Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / HttpValueCollection.cs / 1 / HttpValueCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Ordered String/String[] collection of name/value pairs * Based on NameValueCollection -- adds parsing from string, cookie collection * * Copyright (c) 2000 Microsoft Corporation */ namespace System.Web { using System.Runtime.Serialization; using System.Runtime.Serialization.Formatters; using System.Text; using System.Runtime.InteropServices; using System.Collections; using System.Collections.Specialized; using System.Globalization; using System.Web.Util; using System.Web.UI; [Serializable()] internal class HttpValueCollection : NameValueCollection { internal HttpValueCollection(): base(StringComparer.OrdinalIgnoreCase) { } #if UNUSED_CODE internal HttpValueCollection(String str) : this(str, false, false, Encoding.UTF8) { } internal HttpValueCollection(String str, bool readOnly) : this(str, readOnly, false, Encoding.UTF8) { } #endif internal HttpValueCollection(String str, bool readOnly, bool urlencoded, Encoding encoding): base(StringComparer.OrdinalIgnoreCase) { if (!String.IsNullOrEmpty(str)) FillFromString(str, urlencoded, encoding); IsReadOnly = readOnly; } internal HttpValueCollection(int capacity) : base(capacity, StringComparer.OrdinalIgnoreCase) { } #if UNUSED_CODE internal HttpValueCollection(int capacity, String str) : this(capacity, str, false, false, Encoding.UTF8) { } internal HttpValueCollection(int capacity, String str, bool readOnly) : this(capacity, str, readOnly, false, Encoding.UTF8) { } internal HttpValueCollection(int capacity, String str, bool readOnly, bool urlencoded, Encoding encoding) : base(capacity, StringComparer.OrdinalIgnoreCase) { if (!String.IsNullOrEmpty(str)) FillFromString(str, urlencoded, encoding); IsReadOnly = readOnly; } #endif protected HttpValueCollection(SerializationInfo info, StreamingContext context) : base(info, context) { } internal void MakeReadOnly() { IsReadOnly = true; } internal void MakeReadWrite() { IsReadOnly = false; } internal void FillFromString(String s) { FillFromString(s, false, null); } internal void FillFromString(String s, bool urlencoded, Encoding encoding) { int l = (s != null) ? s.Length : 0; int i = 0; while (i < l) { // find next & while noting first = on the way (and if there are more) int si = i; int ti = -1; while (i < l) { char ch = s[i]; if (ch == '=') { if (ti < 0) ti = i; } else if (ch == '&') { break; } i++; } // extract the name / value pair String name = null; String value = null; if (ti >= 0) { name = s.Substring(si, ti-si); value = s.Substring(ti+1, i-ti-1); } else { value = s.Substring(si, i-si); } // add name / value pair to the collection if (urlencoded) base.Add( HttpUtility.UrlDecode(name, encoding), HttpUtility.UrlDecode(value, encoding)); else base.Add(name, value); // trailing '&' if (i == l-1 && s[i] == '&') base.Add(null, String.Empty); i++; } } internal void FillFromEncodedBytes(byte[] bytes, Encoding encoding) { int l = (bytes != null) ? bytes.Length : 0; int i = 0; while (i < l) { // find next & while noting first = on the way (and if there are more) int si = i; int ti = -1; while (i < l) { byte b = bytes[i]; if (b == '=') { if (ti < 0) ti = i; } else if (b == '&') { break; } i++; } // extract the name / value pair String name, value; if (ti >= 0) { name = HttpUtility.UrlDecode(bytes, si, ti-si, encoding); value = HttpUtility.UrlDecode(bytes, ti+1, i-ti-1, encoding); } else { name = null; value = HttpUtility.UrlDecode(bytes, si, i-si, encoding); } // add name / value pair to the collection base.Add(name, value); // trailing '&' if (i == l-1 && bytes[i] == '&') base.Add(null, String.Empty); i++; } } internal void Add(HttpCookieCollection c) { int n = c.Count; for (int i = 0; i < n; i++) { HttpCookie cookie = c.Get(i); base.Add(cookie.Name, cookie.Value); } } internal void