Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Internal / Synthesis / SpeechSeg.cs / 1 / SpeechSeg.cs
//------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // // // Contains either a reference to an audio audioStream or a list of // text fragments. // // History: // 2/1/2005 jeanfp Created from the Sapi Managed code //----------------------------------------------------------------- using System; using System.Collections.Generic; using System.Speech.Synthesis.TtsEngine; #pragma warning disable 1634, 1691 // Allows suppression of certain PreSharp messages. namespace System.Speech.Internal.Synthesis { ////// /// internal class SpeechSeg { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors internal SpeechSeg (TTSVoice voice, AudioData audio) { _voice = voice; _audio = audio; } #endregion //******************************************************************** // // Internal Properties // //******************************************************************* #region Internal Properties internal ListFragmentList { get { return _textFragments; } } internal AudioData Audio { get { return _audio; } } internal TTSVoice Voice { get { return _voice; } } internal bool IsText { get { return _audio == null; } } #if SPEECHSERVER || PROMPT_ENGINE internal bool ContainsPrompEngineFragment { set { _hasPromptEngineFragment = value; } get { return _hasPromptEngineFragment; } } #endif #endregion //******************************************************************** // // Internal Methods // //******************************************************************** #region Internal Methods internal void AddFrag (TextFragment textFragment) { if (_audio != null) { throw new InvalidOperationException (); } _textFragments.Add (textFragment); } #endregion //******************************************************************* // // Private Fields // //******************************************************************** #region private Fields private TTSVoice _voice; List _textFragments = new List (); #pragma warning disable 56524 // The _audio are not created in this module and should not be disposed private AudioData _audio; #pragma warning enable 56524 #if SPEECHSERVER || PROMPT_ENGINE // Reference to the VoiceSynthesizer that created it private bool _hasPromptEngineFragment; #endif #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // // // Contains either a reference to an audio audioStream or a list of // text fragments. // // History: // 2/1/2005 jeanfp Created from the Sapi Managed code //----------------------------------------------------------------- using System; using System.Collections.Generic; using System.Speech.Synthesis.TtsEngine; #pragma warning disable 1634, 1691 // Allows suppression of certain PreSharp messages. namespace System.Speech.Internal.Synthesis { ////// /// internal class SpeechSeg { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors internal SpeechSeg (TTSVoice voice, AudioData audio) { _voice = voice; _audio = audio; } #endregion //******************************************************************** // // Internal Properties // //******************************************************************* #region Internal Properties internal ListFragmentList { get { return _textFragments; } } internal AudioData Audio { get { return _audio; } } internal TTSVoice Voice { get { return _voice; } } internal bool IsText { get { return _audio == null; } } #if SPEECHSERVER || PROMPT_ENGINE internal bool ContainsPrompEngineFragment { set { _hasPromptEngineFragment = value; } get { return _hasPromptEngineFragment; } } #endif #endregion //******************************************************************** // // Internal Methods // //******************************************************************** #region Internal Methods internal void AddFrag (TextFragment textFragment) { if (_audio != null) { throw new InvalidOperationException (); } _textFragments.Add (textFragment); } #endregion //******************************************************************* // // Private Fields // //******************************************************************** #region private Fields private TTSVoice _voice; List _textFragments = new List (); #pragma warning disable 56524 // The _audio are not created in this module and should not be disposed private AudioData _audio; #pragma warning enable 56524 #if SPEECHSERVER || PROMPT_ENGINE // Reference to the VoiceSynthesizer that created it private bool _hasPromptEngineFragment; #endif #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
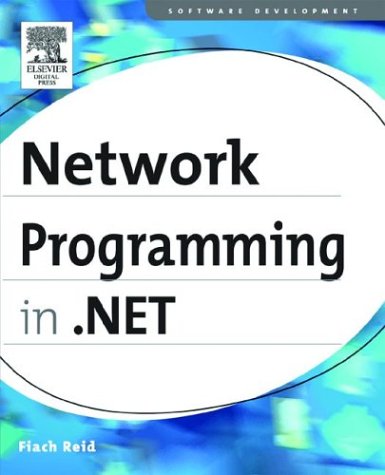
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FileEnumerator.cs
- DataListCommandEventArgs.cs
- WebBrowserPermission.cs
- Util.cs
- SessionStateContainer.cs
- DataServiceClientException.cs
- SqlDataSourceSelectingEventArgs.cs
- PassportAuthenticationEventArgs.cs
- SecureUICommand.cs
- EventLogRecord.cs
- PrintEvent.cs
- LinkGrep.cs
- TriggerCollection.cs
- DateTimeSerializationSection.cs
- CodeDOMProvider.cs
- FixedHyperLink.cs
- StringKeyFrameCollection.cs
- ColorIndependentAnimationStorage.cs
- AutomationPatternInfo.cs
- WFItemsToSpacerVisibility.cs
- ApplicationDirectory.cs
- DesignerActionItem.cs
- FullTextLine.cs
- Form.cs
- Win32MouseDevice.cs
- FacetChecker.cs
- CheckedPointers.cs
- DataGridAddNewRow.cs
- RuleSetCollection.cs
- KeyboardDevice.cs
- HandleCollector.cs
- UInt32Converter.cs
- TypeSource.cs
- TableColumnCollectionInternal.cs
- SizeIndependentAnimationStorage.cs
- ContractListAdapter.cs
- SqlCrossApplyToCrossJoin.cs
- StorageBasedPackageProperties.cs
- ValidationContext.cs
- CharEntityEncoderFallback.cs
- ExternalCalls.cs
- ThreadAttributes.cs
- CharacterString.cs
- ManipulationLogic.cs
- LinqDataSourceContextEventArgs.cs
- HtmlFormParameterWriter.cs
- InProcStateClientManager.cs
- Speller.cs
- RoutedCommand.cs
- Selection.cs
- CharConverter.cs
- TextDocumentView.cs
- WindowsAuthenticationEventArgs.cs
- ReliableSessionBindingElement.cs
- Bidi.cs
- SiteMapNodeItemEventArgs.cs
- APCustomTypeDescriptor.cs
- XmlMessageFormatter.cs
- OrCondition.cs
- MexNamedPipeBindingCollectionElement.cs
- Vector3DKeyFrameCollection.cs
- CharacterBuffer.cs
- NamedPermissionSet.cs
- SoapIgnoreAttribute.cs
- DataPointer.cs
- RequiredFieldValidator.cs
- WebServiceFault.cs
- RequestChannel.cs
- altserialization.cs
- DesignTimeResourceProviderFactoryAttribute.cs
- StaticTextPointer.cs
- EndpointInfo.cs
- cookiecontainer.cs
- SmiRecordBuffer.cs
- DataSysAttribute.cs
- OutputWindow.cs
- userdatakeys.cs
- PeerEndPoint.cs
- NameValueConfigurationElement.cs
- TrackingLocation.cs
- ControlPropertyNameConverter.cs
- TreePrinter.cs
- AssemblyBuilder.cs
- BatchServiceHost.cs
- Image.cs
- Page.cs
- XmlResolver.cs
- AspCompat.cs
- BaseTreeIterator.cs
- SoapAttributes.cs
- TileBrush.cs
- WorkflowDesignerColors.cs
- _SslStream.cs
- FilterEventArgs.cs
- ReaderWriterLockWrapper.cs
- RelatedCurrencyManager.cs
- TextElementEnumerator.cs
- HttpDictionary.cs
- StrongNameKeyPair.cs
- SqlUtil.cs