Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Server / System / Data / Services / Providers / ResourceContainer.cs / 1305376 / ResourceContainer.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Contains information about a resource set. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Providers { using System; using System.Collections.Generic; using System.Diagnostics; using System.Reflection; ////// Structure to keep information about a resource set /// ////// Custom providers can choose to use it as is or derive from it /// in order to flow provider-specific data. /// [DebuggerDisplay("{Name}: {ResourceType}")] public class ResourceSet { #region Fields ///Reference to resource type that this resource set is a collection of private readonly ResourceType elementType; ///Name of the resource set. private readonly string name; ///Cached delegate to read an IQueryable from the context. private Func
Link Menu
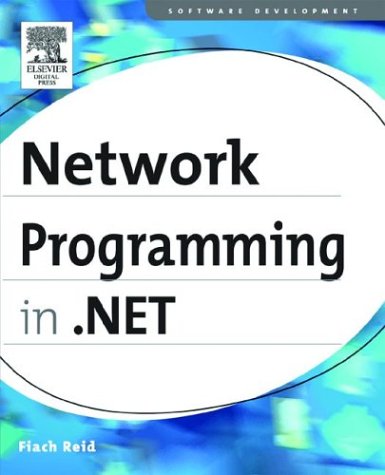
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- VectorValueSerializer.cs
- ListenDesigner.cs
- CustomErrorCollection.cs
- PlaceHolder.cs
- TextViewSelectionProcessor.cs
- UnitySerializationHolder.cs
- ExecutionTracker.cs
- ToolStripItem.cs
- GC.cs
- ToolStripPanelRow.cs
- SocketPermission.cs
- EditorPartDesigner.cs
- DynamicEndpointElement.cs
- DesignerDeviceConfig.cs
- MexBindingBindingCollectionElement.cs
- ProviderException.cs
- CounterNameConverter.cs
- SqlInternalConnection.cs
- DataGridViewCellCollection.cs
- ThreadAttributes.cs
- DataProtection.cs
- DesignerHelpers.cs
- ToolStripAdornerWindowService.cs
- streamingZipPartStream.cs
- NativeMethods.cs
- MenuTracker.cs
- HostingEnvironmentException.cs
- ServicePointManagerElement.cs
- DataRelationCollection.cs
- StylusTip.cs
- CommonDialog.cs
- CommandHelpers.cs
- CorePropertiesFilter.cs
- PauseStoryboard.cs
- ActivityExecutor.cs
- QueryableFilterRepeater.cs
- XmlQueryTypeFactory.cs
- DesignerVerbCollection.cs
- MLangCodePageEncoding.cs
- Timer.cs
- XmlSchemaImport.cs
- CheckBoxAutomationPeer.cs
- Classification.cs
- FixUpCollection.cs
- ResourceIDHelper.cs
- Compilation.cs
- MetadataCache.cs
- NameValueConfigurationCollection.cs
- SmtpNegotiateAuthenticationModule.cs
- ScriptServiceAttribute.cs
- BoundPropertyEntry.cs
- ColumnResizeUndoUnit.cs
- ServiceHttpModule.cs
- FramingDecoders.cs
- ListControl.cs
- ToolStripDropDownClosedEventArgs.cs
- Parser.cs
- SiteMembershipCondition.cs
- FixedTextContainer.cs
- Processor.cs
- DynamicILGenerator.cs
- WebPartZoneDesigner.cs
- PeerCustomResolverElement.cs
- InvalidPropValue.cs
- AffineTransform3D.cs
- EndPoint.cs
- PasswordDeriveBytes.cs
- BlurEffect.cs
- ComAwareEventInfo.cs
- CellRelation.cs
- StrongNameMembershipCondition.cs
- CrossAppDomainChannel.cs
- SafeRightsManagementSessionHandle.cs
- QueryParameter.cs
- RbTree.cs
- TextDecorationCollection.cs
- WindowsTokenRoleProvider.cs
- PolyQuadraticBezierSegment.cs
- TableLayoutPanelBehavior.cs
- TryExpression.cs
- PropertyInfo.cs
- Visual.cs
- TypeValidationEventArgs.cs
- DataObject.cs
- Operand.cs
- ConstantSlot.cs
- Window.cs
- AncestorChangedEventArgs.cs
- StringStorage.cs
- XPathExpr.cs
- HierarchicalDataSourceControl.cs
- SimpleMailWebEventProvider.cs
- PointLight.cs
- ProfilePropertySettings.cs
- GcSettings.cs
- MustUnderstandBehavior.cs
- GlyphInfoList.cs
- PriorityBindingExpression.cs
- Enlistment.cs
- XmlSchemaSimpleContent.cs