Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / Tools / System.Activities.Core.Presentation / System / Activities / Core / Presentation / InteropDesigner.xaml.cs / 1305376 / InteropDesigner.xaml.cs
//---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.Activities.Core.Presentation { using System.Activities.Statements; using System.ComponentModel; using System.Activities.Presentation.PropertyEditing; using System.Activities.Presentation; using System.Activities.Presentation.Metadata; using System.Windows.Threading; using System.Activities.Presentation.View; using System.IO; using System.Runtime; partial class InteropDesigner { static Funcfilter; public InteropDesigner() { this.InitializeComponent(); } public static Func Filter { get { if (InteropDesigner.filter == null) { // We will build tye Tyname for System.Workflow.ComponentModel.Activity string typeName = typeof(Activity).AssemblyQualifiedName; typeName = typeName.Replace("System.Activities", "System.Workflow.ComponentModel"); Type activityType = null; try { activityType = Type.GetType(typeName); } catch (System.Exception e) { if (Fx.IsFatal(e)) { throw; } activityType = null; } if (activityType != null) { //Interop.Body has to be a 3.5 Activity InteropDesigner.filter = (type) => activityType.IsAssignableFrom(type); } } return InteropDesigner.filter; } } protected override void OnModelItemChanged(object newItem) { base.OnModelItemChanged(newItem); this.ModelItem.PropertyChanged += new System.ComponentModel.PropertyChangedEventHandler(OnModelItemPropertyChanged); } void OnModelItemPropertyChanged(object sender, System.ComponentModel.PropertyChangedEventArgs e) { if (e.PropertyName == "ActivityType") { //Whenever ActivityType property changes, the activity will generate a new set of // dynamic properties. the property browser will not pick up the changes till // we select some other modelitem and then select this back. // modelItem.root is theone that will be always available. this.Dispatcher.BeginInvoke(DispatcherPriority.SystemIdle, (Action)(() => { Selection.SelectOnly(this.Context, this.ModelItem.Root); })); this.Dispatcher.BeginInvoke(DispatcherPriority.SystemIdle, (Action)(() => { Selection.SelectOnly(this.Context, this.ModelItem); })); } } public static void RegisterMetadataDelayed() { WorkflowViewService.AddDelayedDesignerRegistration("System.Activities.Statements.Interop", () => { AttributeTableBuilder builder = new AttributeTableBuilder(); string typeName = typeof(InteropDesigner).AssemblyQualifiedName; typeName = typeName.Replace("System.Activities.Core.Presentation.InteropDesigner", "System.Activities.Statements.Interop"); typeName = typeName.Replace("System.Activities.Core.Presentation", "System.Workflow.Runtime"); Type activityType; try { activityType = Type.GetType(typeName); } catch (System.Exception e) { if (Fx.IsFatal(e)) { throw; } activityType = null; } //activityType will be null in ClientSKU since System.Workflow.Runtime.dll is not a part of clientSKU. if (activityType != null) { builder.AddCustomAttributes(activityType, new DesignerAttribute(typeof(InteropDesigner))); builder.AddCustomAttributes( activityType, "ActivityType", new