Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Client / System / Data / Services / Client / ALinq / NavigationPropertySingletonExpression.cs / 1305376 / NavigationPropertySingletonExpression.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Respresents a navigation to a singleton property. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Client { #region Private fields. using System; using System.Collections.Generic; using System.Diagnostics; using System.Linq; using System.Linq.Expressions; #endregion Private fields. ///Expression for a navigation property into a single entity (eg: Customer.BestFriend). internal class NavigationPropertySingletonExpression : ResourceExpression { #region Private fields. ///property member name private readonly Expression memberExpression; ///resource type private readonly Type resourceType; #endregion Private fields. ////// Creates a NavigationPropertySingletonExpression expression /// /// the return type of the expression /// the source expression /// property member name /// resource type for expression /// expand paths for resource set /// count option for the resource set /// custom query options for resourcse set /// projection expression internal NavigationPropertySingletonExpression(Type type, Expression source, Expression memberExpression, Type resourceType, ListexpandPaths, CountOption countOption, Dictionary customQueryOptions, ProjectionQueryOptionExpression projection) : base(source, (ExpressionType)ResourceExpressionType.ResourceNavigationPropertySingleton, type, expandPaths, countOption, customQueryOptions, projection) { Debug.Assert(memberExpression != null, "memberExpression != null"); Debug.Assert(resourceType != null, "resourceType != null"); this.memberExpression = memberExpression; this.resourceType = resourceType; } /// /// Gets the member expression. /// internal MemberExpression MemberExpression { get { return (MemberExpression)this.memberExpression; } } ////// The resource type of the singe instance produced by this singleton navigation. /// internal override Type ResourceType { get { return this.resourceType; } } ////// Singleton navigation properties always produce at most 1 result /// internal override bool IsSingleton { get { return true; } } ////// Does Singleton navigation have query options. /// internal override bool HasQueryOptions { get { return this.ExpandPaths.Count > 0 || this.CountOption == CountOption.InlineAll || this.CustomQueryOptions.Count > 0 || this.Projection != null; } } ////// Cast changes the type of the ResourceExpression /// /// new type ///new NavigationPropertySingletonExpression internal override ResourceExpression CreateCloneWithNewType(Type type) { return new NavigationPropertySingletonExpression( type, this.source, this.MemberExpression, TypeSystem.GetElementType(type), this.ExpandPaths.ToList(), this.CountOption, this.CustomQueryOptions.ToDictionary(kvp => kvp.Key, kvp => kvp.Value), this.Projection); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
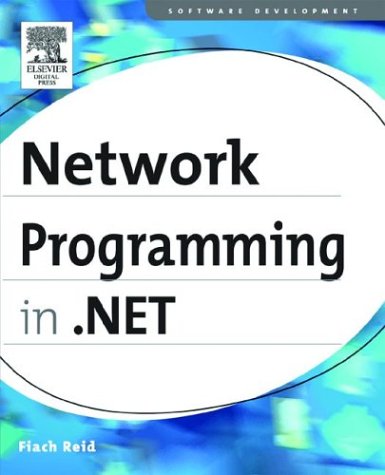
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- User.cs
- CommandID.cs
- Msec.cs
- MailWebEventProvider.cs
- CornerRadiusConverter.cs
- dbdatarecord.cs
- LinearGradientBrush.cs
- DataBindingHandlerAttribute.cs
- PageContentCollection.cs
- HtmlShimManager.cs
- Assert.cs
- SystemUnicastIPAddressInformation.cs
- CodeTypeDeclarationCollection.cs
- XmlDocumentSerializer.cs
- _NestedSingleAsyncResult.cs
- IriParsingElement.cs
- HttpConfigurationContext.cs
- XslTransformFileEditor.cs
- FileLevelControlBuilderAttribute.cs
- DrawTreeNodeEventArgs.cs
- SafeProcessHandle.cs
- CodeNamespaceImportCollection.cs
- BooleanAnimationUsingKeyFrames.cs
- PhysicalFontFamily.cs
- JsonXmlDataContract.cs
- ImpersonationContext.cs
- DefaultMemberAttribute.cs
- ImageIndexConverter.cs
- SuppressIldasmAttribute.cs
- Simplifier.cs
- ProfileManager.cs
- AssemblyNameProxy.cs
- InlinedAggregationOperatorEnumerator.cs
- Decorator.cs
- FamilyMapCollection.cs
- MatchingStyle.cs
- DoubleCollectionConverter.cs
- AppLevelCompilationSectionCache.cs
- ListMarkerLine.cs
- TemplateBindingExpressionConverter.cs
- MouseActionConverter.cs
- ScriptResourceAttribute.cs
- FontInfo.cs
- XmlNodeList.cs
- SystemColors.cs
- UserControl.cs
- InstanceDataCollectionCollection.cs
- ComponentChangingEvent.cs
- GenericUriParser.cs
- SingleSelectRootGridEntry.cs
- SiteMap.cs
- ManifestResourceInfo.cs
- LateBoundChannelParameterCollection.cs
- UrlPath.cs
- Pens.cs
- ItemChangedEventArgs.cs
- RawMouseInputReport.cs
- CodePageUtils.cs
- TokenBasedSetEnumerator.cs
- Schema.cs
- __FastResourceComparer.cs
- MediaEntryAttribute.cs
- Region.cs
- Int16Animation.cs
- TableSectionStyle.cs
- ScriptingSectionGroup.cs
- TdsParser.cs
- SecurityContextSecurityTokenParameters.cs
- XslTransform.cs
- CodeAttributeArgumentCollection.cs
- RewritingSimplifier.cs
- StatusBarAutomationPeer.cs
- VisemeEventArgs.cs
- RuntimeEnvironment.cs
- FixedSOMElement.cs
- SerializationInfoEnumerator.cs
- Win32SafeHandles.cs
- Wizard.cs
- ListViewPagedDataSource.cs
- NotImplementedException.cs
- ObjectSecurity.cs
- FileLoadException.cs
- ResourcesGenerator.cs
- CryptoStream.cs
- RuntimeArgumentHandle.cs
- TransformGroup.cs
- AssertUtility.cs
- TagMapCollection.cs
- CompModSwitches.cs
- ProcessThreadDesigner.cs
- NeutralResourcesLanguageAttribute.cs
- SpAudioStreamWrapper.cs
- BamlRecordHelper.cs
- SafeMILHandle.cs
- returneventsaver.cs
- sqlinternaltransaction.cs
- WebPartExportVerb.cs
- GeometryModel3D.cs
- ToolboxComponentsCreatedEventArgs.cs
- BindingMemberInfo.cs