Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / XmlUtils / System / Xml / Xsl / QIL / QilExpression.cs / 5 / QilExpression.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System.Collections.Generic; using System.Xml.Xsl.Runtime; namespace System.Xml.Xsl.Qil { ////// The CQR implementation of QilExpression. /// ////// internal class QilExpression : QilNode { private QilFactory factory; private QilNode isDebug; private QilNode defWSet; private QilNode wsRules; private QilNode gloVars; private QilNode gloParams; private QilNode earlBnd; private QilNode funList; private QilNode rootNod; //----------------------------------------------- // Constructors //----------------------------------------------- ///QilExpression is the XML Query Intermediate Language invented by Michael Brundage and Chris Suver. /// QilExpression is an intermediate representation (IR) for all XML query and view languages. QilExpression is /// designed for optimization, composition with virtual XML views, translation into other forms, /// and direct execution. See also the QIL specification.
////// Construct QIL from a rooted graph of QilNodes with a new factory. /// public QilExpression(QilNodeType nodeType, QilNode root) : this(nodeType, root, new QilFactory()) { } ////// Construct QIL from a rooted graph of QilNodes with a specific factory. /// public QilExpression(QilNodeType nodeType, QilNode root, QilFactory factory) : base(nodeType) { this.factory = factory; this.isDebug = factory.False(); XmlWriterSettings settings = new XmlWriterSettings(); settings.ConformanceLevel = ConformanceLevel.Auto; this.defWSet = factory.LiteralObject(settings); this.wsRules = factory.LiteralObject(new List()); this.gloVars = factory.GlobalVariableList(); this.gloParams = factory.GlobalParameterList(); this.earlBnd = factory.LiteralObject(new List ()); this.funList = factory.FunctionList(); this.rootNod = root; } //----------------------------------------------- // IList methods -- override //----------------------------------------------- public override int Count { get { return 8; } } public override QilNode this[int index] { get { switch (index) { case 0: return this.isDebug; case 1: return this.defWSet; case 2: return this.wsRules; case 3: return this.gloParams; case 4: return this.gloVars; case 5: return this.earlBnd; case 6: return this.funList; case 7: return this.rootNod; default: throw new IndexOutOfRangeException(); } } set { switch (index) { case 0: this.isDebug = value; break; case 1: this.defWSet = value; break; case 2: this.wsRules = value; break; case 3: this.gloParams = value; break; case 4: this.gloVars = value; break; case 5: this.earlBnd = value; break; case 6: this.funList = value; break; case 7: this.rootNod = value; break; default: throw new IndexOutOfRangeException(); } } } //----------------------------------------------- // QilExpression methods //----------------------------------------------- /// /// QilFactory to be used in constructing nodes in this graph. /// public QilFactory Factory { get { return this.factory; } set { this.factory = value; } } ////// True if this expression contains debugging information. /// public bool IsDebug { get { return this.isDebug.NodeType == QilNodeType.True; } set { this.isDebug = value ? this.factory.True() : this.factory.False(); } } ////// Default serialization options that will be used if the user does not supply a writer at execution time. /// public XmlWriterSettings DefaultWriterSettings { get { return (XmlWriterSettings) ((QilLiteral) this.defWSet).Value; } set { value.ReadOnly = true; ((QilLiteral) this.defWSet).Value = value; } } ////// Xslt whitespace strip/preserve rules. /// public IListWhitespaceRules { get { return (IList ) ((QilLiteral) this.wsRules).Value; } set { ((QilLiteral) this.wsRules).Value = value; } } /// /// External parameters. /// public QilList GlobalParameterList { get { return (QilList) this.gloParams; } set { this.gloParams = value; } } ////// Global variables. /// public QilList GlobalVariableList { get { return (QilList) this.gloVars; } set { this.gloVars = value; } } ////// Early bound function objects. /// public IListEarlyBoundTypes { get { return (IList ) ((QilLiteral) this.earlBnd).Value; } set { ((QilLiteral) this.earlBnd).Value = value; } } /// /// Function definitions. /// public QilList FunctionList { get { return (QilList) this.funList; } set { this.funList = value; } } ////// The root node of the QilExpression graph /// public QilNode Root { get { return this.rootNod; } set { this.rootNod = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System.Collections.Generic; using System.Xml.Xsl.Runtime; namespace System.Xml.Xsl.Qil { ////// The CQR implementation of QilExpression. /// ////// internal class QilExpression : QilNode { private QilFactory factory; private QilNode isDebug; private QilNode defWSet; private QilNode wsRules; private QilNode gloVars; private QilNode gloParams; private QilNode earlBnd; private QilNode funList; private QilNode rootNod; //----------------------------------------------- // Constructors //----------------------------------------------- ///QilExpression is the XML Query Intermediate Language invented by Michael Brundage and Chris Suver. /// QilExpression is an intermediate representation (IR) for all XML query and view languages. QilExpression is /// designed for optimization, composition with virtual XML views, translation into other forms, /// and direct execution. See also the QIL specification.