Reset() { base.Clear(); } public override String ToString() { return ToString(true); } internal virtual String ToString(bool urlencoded) { return ToString(urlencoded, null); } internal virtual String ToString(bool urlencoded, IDictionary excludeKeys) { int n = Count; if (n == 0) return String.Empty; StringBuilder s = new StringBuilder(); String key, keyPrefix, item; bool ignoreViewStateKeys = (excludeKeys != null && excludeKeys[Page.ViewStateFieldPrefixID] != null); for (int i = 0; i < n; i++) { key = GetKey(i); // Review: improve this... Special case hack for __VIEWSTATE# if (ignoreViewStateKeys && key != null && key.StartsWith(Page.ViewStateFieldPrefixID, StringComparison.Ordinal)) continue; if (excludeKeys != null && key != null && excludeKeys[key] != null) continue; if (urlencoded) key = HttpUtility.UrlEncodeUnicode(key); keyPrefix = (!String.IsNullOrEmpty(key)) ? (key + "=") : String.Empty; ArrayList values = (ArrayList)BaseGet(i); int numValues = (values != null) ? values.Count : 0; if (s.Length > 0) s.Append('&'); if (numValues == 1) { s.Append(keyPrefix); item = (String)values[0]; if (urlencoded) item = HttpUtility.UrlEncodeUnicode(item); s.Append(item); } else if (numValues == 0) { s.Append(keyPrefix); } else { for (int j = 0; j < numValues; j++) { if (j > 0) s.Append('&'); s.Append(keyPrefix); item = (String)values[j]; if (urlencoded) item = HttpUtility.UrlEncodeUnicode(item); s.Append(item); } } } return s.ToString(); } } }
Link Menu
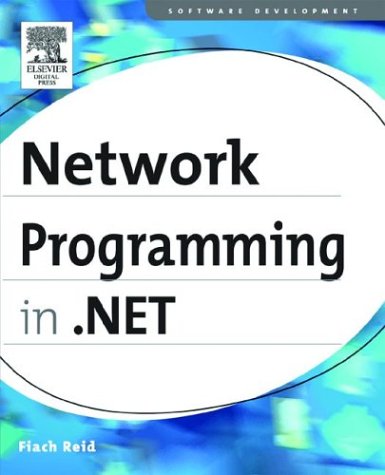
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataColumnChangeEvent.cs
- MimeTypePropertyAttribute.cs
- StatusBar.cs
- UseManagedPresentationElement.cs
- XmlUrlResolver.cs
- LinkUtilities.cs
- PolyQuadraticBezierSegment.cs
- InternalEnumValidatorAttribute.cs
- MissingManifestResourceException.cs
- GridItemPatternIdentifiers.cs
- XamlStyleSerializer.cs
- XmlHierarchicalEnumerable.cs
- Facet.cs
- ExclusiveCanonicalizationTransform.cs
- TextBox.cs
- TextParagraph.cs
- HttpProfileBase.cs
- LinqDataSourceHelper.cs
- NamespaceEmitter.cs
- OptimalBreakSession.cs
- SplayTreeNode.cs
- RayHitTestParameters.cs
- SystemNetworkInterface.cs
- HwndSourceKeyboardInputSite.cs
- XamlInterfaces.cs
- WindowsTab.cs
- RSAOAEPKeyExchangeDeformatter.cs
- PathFigureCollectionConverter.cs
- ObjectStorage.cs
- FieldMetadata.cs
- UnsafeNativeMethods.cs
- SqlWebEventProvider.cs
- Marshal.cs
- DataRow.cs
- OutputCacheSettings.cs
- EntityException.cs
- TraceLog.cs
- EventLogTraceListener.cs
- ProviderConnectionPoint.cs
- Win32KeyboardDevice.cs
- StatusBar.cs
- AppModelKnownContentFactory.cs
- DecimalKeyFrameCollection.cs
- SimpleWebHandlerParser.cs
- PropertyKey.cs
- NamespaceDecl.cs
- QueryIntervalOp.cs
- FrameworkContentElement.cs
- InvariantComparer.cs
- Console.cs
- ResourceProperty.cs
- WebPartDisplayMode.cs
- DocobjHost.cs
- ContainerUIElement3D.cs
- SevenBitStream.cs
- SplineKeyFrames.cs
- FixedPage.cs
- XmlBufferReader.cs
- ContainerControl.cs
- AccessViolationException.cs
- CallbackException.cs
- TableLayoutStyle.cs
- CompositionDesigner.cs
- SerializationBinder.cs
- FunctionParameter.cs
- MetaDataInfo.cs
- DataTableClearEvent.cs
- CaretElement.cs
- MailWriter.cs
- VBIdentifierNameEditor.cs
- SafeBitVector32.cs
- SafeLocalMemHandle.cs
- Zone.cs
- Form.cs
- WinHttpWebProxyFinder.cs
- localization.cs
- ToolboxItemFilterAttribute.cs
- ClientApiGenerator.cs
- ConfigurationLocation.cs
- XmlAttributes.cs
- TextEndOfSegment.cs
- Ticks.cs
- EmbeddedMailObjectsCollection.cs
- ElementUtil.cs
- ThousandthOfEmRealDoubles.cs
- DeploymentExceptionMapper.cs
- TargetInvocationException.cs
- TypeUsage.cs
- CommonDialog.cs
- EdmSchemaAttribute.cs
- DataStreams.cs
- CustomErrorsSectionWrapper.cs
- CompilationLock.cs
- ReflectionHelper.cs
- NativeMethodsCLR.cs
- MD5CryptoServiceProvider.cs
- FileIOPermission.cs
- DataGridTable.cs
- sqlstateclientmanager.cs
- AccessDataSource.cs