Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DLinq / Dlinq / SqlClient / Query / SqlMethodTransformer.cs / 1 / SqlMethodTransformer.cs
using System; using System.Collections.Generic; using System.Text; using System.Data.Linq; namespace System.Data.Linq.SqlClient { ////// After retyping and conversions take place, some functions need to be changed into more suitable calls. /// Example: LEN -> DATALENGTH for long text types. /// internal class SqlMethodTransformer : SqlVisitor { protected SqlFactory sql; internal SqlMethodTransformer(SqlFactory sql) { this.sql = sql; } internal override SqlExpression VisitFunctionCall(SqlFunctionCall fc) { // process the arguments SqlExpression result = base.VisitFunctionCall(fc); if (result is SqlFunctionCall) { SqlFunctionCall resultFunctionCall = (SqlFunctionCall)result; if (resultFunctionCall.Name == "LEN") { SqlExpression expr = resultFunctionCall.Arguments[0]; if (expr.SqlType.IsLargeType && !expr.SqlType.SupportsLength) { result = sql.DATALENGTH(expr); if (expr.SqlType.IsUnicodeType) { result = sql.ConvertToInt(sql.Divide(result, sql.ValueFromObject(2, expr.SourceExpression))); } } } // If the return type of the sql function is not compatible with // the expected CLR type of the function, inject a conversion. This // step must be performed AFTER SqlRetyper has run. Type clrType = resultFunctionCall.SqlType.GetClosestRuntimeType(); bool skipConversion = SqlMethodTransformer.SkipConversionForDateAdd(resultFunctionCall.Name, resultFunctionCall.ClrType, clrType); if ((resultFunctionCall.ClrType != clrType) && !skipConversion) { result = sql.ConvertTo(resultFunctionCall.ClrType, resultFunctionCall); } } return result; } internal override SqlExpression VisitUnaryOperator(SqlUnary fc) { // process the arguments SqlExpression result = base.VisitUnaryOperator(fc); if (result is SqlUnary) { SqlUnary unary = (SqlUnary)result; switch (unary.NodeType) { case SqlNodeType.ClrLength: SqlExpression expr = unary.Operand; result = sql.DATALENGTH(expr); if (expr.SqlType.IsUnicodeType) { result = sql.Divide(result, sql.ValueFromObject(2, expr.SourceExpression)); } result = sql.ConvertToInt(result); break; default: break; } } return result; } // We don't inject a conversion for DATEADD if doing so will downgrade the result to // a less precise type. // private static bool SkipConversionForDateAdd(string functionName, Type expected, Type actual) { if (string.Compare(functionName, "DATEADD", StringComparison.OrdinalIgnoreCase) != 0) return false; return (expected == typeof(DateTime) && actual == typeof(DateTimeOffset)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Collections.Generic; using System.Text; using System.Data.Linq; namespace System.Data.Linq.SqlClient { ////// After retyping and conversions take place, some functions need to be changed into more suitable calls. /// Example: LEN -> DATALENGTH for long text types. /// internal class SqlMethodTransformer : SqlVisitor { protected SqlFactory sql; internal SqlMethodTransformer(SqlFactory sql) { this.sql = sql; } internal override SqlExpression VisitFunctionCall(SqlFunctionCall fc) { // process the arguments SqlExpression result = base.VisitFunctionCall(fc); if (result is SqlFunctionCall) { SqlFunctionCall resultFunctionCall = (SqlFunctionCall)result; if (resultFunctionCall.Name == "LEN") { SqlExpression expr = resultFunctionCall.Arguments[0]; if (expr.SqlType.IsLargeType && !expr.SqlType.SupportsLength) { result = sql.DATALENGTH(expr); if (expr.SqlType.IsUnicodeType) { result = sql.ConvertToInt(sql.Divide(result, sql.ValueFromObject(2, expr.SourceExpression))); } } } // If the return type of the sql function is not compatible with // the expected CLR type of the function, inject a conversion. This // step must be performed AFTER