////// Construct QIL from a rooted graph of QilNodes with a new factory. /// public QilExpression(QilNodeType nodeType, QilNode root) : this(nodeType, root, new QilFactory()) { } ////// Construct QIL from a rooted graph of QilNodes with a specific factory. /// public QilExpression(QilNodeType nodeType, QilNode root, QilFactory factory) : base(nodeType) { this.factory = factory; this.isDebug = factory.False(); XmlWriterSettings settings = new XmlWriterSettings(); settings.ConformanceLevel = ConformanceLevel.Auto; this.defWSet = factory.LiteralObject(settings); this.wsRules = factory.LiteralObject(new List()); this.gloVars = factory.GlobalVariableList(); this.gloParams = factory.GlobalParameterList(); this.earlBnd = factory.LiteralObject(new List ()); this.funList = factory.FunctionList(); this.rootNod = root; } //----------------------------------------------- // IList methods -- override //----------------------------------------------- public override int Count { get { return 8; } } public override QilNode this[int index] { get { switch (index) { case 0: return this.isDebug; case 1: return this.defWSet; case 2: return this.wsRules; case 3: return this.gloParams; case 4: return this.gloVars; case 5: return this.earlBnd; case 6: return this.funList; case 7: return this.rootNod; default: throw new IndexOutOfRangeException(); } } set { switch (index) { case 0: this.isDebug = value; break; case 1: this.defWSet = value; break; case 2: this.wsRules = value; break; case 3: this.gloParams = value; break; case 4: this.gloVars = value; break; case 5: this.earlBnd = value; break; case 6: this.funList = value; break; case 7: this.rootNod = value; break; default: throw new IndexOutOfRangeException(); } } } //----------------------------------------------- // QilExpression methods //----------------------------------------------- /// /// QilFactory to be used in constructing nodes in this graph. /// public QilFactory Factory { get { return this.factory; } set { this.factory = value; } } ////// True if this expression contains debugging information. /// public bool IsDebug { get { return this.isDebug.NodeType == QilNodeType.True; } set { this.isDebug = value ? this.factory.True() : this.factory.False(); } } ////// Default serialization options that will be used if the user does not supply a writer at execution time. /// public XmlWriterSettings DefaultWriterSettings { get { return (XmlWriterSettings) ((QilLiteral) this.defWSet).Value; } set { value.ReadOnly = true; ((QilLiteral) this.defWSet).Value = value; } } ////// Xslt whitespace strip/preserve rules. /// public IListWhitespaceRules { get { return (IList ) ((QilLiteral) this.wsRules).Value; } set { ((QilLiteral) this.wsRules).Value = value; } } /// /// External parameters. /// public QilList GlobalParameterList { get { return (QilList) this.gloParams; } set { this.gloParams = value; } } ////// Global variables. /// public QilList GlobalVariableList { get { return (QilList) this.gloVars; } set { this.gloVars = value; } } ////// Early