Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / MS / Internal / Shaping / GlyphInfoList.cs / 1 / GlyphInfoList.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: GlyphInfoList class // // History: // 09/24/2004: Garyyang: Moved GlyphInfoList class out from ShapingEngine.cs // into this seperate file // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Windows.Media.TextFormatting; using System.Security; using System.Security.Permissions; using MS.Internal; using MS.Internal.PresentationCore; namespace MS.Internal.Shaping { ////// Glyph info list /// ////// A bundle of several per-glyph result data of GetGlyphs API. /// All array members have the same number of elements. They /// grow and shrink at the same degree at the same time. /// internal class GlyphInfoList { internal GlyphInfoList(int capacity, int leap, bool justify) { _glyphs = new UshortList(capacity, leap); _glyphFlags = new UshortList(capacity, leap); _firstChars = new UshortList(capacity, leap); _ligatureCounts = new UshortList(capacity, leap); } ////// Length of the current run /// public int Length { get { return _glyphs.Length; } } ////// Offset of current sublist in storage /// internal int Offset { get { return _glyphs.Offset; } } ////// Validate glyph info length /// [Conditional("DEBUG")] internal void ValidateLength(int cch) { Debug.Assert(_glyphs.Offset + _glyphs.Length == cch, "Invalid glyph length!"); } ////// Limit range of accessing glyphinfo /// public void SetRange(int index, int length) { _glyphs.SetRange(index, length); _glyphFlags.SetRange(index, length); _firstChars.SetRange(index, length); _ligatureCounts.SetRange(index, length); } ////// Set glyph run length (use with care) /// ////// Critical - calls critical code /// [SecurityCritical] public void SetLength(int length) { _glyphs.Length = length; _glyphFlags.Length = length; _firstChars.Length = length; _ligatureCounts.Length = length; } public void Insert(int index, int Count) { _glyphs.Insert(index, Count); _glyphFlags.Insert(index, Count); _firstChars.Insert(index, Count); _ligatureCounts.Insert(index, Count); } public void Remove(int index, int Count) { _glyphs.Remove(index, Count); _glyphFlags.Remove(index, Count); _firstChars.Remove(index, Count); _ligatureCounts.Remove(index, Count); } public UshortList Glyphs { get { return _glyphs; } } public UshortList GlyphFlags { get { return _glyphFlags; } } public UshortList FirstChars { get { return _firstChars; } } public UshortList LigatureCounts { get { return _ligatureCounts; } } private UshortList _glyphs; private UshortList _glyphFlags; private UshortList _firstChars; private UshortList _ligatureCounts; } [Flags] internal enum GlyphFlags : ushort { /**** [Bit 0-7 OpenType flags] ****/ // Value 0-4 used. Value 5 - 15 Reserved Unassigned = 0x0000, Base = 0x0001, Ligature = 0x0002, Mark = 0x0003, Component = 0x0004, Unresolved = 0x0007, GlyphTypeMask = 0x0007, // Bit 4-5 used. Bit 7 Reserved Substituted = 0x0010, Positioned = 0x0020, NotChanged = 0x0000, // reserved for OTLS internal use // inside one call CursiveConnected = 0x0040, //bit 7 - reserved /**** [Bit 8-15 Avalon flags] ****/ ClusterStart = 0x0100, // First glyph of cluster Diacritic = 0x0200, // Diacritic ZeroWidth = 0x0400, // Blank, ZWJ, ZWNJ etc, with no width Missing = 0x0800, // Missing glyph InvalidBase = 0x1000, // Glyph of U+25cc indicating invalid base glyph } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: GlyphInfoList class // // History: // 09/24/2004: Garyyang: Moved GlyphInfoList class out from ShapingEngine.cs // into this seperate file // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Windows.Media.TextFormatting; using System.Security; using System.Security.Permissions; using MS.Internal; using MS.Internal.PresentationCore; namespace MS.Internal.Shaping { ////// Glyph info list /// ////// A bundle of several per-glyph result data of GetGlyphs API. /// All array members have the same number of elements. They /// grow and shrink at the same degree at the same time. /// internal class GlyphInfoList { internal GlyphInfoList(int capacity, int leap, bool justify) { _glyphs = new UshortList(capacity, leap); _glyphFlags = new UshortList(capacity, leap); _firstChars = new UshortList(capacity, leap); _ligatureCounts = new UshortList(capacity, leap); } ////// Length of the current run /// public int Length { get { return _glyphs.Length; } } ////// Offset of current sublist in storage /// internal int Offset { get { return _glyphs.Offset; } } ////// Validate glyph info length /// [Conditional("DEBUG")] internal void ValidateLength(int cch) { Debug.Assert(_glyphs.Offset + _glyphs.Length == cch, "Invalid glyph length!"); } ////// Limit range of accessing glyphinfo /// public void SetRange(int index, int length) { _glyphs.SetRange(index, length); _glyphFlags.SetRange(index, length); _firstChars.SetRange(index, length); _ligatureCounts.SetRange(index, length); } ////// Set glyph run length (use with care) /// ////// Critical - calls critical code /// [SecurityCritical] public void SetLength(int length) { _glyphs.Length = length; _glyphFlags.Length = length; _firstChars.Length = length; _ligatureCounts.Length = length; } public void Insert(int index, int Count) { _glyphs.Insert(index, Count); _glyphFlags.Insert(index, Count); _firstChars.Insert(index, Count); _ligatureCounts.Insert(index, Count); } public void Remove(int index, int Count) { _glyphs.Remove(index, Count); _glyphFlags.Remove(index, Count); _firstChars.Remove(index, Count); _ligatureCounts.Remove(index, Count); } public UshortList Glyphs { get { return _glyphs; } } public UshortList GlyphFlags { get { return _glyphFlags; } } public UshortList FirstChars { get { return _firstChars; } } public UshortList LigatureCounts { get { return _ligatureCounts; } } private UshortList _glyphs; private UshortList _glyphFlags; private UshortList _firstChars; private UshortList _ligatureCounts; } [Flags] internal enum GlyphFlags : ushort { /**** [Bit 0-7 OpenType flags] ****/ // Value 0-4 used. Value 5 - 15 Reserved Unassigned = 0x0000, Base = 0x0001, Ligature = 0x0002, Mark = 0x0003, Component = 0x0004, Unresolved = 0x0007, GlyphTypeMask = 0x0007, // Bit 4-5 used. Bit 7 Reserved Substituted = 0x0010, Positioned = 0x0020, NotChanged = 0x0000, // reserved for OTLS internal use // inside one call CursiveConnected = 0x0040, //bit 7 - reserved /**** [Bit 8-15 Avalon flags] ****/ ClusterStart = 0x0100, // First glyph of cluster Diacritic = 0x0200, // Diacritic ZeroWidth = 0x0400, // Blank, ZWJ, ZWNJ etc, with no width Missing = 0x0800, // Missing glyph InvalidBase = 0x1000, // Glyph of U+25cc indicating invalid base glyph } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
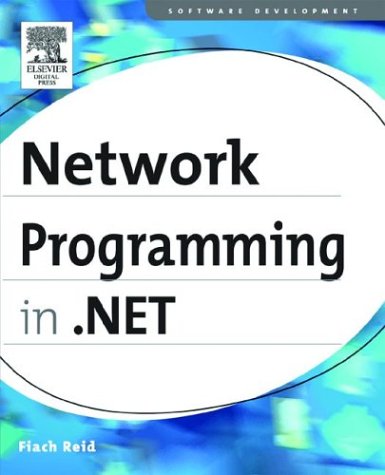
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlSchemaDatatype.cs
- EdmValidator.cs
- NativeWindow.cs
- DataGridSortingEventArgs.cs
- ContentElement.cs
- Rect3DConverter.cs
- TextStore.cs
- CodeGeneratorAttribute.cs
- ToolStripRendererSwitcher.cs
- UrlAuthorizationModule.cs
- CategoryAttribute.cs
- PasswordRecovery.cs
- Visual.cs
- ConfigXmlAttribute.cs
- ChannelPool.cs
- UInt16.cs
- GeneralTransform3D.cs
- RemoteX509Token.cs
- CodeAttributeArgumentCollection.cs
- Misc.cs
- ParameterCollection.cs
- RSAPKCS1KeyExchangeDeformatter.cs
- WpfSharedBamlSchemaContext.cs
- Root.cs
- IsolatedStorageFilePermission.cs
- TraceLog.cs
- MethodAccessException.cs
- ChannelManager.cs
- AddInAdapter.cs
- Oci.cs
- HttpRequestCacheValidator.cs
- XmlArrayItemAttributes.cs
- MetadataArtifactLoaderFile.cs
- ControlCommandSet.cs
- Span.cs
- FormViewInsertEventArgs.cs
- TextFragmentEngine.cs
- DrawingServices.cs
- IgnoreSection.cs
- RIPEMD160Managed.cs
- FixUpCollection.cs
- Point4DConverter.cs
- TypeDefinition.cs
- PenThread.cs
- ImageMetadata.cs
- SqlNamer.cs
- PartitionedStream.cs
- PermissionRequestEvidence.cs
- OdbcParameterCollection.cs
- ListDictionaryInternal.cs
- CellRelation.cs
- DelegateHelpers.cs
- PanelDesigner.cs
- PasswordBoxAutomationPeer.cs
- AppDomainCompilerProxy.cs
- InteropDesigner.xaml.cs
- InternalBufferOverflowException.cs
- XmlFormatExtensionPointAttribute.cs
- EmbeddedMailObjectsCollection.cs
- LinkArea.cs
- ConnectionPointCookie.cs
- RestHandler.cs
- XPathException.cs
- HitTestWithGeometryDrawingContextWalker.cs
- StaticSiteMapProvider.cs
- WebRequestModuleElementCollection.cs
- ProcessModuleCollection.cs
- IndexerNameAttribute.cs
- XmlSchemaComplexContent.cs
- BaseAddressElement.cs
- AnimationClock.cs
- XmlNodeChangedEventManager.cs
- Semaphore.cs
- BamlCollectionHolder.cs
- Image.cs
- RegexRunnerFactory.cs
- TextEditorThreadLocalStore.cs
- OperationBehaviorAttribute.cs
- TdsParserSafeHandles.cs
- SetStoryboardSpeedRatio.cs
- CompatibleIComparer.cs
- ExpressionBuilder.cs
- SqlInfoMessageEvent.cs
- XmlNamespaceDeclarationsAttribute.cs
- NativeMethods.cs
- RectangleHotSpot.cs
- VirtualizedCellInfoCollection.cs
- ConfigurationStrings.cs
- ContextMenu.cs
- XmlSchemaComplexType.cs
- ItemCollection.cs
- WebHttpSecurityElement.cs
- XmlRawWriterWrapper.cs
- IndependentAnimationStorage.cs
- FileDialog.cs
- MultipleCopiesCollection.cs
- AutomationElementCollection.cs
- StringDictionaryCodeDomSerializer.cs
- UiaCoreApi.cs
- VoiceSynthesis.cs