamlTranslator.cs
- DeferrableContent.cs
- PropertyDescriptorGridEntry.cs
- PermissionSetTriple.cs
- pingexception.cs
- ClearTypeHintValidation.cs
- BezierSegment.cs
- FusionWrap.cs
- UriSchemeKeyedCollection.cs
- ServiceHostingEnvironment.cs
- VarRemapper.cs
- Wizard.cs
- EntitySetDataBindingList.cs
- User.cs
- TableLayout.cs
- DmlSqlGenerator.cs
- ProviderConnectionPointCollection.cs
- EventListener.cs
- BoundField.cs
- DbTransaction.cs
- TimeSpanValidator.cs
- TypedAsyncResult.cs
- AuthenticationModulesSection.cs
- DurableMessageDispatchInspector.cs
- X509SubjectKeyIdentifierClause.cs
- SafeNativeMethods.cs
- CustomLineCap.cs
- PageThemeBuildProvider.cs
- ObjectStateEntryOriginalDbUpdatableDataRecord.cs
- CommandBindingCollection.cs
- HebrewCalendar.cs
- ClaimTypes.cs
- OuterGlowBitmapEffect.cs
- ComponentSerializationService.cs
- EntityDataSourceDataSelection.cs
- WhitespaceRuleLookup.cs
- CustomUserNameSecurityTokenAuthenticator.cs
- Equal.cs
- ModuleConfigurationInfo.cs
- TabControlToolboxItem.cs
- Size.cs
- RawStylusInputCustomDataList.cs
- TryLoadRunnableWorkflowCommand.cs
- SamlDelegatingWriter.cs
- CloseCryptoHandleRequest.cs
- Highlights.cs
- WebBrowserHelper.cs
- DependencyPropertyHelper.cs
- CharKeyFrameCollection.cs
- ExceptionUtil.cs
- AesCryptoServiceProvider.cs
- WaitHandleCannotBeOpenedException.cs
- HitTestParameters.cs
- HtmlTableRowCollection.cs
- SqlUnionizer.cs
- HierarchicalDataSourceControl.cs
- ReadOnlyAttribute.cs
- SqlColumnizer.cs
- HttpErrorTraceRecord.cs
- Rectangle.cs
- RecognizerBase.cs
- EventKeyword.cs
- DataTablePropertyDescriptor.cs
- CharUnicodeInfo.cs
- ReadOnlyMetadataCollection.cs
- TypeUsage.cs
- Thread.cs
- OptimalTextSource.cs
- SystemInfo.cs
- HttpFileCollectionBase.cs
- InvalidWMPVersionException.cs
- TreeNodeCollectionEditor.cs
- RtfControlWordInfo.cs
- ExpressionsCollectionEditor.cs
- PeerDuplexChannelListener.cs
- SoapExtensionImporter.cs
- OneWayChannelListener.cs
- DesignBindingValueUIHandler.cs
- BitStream.cs
- LogicalMethodInfo.cs
- XmlEntityReference.cs
- CompletionCallbackWrapper.cs