bound function objects. /// public IListEarlyBoundTypes { get { return (IList ) ((QilLiteral) this.earlBnd).Value; } set { ((QilLiteral) this.earlBnd).Value = value; } } /// /// Function definitions. /// public QilList FunctionList { get { return (QilList) this.funList; } set { this.funList = value; } } ////// The root node of the QilExpression graph /// public QilNode Root { get { return this.rootNod; } set { this.rootNod = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
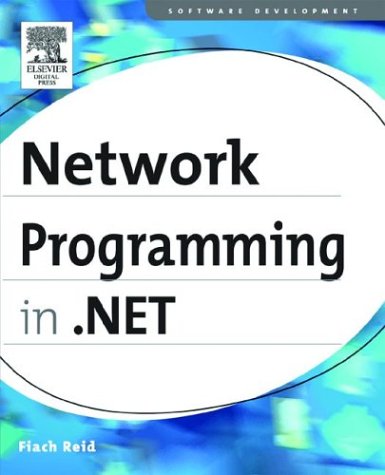
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataGridPagerStyle.cs
- AddInController.cs
- _ProxyRegBlob.cs
- LogWriteRestartAreaAsyncResult.cs
- DataGridViewCellPaintingEventArgs.cs
- ReadOnlyHierarchicalDataSourceView.cs
- XpsInterleavingPolicy.cs
- TextParaClient.cs
- EdmComplexPropertyAttribute.cs
- OrderedDictionary.cs
- PropertyGroupDescription.cs
- controlskin.cs
- SystemIcons.cs
- SecureStringHasher.cs
- ExceptionTranslationTable.cs
- XmlBoundElement.cs
- ISAPIApplicationHost.cs
- XmlSchemaObjectCollection.cs
- QueryContinueDragEvent.cs
- ToolStripSplitStackLayout.cs
- Point3DCollectionConverter.cs
- XmlIncludeAttribute.cs
- RecommendedAsConfigurableAttribute.cs
- SendingRequestEventArgs.cs
- MemberMaps.cs
- Array.cs
- ServicePointManager.cs
- KnownTypes.cs
- FileAuthorizationModule.cs
- FileLogRecordHeader.cs
- GifBitmapDecoder.cs
- NameValuePair.cs
- KeyValuePairs.cs
- StringArrayConverter.cs
- odbcmetadatacolumnnames.cs
- HttpDictionary.cs
- DataGridViewRowCollection.cs
- AuthenticationService.cs
- EncoderReplacementFallback.cs
- InvalidPrinterException.cs
- MatrixStack.cs
- __Filters.cs
- UrlMappingsModule.cs
- XmlReaderSettings.cs
- NameValueConfigurationElement.cs
- XamlReaderHelper.cs
- XmlEventCache.cs
- DesignTimeParseData.cs
- OdbcFactory.cs
- RTLAwareMessageBox.cs
- RowParagraph.cs
- OdbcInfoMessageEvent.cs
- Types.cs
- TransformedBitmap.cs
- ColumnWidthChangedEvent.cs
- TreeBuilderBamlTranslator.cs
- VisualBrush.cs
- PipelineModuleStepContainer.cs
- ComponentResourceManager.cs
- ToolboxCategoryItems.cs
- SubpageParaClient.cs
- EncryptedXml.cs
- JournalEntry.cs
- WebPartDeleteVerb.cs
- MulticastIPAddressInformationCollection.cs
- ServicePointManagerElement.cs
- MethodAccessException.cs
- InitializationEventAttribute.cs
- ServiceModelTimeSpanValidator.cs
- ArcSegment.cs
- RolePrincipal.cs
- Int32AnimationBase.cs
- EmptyQuery.cs
- DesignTimeValidationFeature.cs
- HtmlHead.cs
- SettingsProviderCollection.cs
- XPathAncestorQuery.cs
- ProcessThread.cs
- ReadOnlyCollection.cs
- CommonDialog.cs
- RadioButtonFlatAdapter.cs
- TransformedBitmap.cs
- WebServiceErrorEvent.cs
- GestureRecognitionResult.cs
- XamlTypeMapper.cs
- ZipFileInfoCollection.cs
- cryptoapiTransform.cs
- SqlEnums.cs
- XmlNodeChangedEventArgs.cs
- MarshalByValueComponent.cs
- UIElementHelper.cs
- baseaxisquery.cs
- Utils.cs
- GetChildSubtree.cs
- SafeMarshalContext.cs
- BitmapEffect.cs
- EventProperty.cs
- OleDbSchemaGuid.cs
- CacheRequest.cs
- tibetanshape.cs