Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / System / Windows / Media / FactoryMaker.cs / 1 / FactoryMaker.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2003 // // File: FactoryMaker.cs // //----------------------------------------------------------------------------- using System; using System.Security; using System.Security.Permissions; using System.Diagnostics; using MS.Internal; using MS.Win32; using System.Windows.Media.Composition; using Microsoft.Internal; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using UnsafeNativeMethods=MS.Win32.PresentationCore.UnsafeNativeMethods; namespace System.Windows.Media { ////// This is a internal class which is used by non context affinity objects /// to get access to a MIL factory object. /// internal class FactoryMaker: IDisposable { private bool _disposed = false; ////// Critical - Create unmanaged critical resource /// [SecurityCritical] internal FactoryMaker() { lock (s_factoryMakerLock) { // If we haven't have a factory, create one if (s_pFactory == IntPtr.Zero) { // Create the Core MIL factory. // Note: the call below might throw exception. The caller // should catch it. We won't add ref counter here if this // happens. HRESULT.Check(UnsafeNativeMethods.MILFactory2.CreateFactory(out s_pFactory, MS.Internal.Composition.Version.MilSdkVersion)); } s_cInstance++; _fValidObject = true; } } ~FactoryMaker() { Dispose(false); } ////// Dispose of any resources /// public void Dispose() { Dispose(true); } ////// Critical - performs an elevation to call MILUnknown.ReleaseInterface. /// TreatAsSafe - this function elevates to call release ( on an object we own). /// net effect is a shutdown of the FactoryMaker. Considered safe. /// /// Note that for now there is no SUC on ReleaseInterface. We may want to re-consider this. /// [SecurityCritical, SecurityTreatAsSafe] protected virtual void Dispose(bool fDisposing) { if (!_disposed) { if (_fValidObject == true) { lock (s_factoryMakerLock) { s_cInstance--; // Make sure we don't dispose twice _fValidObject = false; // If there is no FactoryMaker object out there, release // factory object if (s_cInstance == 0) { new SecurityPermission(SecurityPermissionFlag.UnmanagedCode).Assert(); // BlessedAssert: try { UnsafeNativeMethods.MILUnknown.ReleaseInterface(ref s_pFactory); if (s_pImagingFactory != IntPtr.Zero) { UnsafeNativeMethods.MILUnknown.ReleaseInterface(ref s_pImagingFactory); } } finally { CodeAccessPermission.RevertAssert(); s_pFactory = IntPtr.Zero; s_pImagingFactory = IntPtr.Zero; } } } } // Set the sentinel. _disposed = true; // Suppress finalization of this disposed instance. if (fDisposing) { GC.SuppressFinalize(this); } } } ////// Critical - returns critical resource created under an assert /// internal IntPtr FactoryPtr { [SecurityCritical] get { Debug.Assert(s_pFactory != IntPtr.Zero); return s_pFactory; } } ////// Critical - calls unmanaged code, returns unmanaged pointer /// internal IntPtr ImagingFactoryPtr { [SecurityCritical] get { if (s_pImagingFactory == IntPtr.Zero) { lock (s_factoryMakerLock) { HRESULT.Check(UnsafeNativeMethods.WICCodec.CreateImagingFactory(UnsafeNativeMethods.WICCodec.WINCODEC_SDK_VERSION, out s_pImagingFactory)); } } Debug.Assert(s_pImagingFactory != IntPtr.Zero); return s_pImagingFactory; } } ////// Critical - this is a pointer to an unmanaged object that methods are called directly on /// [SecurityCritical] private static IntPtr s_pFactory; ////// Critical - this is a pointer to an unmanaged object that methods are called directly on /// [SecurityCritical] private static IntPtr s_pImagingFactory; ////// Keeps track of how many instance of current object have been passed out /// private static int s_cInstance = 0; ////// "FactoryMaker" is free threaded. This lock is used to synchronize /// access to the FactoryMaker. /// private static object s_factoryMakerLock = new object(); private bool _fValidObject; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2003 // // File: FactoryMaker.cs // //----------------------------------------------------------------------------- using System; using System.Security; using System.Security.Permissions; using System.Diagnostics; using MS.Internal; using MS.Win32; using System.Windows.Media.Composition; using Microsoft.Internal; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using UnsafeNativeMethods=MS.Win32.PresentationCore.UnsafeNativeMethods; namespace System.Windows.Media { ////// This is a internal class which is used by non context affinity objects /// to get access to a MIL factory object. /// internal class FactoryMaker: IDisposable { private bool _disposed = false; ////// Critical - Create unmanaged critical resource /// [SecurityCritical] internal FactoryMaker() { lock (s_factoryMakerLock) { // If we haven't have a factory, create one if (s_pFactory == IntPtr.Zero) { // Create the Core MIL factory. // Note: the call below might throw exception. The caller // should catch it. We won't add ref counter here if this // happens. HRESULT.Check(UnsafeNativeMethods.MILFactory2.CreateFactory(out s_pFactory, MS.Internal.Composition.Version.MilSdkVersion)); } s_cInstance++; _fValidObject = true; } } ~FactoryMaker() { Dispose(false); } ////// Dispose of any resources /// public void Dispose() { Dispose(true); } ////// Critical - performs an elevation to call MILUnknown.ReleaseInterface. /// TreatAsSafe - this function elevates to call release ( on an object we own). /// net effect is a shutdown of the FactoryMaker. Considered safe. /// /// Note that for now there is no SUC on ReleaseInterface. We may want to re-consider this. /// [SecurityCritical, SecurityTreatAsSafe] protected virtual void Dispose(bool fDisposing) { if (!_disposed) { if (_fValidObject == true) { lock (s_factoryMakerLock) { s_cInstance--; // Make sure we don't dispose twice _fValidObject = false; // If there is no FactoryMaker object out there, release // factory object if (s_cInstance == 0) { new SecurityPermission(SecurityPermissionFlag.UnmanagedCode).Assert(); // BlessedAssert: try { UnsafeNativeMethods.MILUnknown.ReleaseInterface(ref s_pFactory); if (s_pImagingFactory != IntPtr.Zero) { UnsafeNativeMethods.MILUnknown.ReleaseInterface(ref s_pImagingFactory); } } finally { CodeAccessPermission.RevertAssert(); s_pFactory = IntPtr.Zero; s_pImagingFactory = IntPtr.Zero; } } } } // Set the sentinel. _disposed = true; // Suppress finalization of this disposed instance. if (fDisposing) { GC.SuppressFinalize(this); } } } ////// Critical - returns critical resource created under an assert /// internal IntPtr FactoryPtr { [SecurityCritical] get { Debug.Assert(s_pFactory != IntPtr.Zero); return s_pFactory; } } ////// Critical - calls unmanaged code, returns unmanaged pointer /// internal IntPtr ImagingFactoryPtr { [SecurityCritical] get { if (s_pImagingFactory == IntPtr.Zero) { lock (s_factoryMakerLock) { HRESULT.Check(UnsafeNativeMethods.WICCodec.CreateImagingFactory(UnsafeNativeMethods.WICCodec.WINCODEC_SDK_VERSION, out s_pImagingFactory)); } } Debug.Assert(s_pImagingFactory != IntPtr.Zero); return s_pImagingFactory; } } ////// Critical - this is a pointer to an unmanaged object that methods are called directly on /// [SecurityCritical] private static IntPtr s_pFactory; ////// Critical - this is a pointer to an unmanaged object that methods are called directly on /// [SecurityCritical] private static IntPtr s_pImagingFactory; ////// Keeps track of how many instance of current object have been passed out /// private static int s_cInstance = 0; ////// "FactoryMaker" is free threaded. This lock is used to synchronize /// access to the FactoryMaker. /// private static object s_factoryMakerLock = new object(); private bool _fValidObject; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
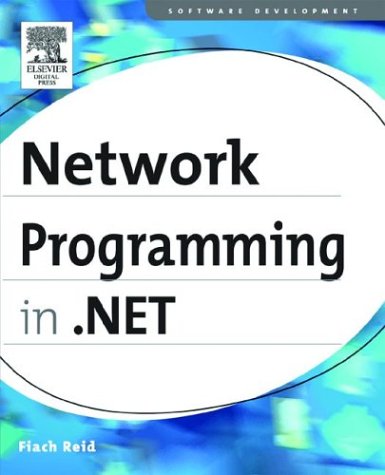
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DocumentViewer.cs
- AuthorizationRule.cs
- FixedPageProcessor.cs
- SpeechSeg.cs
- PropertyInformationCollection.cs
- KeyboardDevice.cs
- BitmapDownload.cs
- TreeChangeInfo.cs
- Literal.cs
- SafeSecurityHelper.cs
- HtmlInputButton.cs
- ConfigurationElementProperty.cs
- InternalBufferOverflowException.cs
- FormViewUpdatedEventArgs.cs
- PageThemeParser.cs
- HttpCookieCollection.cs
- IteratorFilter.cs
- CellTreeNodeVisitors.cs
- ServicePointManagerElement.cs
- XmlSerializableWriter.cs
- URLAttribute.cs
- RuleDefinitions.cs
- Operand.cs
- CompressionTransform.cs
- EventLogPermissionEntry.cs
- InputElement.cs
- CalendarDataBindingHandler.cs
- HtmlControl.cs
- RelationshipNavigation.cs
- InvalidFilterCriteriaException.cs
- XmlTextAttribute.cs
- ApplicationInfo.cs
- WpfWebRequestHelper.cs
- CompositeFontParser.cs
- VirtualizingStackPanel.cs
- WebPartHelpVerb.cs
- DetailsViewModeEventArgs.cs
- _UriTypeConverter.cs
- HtmlElementErrorEventArgs.cs
- RenamedEventArgs.cs
- HtmlInputImage.cs
- LineServices.cs
- SystemIcmpV6Statistics.cs
- NameTable.cs
- HttpContextServiceHost.cs
- OLEDB_Util.cs
- SQLChars.cs
- MatrixAnimationBase.cs
- PropertyTab.cs
- EnumType.cs
- XmlSchemaProviderAttribute.cs
- SessionState.cs
- PostBackOptions.cs
- QueryOperatorEnumerator.cs
- Overlapped.cs
- XmlSchemaSimpleTypeList.cs
- CodeStatement.cs
- WindowsGrip.cs
- InheritanceContextHelper.cs
- TypeExtensionConverter.cs
- ViewStateException.cs
- TextFormatterHost.cs
- MsdtcWrapper.cs
- BuildResult.cs
- MutexSecurity.cs
- ObjectDataSourceEventArgs.cs
- TextUtf8RawTextWriter.cs
- AppDomainCompilerProxy.cs
- xmlglyphRunInfo.cs
- FileRecordSequenceHelper.cs
- OleDbConnectionFactory.cs
- ClientSettingsProvider.cs
- DataGridHeaderBorder.cs
- ListControl.cs
- RectangleGeometry.cs
- WindowsFont.cs
- SrgsSemanticInterpretationTag.cs
- LocalIdKeyIdentifierClause.cs
- VersionPair.cs
- ObjectContext.cs
- HttpModuleActionCollection.cs
- CheckPair.cs
- rsa.cs
- coordinatorscratchpad.cs
- TableLayoutSettingsTypeConverter.cs
- WebPartChrome.cs
- OleServicesContext.cs
- Symbol.cs
- NameScopePropertyAttribute.cs
- ResourcePart.cs
- CategoryNameCollection.cs
- ToolStripPanelSelectionBehavior.cs
- DBSchemaTable.cs
- SemanticTag.cs
- TextSchema.cs
- XamlInt32CollectionSerializer.cs
- SortQuery.cs
- DocumentsTrace.cs
- ImageListUtils.cs
- MachineSettingsSection.cs