SqlRetyper has run. Type clrType = resultFunctionCall.SqlType.GetClosestRuntimeType(); bool skipConversion = SqlMethodTransformer.SkipConversionForDateAdd(resultFunctionCall.Name, resultFunctionCall.ClrType, clrType); if ((resultFunctionCall.ClrType != clrType) && !skipConversion) { result = sql.ConvertTo(resultFunctionCall.ClrType, resultFunctionCall); } } return result; } internal override SqlExpression VisitUnaryOperator(SqlUnary fc) { // process the arguments SqlExpression result = base.VisitUnaryOperator(fc); if (result is SqlUnary) { SqlUnary unary = (SqlUnary)result; switch (unary.NodeType) { case SqlNodeType.ClrLength: SqlExpression expr = unary.Operand; result = sql.DATALENGTH(expr); if (expr.SqlType.IsUnicodeType) { result = sql.Divide(result, sql.ValueFromObject(2, expr.SourceExpression)); } result = sql.ConvertToInt(result); break; default: break; } } return result; } // We don't inject a conversion for DATEADD if doing so will downgrade the result to // a less precise type. // private static bool SkipConversionForDateAdd(string functionName, Type expected, Type actual) { if (string.Compare(functionName, "DATEADD", StringComparison.OrdinalIgnoreCase) != 0) return false; return (expected == typeof(DateTime) && actual == typeof(DateTimeOffset)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
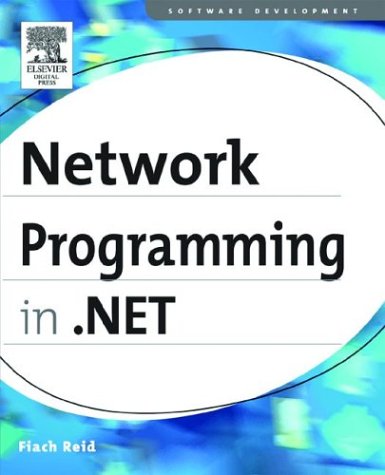
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DecimalMinMaxAggregationOperator.cs
- HttpStreamXmlDictionaryWriter.cs
- HostProtectionPermission.cs
- Dispatcher.cs
- DbConnectionPoolIdentity.cs
- ListComponentEditor.cs
- BitmapSizeOptions.cs
- HtmlContainerControl.cs
- Scene3D.cs
- HashMembershipCondition.cs
- EdmComplexTypeAttribute.cs
- System.Data_BID.cs
- IISMapPath.cs
- RegisteredExpandoAttribute.cs
- XmlWrappingReader.cs
- WorkflowInstance.cs
- PasswordDeriveBytes.cs
- SupportedAddressingMode.cs
- ComboBox.cs
- TextComposition.cs
- UnionCqlBlock.cs
- Vector3DCollectionValueSerializer.cs
- MonikerHelper.cs
- IdentityReference.cs
- SlipBehavior.cs
- WaitHandle.cs
- VisualTarget.cs
- Pool.cs
- KeyManager.cs
- CounterCreationData.cs
- DocumentGridContextMenu.cs
- DefaultWorkflowLoaderService.cs
- GridViewAutomationPeer.cs
- DataStreams.cs
- AlphaSortedEnumConverter.cs
- Evaluator.cs
- SelectionService.cs
- SqlErrorCollection.cs
- MaterialCollection.cs
- GiveFeedbackEvent.cs
- SettingsProviderCollection.cs
- Soap12FormatExtensions.cs
- XPathScanner.cs
- BasicViewGenerator.cs
- StoreItemCollection.cs
- XmlTypeMapping.cs
- FragmentQueryProcessor.cs
- CodeMemberEvent.cs
- DataSetUtil.cs
- DispatcherExceptionFilterEventArgs.cs
- TableStyle.cs
- NativeWindow.cs
- ContextStaticAttribute.cs
- AnimatedTypeHelpers.cs
- IdentityValidationException.cs
- SmiEventStream.cs
- TraceData.cs
- InkPresenterAutomationPeer.cs
- HttpVersion.cs
- __Error.cs
- JumpTask.cs
- RelatedView.cs
- _IPv6Address.cs
- SettingsBase.cs
- TextSelectionProcessor.cs
- Exceptions.cs
- Transform3DCollection.cs
- SetterBase.cs
- TabletDeviceInfo.cs
- IndicFontClient.cs
- EntityKey.cs
- QilExpression.cs
- StylusPointProperties.cs
- MatrixTransform.cs
- DiscriminatorMap.cs
- NativeActivityTransactionContext.cs
- InstalledFontCollection.cs
- DynamicDataRouteHandler.cs
- XmlReturnWriter.cs
- TreeNodeEventArgs.cs
- ContentValidator.cs
- CustomErrorsSectionWrapper.cs
- SystemParameters.cs
- XmlUrlResolver.cs
- SqlBulkCopyColumnMappingCollection.cs
- ObjectView.cs
- Pen.cs
- CodeDelegateCreateExpression.cs
- SpeechSeg.cs
- PropertyGeneratedEventArgs.cs
- BrowserDefinitionCollection.cs
- EmptyReadOnlyDictionaryInternal.cs
- FormatConvertedBitmap.cs
- HelpKeywordAttribute.cs
- AccessViolationException.cs
- DataControlCommands.cs
- SmiEventSink_DeferedProcessing.cs
- BuildResultCache.cs
- _TimerThread.cs
- FieldMetadata.cs