EditorOptionsAttribute { Name = TypePropertyEditor.BrowseTypeDirectly, Value = true }); builder.AddCustomAttributes( activityType, "ActivityType", new EditorOptionsAttribute { Name = TypePropertyEditor.Filter, Value = Filter }); builder.AddCustomAttributes( activityType, "ActivityType", new RefreshPropertiesAttribute(RefreshProperties.All)); builder.AddCustomAttributes(activityType, new ActivityDesignerOptionsAttribute { AllowDrillIn = false }); MetadataStore.AddAttributeTable(builder.CreateTable()); } }); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
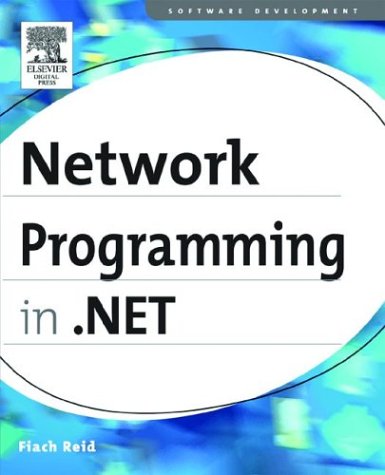
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RetrieveVirtualItemEventArgs.cs
- RTLAwareMessageBox.cs
- SourceElementsCollection.cs
- WindowsNonControl.cs
- EndOfStreamException.cs
- LinkConverter.cs
- LinqDataSourceValidationException.cs
- FormsAuthenticationUserCollection.cs
- InheritanceAttribute.cs
- OdbcCommand.cs
- SoapHeaderException.cs
- ContentDefinition.cs
- QilLiteral.cs
- AssemblyBuilderData.cs
- CompositeDataBoundControl.cs
- GraphicsContext.cs
- CompilerErrorCollection.cs
- MessageVersion.cs
- DistinctQueryOperator.cs
- Classification.cs
- TextRunCacheImp.cs
- ClientTargetCollection.cs
- AlignmentXValidation.cs
- RubberbandSelector.cs
- TextRange.cs
- VirtualizingPanel.cs
- Point3DCollection.cs
- SerializerWriterEventHandlers.cs
- GZipDecoder.cs
- WebDisplayNameAttribute.cs
- CodeBlockBuilder.cs
- SmtpFailedRecipientsException.cs
- DockAndAnchorLayout.cs
- WebServiceTypeData.cs
- VersionedStream.cs
- safex509handles.cs
- PipeException.cs
- ContourSegment.cs
- TemplateBindingExpressionConverter.cs
- XmlSchemaSimpleTypeRestriction.cs
- XmlQueryOutput.cs
- ScrollViewerAutomationPeer.cs
- TreeNodeStyleCollectionEditor.cs
- DuplexChannel.cs
- MemoryRecordBuffer.cs
- SQLDoubleStorage.cs
- Viewport3DVisual.cs
- DocumentAutomationPeer.cs
- TimeoutTimer.cs
- ZipIOModeEnforcingStream.cs
- Script.cs
- WebPartZoneCollection.cs
- EllipseGeometry.cs
- DependencyPropertyAttribute.cs
- CurrentChangingEventManager.cs
- OnOperation.cs
- BroadcastEventHelper.cs
- EvidenceBase.cs
- TypeResolver.cs
- ScriptRegistrationManager.cs
- ValidationRuleCollection.cs
- ResponseStream.cs
- Errors.cs
- GiveFeedbackEvent.cs
- CallbackValidatorAttribute.cs
- Menu.cs
- XhtmlBasicLinkAdapter.cs
- TreeViewItem.cs
- WindowsListViewItemStartMenu.cs
- UnmanagedHandle.cs
- SimpleTypeResolver.cs
- ToolBarButtonClickEvent.cs
- WebEvents.cs
- XmlDictionaryReader.cs
- WorkflowInstanceUnhandledExceptionRecord.cs
- TextServicesPropertyRanges.cs
- SafeNativeMethodsMilCoreApi.cs
- ManagedFilter.cs
- ItemMap.cs
- LinearGradientBrush.cs
- SmiGettersStream.cs
- MembershipPasswordException.cs
- SmtpClient.cs
- ListSortDescriptionCollection.cs
- SoapExtensionTypeElement.cs
- ToolStripOverflowButton.cs
- IntegerCollectionEditor.cs
- XmlTextAttribute.cs
- TextReturnReader.cs
- Translator.cs
- TextTabProperties.cs
- TraceSource.cs
- HttpErrorTraceRecord.cs
- FileDetails.cs
- TextTreePropertyUndoUnit.cs
- ComboBoxAutomationPeer.cs
- SqlCommand.cs
- HttpListenerResponse.cs
- Convert.cs
- ResourceReferenceExpressionConverter.cs